用Python写一个时钟的步骤包括:创建窗口、绘制时钟界面、更新时间、实现动画效果,其中一个重要步骤是更新时间。详细描述如下:
更新时间:为了让时钟显示当前时间,需要使用Python的time
模块获取系统的当前时间,并定期更新。可以通过定时器或循环来实现,使得每秒钟更新一次时间显示。
以下是一个详细的Python代码示例,展示了如何用Python和Tkinter库编写一个简单的时钟。Tkinter是Python的标准GUI库,可以很方便地用来创建图形界面。
import tkinter as tk
import time
class Clock:
def __init__(self, root):
self.root = root
self.root.title("Python Clock")
self.label = tk.Label(root, font=('calibri', 40, 'bold'), background='purple', foreground='white')
self.label.pack(anchor='center')
self.update_time()
def update_time(self):
current_time = time.strftime('%H:%M:%S')
self.label.config(text=current_time)
self.root.after(1000, self.update_time)
if __name__ == "__main__":
root = tk.Tk()
clock = Clock(root)
root.mainloop()
上述代码展示了一个简单的时钟应用,以下是更详细的解释和扩展。
一、创建窗口
在使用Tkinter创建时钟时,首先需要创建一个主窗口。Tkinter的根窗口是所有Tkinter应用程序的基础。可以通过实例化tk.Tk()
来创建一个根窗口。
root = tk.Tk()
root.title("Python Clock")
二、绘制时钟界面
接下来,我们需要在窗口中绘制时钟界面。可以使用Label
小部件来显示时间。Label
小部件用于显示文本或图像,在这个例子中,我们用它来显示当前时间。
label = tk.Label(root, font=('calibri', 40, 'bold'), background='purple', foreground='white')
label.pack(anchor='center')
这里,我们设置了字体、背景颜色和前景颜色,并将其放置在窗口的中心。
三、更新时间
为了让时钟显示当前时间,需要不断更新标签的文本。可以使用time.strftime
获取当前时间,并使用label.config
方法更新标签的文本。为了实现定期更新,可以使用after
方法,该方法会在指定的毫秒数后调用一个函数。
def update_time():
current_time = time.strftime('%H:%M:%S')
label.config(text=current_time)
root.after(1000, update_time)
四、实现动画效果
为了让时钟每秒钟更新一次,我们在Clock
类的构造函数中调用update_time
方法。这将启动一个循环,每秒钟更新一次时间。
class Clock:
def __init__(self, root):
self.root = root
self.root.title("Python Clock")
self.label = tk.Label(root, font=('calibri', 40, 'bold'), background='purple', foreground='white')
self.label.pack(anchor='center')
self.update_time()
def update_time(self):
current_time = time.strftime('%H:%M:%S')
self.label.config(text=current_time)
self.root.after(1000, self.update_time)
五、运行程序
最后,我们需要创建Clock
对象,并启动Tkinter的主循环。
if __name__ == "__main__":
root = tk.Tk()
clock = Clock(root)
root.mainloop()
这个简单的时钟应用程序将显示当前时间,并每秒钟更新一次。通过使用Tkinter库,我们可以很容易地创建一个漂亮的图形界面,并实现动画效果。
六、进一步扩展
你可以进一步扩展这个时钟应用程序,增加更多的功能和美化界面。以下是一些建议:
1、添加日期显示
你可以在时钟上添加当前日期的显示。只需修改update_time
方法,使其同时更新日期和时间。
def update_time(self):
current_time = time.strftime('%H:%M:%S')
current_date = time.strftime('%Y-%m-%d')
self.label.config(text=f"{current_date}\n{current_time}")
self.root.after(1000, self.update_time)
2、更改字体和颜色
你可以根据自己的喜好更改字体和颜色,使时钟看起来更美观。可以在创建Label
时传递不同的字体和颜色参数。
self.label = tk.Label(root, font=('helvetica', 48, 'bold'), background='black', foreground='green')
3、添加秒表功能
你可以为时钟添加一个秒表功能,允许用户启动、停止和重置秒表。可以通过添加按钮和相关的回调函数来实现。
start_button = tk.Button(root, text='Start', command=self.start_stopwatch)
start_button.pack(side='left')
stop_button = tk.Button(root, text='Stop', command=self.stop_stopwatch)
stop_button.pack(side='left')
reset_button = tk.Button(root, text='Reset', command=self.reset_stopwatch)
reset_button.pack(side='left')
4、添加闹钟功能
你可以为时钟添加闹钟功能,允许用户设置一个特定的时间,并在到达该时间时播放声音或显示消息。可以使用datetime
模块来比较当前时间和闹钟时间,并在匹配时触发事件。
import datetime
def check_alarm(self):
current_time = datetime.datetime.now()
if current_time.hour == self.alarm_hour and current_time.minute == self.alarm_minute:
self.play_alarm_sound()
self.root.after(60000, self.check_alarm)
七、完整的时钟应用程序
以下是一个更完整的时钟应用程序示例,包含日期显示、秒表功能和闹钟功能。
import tkinter as tk
import time
import datetime
class Clock:
def __init__(self, root):
self.root = root
self.root.title("Python Clock")
self.time_label = tk.Label(root, font=('calibri', 40, 'bold'), background='purple', foreground='white')
self.time_label.pack(anchor='center')
self.date_label = tk.Label(root, font=('calibri', 20, 'bold'), background='purple', foreground='white')
self.date_label.pack(anchor='center')
self.update_time()
self.stopwatch_running = False
self.stopwatch_start_time = None
self.stopwatch_label = tk.Label(root, font=('calibri', 20, 'bold'), background='black', foreground='green')
self.stopwatch_label.pack(anchor='center')
start_button = tk.Button(root, text='Start', command=self.start_stopwatch)
start_button.pack(side='left')
stop_button = tk.Button(root, text='Stop', command=self.stop_stopwatch)
stop_button.pack(side='left')
reset_button = tk.Button(root, text='Reset', command=self.reset_stopwatch)
reset_button.pack(side='left')
self.alarm_hour = None
self.alarm_minute = None
alarm_button = tk.Button(root, text='Set Alarm', command=self.set_alarm)
alarm_button.pack(side='left')
def update_time(self):
current_time = time.strftime('%H:%M:%S')
current_date = time.strftime('%Y-%m-%d')
self.time_label.config(text=current_time)
self.date_label.config(text=current_date)
self.root.after(1000, self.update_time)
def start_stopwatch(self):
if not self.stopwatch_running:
self.stopwatch_start_time = datetime.datetime.now()
self.stopwatch_running = True
self.update_stopwatch()
def stop_stopwatch(self):
self.stopwatch_running = False
def reset_stopwatch(self):
self.stopwatch_running = False
self.stopwatch_label.config(text='00:00:00')
def update_stopwatch(self):
if self.stopwatch_running:
elapsed_time = datetime.datetime.now() - self.stopwatch_start_time
elapsed_seconds = int(elapsed_time.total_seconds())
hours, remainder = divmod(elapsed_seconds, 3600)
minutes, seconds = divmod(remainder, 60)
self.stopwatch_label.config(text=f'{hours:02}:{minutes:02}:{seconds:02}')
self.root.after(1000, self.update_stopwatch)
def set_alarm(self):
alarm_time = tk.simpledialog.askstring("Alarm", "Enter alarm time (HH:MM):")
if alarm_time:
alarm_hour, alarm_minute = map(int, alarm_time.split(':'))
self.alarm_hour = alarm_hour
self.alarm_minute = alarm_minute
self.check_alarm()
def check_alarm(self):
current_time = datetime.datetime.now()
if current_time.hour == self.alarm_hour and current_time.minute == self.alarm_minute:
self.play_alarm_sound()
self.root.after(60000, self.check_alarm)
def play_alarm_sound(self):
# Implement your alarm sound playing logic here
print("Alarm! Wake up!")
if __name__ == "__main__":
root = tk.Tk()
clock = Clock(root)
root.mainloop()
这个完整的时钟应用程序包括了日期显示、秒表功能和闹钟功能。你可以根据自己的需求进一步扩展和优化。
八、总结
用Python编写一个时钟应用程序并不难,通过使用Tkinter库可以很方便地创建图形界面,并实现时间更新和动画效果。你可以根据自己的需求进一步扩展应用程序,添加更多功能和美化界面。希望这篇文章对你有所帮助,祝你编程愉快!
相关问答FAQs:
如何在Python中创建一个简单的时钟应用程序?
在Python中,可以使用Tkinter库来创建一个简单的时钟应用程序。Tkinter是Python的标准GUI库,能够帮助你快速构建图形界面。以下是一个基本示例代码,展示如何实现一个实时更新的时钟界面:
import tkinter as tk
import time
def update_clock():
current_time = time.strftime('%H:%M:%S')
clock_label.config(text=current_time)
clock_label.after(1000, update_clock) # 每1000毫秒更新一次
root = tk.Tk()
root.title('时钟')
clock_label = tk.Label(root, font=('calibri', 40), background='black', foreground='white')
clock_label.pack(anchor='center')
update_clock()
root.mainloop()
使用Python编写时钟时需要注意哪些性能问题?
在编写时钟应用时,性能并不是主要关注点,因为时钟的更新频率相对较低。然而,确保你的代码高效运行仍然很重要。避免使用过多的资源,例如不必要的循环或复杂的计算。另外,Tkinter的after
方法能够有效管理时间更新,避免了使用 while
循环可能导致的界面卡顿。
可以用Python实现哪些高级时钟功能?
除了基本的时钟显示,你可以通过Python实现多种高级功能。比如,添加闹钟功能,允许用户设置提醒时间;实现世界时钟,显示不同地区的时间;或者创建一个计时器,适用于烹饪、运动等场景。使用datetime
模块可以帮助你处理时间和日期,结合Tkinter,你能创建一个功能更为丰富的时钟应用。
如何在Python时钟中使用自定义样式和主题?
使用Tkinter时,你可以通过修改标签的属性来实现自定义样式和主题。可以更改字体、颜色、背景以及布局。使用Tkinter的ttk
模块可以进一步美化你的界面,提供更现代的外观。你还可以结合CSS样式或其他图形库,如Pygame,来实现更复杂的视觉效果。
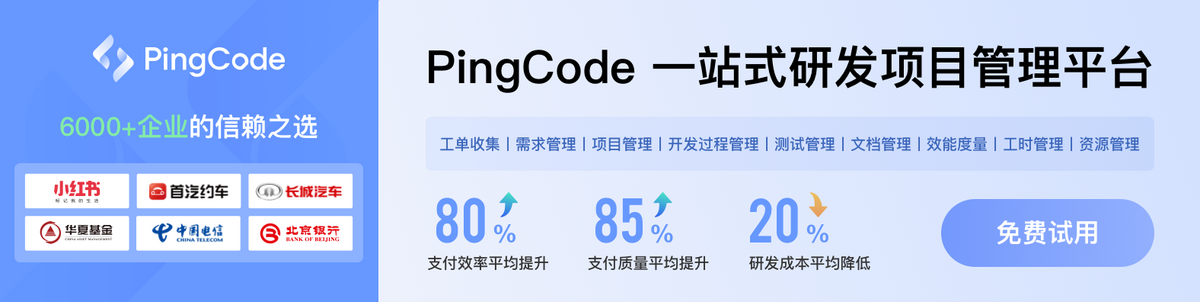