Python的super()函数用于调用父类的方法、在多重继承时管理复杂的继承关系、增强代码的可读性。 其中,调用父类的方法是super()函数最常见的用法。通过super(),我们可以避免显式地引用父类,从而使代码更灵活和可维护。接下来,我们将详细探讨如何在不同情况下使用super()函数。
一、定义和基本用法
super()函数是Python内置的一个函数,用于调用父类的一个方法。通常,我们在子类中调用父类的方法时会使用super()函数。其基本语法如下:
super().method_name(arguments)
在单继承的情况下,super()函数会返回父类的一个临时对象,这样我们就可以调用父类的方法。来看一个简单的例子:
class Parent:
def __init__(self, name):
self.name = name
def display(self):
print("Parent name:", self.name)
class Child(Parent):
def __init__(self, name, age):
super().__init__(name)
self.age = age
def display(self):
super().display()
print("Child age:", self.age)
child = Child("John", 12)
child.display()
在这个例子中,Child类继承了Parent类,并在其构造函数和display方法中使用了super()函数来调用父类的对应方法。
二、在多重继承中的使用
在多重继承中,super()函数的使用会变得更加复杂。Python使用C3线性化算法来确定方法解析顺序(MRO),这确保了在多重继承中的一致性和可预测性。
class A:
def __init__(self):
print("A.__init__")
super().__init__()
class B:
def __init__(self):
print("B.__init__")
super().__init__()
class C(A, B):
def __init__(self):
print("C.__init__")
super().__init__()
c = C()
在这个例子中,class C继承了class A和class B。调用super()会按照MRO的顺序调用父类的方法。运行结果将是:
C.__init__
A.__init__
B.__init__
这展示了如何在多重继承中使用super()函数来确保所有父类的初始化方法都被调用。
三、在不同方法中使用super()
super()函数不仅可以用于__init__方法中,还可以用于其他任何方法中。例如:
class Base:
def greet(self):
print("Hello from Base")
class Derived(Base):
def greet(self):
super().greet()
print("Hello from Derived")
derived = Derived()
derived.greet()
在这个例子中,Derived类重写了greet方法,并在其中使用super()函数来调用Base类的greet方法。运行结果将是:
Hello from Base
Hello from Derived
这展示了如何在不同的方法中使用super()函数来调用父类的方法。
四、在属性访问中使用super()
super()函数不仅可以用于方法调用,还可以用于属性访问。例如:
class Base:
def __init__(self, value):
self._value = value
@property
def value(self):
return self._value
class Derived(Base):
@property
def value(self):
return super().value + 10
derived = Derived(5)
print(derived.value)
在这个例子中,Derived类重写了value属性,并在其中使用super()函数来访问Base类的value属性。运行结果将是:
15
这展示了如何在属性访问中使用super()函数来访问父类的属性。
五、在多个继承层次中的使用
super()函数可以在多个继承层次中使用,以确保所有父类的方法都被正确调用。例如:
class A:
def method(self):
print("A.method")
super().method()
class B:
def method(self):
print("B.method")
super().method()
class C(A, B):
def method(self):
print("C.method")
super().method()
c = C()
c.method()
在这个例子中,class C继承了class A和class B,并在其method方法中使用super()函数来调用父类的方法。运行结果将是:
C.method
A.method
B.method
这展示了如何在多个继承层次中使用super()函数来确保所有父类的方法都被正确调用。
六、在协同方法中使用super()
super()函数在协同方法中也可以使用,例如在__enter__和__exit__方法中:
class Resource:
def __enter__(self):
print("Resource.__enter__")
return self
def __exit__(self, exc_type, exc_value, traceback):
print("Resource.__exit__")
class ManagedResource(Resource):
def __enter__(self):
super().__enter__()
print("ManagedResource.__enter__")
return self
def __exit__(self, exc_type, exc_value, traceback):
super().__exit__(exc_type, exc_value, traceback)
print("ManagedResource.__exit__")
with ManagedResource() as mr:
print("Using managed resource")
在这个例子中,ManagedResource类继承了Resource类,并在其__enter__和__exit__方法中使用super()函数来调用父类的对应方法。运行结果将是:
Resource.__enter__
ManagedResource.__enter__
Using managed resource
Resource.__exit__
ManagedResource.__exit__
这展示了如何在协同方法中使用super()函数来确保所有父类的方法都被正确调用。
七、在多层嵌套继承中的使用
在复杂的多层嵌套继承中,使用super()函数可以帮助我们更好地管理方法调用顺序。例如:
class GrandParent:
def greet(self):
print("Hello from GrandParent")
class Parent(GrandParent):
def greet(self):
super().greet()
print("Hello from Parent")
class Child(Parent):
def greet(self):
super().greet()
print("Hello from Child")
child = Child()
child.greet()
在这个例子中,Child类继承了Parent类,Parent类又继承了GrandParent类,并在各自的greet方法中使用super()函数来调用父类的方法。运行结果将是:
Hello from GrandParent
Hello from Parent
Hello from Child
这展示了如何在多层嵌套继承中使用super()函数来确保所有父类的方法都被正确调用。
八、在动态类创建中的使用
super()函数还可以在动态类创建中使用,例如通过type函数创建类:
Base = type('Base', (object,), {
'greet': lambda self: print("Hello from Base")
})
Derived = type('Derived', (Base,), {
'greet': lambda self: (super(type(self), self).greet(), print("Hello from Derived"))
})
derived = Derived()
derived.greet()
在这个例子中,我们通过type函数动态创建了Base类和Derived类,并在Derived类的greet方法中使用super()函数来调用Base类的greet方法。运行结果将是:
Hello from Base
Hello from Derived
这展示了如何在动态类创建中使用super()函数来调用父类的方法。
九、在元类中的使用
元类是用于创建类的类,super()函数在元类中也可以使用。例如:
class Meta(type):
def __new__(cls, name, bases, dct):
print("Creating class", name)
return super().__new__(cls, name, bases, dct)
class Base(metaclass=Meta):
pass
class Derived(Base):
pass
在这个例子中,Meta类是一个元类,并在其__new__方法中使用super()函数来调用父类的__new__方法。运行结果将是:
Creating class Base
Creating class Derived
这展示了如何在元类中使用super()函数来调用父类的方法。
十、在非绑定方法中的使用
非绑定方法是那些没有绑定到实例的方法,super()函数在非绑定方法中也可以使用。例如:
class Base:
@staticmethod
def greet():
print("Hello from Base")
class Derived(Base):
@staticmethod
def greet():
super(Derived, Derived).greet()
print("Hello from Derived")
Derived.greet()
在这个例子中,Derived类重写了Base类的greet方法,并在其中使用super()函数来调用Base类的greet方法。运行结果将是:
Hello from Base
Hello from Derived
这展示了如何在非绑定方法中使用super()函数来调用父类的方法。
十一、在装饰器中使用super()
装饰器是一种用于修改函数或方法行为的函数,super()函数在装饰器中也可以使用。例如:
def log_method_call(method):
def wrapper(self, *args, kwargs):
print(f"Calling method {method.__name__}")
return method(self, *args, kwargs)
return wrapper
class Base:
@log_method_call
def greet(self):
print("Hello from Base")
class Derived(Base):
@log_method_call
def greet(self):
super().greet()
print("Hello from Derived")
derived = Derived()
derived.greet()
在这个例子中,我们定义了一个log_method_call装饰器,并在Base类和Derived类的greet方法中使用它。Derived类的greet方法还使用了super()函数来调用Base类的greet方法。运行结果将是:
Calling method greet
Hello from Base
Calling method greet
Hello from Derived
这展示了如何在装饰器中使用super()函数来调用父类的方法。
十二、在混合类中的使用
混合类(Mixin)是一种用于将多个类的功能组合在一起的技术,super()函数在混合类中也可以使用。例如:
class Base:
def greet(self):
print("Hello from Base")
class Mixin:
def greet(self):
super().greet()
print("Hello from Mixin")
class Derived(Mixin, Base):
def greet(self):
super().greet()
print("Hello from Derived")
derived = Derived()
derived.greet()
在这个例子中,Derived类继承了Mixin类和Base类,并在其greet方法中使用super()函数来调用父类的方法。运行结果将是:
Hello from Base
Hello from Mixin
Hello from Derived
这展示了如何在混合类中使用super()函数来调用父类的方法。
十三、在异常处理中的使用
super()函数在异常处理中的使用也非常重要,例如在__exit__方法中:
class Resource:
def __enter__(self):
print("Resource.__enter__")
return self
def __exit__(self, exc_type, exc_value, traceback):
print("Resource.__exit__")
class ManagedResource(Resource):
def __enter__(self):
super().__enter__()
print("ManagedResource.__enter__")
return self
def __exit__(self, exc_type, exc_value, traceback):
try:
return super().__exit__(exc_type, exc_value, traceback)
finally:
print("ManagedResource.__exit__")
with ManagedResource() as mr:
print("Using managed resource")
在这个例子中,ManagedResource类继承了Resource类,并在其__enter__和__exit__方法中使用super()函数来调用父类的对应方法。运行结果将是:
Resource.__enter__
ManagedResource.__enter__
Using managed resource
Resource.__exit__
ManagedResource.__exit__
这展示了如何在异常处理中的__exit__方法中使用super()函数来确保所有父类的方法都被正确调用。
十四、在组合设计模式中的使用
在组合设计模式中,super()函数可以帮助我们调用父类的方法,例如在一个组合对象中:
class Component:
def operation(self):
print("Component.operation")
class Decorator(Component):
def __init__(self, component):
self._component = component
def operation(self):
super().operation()
self._component.operation()
class ConcreteComponent(Component):
def operation(self):
print("ConcreteComponent.operation")
component = ConcreteComponent()
decorator = Decorator(component)
decorator.operation()
在这个例子中,Decorator类继承了Component类,并在其operation方法中使用super()函数来调用父类的operation方法。运行结果将是:
Component.operation
ConcreteComponent.operation
这展示了如何在组合设计模式中使用super()函数来调用父类的方法。
十五、在单例模式中的使用
单例模式是一种确保一个类只有一个实例的设计模式,super()函数在单例模式中也可以使用。例如:
class SingletonMeta(type):
_instance = None
def __call__(cls, *args, kwargs):
if cls._instance is None:
cls._instance = super().__call__(*args, kwargs)
return cls._instance
class Singleton(metaclass=SingletonMeta):
pass
singleton1 = Singleton()
singleton2 = Singleton()
print(singleton1 is singleton2)
在这个例子中,SingletonMeta是一个元类,并在其__call__方法中使用super()函数来调用父类的__call__方法。运行结果将是:
True
这展示了如何在单例模式中使用super()函数来调用父类的方法。
通过以上多个案例,我们详细探讨了super()函数在不同情况下的使用。通过理解这些案例,您可以更好地掌握如何在实际项目中使用super()函数,增强代码的可读性和可维护性。
相关问答FAQs:
什么是Python中的super函数?
super函数是Python中的一个内置函数,用于调用父类(超类)的方法。它可以帮助开发者在子类中访问父类的属性和方法,从而实现代码的重用和更好的可维护性。
在使用super函数时,有哪些注意事项?
使用super函数时,需确保在类中正确使用,特别是在多重继承的情况下。确保调用super时提供正确的参数,以确保其能正确定位到父类。此外,了解类的解析顺序(MRO)对于理解super的行为非常重要。
如何在子类中覆盖父类的方法并使用super函数?
在子类中定义与父类同名的方法时,可以在子类的方法中使用super函数来调用父类的方法。这样可以在子类中扩展或修改父类的功能,而不完全覆盖。示例代码如下:
class Parent:
def show(self):
print("Parent method")
class Child(Parent):
def show(self):
super().show() # 调用父类的show方法
print("Child method")
child = Child()
child.show()
在这个例子中,Child类中的show方法调用了Parent类的show方法,确保了父类逻辑的执行。
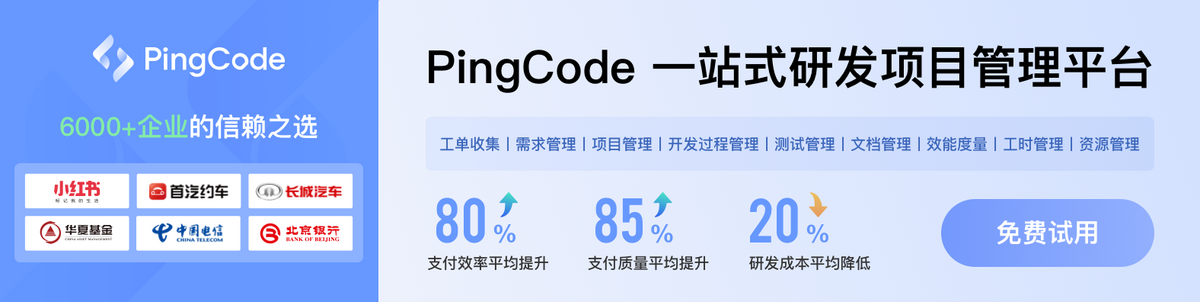