Python暂停某个进程可以通过信号机制、psutil
库、线程控制等方法实现,例如使用os.kill()
函数向进程发送信号、使用psutil
库中的suspend()
方法、使用threading
模块中的事件对象控制线程的执行等。下面将详细介绍如何使用psutil
库中的suspend()
方法来暂停某个进程。
psutil
是一个跨平台库,用于检索系统信息和管理进程。使用psutil
库中的Process
类可以轻松获取进程的相关信息并进行操作,例如暂停和恢复进程。下面将详细介绍如何使用psutil
库来暂停某个进程。
一、安装psutil库
在使用psutil
库之前,需要先安装该库。可以使用pip
命令来安装:
pip install psutil
二、使用psutil库暂停进程
- 获取进程ID(PID)
在暂停某个进程之前,需要知道该进程的PID。可以通过任务管理器或使用psutil
库中的方法来获取PID。
- 使用psutil库中的suspend()方法暂停进程
下面是一个示例代码,演示了如何使用psutil
库暂停某个进程:
import psutil
import time
def pause_process(pid):
try:
p = psutil.Process(pid)
p.suspend()
print(f"Process {pid} has been paused.")
except psutil.NoSuchProcess:
print(f"No process found with PID {pid}.")
except Exception as e:
print(f"An error occurred: {e}")
def resume_process(pid):
try:
p = psutil.Process(pid)
p.resume()
print(f"Process {pid} has been resumed.")
except psutil.NoSuchProcess:
print(f"No process found with PID {pid}.")
except Exception as e:
print(f"An error occurred: {e}")
if __name__ == "__main__":
pid = int(input("Enter the PID of the process to be paused: "))
pause_process(pid)
time.sleep(5) # Pausing for 5 seconds
resume_process(pid)
在上述代码中,用户输入需要暂停的进程PID,然后使用psutil
库中的Process
类获取进程对象,并调用suspend()
方法暂停进程。暂停5秒钟后,调用resume()
方法恢复进程的执行。
三、使用信号机制暂停进程
在类Unix系统中,进程管理可以通过信号机制来实现。使用os.kill()
函数向进程发送信号SIGSTOP
可以暂停进程,发送信号SIGCONT
可以恢复进程。下面是一个示例代码:
import os
import signal
import time
def pause_process(pid):
try:
os.kill(pid, signal.SIGSTOP)
print(f"Process {pid} has been paused.")
except ProcessLookupError:
print(f"No process found with PID {pid}.")
except Exception as e:
print(f"An error occurred: {e}")
def resume_process(pid):
try:
os.kill(pid, signal.SIGCONT)
print(f"Process {pid} has been resumed.")
except ProcessLookupError:
print(f"No process found with PID {pid}.")
except Exception as e:
print(f"An error occurred: {e}")
if __name__ == "__main__":
pid = int(input("Enter the PID of the process to be paused: "))
pause_process(pid)
time.sleep(5) # Pausing for 5 seconds
resume_process(pid)
在上述代码中,用户输入需要暂停的进程PID,然后使用os.kill()
函数向进程发送信号SIGSTOP
暂停进程。暂停5秒钟后,使用os.kill()
函数向进程发送信号SIGCONT
恢复进程的执行。
四、使用线程控制暂停进程
如果需要暂停和恢复Python线程,可以使用threading
模块中的事件对象来控制线程的执行。下面是一个示例代码:
import threading
import time
def worker(event):
while not event.is_set():
print("Working...")
time.sleep(1)
print("Worker thread is paused.")
def main():
event = threading.Event()
thread = threading.Thread(target=worker, args=(event,))
thread.start()
time.sleep(5)
event.set() # Pause the worker thread
print("Main thread paused the worker thread.")
time.sleep(5)
event.clear() # Resume the worker thread
print("Main thread resumed the worker thread.")
if __name__ == "__main__":
main()
在上述代码中,主线程使用事件对象event
控制工作线程的执行。工作线程在事件对象未设置时持续执行,当事件对象设置后,工作线程暂停执行。主线程可以清除事件对象来恢复工作线程的执行。
五、总结
通过上述方法,可以在Python中实现对进程和线程的暂停和恢复操作。使用psutil库中的suspend()方法、信号机制中的SIGSTOP和SIGCONT信号、以及threading模块中的事件对象,都可以实现对进程或线程的控制。每种方法都有其适用的场景和限制,开发者可以根据具体需求选择合适的方法。
六、注意事项
在实际应用中,需要注意以下几点:
- 权限问题:在操作系统中,暂停和恢复进程通常需要管理员权限。如果没有足够的权限,可能会导致操作失败。
- 稳定性和兼容性:不同操作系统和Python版本之间可能存在差异,需要进行充分的测试和验证,确保代码在目标环境中稳定运行。
- 资源管理:暂停进程可能会导致资源占用,恢复进程时需要确保资源的合理分配和管理,避免资源泄漏和冲突。
通过对上述方法的学习和应用,可以在Python中实现对进程和线程的灵活控制,提高程序的稳定性和性能。
相关问答FAQs:
如何在Python中暂停特定进程的执行?
在Python中,可以使用os
模块结合signal
模块来暂停特定进程的执行。首先,使用os.kill(pid, signal.SIGSTOP)
来发送停止信号,pid
是要暂停的进程ID。要恢复进程,可以使用os.kill(pid, signal.SIGCONT)
发送继续信号。
使用哪些库可以更方便地管理进程?
除了os
和signal
模块,subprocess
库也提供了更高级的进程管理功能。使用subprocess.Popen
可以启动新的进程,并且可以通过调用Popen.send_signal(signal.SIGSTOP)
暂停它。这个方法相对简单,更适合于需要频繁管理子进程的场景。
在多线程环境中,如何安全地暂停某个进程?
在多线程环境中暂停进程时,确保正确管理线程间的资源和锁。可以使用threading.Event
对象来标记进程的暂停状态,并在需要暂停时通过设置事件来实现。通过这种方式,可以在不中断其他线程的情况下,有效地控制特定进程的执行。
在什么情况下需要暂停Python进程?
暂停Python进程通常在需要进行资源管理、调试或临时中断长时间运行的任务时使用。例如,在处理大型数据集时,若系统负载过高,可以暂时暂停进程以释放资源,待系统恢复后再继续执行。
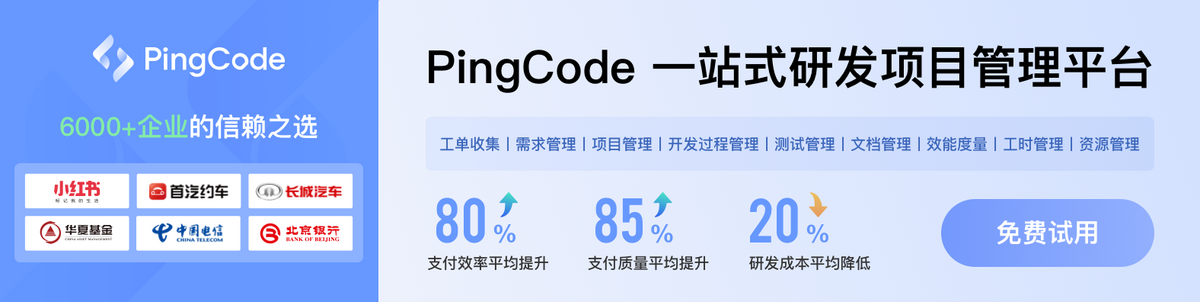