利用Python统计词频的方法包括:使用collections.Counter、使用字典、使用正则表达式、使用NLTK库。其中,使用collections.Counter是最简单且高效的方法,下面将对其展开详细描述。
collections.Counter 是Python内置collections模块中的一个类,专门用于计数。它可以轻松地统计可迭代对象中各元素的频次。使用collections.Counter统计词频的步骤如下:
- 导入collections模块;
- 读取文本内容;
- 使用split方法将文本按空格分割成单词列表;
- 使用Counter统计每个单词出现的频次。
下面是一个具体的例子:
from collections import Counter
读取文本内容
text = "Python is great and Python is easy to learn. Python programming is fun."
将文本按空格分割成单词列表
words = text.split()
使用Counter统计每个单词出现的频次
word_count = Counter(words)
print(word_count)
这个代码会输出每个单词及其出现的次数。
一、使用collections.Counter
Collections模块是Python的一个内置模块,提供了许多有用的集合类和方法。其中,Counter类是一个非常有用的工具,可以用来轻松统计可迭代对象中元素的频次。以下是使用collections.Counter统计词频的具体步骤和示例代码。
1. 导入模块和读取文本
首先,我们需要导入collections模块并读取文本内容。可以通过文件读取或直接使用字符串。
from collections import Counter
读取文本内容
text = "Python is great and Python is easy to learn. Python programming is fun."
2. 分割文本
接下来,我们需要将文本分割成单词列表。可以使用split方法按空格分割。
# 将文本按空格分割成单词列表
words = text.split()
3. 统计词频
使用Counter类对单词列表进行统计,得到每个单词出现的频次。
# 使用Counter统计每个单词出现的频次
word_count = Counter(words)
print(word_count)
4. 完整示例
下面是完整的代码示例:
from collections import Counter
读取文本内容
text = "Python is great and Python is easy to learn. Python programming is fun."
将文本按空格分割成单词列表
words = text.split()
使用Counter统计每个单词出现的频次
word_count = Counter(words)
print(word_count)
输出结果将显示每个单词及其出现的次数,如:
Counter({'Python': 3, 'is': 3, 'great': 1, 'and': 1, 'easy': 1, 'to': 1, 'learn.': 1, 'programming': 1, 'fun.': 1})
二、使用字典统计词频
除了使用collections.Counter,我们还可以使用Python的字典(dictionary)来统计词频。这种方法虽然没有Counter简单,但也是一种常见的方法。以下是具体步骤和示例代码。
1. 导入模块和读取文本
首先,我们需要读取文本内容。
# 读取文本内容
text = "Python is great and Python is easy to learn. Python programming is fun."
2. 分割文本
将文本分割成单词列表。
# 将文本按空格分割成单词列表
words = text.split()
3. 统计词频
使用字典来统计每个单词出现的频次。
# 使用字典统计每个单词出现的频次
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
print(word_count)
4. 完整示例
下面是完整的代码示例:
# 读取文本内容
text = "Python is great and Python is easy to learn. Python programming is fun."
将文本按空格分割成单词列表
words = text.split()
使用字典统计每个单词出现的频次
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
print(word_count)
输出结果将显示每个单词及其出现的次数。
三、使用正则表达式
有时候文本中不仅有空格,还有标点符号等非单词字符。此时可以使用正则表达式将这些字符去掉,只保留单词。以下是具体步骤和示例代码。
1. 导入模块和读取文本
首先,我们需要导入re模块并读取文本内容。
import re
读取文本内容
text = "Python is great and Python is easy to learn. Python programming is fun."
2. 分割文本
使用正则表达式去除非单词字符,并将文本分割成单词列表。
# 使用正则表达式去除非单词字符
words = re.findall(r'\b\w+\b', text.lower())
3. 统计词频
使用Counter类对单词列表进行统计,得到每个单词出现的频次。
from collections import Counter
使用Counter统计每个单词出现的频次
word_count = Counter(words)
print(word_count)
4. 完整示例
下面是完整的代码示例:
import re
from collections import Counter
读取文本内容
text = "Python is great and Python is easy to learn. Python programming is fun."
使用正则表达式去除非单词字符
words = re.findall(r'\b\w+\b', text.lower())
使用Counter统计每个单词出现的频次
word_count = Counter(words)
print(word_count)
输出结果将显示每个单词及其出现的次数。
四、使用NLTK库
NLTK(Natural Language Toolkit)是一个强大的自然语言处理库。它提供了许多工具和方法来处理和分析文本数据。以下是使用NLTK库统计词频的具体步骤和示例代码。
1. 安装和导入NLTK库
首先,我们需要安装NLTK库并导入相关模块。
pip install nltk
import nltk
from nltk.tokenize import word_tokenize
from nltk.probability import FreqDist
下载必要的数据
nltk.download('punkt')
2. 读取文本和分割文本
读取文本内容,并使用NLTK的word_tokenize方法将文本分割成单词列表。
# 读取文本内容
text = "Python is great and Python is easy to learn. Python programming is fun."
使用word_tokenize方法将文本分割成单词列表
words = word_tokenize(text.lower())
3. 统计词频
使用FreqDist类对单词列表进行统计,得到每个单词出现的频次。
# 使用FreqDist统计每个单词出现的频次
word_count = FreqDist(words)
print(word_count)
4. 完整示例
下面是完整的代码示例:
import nltk
from nltk.tokenize import word_tokenize
from nltk.probability import FreqDist
下载必要的数据
nltk.download('punkt')
读取文本内容
text = "Python is great and Python is easy to learn. Python programming is fun."
使用word_tokenize方法将文本分割成单词列表
words = word_tokenize(text.lower())
使用FreqDist统计每个单词出现的频次
word_count = FreqDist(words)
print(word_count)
输出结果将显示每个单词及其出现的次数。
五、处理大文本文件
在处理大文本文件时,需要考虑内存和处理时间。可以使用生成器和逐行读取文件的方法来处理大文本文件。以下是具体步骤和示例代码。
1. 逐行读取文件
首先,我们需要逐行读取大文本文件的内容。
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
yield line
2. 统计词频
使用Counter类统计每行文本中的单词频次,并将结果累加。
from collections import Counter
import re
def count_words(file_path):
word_count = Counter()
for line in read_file(file_path):
words = re.findall(r'\b\w+\b', line.lower())
word_count.update(words)
return word_count
3. 完整示例
下面是完整的代码示例:
from collections import Counter
import re
定义逐行读取文件的函数
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
yield line
定义统计词频的函数
def count_words(file_path):
word_count = Counter()
for line in read_file(file_path):
words = re.findall(r'\b\w+\b', line.lower())
word_count.update(words)
return word_count
统计大文本文件的词频
file_path = 'large_text_file.txt'
word_count = count_words(file_path)
print(word_count)
这种方法可以有效处理大文本文件,避免内存溢出问题。
六、可视化词频
为了更直观地展示词频,可以使用matplotlib或seaborn库进行可视化。以下是具体步骤和示例代码。
1. 安装和导入相关库
首先,我们需要安装并导入matplotlib或seaborn库。
pip install matplotlib seaborn
import matplotlib.pyplot as plt
import seaborn as sns
2. 绘制词频图
使用统计结果绘制词频图。
# 读取文本内容
text = "Python is great and Python is easy to learn. Python programming is fun."
使用正则表达式去除非单词字符
words = re.findall(r'\b\w+\b', text.lower())
使用Counter统计每个单词出现的频次
word_count = Counter(words)
绘制词频图
plt.figure(figsize=(10, 6))
sns.barplot(x=list(word_count.keys()), y=list(word_count.values()))
plt.title('Word Frequency')
plt.xlabel('Words')
plt.ylabel('Frequency')
plt.xticks(rotation=45)
plt.show()
3. 完整示例
下面是完整的代码示例:
import re
from collections import Counter
import matplotlib.pyplot as plt
import seaborn as sns
读取文本内容
text = "Python is great and Python is easy to learn. Python programming is fun."
使用正则表达式去除非单词字符
words = re.findall(r'\b\w+\b', text.lower())
使用Counter统计每个单词出现的频次
word_count = Counter(words)
绘制词频图
plt.figure(figsize=(10, 6))
sns.barplot(x=list(word_count.keys()), y=list(word_count.values()))
plt.title('Word Frequency')
plt.xlabel('Words')
plt.ylabel('Frequency')
plt.xticks(rotation=45)
plt.show()
通过可视化,可以更直观地了解每个单词的出现频次。
七、总结
本文介绍了利用Python统计词频的多种方法,包括使用collections.Counter、使用字典、使用正则表达式、使用NLTK库、处理大文本文件以及可视化词频。这些方法各有优缺点,适用于不同的场景。
- 使用collections.Counter 是最简单且高效的方法,适用于大多数情况。
- 使用字典 方法灵活性高,但代码相对复杂。
- 使用正则表达式 可以处理含有标点符号的文本。
- 使用NLTK库 提供了更多的自然语言处理工具,适用于复杂文本分析。
- 处理大文本文件 需要考虑内存和处理时间,可以使用生成器和逐行读取的方法。
- 可视化词频 可以帮助更直观地展示统计结果。
通过掌握这些方法,可以根据具体需求选择合适的方式统计词频,并进行进一步的文本分析和处理。
相关问答FAQs:
如何用Python统计文本中的词频?
在Python中,可以使用内置的字符串处理和集合功能来统计词频。首先,读取文本数据,然后将其分割成单词,接着使用字典来记录每个单词出现的次数。下面是一个简单的代码示例:
from collections import Counter
# 读取文本
with open('text.txt', 'r', encoding='utf-8') as file:
text = file.read()
# 分词
words = text.split()
# 统计词频
word_counts = Counter(words)
# 输出结果
for word, count in word_counts.items():
print(f'{word}: {count}')
这种方法可以有效地统计出文本中每个单词的出现次数。
使用Python库进行词频统计有哪些推荐?
为了简化词频统计的过程,推荐使用一些流行的Python库,例如nltk
和collections
。nltk
提供了丰富的文本处理功能,包括分词、去停用词等,可以有效提高统计的准确性。而collections.Counter
非常适合直接统计单词频率,操作简单且效率高。
在统计词频时,有哪些常见的错误需要避免?
在进行词频统计时,常见的错误包括忽略大小写、未去除标点符号和停用词。确保将所有单词转换为小写,以避免同一单词因大小写不同而被重复统计。此外,使用正则表达式或文本处理库可以帮助去除标点符号,而通过停用词表可以排除一些常见但无意义的词汇,比如“的”、“是”等。
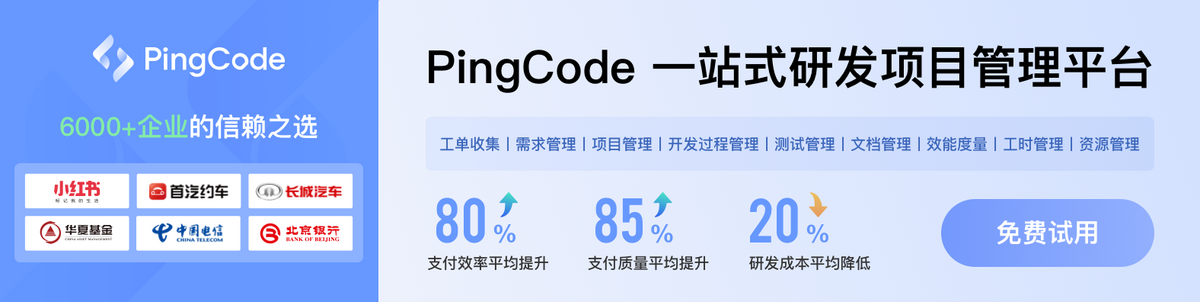