在Python编程中,换行的方法有很多种,可以使用换行符 \n、三引号字符串、多行注释、括号包裹的多行代码、反斜杠 \ 来实现。这些方法可以在不同的场景下应用,以提高代码的可读性和可维护性。本文将详细解释这些方法,并举例说明它们的具体使用方式。
一、使用换行符 \n
换行符 \n 是一种常用的方法来实现字符串的换行。在字符串中插入 \n 即可在输出时换行。
print("Hello\nWorld")
上述代码将输出:
Hello
World
二、使用三引号字符串
三引号字符串(''' 或 """)可以让字符串跨越多行,不需要显式地使用换行符。
multi_line_string = """This is a string
that spans multiple
lines."""
print(multi_line_string)
上述代码将输出:
This is a string
that spans multiple
lines.
三、使用多行注释
虽然多行注释主要用于注释代码,但它们也可以用于定义多行字符串,与三引号字符串类似。
multi_line_comment = '''This is another way
to define a multi-line
string.'''
print(multi_line_comment)
四、括号包裹的多行代码
在Python中,可以使用括号(圆括号、方括号、大括号)将代码分割成多行。这在长的表达式或列表、字典等数据结构中非常有用。
long_expression = (
"This is a long string "
"that is split over "
"multiple lines."
)
print(long_expression)
上述代码将输出:
This is a long string that is split over multiple lines.
五、使用反斜杠
反斜杠 \ 可以用来将一行代码分割成多行,但它并不常用,因为容易造成代码的可读性问题。
long_string = "This is a long string " \
"that is split over " \
"multiple lines."
print(long_string)
上述代码将输出:
This is a long string that is split over multiple lines.
六、在函数定义和调用中的换行
在定义和调用函数时,尤其是当参数较多时,换行可以使代码更简洁和易读。例如:
def long_function_name(
var_one, var_two, var_three,
var_four):
print(var_one)
long_function_name(
'first_argument', 'second_argument',
'third_argument', 'fourth_argument')
七、在条件语句和循环中的换行
在条件语句和循环中,可以使用括号或反斜杠来换行,使代码更清晰。
if (some_condition and
another_condition):
print("Conditions met")
for i in range(1, 11):
print(i)
八、在列表、字典和集合中的换行
在定义长的列表、字典和集合时,换行可以使代码更易于阅读和维护。
my_list = [
1, 2, 3,
4, 5, 6
]
my_dict = {
'key1': 'value1',
'key2': 'value2',
'key3': 'value3'
}
my_set = {
'apple',
'banana',
'cherry'
}
九、在类和方法定义中的换行
在定义类和方法时,也可以使用换行来提高代码的可读性。
class MyClass:
def __init__(self, var_one, var_two,
var_three, var_four):
self.var_one = var_one
self.var_two = var_two
self.var_three = var_three
self.var_four = var_four
def long_method_name(
self, arg_one, arg_two,
arg_three, arg_four):
pass
十、使用文本处理模块
在Python中,有许多模块可以帮助处理文本和实现换行。例如,textwrap 模块可以用来格式化和包装文本。
import textwrap
long_text = "This is a long piece of text that needs to be wrapped and formatted properly."
wrapped_text = textwrap.fill(long_text, width=40)
print(wrapped_text)
上述代码将输出:
This is a long piece of text that
needs to be wrapped and formatted
properly.
十一、在数据输出中使用换行
在将数据输出到文件或其他地方时,也可以使用换行符 \n 或其他方法来实现换行。
with open('output.txt', 'w') as file:
file.write("Hello\nWorld")
十二、使用格式化字符串
格式化字符串(f-strings)可以结合换行符 \n 来实现更复杂的换行需求。
name = "Alice"
age = 30
formatted_string = f"Name: {name}\nAge: {age}"
print(formatted_string)
上述代码将输出:
Name: Alice
Age: 30
十三、在正则表达式中使用换行
在正则表达式中,可以使用换行符 \n 来匹配多行文本。
import re
text = "Hello\nWorld"
pattern = re.compile(r"Hello\nWorld")
match = pattern.match(text)
if match:
print("Match found")
十四、使用日志模块
在记录日志时,也可以使用换行符 \n 或其他方法来格式化日志信息。
import logging
logging.basicConfig(level=logging.DEBUG)
logging.debug("This is a debug message\nwith a new line.")
十五、在Jupyter Notebook中使用换行
在Jupyter Notebook中,可以使用换行符 \n 或者直接按 Enter 键来实现换行。
print("Hello\nWorld")
十六、在Web开发中的换行
在Web开发中,换行符 \n 和 HTML 标签
都可以用来实现换行。
html_content = "Hello<br>World"
print(html_content)
上述代码将输出:
Hello<br>World
十七、在GUI应用程序中的换行
在使用GUI库(如Tkinter、PyQt等)开发应用程序时,也需要处理文本的换行。
import tkinter as tk
root = tk.Tk()
label = tk.Label(root, text="Hello\nWorld")
label.pack()
root.mainloop()
十八、在多行注释中的换行
多行注释不仅仅用于注释代码,也可以用于长字符串的定义,尤其是在文档字符串(docstring)中。
def example_function():
"""
This is an example function.
It demonstrates the use of multi-line
docstrings in Python.
"""
pass
十九、在数据分析中的换行
在数据分析中,处理长字符串数据时,换行是必不可少的。例如,使用 pandas 库处理数据时,可以使用换行符来格式化输出。
import pandas as pd
data = {'Column1': ['Value1\nLine2', 'Value2\nLine2'], 'Column2': [1, 2]}
df = pd.DataFrame(data)
print(df)
二十、在机器学习中的换行
在机器学习项目中,处理文本数据时,换行符也是常见的需求。例如,在预处理文本数据时,可以使用换行符来分割句子。
text_data = "This is a sentence.\nThis is another sentence."
sentences = text_data.split('\n')
print(sentences)
二十一、在网络编程中的换行
在网络编程中,发送和接收数据时,换行符也是常见的需求。例如,在使用 sockets 进行通信时,可以使用换行符来分割消息。
import socket
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 8080))
server_socket.listen(1)
client_socket, address = server_socket.accept()
client_socket.sendall(b"Hello\nWorld")
client_socket.close()
server_socket.close()
二十二、在文件操作中的换行
在处理文件操作时,换行符 \n 是必不可少的。例如,在读取和写入文件时,可以使用换行符来分割行。
with open('example.txt', 'w') as file:
file.write("Line1\nLine2\nLine3")
with open('example.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line.strip())
二十三、在数据可视化中的换行
在使用数据可视化库(如 matplotlib、seaborn 等)绘制图表时,也可以使用换行符来处理长的标签和注释。
import matplotlib.pyplot as plt
plt.title("This is a long title\nwith a new line")
plt.xlabel("X-axis\nlabel")
plt.ylabel("Y-axis\nlabel")
plt.plot([1, 2, 3], [4, 5, 6])
plt.show()
二十四、在自然语言处理中的换行
在自然语言处理(NLP)项目中,处理文本数据时,换行符也是非常常见的。例如,在分割段落和句子时,可以使用换行符。
text_data = "This is a paragraph.\nThis is another paragraph."
paragraphs = text_data.split('\n')
print(paragraphs)
二十五、在科学计算中的换行
在科学计算项目中,处理长的公式和表达式时,换行符可以提高代码的可读性和可维护性。
import numpy as np
matrix = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
print(matrix)
二十六、在配置文件中的换行
在处理配置文件(如 .ini 文件、.yaml 文件等)时,换行符可以帮助分割不同的配置项,提高文件的可读性。
[section1]
key1 = value1
key2 = value2
[section2]
key3 = value3
key4 = value4
二十七、在游戏开发中的换行
在游戏开发中,处理长的对话和文本数据时,换行符也是非常常见的。例如,在显示游戏对话时,可以使用换行符来分割行。
dialogue = "Hello adventurer!\nWelcome to the world of Python."
print(dialogue)
二十八、在嵌入式系统编程中的换行
在嵌入式系统编程中,处理长的指令和数据时,换行符可以提高代码的可读性和可维护性。
const char *message = "Hello\nWorld";
printf("%s", message);
二十九、在自动化脚本中的换行
在编写自动化脚本时,处理长的命令和输出时,换行符也是非常常见的。例如,在使用 shell 脚本时,可以使用换行符来分割命令。
echo "Hello\nWorld"
三十、在测试代码中的换行
在编写测试代码时,处理长的测试用例和输出时,换行符可以提高代码的可读性和可维护性。
def test_example():
assert "Hello\nWorld" == "Hello\nWorld"
三十一、在数据处理中的换行
在处理大规模数据时,换行符可以帮助分割数据,提高处理效率和可读性。例如,在处理 CSV 文件时,可以使用换行符来分割行。
import csv
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(["Column1", "Column2"])
writer.writerow(["Value1\nLine2", "Value2\nLine2"])
三十二、在多语言开发中的换行
在多语言开发项目中,处理长的翻译文本时,换行符也是非常常见的。例如,在处理多语言翻译文件时,可以使用换行符来分割行。
{
"hello": "Hello\nWorld",
"welcome": "Welcome\nto Python"
}
三十三、在网络爬虫中的换行
在编写网络爬虫时,处理长的网页内容时,换行符可以帮助分割文本,提高处理效率和可读性。
import requests
response = requests.get('https://example.com')
content = response.text
lines = content.split('\n')
for line in lines:
print(line)
三十四、在人工智能项目中的换行
在人工智能项目中,处理长的训练数据和输出时,换行符也是非常常见的。例如,在处理长的文本数据时,可以使用换行符来分割行。
training_data = "This is a training example.\nThis is another training example."
examples = training_data.split('\n')
print(examples)
三十五、在金融分析中的换行
在金融分析项目中,处理长的金融数据和报告时,换行符可以提高数据的可读性和可维护性。
financial_report = "Revenue: $1000\nProfit: $200"
print(financial_report)
三十六、在物联网项目中的换行
在物联网(IoT)项目中,处理长的传感器数据和指令时,换行符可以提高数据的可读性和可维护性。
sensor_data = "Temperature: 25°C\nHumidity: 60%"
print(sensor_data)
三十七、在区块链项目中的换行
在区块链项目中,处理长的交易数据和区块信息时,换行符也是非常常见的。例如,在显示区块信息时,可以使用换行符来分割行。
block_info = "Block Number: 12345\nTransaction Count: 10"
print(block_info)
三十八、在云计算项目中的换行
在云计算项目中,处理长的配置文件和日志数据时,换行符可以提高数据的可读性和可维护性。
apiVersion: v1
kind: Pod
metadata:
name: mypod
spec:
containers:
- name: mycontainer
image: myimage
三十九、在安全分析中的换行
在安全分析项目中,处理长的日志数据和报告时,换行符也是非常常见的。例如,在显示安全日志时,可以使用换行符来分割行。
security_log = "Login attempt failed\nUser: admin"
print(security_log)
四十、在大数据项目中的换行
在大数据项目中,处理长的日志数据和报告时,换行符可以提高数据的可读性和可维护性。
log_data = "2023-10-01 10:00:00 INFO Start processing data\n2023-10-01 10:05:00 INFO Data processed"
print(log_data)
以上就是在Python编程中实现换行的多种方法和应用场景。掌握这些方法可以帮助你在不同的编程任务中有效地处理换行,提高代码的可读性和可维护性。
相关问答FAQs:
在Python中,如何在字符串中插入换行符?
在Python中,可以通过使用特殊字符\n
来插入换行符。比如,如果你想在打印的输出中换行,可以这样写:
print("第一行\n第二行")
这段代码会在输出中显示为:
第一行
第二行
这种方法在处理多行文本时非常有效,尤其是在需要格式化输出时。
使用三重引号在Python中如何实现多行字符串?
三重引号('''
或"""
)可以让你在字符串中直接换行,而无需使用\n
。例如:
multi_line_string = """这是第一行
这是第二行
这是第三行"""
print(multi_line_string)
这种方式使得代码更易读,也更方便用于长文本的定义。
在Python中换行时如何处理空行?
如果希望在输出中插入空行,可以连续使用print()
函数或在字符串中添加额外的换行符。例如:
print("第一行")
print() # 空行
print("第二行")
或者在字符串中添加多个换行符:
print("第一行\n\n第二行")
这样可以根据需要在输出中控制行间距,增强文本的可读性。
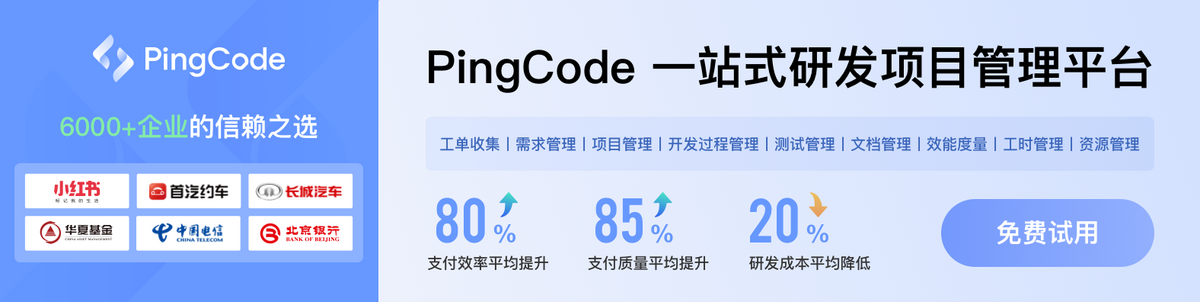