在Python中,可以通过len()函数、shape属性、size属性来求array的长度。 其中,len()函数用于求一维数组的长度,shape属性返回数组的各维度大小,size属性返回数组中所有元素的总数量。len()函数是最常用的方法,下面将详细描述。
使用len()函数求一维数组的长度非常简单直接。假设我们有一个一维数组a,我们只需要调用len(a),它将返回数组a的长度。以下是一个简单的示例:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
length = len(a)
print("The length of the array is:", length)
在上述示例中,len(a)将返回5,因为数组a包含5个元素。len()函数适用于一维数组,对于多维数组,它只返回第一个维度的长度。
接下来,我将详细介绍其他两种方法来求array的长度。
一、使用shape属性
1.1 了解shape属性
shape属性返回一个包含数组各维度大小的元组。在多维数组中,shape属性非常有用,因为它可以提供数组每一维度的大小。例如,对于一个二维数组,shape属性将返回一个包含两个元素的元组,第一个元素表示行数,第二个元素表示列数。
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6]])
shape = a.shape
print("The shape of the array is:", shape)
在上述示例中,a.shape将返回(2, 3),表示数组a有2行3列。对于多维数组,可以使用shape属性来获取每一维度的大小。
1.2 使用shape属性求长度
对于一维数组,shape属性返回一个包含一个元素的元组,该元素即为数组的长度。对于多维数组,可以通过shape属性获取特定维度的长度。例如,要获取二维数组的行数和列数,可以分别访问shape属性的第一个和第二个元素。
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6]])
rows = a.shape[0]
cols = a.shape[1]
print("The number of rows is:", rows)
print("The number of columns is:", cols)
在上述示例中,a.shape[0]返回2,即数组a的行数,a.shape[1]返回3,即数组a的列数。通过访问shape属性的不同元素,可以获取多维数组的各维度大小。
二、使用size属性
2.1 了解size属性
size属性返回数组中所有元素的总数量。与shape属性不同,size属性不提供各维度的大小,只返回一个整数值表示数组中所有元素的总数。size属性适用于所有维度的数组。
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6]])
total_elements = a.size
print("The total number of elements in the array is:", total_elements)
在上述示例中,a.size返回6,因为数组a包含6个元素。size属性提供了一个简单的方法来获取数组中所有元素的总数。
2.2 使用size属性求长度
size属性返回数组中所有元素的总数量,对于一维数组,size属性的值即为数组的长度。对于多维数组,size属性的值为所有维度元素数量的乘积。
import numpy as np
a = np.array([1, 2, 3, 4, 5])
length = a.size
print("The length of the array is:", length)
b = np.array([[1, 2, 3], [4, 5, 6]])
total_elements = b.size
print("The total number of elements in the array is:", total_elements)
在上述示例中,a.size返回5,即数组a的长度,b.size返回6,即数组b中所有元素的总数。size属性提供了一种简单的方法来获取数组中所有元素的总数量。
三、实例分析
通过上述方法,我们可以轻松求得一维数组和多维数组的长度以及各维度的大小。接下来,我们将通过一些实例来进一步了解这些方法的应用。
3.1 求一维数组的长度
import numpy as np
创建一维数组
a = np.array([10, 20, 30, 40, 50])
使用len()函数求长度
length_len = len(a)
使用shape属性求长度
length_shape = a.shape[0]
使用size属性求长度
length_size = a.size
print("Using len() function, the length of the array is:", length_len)
print("Using shape attribute, the length of the array is:", length_shape)
print("Using size attribute, the length of the array is:", length_size)
在上述实例中,使用len()函数、shape属性和size属性均能求得一维数组的长度,结果均为5。
3.2 求多维数组的长度
import numpy as np
创建二维数组
b = np.array([[1, 2, 3], [4, 5, 6]])
使用shape属性求行数和列数
rows = b.shape[0]
cols = b.shape[1]
使用size属性求元素总数量
total_elements = b.size
print("The number of rows is:", rows)
print("The number of columns is:", cols)
print("The total number of elements in the array is:", total_elements)
在上述实例中,通过shape属性求得二维数组的行数和列数分别为2和3,通过size属性求得数组中所有元素的总数量为6。
3.3 求三维数组的长度
import numpy as np
创建三维数组
c = np.array([[[1, 2, 3], [4, 5, 6]], [[7, 8, 9], [10, 11, 12]]])
使用shape属性求各维度大小
depth = c.shape[0]
rows = c.shape[1]
cols = c.shape[2]
使用size属性求元素总数量
total_elements = c.size
print("The depth of the array is:", depth)
print("The number of rows is:", rows)
print("The number of columns is:", cols)
print("The total number of elements in the array is:", total_elements)
在上述实例中,通过shape属性求得三维数组的深度、行数和列数分别为2、2和3,通过size属性求得数组中所有元素的总数量为12。
四、总结
在Python中求array长度的方法有多种,包括len()函数、shape属性和size属性。len()函数适用于求一维数组的长度,shape属性适用于获取多维数组的各维度大小,size属性用于求数组中所有元素的总数量。通过这些方法,我们可以轻松求得一维数组和多维数组的长度以及各维度的大小。
希望通过这篇文章,您能够更好地理解如何在Python中求array的长度,并能够灵活运用这些方法来处理不同的数组类型和维度。如果您有任何问题或需要进一步的指导,请随时联系我。
相关问答FAQs:
如何在Python中计算数组的长度?
在Python中,可以使用内置的len()
函数来获取数组(如列表或元组)的长度。只需将数组作为参数传递给len()
函数,它将返回数组中元素的数量。例如,len(my_array)
将返回my_array
中元素的总数。
如果我有一个多维数组,如何获取其长度?
对于多维数组,len()
函数返回的是最外层数组的长度。如果需要获取所有维度的长度,可以使用循环或列表推导式来遍历各个维度。例如,使用NumPy库处理多维数组时,可以使用array.shape
属性来获取每个维度的长度,这样就能更直观地了解数组的结构。
在Python中,如何处理空数组的长度?
即使数组为空,使用len()
函数也会返回0
,这表明数组中没有元素。无论是列表、元组还是NumPy数组,空数组的长度都是0
。这使得检查数组是否为空变得简单,只需判断len(my_array) == 0
即可。
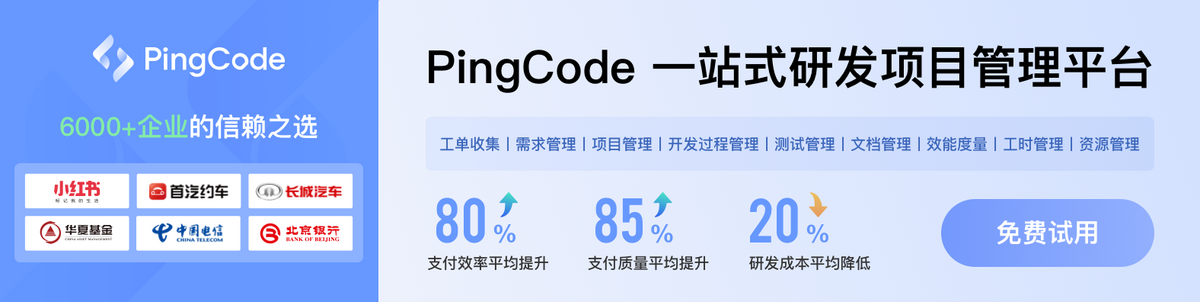