要在Python中查找相近地址,可以使用地理编码和反向地理编码技术,使用地理编码库(如Geopy)、使用距离计算(如Haversine公式)、使用空间索引(如R-tree)。下面将详细描述如何使用Geopy库进行地理编码,并结合Haversine公式来查找相近地址。
使用Geopy库进行地理编码和反向地理编码非常方便。首先,你需要安装Geopy库,可以通过以下命令进行安装:
pip install geopy
安装完成后,你可以使用Geopy库将地址转换为经纬度坐标,并使用这些坐标计算两个地址之间的距离。下面是一个示例代码,展示了如何使用Geopy和Haversine公式来查找相近地址:
from geopy.geocoders import Nominatim
from geopy.distance import geodesic
初始化地理编码器
geolocator = Nominatim(user_agent="geoapiExercises")
定义计算两个坐标之间距离的函数
def calculate_distance(coord1, coord2):
return geodesic(coord1, coord2).kilometers
获取地址的经纬度坐标
def get_coordinates(address):
location = geolocator.geocode(address)
if location:
return (location.latitude, location.longitude)
else:
return None
示例地址
address1 = "1600 Amphitheatre Parkway, Mountain View, CA"
address2 = "1 Infinite Loop, Cupertino, CA"
获取地址的经纬度坐标
coord1 = get_coordinates(address1)
coord2 = get_coordinates(address2)
计算两个地址之间的距离
if coord1 and coord2:
distance = calculate_distance(coord1, coord2)
print(f"The distance between the addresses is {distance:.2f} kilometers.")
else:
print("Unable to get the coordinates for one or both addresses.")
这段代码展示了如何使用Geopy库将地址转换为经纬度坐标,并使用geopy.distance.geodesic函数计算两个地址之间的距离。通过这种方式,你可以查找相近的地址。
接下来,将进一步探讨如何使用其他方法来查找相近地址,包括空间索引和其他距离计算方法。
一、使用地理编码和反向地理编码
地理编码是将地址转换为地理坐标(经纬度)的过程,反向地理编码是将地理坐标转换为地址的过程。Geopy库提供了方便的接口来进行这两种操作。
1. 使用Geopy进行地理编码
Geopy库支持多种地理编码服务,如Nominatim、Google Geocoding API、Bing Maps等。以下是使用Nominatim进行地理编码的示例:
from geopy.geocoders import Nominatim
def geocode_address(address):
geolocator = Nominatim(user_agent="geoapiExercises")
location = geolocator.geocode(address)
if location:
return (location.latitude, location.longitude)
else:
return None
address = "1600 Amphitheatre Parkway, Mountain View, CA"
coordinates = geocode_address(address)
if coordinates:
print(f"The coordinates for the address are: {coordinates}")
else:
print("Address not found.")
2. 使用Geopy进行反向地理编码
反向地理编码是将经纬度坐标转换为地址。以下是使用Nominatim进行反向地理编码的示例:
from geopy.geocoders import Nominatim
def reverse_geocode(lat, lon):
geolocator = Nominatim(user_agent="geoapiExercises")
location = geolocator.reverse((lat, lon), exactly_one=True)
if location:
return location.address
else:
return None
latitude = 37.4221
longitude = -122.0841
address = reverse_geocode(latitude, longitude)
if address:
print(f"The address for the coordinates is: {address}")
else:
print("Address not found.")
二、使用Haversine公式计算距离
Haversine公式是一种计算两个地理坐标点之间距离的数学公式。它假设地球是一个完美的球体,因此计算的距离是球面上的大圆距离。
1. Haversine公式的实现
以下是Haversine公式的Python实现:
from math import radians, cos, sin, sqrt, atan2
def haversine(coord1, coord2):
R = 6371.0 # 地球半径,单位为公里
lat1, lon1 = radians(coord1[0]), radians(coord1[1])
lat2, lon2 = radians(coord2[0]), radians(coord2[1])
dlon = lon2 - lon1
dlat = lat2 - lat1
a = sin(dlat / 2)<strong>2 + cos(lat1) * cos(lat2) * sin(dlon / 2)</strong>2
c = 2 * atan2(sqrt(a), sqrt(1 - a))
distance = R * c
return distance
coord1 = (37.4221, -122.0841)
coord2 = (37.3318, -122.0312)
distance = haversine(coord1, coord2)
print(f"The distance between the coordinates is {distance:.2f} kilometers.")
三、使用空间索引
空间索引是一种数据结构,用于高效地存储和查询空间数据。R-tree是一种常用的空间索引结构,它可以高效地执行空间查询,如范围查询和最近邻查询。
1. 使用R-tree进行空间索引
以下是使用R-tree进行空间索引的示例:
from rtree import index
创建R-tree索引
idx = index.Index()
插入数据
coordinates = [
(1, (37.4221, -122.0841)),
(2, (37.3318, -122.0312)),
(3, (37.7749, -122.4194))
]
for i, coord in coordinates:
idx.insert(i, (coord[1], coord[0], coord[1], coord[0]))
查询最近的坐标点
query_point = (37.3382, -121.8863)
nearest = list(idx.nearest((query_point[1], query_point[0], query_point[1], query_point[0]), 1))
print(f"The nearest coordinate ID is: {nearest[0]}")
四、结合所有方法查找相近地址
通过结合地理编码、Haversine公式和空间索引技术,可以实现一个高效的查找相近地址的系统。以下是一个完整的示例,展示了如何将这些技术结合起来使用:
from geopy.geocoders import Nominatim
from geopy.distance import geodesic
from rtree import index
初始化地理编码器
geolocator = Nominatim(user_agent="geoapiExercises")
获取地址的经纬度坐标
def get_coordinates(address):
location = geolocator.geocode(address)
if location:
return (location.latitude, location.longitude)
else:
return None
创建R-tree索引
idx = index.Index()
插入示例数据
addresses = [
"1600 Amphitheatre Parkway, Mountain View, CA",
"1 Infinite Loop, Cupertino, CA",
"1601 Willow Road, Menlo Park, CA"
]
coordinates = []
for i, address in enumerate(addresses):
coord = get_coordinates(address)
if coord:
coordinates.append((i, coord))
idx.insert(i, (coord[1], coord[0], coord[1], coord[0]))
定义计算两个坐标之间距离的函数
def calculate_distance(coord1, coord2):
return geodesic(coord1, coord2).kilometers
查询最近的地址
query_address = "Stanford University, Stanford, CA"
query_coord = get_coordinates(query_address)
if query_coord:
nearest = list(idx.nearest((query_coord[1], query_coord[0], query_coord[1], query_coord[0]), 1))
nearest_coord = coordinates[nearest[0]][1]
distance = calculate_distance(query_coord, nearest_coord)
print(f"The nearest address is {addresses[nearest[0]]} with a distance of {distance:.2f} kilometers.")
else:
print("Unable to get the coordinates for the query address.")
五、优化和扩展
在实际应用中,可能需要对查找相近地址的系统进行进一步优化和扩展。以下是一些可能的优化和扩展方向:
1. 批量地理编码
如果需要对大量地址进行地理编码,可以考虑使用批量地理编码服务。这些服务可以一次性处理多个地理编码请求,从而提高效率。
2. 缓存结果
为了提高性能,可以缓存地理编码和距离计算的结果。这样可以避免对相同地址或坐标进行重复查询和计算。
3. 多线程和异步处理
对于大量并发请求,可以使用多线程或异步处理技术来提高系统的响应速度。例如,可以使用Python的concurrent.futures
库或asyncio
库来实现并发处理。
六、示例代码优化
以下是一个优化后的查找相近地址的示例代码,使用了批量地理编码和多线程处理:
from geopy.geocoders import Nominatim
from geopy.distance import geodesic
from rtree import index
from concurrent.futures import ThreadPoolExecutor
初始化地理编码器
geolocator = Nominatim(user_agent="geoapiExercises")
获取地址的经纬度坐标
def get_coordinates(address):
location = geolocator.geocode(address)
if location:
return (location.latitude, location.longitude)
else:
return None
创建R-tree索引
idx = index.Index()
插入示例数据
addresses = [
"1600 Amphitheatre Parkway, Mountain View, CA",
"1 Infinite Loop, Cupertino, CA",
"1601 Willow Road, Menlo Park, CA"
]
coordinates = []
with ThreadPoolExecutor(max_workers=5) as executor:
future_to_address = {executor.submit(get_coordinates, address): address for address in addresses}
for future in future_to_address:
address = future_to_address[future]
coord = future.result()
if coord:
coordinates.append((addresses.index(address), coord))
idx.insert(addresses.index(address), (coord[1], coord[0], coord[1], coord[0]))
定义计算两个坐标之间距离的函数
def calculate_distance(coord1, coord2):
return geodesic(coord1, coord2).kilometers
查询最近的地址
query_address = "Stanford University, Stanford, CA"
query_coord = get_coordinates(query_address)
if query_coord:
nearest = list(idx.nearest((query_coord[1], query_coord[0], query_coord[1], query_coord[0]), 1))
nearest_coord = coordinates[nearest[0]][1]
distance = calculate_distance(query_coord, nearest_coord)
print(f"The nearest address is {addresses[nearest[0]]} with a distance of {distance:.2f} kilometers.")
else:
print("Unable to get the coordinates for the query address.")
结论
通过使用地理编码、Haversine公式和空间索引技术,可以在Python中高效地查找相近地址。Geopy库提供了便捷的地理编码和反向地理编码功能,Haversine公式可以用于精确计算两个地址之间的距离,而R-tree索引则可以高效地进行空间查询。通过结合这些技术,可以构建一个高效的查找相近地址的系统,并根据需要进行优化和扩展。
相关问答FAQs:
如何使用Python查找附近的地址?
在Python中,可以使用一些地理编码库和API来查找相近的地址。例如,使用Google Maps API或Geopy库可以帮助你获取指定位置附近的地址。你需要先注册获取API密钥,然后通过Python代码发送请求以获得地址信息。
有哪些Python库可以帮助我找到相近地址?
常用的库包括Geopy、Google Maps API、Nominatim等。Geopy是一个简单易用的库,支持多种地理编码服务。Google Maps API提供更强大的功能,但需要付费和注册API密钥。Nominatim是OpenStreetMap的一个API,适合开源项目。
如何处理查找结果中的错误或不准确的地址?
当使用API或库查找地址时,可能会遇到返回错误或不准确地址的情况。可以通过验证返回的地址信息,例如检查地址的完整性、格式和实际存在性,来提高结果的准确性。此外,也可以结合使用多个API,取最佳结果以减少错误的可能性。
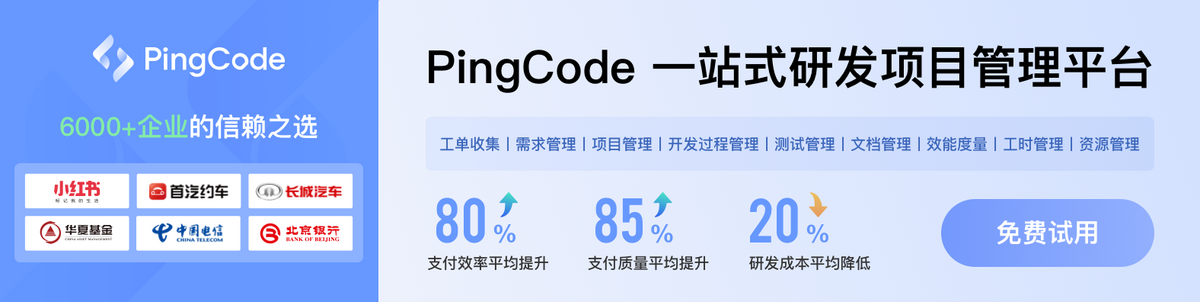