Python设置按钮位置可以使用Tkinter库、Grid布局管理器、Pack布局管理器、Place布局管理器。其中,Grid布局管理器使用网格系统来定位按钮,Pack布局管理器用于按照顺序排列按钮,而Place布局管理器则允许通过绝对位置精确定位按钮。Grid布局管理器是最灵活和常用的一种,它使用行和列的概念来布局控件。下面将详细介绍Grid布局管理器的使用方法。
一、Grid布局管理器
Grid布局管理器使用网格系统来定位按钮。每个按钮可以被放置在一个特定的行和列中,这使得布局更加灵活和可控。
1、基础使用方法
要使用Grid布局管理器,我们需要通过grid()
方法来指定按钮的位置。grid()
方法的参数包括row
和column
,分别表示按钮在网格中的行和列。
import tkinter as tk
root = tk.Tk()
root.title("Grid Layout Example")
button1 = tk.Button(root, text="Button 1")
button1.grid(row=0, column=0)
button2 = tk.Button(root, text="Button 2")
button2.grid(row=0, column=1)
button3 = tk.Button(root, text="Button 3")
button3.grid(row=1, column=0, columnspan=2)
root.mainloop()
在上述示例中,button1
被放置在第0行第0列,button2
被放置在第0行第1列,button3
被放置在第1行并跨越两列。
2、调整控件对齐方式
可以通过sticky
参数来调整控件在单元格中的对齐方式。sticky
参数接受四个方向的组合:n
(北)、s
(南)、e
(东)和w
(西)。
button1.grid(row=0, column=0, sticky="w")
button2.grid(row=0, column=1, sticky="e")
在这个示例中,button1
被对齐到单元格的左侧,而button2
被对齐到单元格的右侧。
3、调整控件的大小
可以通过padx
和pady
参数来调整控件的外部填充(即控件与单元格边缘之间的距离),以及通过ipadx
和ipady
参数来调整控件的内部填充(即控件内部内容与控件边框之间的距离)。
button1.grid(row=0, column=0, padx=10, pady=10)
button2.grid(row=0, column=1, ipadx=10, ipady=10)
在这个示例中,button1
的外部填充为10像素,而button2
的内部填充为10像素。
二、Pack布局管理器
Pack布局管理器是Tkinter中最简单的布局管理器之一,它按照顺序排列控件,并允许通过side
参数指定排列方向。
1、基础使用方法
要使用Pack布局管理器,我们需要通过pack()
方法来排列按钮。pack()
方法的参数包括side
,可以选择排列方向:top
(默认)、bottom
、left
或right
。
import tkinter as tk
root = tk.Tk()
root.title("Pack Layout Example")
button1 = tk.Button(root, text="Button 1")
button1.pack(side="top")
button2 = tk.Button(root, text="Button 2")
button2.pack(side="left")
button3 = tk.Button(root, text="Button 3")
button3.pack(side="right")
root.mainloop()
在上述示例中,button1
被排列在顶部,button2
被排列在左侧,而button3
被排列在右侧。
2、调整控件对齐方式
可以通过anchor
参数来调整控件在容器中的对齐方式。anchor
参数接受八个方向的组合:n
(北)、s
(南)、e
(东)、w
(西)、ne
(东北)、nw
(西北)、se
(东南)和sw
(西南)。
button1.pack(side="top", anchor="w")
button2.pack(side="left", anchor="n")
在这个示例中,button1
被对齐到容器的左侧,而button2
被对齐到容器的顶部。
3、调整控件的填充
可以通过fill
参数来调整控件的填充方式。fill
参数接受两个值:x
(横向填充)和y
(纵向填充)。
button1.pack(side="top", fill="x")
button2.pack(side="left", fill="y")
在这个示例中,button1
将横向填充整个容器,而button2
将纵向填充整个容器。
三、Place布局管理器
Place布局管理器允许通过绝对位置精确定位按钮。它使用x
和y
坐标来指定按钮的位置。
1、基础使用方法
要使用Place布局管理器,我们需要通过place()
方法来指定按钮的位置。place()
方法的参数包括x
和y
,分别表示按钮在容器中的横坐标和纵坐标。
import tkinter as tk
root = tk.Tk()
root.title("Place Layout Example")
button1 = tk.Button(root, text="Button 1")
button1.place(x=50, y=50)
button2 = tk.Button(root, text="Button 2")
button2.place(x=150, y=150)
root.mainloop()
在上述示例中,button1
被放置在坐标(50, 50)处,而button2
被放置在坐标(150, 150)处。
2、调整控件的大小
可以通过width
和height
参数来指定控件的宽度和高度。
button1.place(x=50, y=50, width=100, height=50)
在这个示例中,button1
的宽度被设置为100像素,高度被设置为50像素。
3、调整控件的对齐方式
可以通过anchor
参数来调整控件在指定位置的对齐方式。anchor
参数接受八个方向的组合:n
(北)、s
(南)、e
(东)、w
(西)、ne
(东北)、nw
(西北)、se
(东南)和sw
(西南)。
button1.place(x=50, y=50, anchor="center")
在这个示例中,button1
的中心被对齐到坐标(50, 50)处。
四、综合使用示例
为了更好地理解这三种布局管理器的使用方法,我们将通过一个综合示例来展示如何在一个Tkinter应用程序中同时使用Grid、Pack和Place布局管理器。
import tkinter as tk
root = tk.Tk()
root.title("Combined Layout Example")
使用Grid布局管理器
frame1 = tk.Frame(root, bg="lightblue")
frame1.grid(row=0, column=0, padx=10, pady=10)
button1 = tk.Button(frame1, text="Grid Button 1")
button1.grid(row=0, column=0)
button2 = tk.Button(frame1, text="Grid Button 2")
button2.grid(row=0, column=1)
使用Pack布局管理器
frame2 = tk.Frame(root, bg="lightgreen")
frame2.grid(row=0, column=1, padx=10, pady=10)
button3 = tk.Button(frame2, text="Pack Button 1")
button3.pack(side="top", fill="x")
button4 = tk.Button(frame2, text="Pack Button 2")
button4.pack(side="bottom", fill="x")
使用Place布局管理器
frame3 = tk.Frame(root, bg="lightyellow", width=200, height=200)
frame3.grid(row=1, column=0, columnspan=2, pady=10)
button5 = tk.Button(frame3, text="Place Button 1")
button5.place(x=50, y=50, anchor="center")
button6 = tk.Button(frame3, text="Place Button 2")
button6.place(x=150, y=150, anchor="center")
root.mainloop()
在这个综合示例中,我们创建了三个框架(frame),分别使用了Grid、Pack和Place布局管理器来排列按钮。frame1
使用Grid布局管理器,frame2
使用Pack布局管理器,frame3
使用Place布局管理器。
五、布局管理器的选择
在选择布局管理器时,需要考虑应用程序的具体需求和布局复杂度。
1、Grid布局管理器
适用于需要复杂布局的应用程序,例如表格、网格等。它提供了行和列的概念,使得布局更加灵活和可控。
2、Pack布局管理器
适用于简单的顺序布局,例如垂直或水平排列的控件。它易于使用,但不适合复杂布局。
3、Place布局管理器
适用于需要精确定位控件的应用程序,例如图形界面设计工具。它允许通过绝对位置精确定位控件,但布局的可维护性较差。
六、布局管理器的组合使用
在实际开发中,经常需要组合使用多个布局管理器来实现复杂布局。可以通过嵌套框架(frame)来实现这种组合使用。
import tkinter as tk
root = tk.Tk()
root.title("Mixed Layout Example")
顶部框架,使用Pack布局管理器
top_frame = tk.Frame(root, bg="lightgray")
top_frame.pack(side="top", fill="x")
左侧框架,使用Grid布局管理器
left_frame = tk.Frame(root, bg="lightblue")
left_frame.pack(side="left", fill="y")
button1 = tk.Button(left_frame, text="Grid Button 1")
button1.grid(row=0, column=0)
button2 = tk.Button(left_frame, text="Grid Button 2")
button2.grid(row=1, column=0)
右侧框架,使用Place布局管理器
right_frame = tk.Frame(root, bg="lightyellow", width=200, height=200)
right_frame.pack(side="right", fill="both", expand=True)
button3 = tk.Button(right_frame, text="Place Button 1")
button3.place(x=50, y=50, anchor="center")
button4 = tk.Button(right_frame, text="Place Button 2")
button4.place(x=150, y=150, anchor="center")
root.mainloop()
在这个示例中,我们创建了一个顶层框架top_frame
,使用Pack布局管理器将其放置在顶部。然后创建了两个子框架left_frame
和right_frame
,分别使用Grid和Place布局管理器来排列按钮。通过这种方式,可以实现更复杂和灵活的布局。
七、布局管理器的高级用法
在一些高级应用场景中,可能需要使用一些高级用法来实现特定的布局需求。例如,使用权重(weight)来调整行和列的比例,使用网格合并(grid_span)来合并多个单元格等。
1、使用权重调整行和列的比例
可以通过grid_rowconfigure
和grid_columnconfigure
方法来设置行和列的权重。权重用于确定行和列在调整窗口大小时的比例。
root = tk.Tk()
root.title("Grid Weight Example")
root.grid_rowconfigure(0, weight=1)
root.grid_columnconfigure(0, weight=1)
root.grid_columnconfigure(1, weight=2)
button1 = tk.Button(root, text="Button 1")
button1.grid(row=0, column=0, sticky="nsew")
button2 = tk.Button(root, text="Button 2")
button2.grid(row=0, column=1, sticky="nsew")
root.mainloop()
在这个示例中,root
窗口的第0行和第0、1列被设置了权重。第0列的权重为1,第1列的权重为2,这意味着在调整窗口大小时,第1列的扩展比例是第0列的两倍。
2、使用网格合并
可以通过rowspan
和columnspan
参数来合并多个单元格。
button3 = tk.Button(root, text="Button 3")
button3.grid(row=1, column=0, columnspan=2, sticky="nsew")
在这个示例中,button3
被放置在第1行并跨越两列。
八、布局管理器的最佳实践
在实际开发中,遵循一些最佳实践可以提高布局的可维护性和灵活性。
1、使用嵌套框架
通过使用嵌套框架,可以将复杂布局分解为多个简单布局,从而提高代码的可读性和可维护性。
2、合理使用填充和对齐
通过合理使用填充(padding)和对齐(alignment),可以使布局更加美观和一致。
3、使用布局管理器的组合
通过组合使用多个布局管理器,可以实现更复杂和灵活的布局。
4、避免使用绝对位置
尽量避免使用Place布局管理器的绝对位置,以提高布局的可维护性和适应性。
九、总结
在Python中,通过使用Tkinter库和布局管理器,可以实现各种复杂的按钮位置设置。Grid布局管理器是最灵活和常用的一种,适用于需要复杂布局的应用程序。Pack布局管理器适用于简单的顺序布局,而Place布局管理器适用于需要精确定位控件的应用程序。在实际开发中,可以通过嵌套框架和组合使用多个布局管理器来实现更复杂和灵活的布局。通过遵循一些最佳实践,可以提高布局的可维护性和灵活性。
相关问答FAQs:
在Python中如何自定义按钮的大小和位置?
在Python中,可以使用Tkinter库来创建图形用户界面(GUI)并设置按钮的大小和位置。通过使用place()
、pack()
或grid()
方法,可以精确控制按钮的布局。place()
方法允许您通过设置x和y坐标来直接指定按钮的位置,同时可以通过设置width
和height
参数来定义按钮的大小。
使用Tkinter时,按钮位置的常见问题是什么?
在使用Tkinter创建按钮时,用户可能会遇到按钮重叠或布局不一致的问题。这通常是因为使用了不当的布局管理器。确保在同一容器中仅使用一种布局管理器,例如,如果使用pack()
,不要混合使用grid()
或place()
。此外,设置合适的边距和填充参数可以帮助改善按钮位置。
如何在按钮点击事件中使用坐标信息?
可以通过绑定按钮的点击事件来获取当前鼠标位置。在Tkinter中,您可以使用bind()
方法将事件与按钮关联,并在事件处理函数中使用event.x
和event.y
获取鼠标的坐标信息。这对于需要根据用户输入动态调整按钮位置的应用程序非常有用。
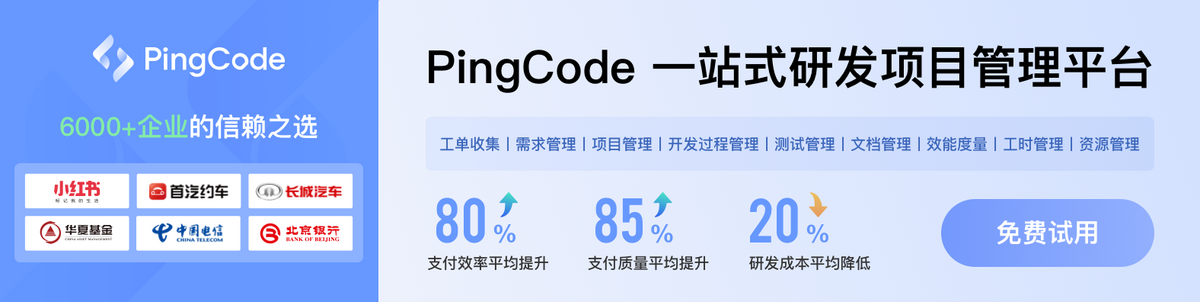