使用Python保存网页图片的方法有多种,可以通过requests
库获取图像数据、BeautifulSoup
解析网页中的图像URL、os
库管理文件系统。常用方法包括:使用requests
库下载并保存图片、使用urllib
库下载图片、使用selenium
库处理动态加载的图片。下面将详细介绍如何使用这些方法保存网页图片。
一、使用 requests
库下载并保存图片
requests
库是一个简单且功能强大的HTTP库,可以方便地下载网页上的图片。
import requests
def download_image(url, file_name):
response = requests.get(url)
if response.status_code == 200:
with open(file_name, 'wb') as file:
file.write(response.content)
print(f"Image saved as {file_name}")
else:
print(f"Failed to retrieve image from {url}")
示例
image_url = 'https://example.com/image.jpg'
download_image(image_url, 'downloaded_image.jpg')
在这个示例中,通过requests.get
方法获取图片数据,然后将数据写入文件。如果状态码为200,表示请求成功,图片会被保存到本地。
二、使用 urllib
库下载图片
urllib
库是Python内置的库,不需要额外安装。它也可以用于下载并保存图片。
import urllib.request
def download_image(url, file_name):
try:
urllib.request.urlretrieve(url, file_name)
print(f"Image saved as {file_name}")
except Exception as e:
print(f"Failed to retrieve image from {url}. Error: {e}")
示例
image_url = 'https://example.com/image.jpg'
download_image(image_url, 'downloaded_image.jpg')
urllib.request.urlretrieve
方法直接下载图片并保存到指定路径,这种方法非常简洁。
三、使用 BeautifulSoup
解析网页中的图片URL
有时,图片URL嵌套在网页的HTML中。可以使用BeautifulSoup
解析网页,提取图片URL。
import requests
from bs4 import BeautifulSoup
def fetch_image_urls(page_url):
response = requests.get(page_url)
soup = BeautifulSoup(response.content, 'html.parser')
img_tags = soup.find_all('img')
img_urls = [img['src'] for img in img_tags if 'src' in img.attrs]
return img_urls
def download_image(url, file_name):
response = requests.get(url)
if response.status_code == 200:
with open(file_name, 'wb') as file:
file.write(response.content)
print(f"Image saved as {file_name}")
else:
print(f"Failed to retrieve image from {url}")
示例
page_url = 'https://example.com'
image_urls = fetch_image_urls(page_url)
for i, img_url in enumerate(image_urls):
download_image(img_url, f'image_{i}.jpg')
通过BeautifulSoup
解析网页HTML,提取出所有<img>
标签的src
属性,即图片URL。然后使用requests
库下载并保存这些图片。
四、使用 selenium
处理动态加载的图片
有些网页使用JavaScript动态加载图片,requests
库无法直接获取。这时可以使用selenium
自动化工具加载网页并提取图片URL。
from selenium import webdriver
import time
import os
def fetch_image_urls(page_url):
driver = webdriver.Chrome()
driver.get(page_url)
time.sleep(5) # 等待页面加载
img_elements = driver.find_elements_by_tag_name('img')
img_urls = [img.get_attribute('src') for img in img_elements]
driver.quit()
return img_urls
def download_image(url, file_name):
response = requests.get(url)
if response.status_code == 200:
with open(file_name, 'wb') as file:
file.write(response.content)
print(f"Image saved as {file_name}")
else:
print(f"Failed to retrieve image from {url}")
示例
page_url = 'https://example.com'
image_urls = fetch_image_urls(page_url)
for i, img_url in enumerate(image_urls):
download_image(img_url, f'image_{i}.jpg')
使用selenium
库控制浏览器加载网页,找到所有<img>
标签,提取src
属性。这种方法可以处理动态加载的图片。
五、保存图片到特定目录
在保存图片时,可以使用os
库将图片保存到特定目录中。
import os
import requests
def download_image(url, directory, file_name):
if not os.path.exists(directory):
os.makedirs(directory)
file_path = os.path.join(directory, file_name)
response = requests.get(url)
if response.status_code == 200:
with open(file_path, 'wb') as file:
file.write(response.content)
print(f"Image saved as {file_path}")
else:
print(f"Failed to retrieve image from {url}")
示例
image_url = 'https://example.com/image.jpg'
download_image(image_url, 'images', 'downloaded_image.jpg')
在这个示例中,首先检查目标目录是否存在,如果不存在则创建目录。然后将图片保存到指定目录中。
六、多线程并发下载图片
为了提高下载效率,可以使用多线程并发下载图片。
import requests
from concurrent.futures import ThreadPoolExecutor
def download_image(url, file_name):
response = requests.get(url)
if response.status_code == 200:
with open(file_name, 'wb') as file:
file.write(response.content)
print(f"Image saved as {file_name}")
else:
print(f"Failed to retrieve image from {url}")
def download_images(image_urls):
with ThreadPoolExecutor(max_workers=5) as executor:
for i, url in enumerate(image_urls):
executor.submit(download_image, url, f'image_{i}.jpg')
示例
image_urls = ['https://example.com/image1.jpg', 'https://example.com/image2.jpg']
download_images(image_urls)
使用ThreadPoolExecutor
创建线程池,并发下载图片,提高下载效率。
七、总结
在使用Python保存网页图片时,可以根据具体需求选择合适的方法。如果图片URL是静态的,可以直接使用requests
或urllib
库下载图片。如果图片URL嵌套在网页HTML中,可以使用BeautifulSoup
解析。如果网页使用JavaScript动态加载图片,可以使用selenium
处理。为了提高下载效率,可以使用多线程并发下载图片。通过这些方法,可以高效地保存网页上的图片。
相关问答FAQs:
如何使用Python下载特定网页上的图片?
可以使用Python的requests库和BeautifulSoup库来抓取网页内容,找到图片的URL,然后下载它们。首先,使用requests库获取网页的HTML内容,再利用BeautifulSoup解析HTML,提取出所有的图片链接,最后使用requests库下载这些图片。示例代码如下:
import requests
from bs4 import BeautifulSoup
import os
url = '目标网页的URL'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
images = soup.find_all('img')
if not os.path.exists('下载的图片'):
os.makedirs('下载的图片')
for img in images:
img_url = img['src']
if not img_url.startswith('http'):
img_url = url + img_url # 处理相对路径
img_response = requests.get(img_url)
img_name = os.path.join('下载的图片', img_url.split('/')[-1])
with open(img_name, 'wb') as f:
f.write(img_response.content)
在Python中如何处理下载的图片格式问题?
在下载图片时,确保提取的URL是完整的,并且包含文件扩展名(如.jpg、.png等)。在保存时,使用适当的文件格式进行命名。如果图片没有扩展名,可以通过requests库的Content-Type来判断文件类型,进而决定保存时的格式。
下载网页图片时,如何处理反爬虫机制?
许多网站会实施反爬虫措施来阻止自动化下载。为规避这些限制,可以在请求头中添加一些常见的浏览器信息,例如User-Agent。通过随机化请求间隔和使用代理IP等方法,也能够减少被封禁的风险。示例代码如下:
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/87.0.4280.88 Safari/537.36'}
response = requests.get(url, headers=headers)
通过这些方法,您可以有效下载网页上的图片,并应对可能遇到的技术挑战。
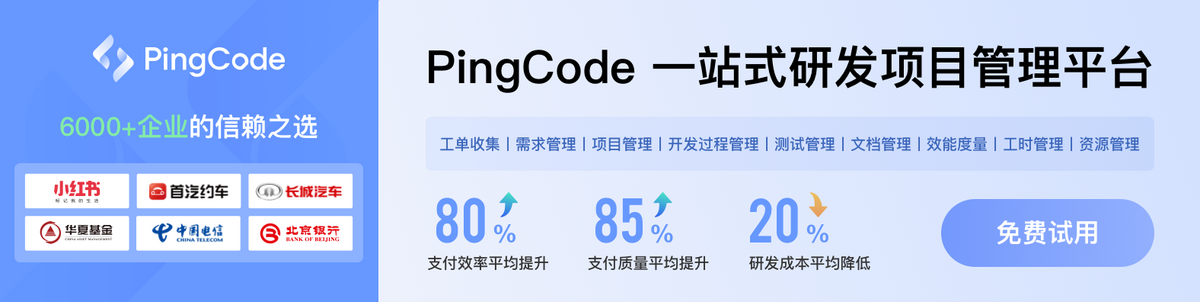