Python调用全部CPU的方法主要有:多线程、多进程、并发库、NumPy并行运算。本文将详细讲解如何使用这些方法来充分利用CPU资源,提高程序执行效率。
一、多线程
Python的多线程虽然受到GIL(全局解释器锁)的限制,但在I/O密集型任务中仍然可以显著提升性能。通过threading
模块,我们可以创建多个线程,分配不同的任务,使多个线程同时运行。
import threading
import time
def task():
print("Thread started")
time.sleep(2)
print("Thread finished")
threads = []
for i in range(4): # 假设有4个CPU核心
thread = threading.Thread(target=task)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
在上面的例子中,我们创建了4个线程,并让它们同时执行一个简单的任务。尽管Python的GIL会限制多线程的性能,但对于I/O密集型任务,多线程仍然是一个有效的解决方案。
二、多进程
对于CPU密集型任务,使用多进程来绕过GIL限制是更好的选择。Python的multiprocessing
模块允许我们创建多个进程,每个进程都有自己的Python解释器和GIL,从而可以充分利用多核CPU的性能。
import multiprocessing
import time
def task():
print(f"Process {multiprocessing.current_process().name} started")
time.sleep(2)
print(f"Process {multiprocessing.current_process().name} finished")
processes = []
for i in range(4): # 假设有4个CPU核心
process = multiprocessing.Process(target=task, name=f"Process-{i}")
processes.append(process)
process.start()
for process in processes:
process.join()
在这个例子中,我们创建了4个进程,每个进程都执行同样的任务。由于每个进程都有独立的GIL,这种方法可以充分利用多核CPU的性能。
三、并发库
Python的并发库如concurrent.futures
提供了更高级的接口,简化了多线程和多进程的使用。ThreadPoolExecutor
和ProcessPoolExecutor
分别用于管理线程池和进程池。
1. 使用ThreadPoolExecutor
from concurrent.futures import ThreadPoolExecutor
import time
def task():
print("Thread started")
time.sleep(2)
print("Thread finished")
with ThreadPoolExecutor(max_workers=4) as executor: # 假设有4个CPU核心
futures = [executor.submit(task) for _ in range(4)]
for future in futures:
future.result()
2. 使用ProcessPoolExecutor
from concurrent.futures import ProcessPoolExecutor
import time
def task():
print(f"Process {multiprocessing.current_process().name} started")
time.sleep(2)
print(f"Process {multiprocessing.current_process().name} finished")
with ProcessPoolExecutor(max_workers=4) as executor: # 假设有4个CPU核心
futures = [executor.submit(task) for _ in range(4)]
for future in futures:
future.result()
在这两个例子中,我们使用concurrent.futures
模块分别创建线程池和进程池,并提交多个任务到池中。ThreadPoolExecutor
适用于I/O密集型任务,而ProcessPoolExecutor
适用于CPU密集型任务。
四、NumPy并行运算
对于科学计算和数据处理,NumPy是一个非常强大的库。NumPy本身已经对一些操作进行了多线程优化,但我们也可以使用外部库如Numba
和Dask
来进一步提升性能。
1. 使用Numba加速NumPy运算
Numba是一个针对NumPy数组的JIT编译器,可以显著提升数值计算的性能。我们可以使用@njit
装饰器来加速函数,并使用parallel=True
选项来启用并行计算。
import numpy as np
from numba import njit, prange
@njit(parallel=True)
def parallel_sum(arr):
total = 0.0
for i in prange(arr.shape[0]):
total += arr[i]
return total
arr = np.random.rand(1000000)
result = parallel_sum(arr)
print(result)
在这个例子中,我们使用Numba的@njit
装饰器和prange
函数对一个简单的数组求和操作进行了并行化。这样可以充分利用多核CPU的性能。
2. 使用Dask进行并行计算
Dask是一个并行计算库,可以处理大规模的数据集,并支持分布式计算。我们可以使用Dask的数组和数据帧来并行处理大规模数据。
import dask.array as da
arr = da.random.random((10000, 10000), chunks=(1000, 1000))
result = arr.sum().compute()
print(result)
在这个例子中,我们使用Dask创建了一个大规模的随机数组,并对其求和。Dask会自动将计算任务分配到多个核心,充分利用CPU资源。
五、并行编程的注意事项
尽管并行编程可以显著提升性能,但也带来了一些复杂性和挑战。以下是一些常见的注意事项:
1. 数据竞争和锁
在多线程编程中,多个线程同时访问和修改共享数据时,可能会导致数据竞争问题。为了解决这个问题,我们可以使用锁来确保同一时间只有一个线程访问共享数据。
import threading
lock = threading.Lock()
shared_data = 0
def task():
global shared_data
with lock:
local_copy = shared_data
local_copy += 1
shared_data = local_copy
threads = [threading.Thread(target=task) for _ in range(100)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
print(shared_data)
在这个例子中,我们使用锁来确保只有一个线程可以访问和修改共享数据shared_data
,从而避免数据竞争问题。
2. 进程间通信
在多进程编程中,进程间的通信和数据共享是一个重要的问题。Python的multiprocessing
模块提供了多种进程间通信的机制,如队列、管道和共享内存。
import multiprocessing
def task(queue):
queue.put("Hello from process!")
queue = multiprocessing.Queue()
process = multiprocessing.Process(target=task, args=(queue,))
process.start()
process.join()
message = queue.get()
print(message)
在这个例子中,我们使用队列在进程间传递消息。队列是一个线程安全的数据结构,可以用于进程间的通信。
六、高性能计算库的使用
除了上述方法,Python还有许多高性能计算库,可以帮助我们充分利用CPU资源,如Cython
、PyOpenCL
和PyCUDA
等。
1. 使用Cython加速Python代码
Cython是一个将Python代码转换为C代码的工具,可以显著提升Python代码的执行效率。我们可以使用Cython对关键代码进行优化,从而提高性能。
# example.pyx
def sum_array(arr):
cdef int i, total = 0
for i in range(len(arr)):
total += arr[i]
return total
编译Cython代码:
cythonize -i example.pyx
在Python中使用编译后的Cython模块:
import example
arr = [i for i in range(1000000)]
result = example.sum_array(arr)
print(result)
在这个例子中,我们使用Cython将一个简单的数组求和操作编译为C代码,从而显著提升性能。
2. 使用PyOpenCL和PyCUDA进行GPU加速
对于需要大量计算的任务,我们可以使用GPU来加速。PyOpenCL和PyCUDA是两个常用的Python库,可以帮助我们利用GPU进行高性能计算。
import pyopencl as cl
import numpy as np
创建OpenCL上下文和队列
context = cl.create_some_context()
queue = cl.CommandQueue(context)
定义OpenCL内核
kernel_code = """
__kernel void sum_array(__global const float *a, __global const float *b, __global float *c) {
int gid = get_global_id(0);
c[gid] = a[gid] + b[gid];
}
"""
编译内核
program = cl.Program(context, kernel_code).build()
创建输入和输出缓冲区
a = np.random.rand(1000000).astype(np.float32)
b = np.random.rand(1000000).astype(np.float32)
c = np.empty_like(a)
a_buf = cl.Buffer(context, cl.mem_flags.READ_ONLY | cl.mem_flags.COPY_HOST_PTR, hostbuf=a)
b_buf = cl.Buffer(context, cl.mem_flags.READ_ONLY | cl.mem_flags.COPY_HOST_PTR, hostbuf=b)
c_buf = cl.Buffer(context, cl.mem_flags.WRITE_ONLY, c.nbytes)
执行内核
program.sum_array(queue, a.shape, None, a_buf, b_buf, c_buf)
读取结果
cl.enqueue_copy(queue, c, c_buf).wait()
print(c)
在这个例子中,我们使用PyOpenCL在GPU上执行一个简单的数组求和操作,从而显著提升计算性能。
七、总结
通过多线程、多进程、并发库、NumPy并行运算以及高性能计算库,我们可以充分利用CPU资源,提高Python程序的执行效率。虽然并行编程带来了一些复杂性和挑战,但通过合理的设计和优化,我们可以显著提升程序的性能,为数据处理、科学计算和机器学习等领域提供强有力的支持。
在实际应用中,我们需要根据具体的任务类型和需求选择合适的并行编程方法,并注意处理数据竞争、进程间通信等问题,以确保程序的正确性和高效性。通过不断学习和实践,我们可以更好地掌握并行编程技术,为Python程序的性能优化提供更多的可能性。
相关问答FAQs:
如何在Python中实现多线程以利用全部CPU?
在Python中,利用多线程可以在一定程度上提高程序的执行效率,尤其是I/O密集型的任务。然而,由于Python的全局解释器锁(GIL),多线程在CPU密集型任务上效果有限。为了充分利用多核CPU,推荐使用multiprocessing
模块,它可以创建多个进程,每个进程可以独立运行在不同的CPU核心上。
在Python中如何使用multiprocessing模块?
使用multiprocessing
模块非常简单。你需要导入该模块,并定义一个需要并行处理的函数。然后,可以通过Process
类创建多个进程,并使用start()
方法启动它们。示例代码如下:
import multiprocessing
def worker(num):
print(f'Worker: {num}')
if __name__ == '__main__':
processes = []
for i in range(multiprocessing.cpu_count()):
p = multiprocessing.Process(target=worker, args=(i,))
processes.append(p)
p.start()
for p in processes:
p.join()
这段代码将创建与CPU核心数相同的进程,并让每个进程执行worker
函数。
在Python中如何管理并发进程的资源?
管理并发进程的资源非常重要,尤其是在处理大量数据时。可以使用multiprocessing.Queue
或multiprocessing.Pool
来高效管理进程间的资源。Pool
类可以创建一个进程池,通过apply
或map
等方法分配任务,确保资源的有效利用。
如何监控Python程序的CPU使用情况?
在开发过程中,监控CPU使用情况可以帮助你评估程序性能。可以使用psutil
库来获取系统的CPU信息,包括当前的CPU使用率、每个核心的使用情况等。安装此库后,可以通过以下代码监控CPU使用情况:
import psutil
print(f'CPU使用率: {psutil.cpu_percent(interval=1)}%')
print(f'每个核心的使用率: {psutil.cpu_percent(interval=1, percpu=True)}')
使用这些工具和方法,你能够更好地在Python中调用并利用全部CPU。
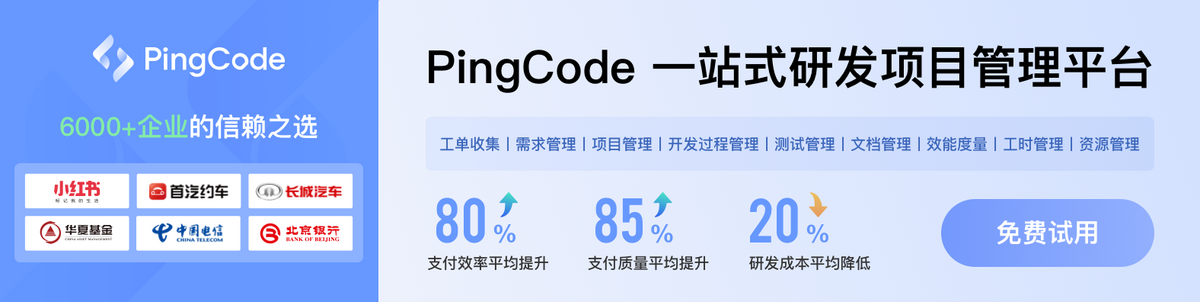