Python中使用选择结构的主要方法包括if语句、elif语句和else语句。选择结构可以帮助程序根据条件判断来执行不同的代码段,从而实现程序的逻辑控制。常用的选择结构有以下几种:if语句、if-else语句、if-elif-else语句。其中,if语句是最基本的形式,用于单一条件判断;if-else语句用于二选一的条件判断;if-elif-else语句用于多条件的判断。下面将详细介绍这些选择结构的使用方法。
一、IF语句
if语句是Python中最基本的选择结构,用于在某个条件为真时执行一段代码。其基本语法如下:
if condition:
# code to execute if condition is true
示例:
age = 18
if age >= 18:
print("You are an adult.")
在这个示例中,程序会检查变量age
是否大于或等于18,如果条件为真,程序将输出"You are an adult."。
二、IF-ELSE语句
if-else语句用于在条件为真时执行一段代码,在条件为假时执行另一段代码。其基本语法如下:
if condition:
# code to execute if condition is true
else:
# code to execute if condition is false
示例:
age = 17
if age >= 18:
print("You are an adult.")
else:
print("You are not an adult.")
在这个示例中,程序会检查变量age
是否大于或等于18,如果条件为真,程序将输出"You are an adult.",否则将输出"You are not an adult."。
三、IF-ELIF-ELSE语句
if-elif-else语句用于在多个条件中进行选择。其基本语法如下:
if condition1:
# code to execute if condition1 is true
elif condition2:
# code to execute if condition2 is true
else:
# code to execute if none of the above conditions are true
示例:
score = 85
if score >= 90:
print("Grade: A")
elif score >= 80:
print("Grade: B")
elif score >= 70:
print("Grade: C")
elif score >= 60:
print("Grade: D")
else:
print("Grade: F")
在这个示例中,程序会根据变量score
的值来判断该输出哪个等级。
四、嵌套IF语句
在实际编程中,有时候需要在一个if-else语句内部再嵌套另一个if-else语句,这种结构称为嵌套if语句。其基本语法如下:
if condition1:
if condition2:
# code to execute if both condition1 and condition2 are true
else:
# code to execute if condition1 is true and condition2 is false
else:
# code to execute if condition1 is false
示例:
age = 20
if age >= 18:
if age >= 21:
print("You are an adult and you can legally drink alcohol.")
else:
print("You are an adult but you cannot legally drink alcohol.")
else:
print("You are not an adult.")
在这个示例中,程序首先检查变量age
是否大于或等于18,如果条件为真,再检查是否大于或等于21,根据不同的条件输出不同的信息。
五、条件表达式(Ternary Operator)
Python中还提供了一种更简洁的条件表达式(也称为三元运算符),用于简化简单的if-else语句。其基本语法如下:
value_if_true if condition else value_if_false
示例:
age = 20
message = "You are an adult." if age >= 18 else "You are not an adult."
print(message)
在这个示例中,使用条件表达式简化了if-else语句,根据变量age
的值直接赋值给变量message
。
六、多个条件的组合使用
在实际编程中,经常需要结合多个条件进行判断。可以使用逻辑运算符and
、or
和not
来组合多个条件。其基本语法如下:
if condition1 and condition2:
# code to execute if both condition1 and condition2 are true
if condition1 or condition2:
# code to execute if either condition1 or condition2 is true
if not condition:
# code to execute if condition is false
示例:
age = 20
has_id = True
if age >= 18 and has_id:
print("You can enter the club.")
else:
print("You cannot enter the club.")
在这个示例中,只有当age
大于或等于18且has_id
为真时,程序才会输出"You can enter the club."。
七、选择结构在函数中的应用
选择结构在函数中同样适用,可以根据不同的输入条件执行不同的函数逻辑。其基本语法如下:
def function_name(parameter):
if condition1:
# code to execute if condition1 is true
elif condition2:
# code to execute if condition2 is true
else:
# code to execute if none of the above conditions are true
示例:
def determine_grade(score):
if score >= 90:
return "A"
elif score >= 80:
return "B"
elif score >= 70:
return "C"
elif score >= 60:
return "D"
else:
return "F"
grade = determine_grade(85)
print(f"The grade is {grade}.")
在这个示例中,函数determine_grade
根据输入的score
值返回相应的等级。
八、使用选择结构处理异常
在实际编程中,还需要处理各种可能出现的异常情况,可以结合选择结构和异常处理机制来实现。其基本语法如下:
try:
# code that may raise an exception
except SpecificException as e:
# code to handle the specific exception
else:
# code to execute if no exception is raised
finally:
# code to execute no matter what
示例:
try:
number = int(input("Enter a number: "))
if number > 0:
print("The number is positive.")
elif number < 0:
print("The number is negative.")
else:
print("The number is zero.")
except ValueError:
print("Invalid input. Please enter a valid number.")
finally:
print("Program execution completed.")
在这个示例中,程序会尝试将用户输入的内容转换为整数,如果输入无效会捕获ValueError
异常并输出提示信息。
九、选择结构的最佳实践
在编写选择结构时,需要遵循一些最佳实践,以提高代码的可读性和可维护性:
-
保持代码简洁明了:避免过于复杂的嵌套选择结构,可以考虑将复杂的逻辑拆分为多个函数。
-
使用合适的条件表达式:在适当的情况下,可以使用条件表达式简化代码。
-
注意代码缩进:Python使用缩进来表示代码块,确保所有代码块正确缩进,以避免语法错误。
-
合理使用逻辑运算符:在组合多个条件时,合理使用
and
、or
和not
逻辑运算符,确保逻辑判断准确。 -
处理所有可能的情况:在选择结构中,确保处理所有可能的输入情况,避免遗漏。
通过以上介绍,希望读者能够掌握Python中选择结构的使用方法,并在实际编程中灵活应用这些技巧,提高代码的逻辑控制能力。
相关问答FAQs:
选择结构在Python中是如何工作的?
选择结构是控制程序流程的一种方式,主要用于根据条件执行不同的代码块。在Python中,最常用的选择结构是if
语句。通过使用if
、elif
和else
关键字,程序可以根据不同的条件执行相应的代码。例如:
age = 20
if age < 18:
print("未成年人")
elif age < 65:
print("成年人")
else:
print("老年人")
在这个示例中,程序会根据age
变量的值输出不同的结果。
如何在Python中处理多条件选择?
在Python中,可以使用多个elif
语句来处理多条件选择。每个elif
都可以设置一个新的条件,只有当前面的条件都不满足时,才会检查这个条件。此外,使用逻辑运算符如and
、or
可以组合多个条件。例如:
score = 85
if score >= 90:
print("优秀")
elif score >= 75 and score < 90:
print("良好")
elif score >= 60:
print("及格")
else:
print("不及格")
此代码片段根据分数范围输出不同的评价。
在选择结构中如何避免使用多个嵌套?
避免多个嵌套可以通过合理利用elif
和逻辑运算符来实现。嵌套结构虽然有效,但会使代码难以阅读和维护。通过将复杂条件分解为简单条件,使用elif
来替代多层嵌套可以让代码更加清晰。例如,使用字典来替代多个条件判断也是一种有效的方法,具体示例如下:
grade = {
'A': "优秀",
'B': "良好",
'C': "及格",
'D': "不及格"
}
score = 'B'
print(grade.get(score, "无效成绩"))
这里通过字典的方式实现了条件的选择,使得代码更加简洁明了。
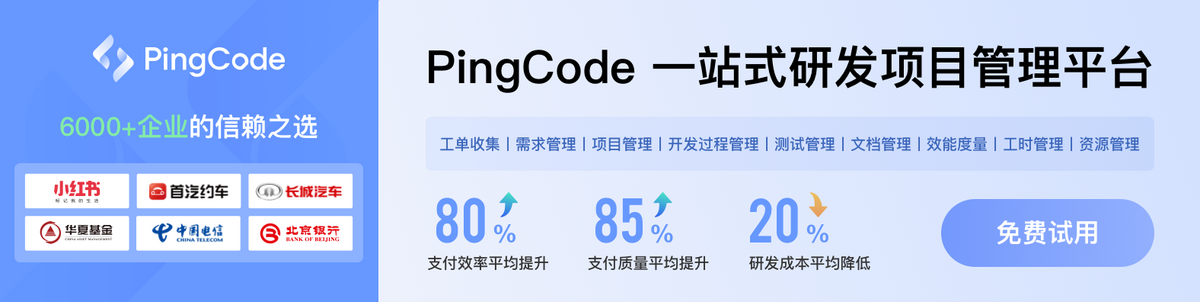