在Python中,替换操作通常可以通过字符串方法、正则表达式模块等实现。常用的方法有:使用str.replace()方法、使用re模块的sub()方法、使用字典进行批量替换。其中,使用str.replace()方法是最常见和直接的方法。
例如,str.replace()方法可以简单地实现字符串中的字符替换。假设有一个字符串text = "Hello World"
,我们可以使用text.replace("World", "Python")
将"World"替换为"Python"。
text = "Hello World"
new_text = text.replace("World", "Python")
print(new_text) # 输出: Hello Python
下面将详细介绍Python中常用的几种替换方法。
一、使用str.replace()方法
str.replace()
是Python字符串对象的一个方法,它用于将字符串中的某个子串替换为另一个子串。
基本用法
original_string = "I love apples, apples are my favorite fruit."
new_string = original_string.replace("apples", "oranges")
print(new_string) # 输出: I love oranges, oranges are my favorite fruit.
使用replace()
替换多个字符
如果需要替换多个不同的字符,可以多次调用replace()
方法。
text = "The quick brown fox jumps over the lazy dog."
text = text.replace("quick", "slow").replace("brown", "black").replace("lazy", "active")
print(text) # 输出: The slow black fox jumps over the active dog.
替换指定次数
replace()
方法还可以指定最多替换的次数。
text = "banana banana banana"
new_text = text.replace("banana", "apple", 2)
print(new_text) # 输出: apple apple banana
二、使用re模块的sub()方法
对于更复杂的替换操作,Python的re
模块提供了sub()
方法,支持使用正则表达式进行替换。
基本用法
import re
text = "The rain in Spain stays mainly in the plain."
new_text = re.sub(r"ain", "XXX", text)
print(new_text) # 输出: The rXXX in SpXXX stays mXXXly in the plXXX.
使用正则表达式进行复杂替换
import re
text = "Contact us at support@example.com or sales@example.com."
new_text = re.sub(r"(\w+)@example.com", r"\1@newdomain.com", text)
print(new_text) # 输出: Contact us at support@newdomain.com or sales@newdomain.com.
替换指定次数
类似于replace()
方法,sub()
也可以指定替换的次数。
import re
text = "cat bat rat cat bat rat"
new_text = re.sub(r"cat", "dog", text, 1)
print(new_text) # 输出: dog bat rat cat bat rat
三、使用字典进行批量替换
如果需要进行多个替换操作,并且每个替换操作都不同,可以使用字典来进行批量替换。
基本用法
def multiple_replace(text, replacements):
for old, new in replacements.items():
text = text.replace(old, new)
return text
replacements = {"cat": "dog", "bat": "rat"}
text = "cat bat rat cat bat rat"
new_text = multiple_replace(text, replacements)
print(new_text) # 输出: dog rat rat dog rat rat
使用正则表达式和字典进行批量替换
import re
def multiple_replace(text, replacements):
pattern = re.compile("|".join(re.escape(key) for key in replacements.keys()))
return pattern.sub(lambda match: replacements[match.group(0)], text)
replacements = {"cat": "dog", "bat": "rat"}
text = "cat bat rat cat bat rat"
new_text = multiple_replace(text, replacements)
print(new_text) # 输出: dog rat rat dog rat rat
四、使用lambda表达式进行动态替换
有时,替换操作不仅依赖于静态的替换文本,还需要根据具体情况动态生成替换文本。此时,可以使用sub()
方法的替换函数功能。
动态替换
import re
def dynamic_replacement(match):
return match.group(0).upper()
text = "hello world! welcome to python."
new_text = re.sub(r"\b\w+\b", dynamic_replacement, text)
print(new_text) # 输出: HELLO WORLD! WELCOME TO PYTHON.
五、使用字符串模板进行替换
Python的string
模块提供了Template
类,可以用来进行字符串模板替换操作。
基本用法
from string import Template
template = Template("Hello, $name! Welcome to $place.")
result = template.substitute(name="Alice", place="Wonderland")
print(result) # 输出: Hello, Alice! Welcome to Wonderland.
使用safe_substitute()
方法
safe_substitute()
方法在替换时不会因缺少参数而抛出异常。
from string import Template
template = Template("Hello, $name! Welcome to $place.")
result = template.safe_substitute(name="Alice")
print(result) # 输出: Hello, Alice! Welcome to $place.
六、使用自定义函数进行替换
有时需要对字符串进行更复杂的操作,可以定义一个自定义函数来处理替换操作。
基本用法
def custom_replace(text, target, replacement):
return text.replace(target, replacement)
text = "I love programming."
new_text = custom_replace(text, "programming", "Python")
print(new_text) # 输出: I love Python.
使用自定义函数进行复杂替换
def complex_replace(text, replacements):
for target, replacement in replacements:
text = text.replace(target, replacement)
return text
replacements = [("quick", "slow"), ("brown", "black"), ("lazy", "active")]
text = "The quick brown fox jumps over the lazy dog."
new_text = complex_replace(text, replacements)
print(new_text) # 输出: The slow black fox jumps over the active dog.
七、使用正则表达式和lambda表达式进行批量替换
结合正则表达式和lambda表达式,可以实现更加灵活的替换操作。
基本用法
import re
text = "The price of the item is $20.99, and the discount is 15%."
new_text = re.sub(r"\$\d+\.\d+", lambda x: "${:.2f}".format(float(x.group().replace("$", "")) * 1.1), text)
print(new_text) # 输出: The price of the item is $23.09, and the discount is 15%.
八、使用第三方库进行替换
有时需要更强大的替换功能,可以借助第三方库。一个常见的库是pandas
,它提供了丰富的数据处理功能。
使用pandas进行替换
import pandas as pd
data = {'text': ['I love apples', 'Apples are great', 'I eat apples every day']}
df = pd.DataFrame(data)
df['text'] = df['text'].str.replace('apples', 'oranges')
print(df)
以上介绍了多种在Python中实现替换的方法。选择合适的方法取决于具体需求,例如简单的替换可以直接使用str.replace()
,而复杂的替换则可以考虑re.sub()
或者结合字典、lambda表达式等方法。掌握这些技巧可以帮助我们高效地进行字符串替换操作。
相关问答FAQs:
如何在Python中替换字符串中的特定字符?
在Python中,可以使用str.replace()
方法来替换字符串中的特定字符或子字符串。该方法接受两个参数:要替换的字符串和替换后的字符串。例如,my_string.replace("old", "new")
将把my_string
中的所有"old"替换为"new"。需要注意的是,该方法返回一个新的字符串,原字符串不会被改变。
Python中是否有其他库可以进行复杂的替换操作?
是的,Python的re
模块提供了强大的正则表达式功能,允许进行更复杂的替换。使用re.sub()
方法,可以根据模式匹配来替换字符串,例如,re.sub(r'\d+', 'number', my_string)
会将所有数字替换为"number"。这种方式适合需要基于模式的替换操作。
在Python中如何替换列表中的多个元素?
如果需要在列表中替换多个元素,可以使用列表推导式结合str.replace()
方法,或者使用循环遍历列表。比如,使用列表推导式可以这样实现:new_list = [item.replace("old", "new") for item in my_list]
。这种方法简洁高效,适用于需要批量替换的场景。
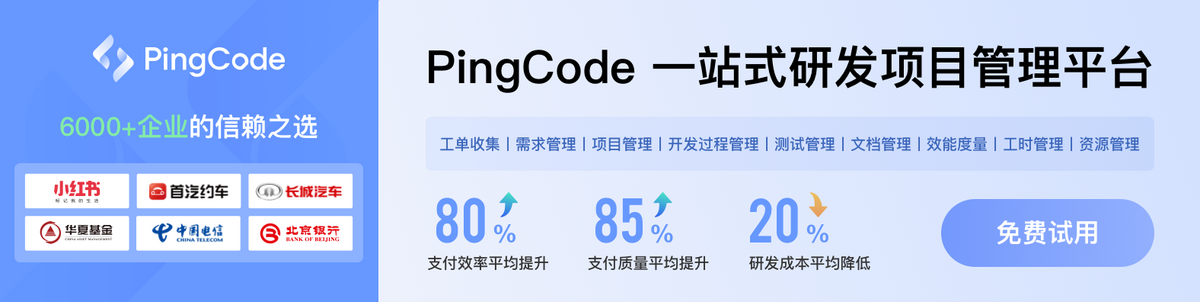