在Python中,可以使用第三方库来下载BitTorrent文件(BT)。使用libtorrent
库、使用requests
库下载种子文件、解析并使用libtorrent
进行下载是常见的方法。下面将详细介绍使用libtorrent
库的步骤。
一、安装必要的库
- 安装
libtorrent
库
首先,你需要安装libtorrent
库,可以通过pip
来安装:
pip install python-libtorrent
二、使用libtorrent
下载BT文件
1、导入必要的库
import libtorrent as lt
import time
import os
2、设置会话和下载参数
# 创建会话
ses = lt.session()
ses.listen_on(6881, 6891)
读取种子文件
info = lt.torrent_info('path_to_your_torrent_file.torrent')
添加种子
h = ses.add_torrent({
'ti': info,
'save_path': 'path_where_you_want_to_save_files'
})
3、下载过程
# 获取种子状态
print('starting', h.name())
while not h.is_seed():
s = h.status()
print('%.2f%% complete (down: %.1f kB/s up: %.1f kB/s peers: %d) %s' % (
s.progress * 100, s.download_rate / 1000, s.upload_rate / 1000,
s.num_peers, s.state))
time.sleep(1)
三、使用requests
库下载种子文件
1、安装requests
库
pip install requests
2、下载种子文件
import requests
url = 'http://example.com/path_to_your_torrent_file.torrent'
response = requests.get(url)
with open('path_to_save_torrent_file.torrent', 'wb') as f:
f.write(response.content)
四、解析种子文件并使用libtorrent
进行下载
1、解析种子文件
import libtorrent as lt
info = lt.torrent_info('path_to_save_torrent_file.torrent')
2、添加种子和下载文件
ses = lt.session()
ses.listen_on(6881, 6891)
h = ses.add_torrent({
'ti': info,
'save_path': 'path_where_you_want_to_save_files'
})
print('starting', h.name())
while not h.is_seed():
s = h.status()
print('%.2f%% complete (down: %.1f kB/s up: %.1f kB/s peers: %d) %s' % (
s.progress * 100, s.download_rate / 1000, s.upload_rate / 1000,
s.num_peers, s.state))
time.sleep(1)
五、下载完成后的处理
1、下载完成后的处理
当下载完成后,可以对下载的文件进行一些处理,比如解压、移动到特定目录等。
if h.is_seed():
print('Download complete')
# 对下载的文件进行处理
# 如解压、移动到特定目录等
2、示例代码
完整示例代码如下:
import libtorrent as lt
import time
import requests
下载种子文件
url = 'http://example.com/path_to_your_torrent_file.torrent'
response = requests.get(url)
torrent_file_path = 'path_to_save_torrent_file.torrent'
with open(torrent_file_path, 'wb') as f:
f.write(response.content)
解析种子文件
info = lt.torrent_info(torrent_file_path)
创建会话并添加种子
ses = lt.session()
ses.listen_on(6881, 6891)
h = ses.add_torrent({
'ti': info,
'save_path': 'path_where_you_want_to_save_files'
})
print('starting', h.name())
下载过程
while not h.is_seed():
s = h.status()
print('%.2f%% complete (down: %.1f kB/s up: %.1f kB/s peers: %d) %s' % (
s.progress * 100, s.download_rate / 1000, s.upload_rate / 1000,
s.num_peers, s.state))
time.sleep(1)
下载完成后的处理
if h.is_seed():
print('Download complete')
# 对下载的文件进行处理
# 如解压、移动到特定目录等
六、总结
通过以上步骤,你可以使用Python下载BT文件。使用libtorrent
库、使用requests
库下载种子文件、解析并使用libtorrent
进行下载是常见的方法。libtorrent
库是一个功能强大且易于使用的BitTorrent库,能够帮助你快速实现BT文件的下载功能。在实际使用中,可以根据具体需求进行相应的调整和优化。
相关问答FAQs:
如何用Python编写一个BT下载器?
要编写一个BT下载器,您可以使用像libtorrent
这样的库。这个库提供了丰富的功能来处理BitTorrent协议。您需要安装python-libtorrent
,然后可以创建一个下载会话,添加Torrent文件或磁力链接,最后开始下载。确保您熟悉异步编程,因为下载过程通常是异步的。
使用Python下载BT文件时需要注意哪些法律问题?
在下载BT文件时,您应确保遵守当地的版权法律。某些内容可能受版权保护,下载这些内容可能会导致法律责任。建议在下载前确认文件的版权状态,尽量选择开源或公共领域的资源,避免侵权。
有哪些Python库可以帮助实现BT下载功能?
除了libtorrent
,还有其他一些库可以实现BT下载功能。例如,transmissionrpc
可以通过Transmission客户端进行BT下载,Deluge
也提供了Python API来操控其下载任务。这些库各有特点,您可以根据自己的需求选择合适的库来实现BT下载。
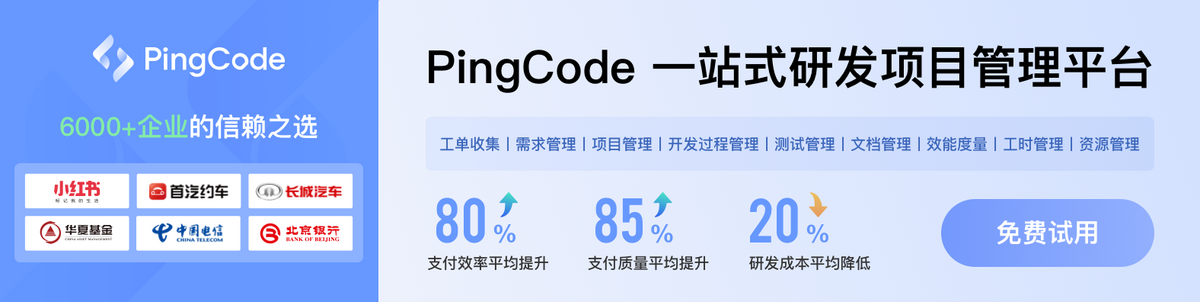