Python可以通过多种方式实现自动发送短信,使用Twilio API、使用SMPP协议、使用SMTP协议发送邮件到短信网关、使用第三方短信服务提供商的API。其中,使用Twilio API是一种非常流行和简单的方式。Twilio是一个提供电话和短信服务的云通信平台,使用它可以非常方便地在Python程序中集成短信发送功能。下面详细介绍如何使用Twilio API发送短信。
一、使用Twilio API
1、注册并获取Twilio账户
首先,你需要在Twilio官网注册一个账户,并获取你的账户SID和身份验证令牌(Auth Token)。这些信息将在你使用Twilio API时需要用到。注册成功后,Twilio会提供一个试用号码,你可以用这个号码来发送短信。
2、安装Twilio库
在使用Twilio API之前,你需要先安装Twilio的Python库。你可以使用pip进行安装:
pip install twilio
3、编写Python代码
安装完成后,你可以使用以下代码发送短信:
from twilio.rest import Client
你的 Account SID 和 Auth Token
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
发送短信
message = client.messages.create(
body="Hello, this is a test message from Twilio!",
from_='+1234567890', # Twilio提供的试用号码
to='+0987654321' # 目标电话号码
)
print(message.sid)
在上面的代码中,Client
类用来创建一个Twilio客户端,messages.create
方法用来创建并发送短信。你需要替换your_account_sid
和your_auth_token
为你自己Twilio账户的SID和Auth Token,替换+1234567890
和+0987654321
为你的Twilio号码和目标号码。
二、使用SMPP协议
SMPP(Short Message Peer-to-Peer)是一种用于发送和接收短信的协议。你可以使用Python的pysmpp
库来实现这一功能。
1、安装pysmpp库
使用pip安装pysmpp库:
pip install pysmpp
2、编写Python代码
以下是一个使用SMPP发送短信的示例:
import smpplib.client
import smpplib.consts
import smpplib.gsm
import smpplib.pdu
创建SMPP客户端
client = smpplib.client.Client('smpp.server.address', 2775)
绑定到SMPP服务器
client.connect()
client.bind_transmitter(system_id='your_system_id', password='your_password')
发送短信
parts, encoding_flag, msg_type_flag = smpplib.gsm.make_parts('Hello, this is a test message!')
for part in parts:
pdu = client.send_message(
source_addr_ton=smpplib.consts.SMPP_TON_INTL,
source_addr_npi=smpplib.consts.SMPP_NPI_ISDN,
source_addr='source_number',
dest_addr_ton=smpplib.consts.SMPP_TON_INTL,
dest_addr_npi=smpplib.consts.SMPP_NPI_ISDN,
destination_addr='destination_number',
short_message=part,
data_coding=encoding_flag,
esm_class=msg_type_flag,
)
print(pdu.sequence)
client.unbind()
client.disconnect()
在上面的代码中,smpplib.client.Client
类用来创建一个SMPP客户端,client.send_message
方法用来创建并发送短信。你需要替换smpp.server.address
、your_system_id
、your_password
、source_number
和destination_number
为你自己的SMPP服务器地址、系统ID、密码、源号码和目标号码。
三、使用SMTP协议发送邮件到短信网关
一些移动运营商提供了将电子邮件转换为短信的服务。你可以使用Python的smtplib
库来发送电子邮件到这些网关,从而实现短信发送。
1、编写Python代码
以下是一个使用SMTP发送邮件到短信网关的示例:
import smtplib
from email.mime.text import MIMEText
创建邮件内容
msg = MIMEText('Hello, this is a test message!')
msg['Subject'] = 'Test Message'
msg['From'] = 'your_email@example.com'
msg['To'] = 'destination_number@sms_gateway.com'
发送邮件
with smtplib.SMTP('smtp.example.com', 587) as server:
server.starttls()
server.login('your_email@example.com', 'your_email_password')
server.sendmail('your_email@example.com', 'destination_number@sms_gateway.com', msg.as_string())
在上面的代码中,smtplib.SMTP
类用来创建一个SMTP客户端,server.sendmail
方法用来发送邮件。你需要替换your_email@example.com
、your_email_password
、smtp.example.com
、destination_number
和sms_gateway.com
为你自己的电子邮件地址、电子邮件密码、SMTP服务器地址、目标号码和短信网关域名。
四、使用第三方短信服务提供商的API
除了Twilio,还有许多其他第三方短信服务提供商,如Nexmo、Plivo、MessageBird等。你可以根据自己的需求选择合适的服务提供商,并使用他们提供的API来实现短信发送。
1、注册并获取账户信息
首先,你需要在服务提供商的官网注册一个账户,并获取API密钥和其他必要的信息。
2、安装相应的Python库
大多数服务提供商都会提供相应的Python库,你可以使用pip进行安装。例如,Nexmo的Python库可以通过以下命令安装:
pip install nexmo
3、编写Python代码
以下是一个使用Nexmo API发送短信的示例:
import nexmo
创建Nexmo客户端
client = nexmo.Client(key='your_api_key', secret='your_api_secret')
发送短信
response = client.send_message({
'from': 'NEXMO',
'to': 'destination_number',
'text': 'Hello, this is a test message!'
})
if response['messages'][0]['status'] == '0':
print('Message sent successfully.')
else:
print(f"Message failed with error: {response['messages'][0]['error-text']}")
在上面的代码中,nexmo.Client
类用来创建一个Nexmo客户端,client.send_message
方法用来创建并发送短信。你需要替换your_api_key
、your_api_secret
和destination_number
为你自己的Nexmo API密钥、API密钥、API秘密和目标号码。
五、总结
通过上面的介绍,我们可以看到,Python提供了多种方式来实现自动发送短信。使用Twilio API是一种非常流行和简单的方式,你只需要注册一个Twilio账户,安装Twilio库,并编写几行代码即可实现短信发送。此外,你还可以选择使用SMPP协议、使用SMTP协议发送邮件到短信网关、使用第三方短信服务提供商的API等方式来实现自动发送短信。每种方式都有其优缺点,你可以根据自己的需求选择合适的方式。
相关问答FAQs:
如何使用Python发送短信?
使用Python发送短信通常需要利用第三方服务,如Twilio、Nexmo或其他短信网关。这些服务提供API,可以通过HTTP请求与之交互。您需要注册一个账户,获取API密钥,并按照文档说明进行集成。示例代码通常包括导入相应的库、设置API凭证和发送短信的函数调用。
发送短信的过程中需要注意哪些事项?
在发送短信时,有几个关键点需要关注。首先,确保您遵守相关法律法规,尤其是关于隐私和用户同意的部分。其次,了解短信发送的费用结构,不同的服务商可能会有不同的计费方式。最后,确保您的代码具备异常处理机制,以便在发送失败时能够进行相应的处理。
是否可以在Python中定时发送短信?
可以实现定时发送短信的功能。您可以使用Python的schedule
库或time
模块结合循环来设置定时任务。通过这些工具,您可以设定特定的时间间隔,自动调用发送短信的函数。此外,还可以结合其他调度工具,如cron
(Linux系统)或Windows Task Scheduler,来实现更复杂的调度需求。
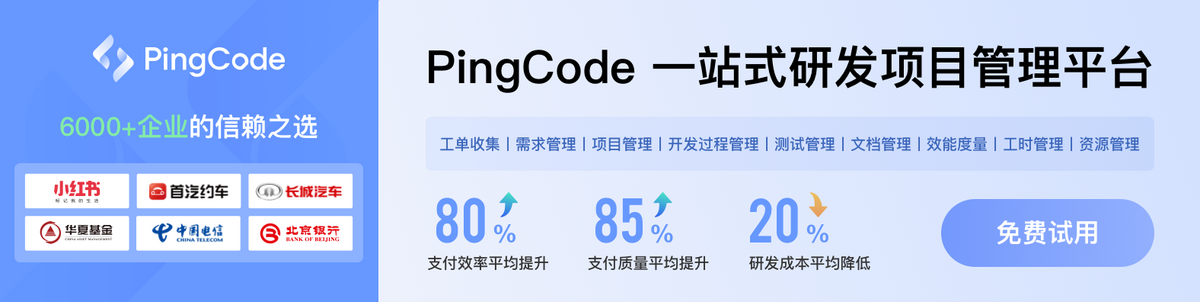