线程调用函数的关键步骤包括:创建线程对象、定义线程函数、启动线程、管理线程、避免竞态条件。在Python中,可以使用threading
模块来创建和管理线程。下面将详细描述如何在Python中使用线程调用函数。
一、创建线程对象
在Python中,线程是通过threading.Thread
类创建的。创建线程对象的第一步是导入threading
模块。接下来,可以实例化一个Thread
对象并传递目标函数和参数给它。
import threading
def my_function(arg1, arg2):
print(f"Arguments received: {arg1}, {arg2}")
创建线程对象
thread = threading.Thread(target=my_function, args=(10, 20))
二、定义线程函数
线程函数是在线程中执行的函数。它可以是任何接受参数的函数。在定义线程函数时,需要考虑线程安全性,特别是当多个线程可能同时访问共享资源时。
def my_function(arg1, arg2):
print(f"Arguments received: {arg1}, {arg2}")
这里定义了一个简单的函数,该函数将在新线程中运行
三、启动线程
创建线程对象后,需要启动线程。调用线程对象的start()
方法来启动线程。这将使线程进入可运行状态,并且线程将调用目标函数。
# 启动线程
thread.start()
四、管理线程
在大多数情况下,您希望等待线程完成其任务。可以使用join()
方法来等待线程完成。这可以确保主线程在子线程完成之前不会终止。
# 等待线程完成
thread.join()
五、避免竞态条件
当多个线程同时访问共享资源时,可能会导致竞态条件。可以使用线程锁(Lock)来避免这种情况。threading.Lock
对象可以确保一次只有一个线程访问共享资源。
import threading
创建一个锁对象
lock = threading.Lock()
def thread_function(name):
lock.acquire()
try:
print(f"Thread {name} is running")
# 访问共享资源
finally:
lock.release()
创建多个线程并启动
threads = []
for i in range(5):
thread = threading.Thread(target=thread_function, args=(i,))
threads.append(thread)
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
六、线程安全的数据结构
Python标准库提供了一些线程安全的数据结构,例如queue.Queue
,可以用于在线程之间安全地共享数据。使用这些数据结构可以减少处理线程同步的复杂性。
import threading
import queue
def worker(q):
while not q.empty():
item = q.get()
print(f"Processing item: {item}")
q.task_done()
创建一个Queue对象并添加一些项目
q = queue.Queue()
for item in range(10):
q.put(item)
创建并启动多个线程
threads = []
for _ in range(4):
thread = threading.Thread(target=worker, args=(q,))
thread.start()
threads.append(thread)
等待所有项目完成
q.join()
等待所有线程完成
for thread in threads:
thread.join()
七、线程池
使用线程池可以更有效地管理线程。concurrent.futures
模块提供了ThreadPoolExecutor
类,用于创建和管理线程池。线程池可以重用线程,减少线程创建和销毁的开销。
from concurrent.futures import ThreadPoolExecutor
def task(n):
print(f"Processing {n}")
创建一个线程池
with ThreadPoolExecutor(max_workers=5) as executor:
# 提交多个任务
for i in range(10):
executor.submit(task, i)
八、守护线程
守护线程是在主线程结束时自动终止的线程。可以通过将线程对象的daemon
属性设置为True
来创建守护线程。守护线程通常用于后台任务,例如日志记录或监控。
import threading
import time
def background_task():
while True:
print("Background task is running")
time.sleep(1)
创建守护线程
thread = threading.Thread(target=background_task)
thread.daemon = True
thread.start()
主线程继续执行
time.sleep(5)
print("Main thread is terminating")
九、线程本地数据
threading.local
类用于创建线程本地数据。每个线程可以独立地访问线程本地数据,而不会与其他线程冲突。这对于需要在线程之间保持独立状态的数据非常有用。
import threading
创建线程本地数据对象
local_data = threading.local()
def process_data():
local_data.value = threading.current_thread().name
print(f"Thread {local_data.value} is processing data")
创建并启动多个线程
threads = []
for _ in range(5):
thread = threading.Thread(target=process_data)
thread.start()
threads.append(thread)
等待所有线程完成
for thread in threads:
thread.join()
十、线程间通信
线程间通信可以通过条件变量(Condition)、事件(Event)和信号量(Semaphore)等同步原语实现。条件变量允许一个线程等待另一个线程发出信号。事件用于实现线程之间的简单通知机制。信号量用于控制对共享资源的访问。
import threading
使用条件变量实现线程间通信
condition = threading.Condition()
shared_data = []
def producer():
with condition:
for i in range(5):
shared_data.append(i)
print(f"Produced: {i}")
condition.notify()
condition.wait()
def consumer():
with condition:
while True:
condition.wait()
if shared_data:
item = shared_data.pop(0)
print(f"Consumed: {item}")
condition.notify()
创建并启动生产者和消费者线程
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
producer_thread.start()
consumer_thread.start()
等待线程完成
producer_thread.join()
consumer_thread.join()
十一、线程死锁
死锁是指两个或多个线程彼此等待对方释放资源,从而导致程序停止响应。避免死锁的方法包括:使用锁的顺序、使用超时机制、避免嵌套锁等。
import threading
lock1 = threading.Lock()
lock2 = threading.Lock()
def thread1():
with lock1:
print("Thread 1 acquired lock 1")
with lock2:
print("Thread 1 acquired lock 2")
def thread2():
with lock2:
print("Thread 2 acquired lock 2")
with lock1:
print("Thread 2 acquired lock 1")
创建并启动线程
t1 = threading.Thread(target=thread1)
t2 = threading.Thread(target=thread2)
t1.start()
t2.start()
等待线程完成
t1.join()
t2.join()
十二、线程优先级
在Python中,无法直接设置线程优先级。线程调度由操作系统管理,Python线程没有提供直接设置优先级的功能。但是,可以通过合理的设计和使用queue.PriorityQueue
来间接实现任务的优先级。
import threading
import queue
import time
def task(priority, message):
time.sleep(1)
print(f"Priority: {priority}, Message: {message}")
创建优先级队列
pq = queue.PriorityQueue()
向队列添加任务
pq.put((1, "Low priority task"))
pq.put((0, "High priority task"))
创建并启动线程
threads = []
for _ in range(2):
priority, message = pq.get()
thread = threading.Thread(target=task, args=(priority, message))
thread.start()
threads.append(thread)
等待线程完成
for thread in threads:
thread.join()
十三、线程和多进程
虽然线程可以并发执行任务,但由于GIL(全局解释器锁)的存在,Python线程在执行CPU密集型任务时并不能真正并行。对于CPU密集型任务,可以使用multiprocessing
模块来创建多个进程,每个进程有自己的Python解释器实例,从而实现真正的并行执行。
from multiprocessing import Process
def cpu_bound_task(n):
print(f"Processing: {n}")
创建并启动多个进程
processes = []
for i in range(5):
process = Process(target=cpu_bound_task, args=(i,))
process.start()
processes.append(process)
等待所有进程完成
for process in processes:
process.join()
十四、线程池与多进程池
concurrent.futures
模块不仅提供了ThreadPoolExecutor
,还提供了ProcessPoolExecutor
,用于创建和管理多进程池。可以根据任务的性质选择合适的执行器。
from concurrent.futures import ProcessPoolExecutor
def cpu_bound_task(n):
return n * n
创建一个多进程池
with ProcessPoolExecutor(max_workers=4) as executor:
results = executor.map(cpu_bound_task, range(10))
打印结果
for result in results:
print(result)
十五、总结
通过合理使用线程,可以显著提升程序的并发能力和响应速度。然而,使用线程也可能带来一些挑战,如竞态条件、死锁等问题。在编写多线程程序时,需要仔细考虑线程安全性,并使用适当的同步机制来保护共享资源。同时,对于CPU密集型任务,可以考虑使用多进程来实现真正的并行执行。
Python提供了丰富的多线程和多进程支持,使开发者能够根据具体需求选择合适的并发模型。无论是使用threading
模块创建和管理线程,还是使用concurrent.futures
模块创建线程池和多进程池,都可以有效地提高程序的性能和并发能力。希望本文能够帮助您更好地理解和使用Python中的线程和多进程技术。
相关问答FAQs:
在Python中如何创建和启动一个线程以调用函数?
要在Python中创建和启动一个线程来调用函数,可以使用threading
模块。你需要定义一个函数,然后创建一个Thread
对象,传入你的函数作为目标,最后调用start()
方法来启动线程。例如:
import threading
def my_function():
print("Hello from the thread!")
thread = threading.Thread(target=my_function)
thread.start()
这样,my_function
将会在新的线程中执行。
Python线程与进程有什么不同,为什么选择使用线程?
线程是轻量级的执行单元,它们共享同一进程的内存空间,而进程则是完全独立的执行环境。使用线程的优点包括较低的内存开销和更快的上下文切换速度,适合于I/O密集型任务,比如网络请求或文件读写。然而,Python的全局解释器锁(GIL)使得CPU密集型任务可能不适合使用线程。
如何在Python线程中处理异常?
在Python线程中处理异常非常重要,以避免线程崩溃而导致程序无法正常运行。可以在目标函数中使用try...except
语句来捕获异常。例如:
def my_function():
try:
# 可能会引发异常的代码
raise ValueError("An error occurred!")
except Exception as e:
print(f"Exception caught: {e}")
thread = threading.Thread(target=my_function)
thread.start()
这样,即使发生异常,程序也能继续运行。
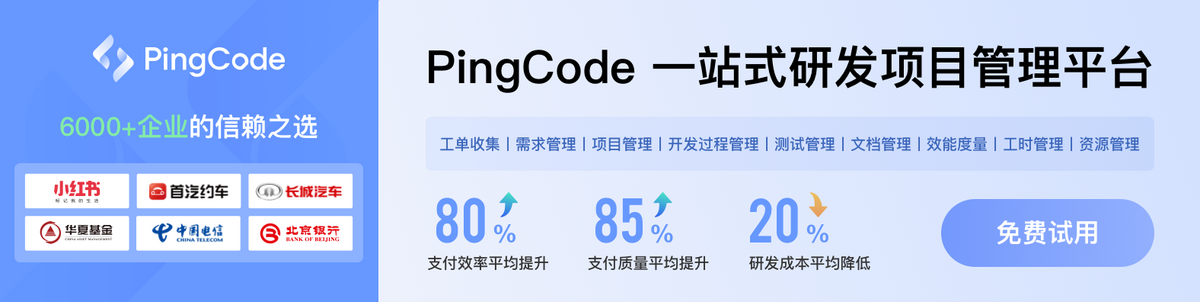