在Python中打开exe文件有几种方法,使用subprocess模块、使用os模块、使用ctypes库。最常用的是subprocess模块,因为它提供了更多的功能和更高的灵活性。下面将详细介绍如何使用subprocess模块打开exe文件。
一、使用subprocess模块
subprocess模块允许你生成新的进程、连接到它们的输入/输出/错误管道,并获取它们的返回码。它将创建和管理子进程的任务变得更简单。
1. 简单打开exe文件
import subprocess
指定exe文件的路径
exe_path = r'C:\path\to\your\file.exe'
使用subprocess.Popen方法打开exe文件
subprocess.Popen(exe_path)
2. 捕获输出
有时你可能需要捕获exe文件的输出,此时可以使用subprocess.run方法。
import subprocess
exe_path = r'C:\path\to\your\file.exe'
result = subprocess.run([exe_path], capture_output=True, text=True)
输出返回的stdout和stderr
print("stdout:", result.stdout)
print("stderr:", result.stderr)
二、使用os模块
os模块提供了与操作系统进行交互的简单方法,可以使用os.system或os.startfile方法打开exe文件。
1. 使用os.system
os.system执行系统命令,类似于在命令行中输入命令。
import os
exe_path = r'C:\path\to\your\file.exe'
os.system(exe_path)
2. 使用os.startfile
os.startfile在Windows系统中特别有用。
import os
exe_path = r'C:\path\to\your\file.exe'
os.startfile(exe_path)
三、使用ctypes库
ctypes库允许调用系统的动态链接库,可以直接调用ShellExecute函数打开exe文件。
import ctypes
调用ShellExecute函数
ctypes.windll.shell32.ShellExecuteW(None, "open", r"C:\path\to\your\file.exe", None, None, 1)
四、总结
在Python中打开exe文件的方法有多种,subprocess模块提供了更高的灵活性和更多的功能选项,是最常用的方法。如果你只需要简单地打开一个文件,os模块的方法也很方便。如果需要更底层的操作,ctypes库是一个很好的选择。根据具体的需求选择最适合的方法。
五、详细描述subprocess模块
1. subprocess.run
subprocess.run是Python 3.5中引入的,能够简化子进程的调用并提供更多功能。
import subprocess
exe_path = r'C:\path\to\your\file.exe'
result = subprocess.run([exe_path], capture_output=True, text=True)
print("Return code:", result.returncode)
print("stdout:", result.stdout)
print("stderr:", result.stderr)
- capture_output: 如果设置为True,stdout和stderr将被捕获。
- text: 如果设置为True,输出将以字符串形式返回,而不是字节。
2. subprocess.Popen
subprocess.Popen提供了更多的灵活性,可以异步执行子进程并与其进行交互。
import subprocess
exe_path = r'C:\path\to\your\file.exe'
process = subprocess.Popen(exe_path, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
等待子进程完成
stdout, stderr = process.communicate()
print("stdout:", stdout)
print("stderr:", stderr)
- stdout: 子进程的标准输出。
- stderr: 子进程的标准错误。
- text: 如果设置为True,输出将以字符串形式返回。
3. 与子进程交互
有时候需要与子进程进行交互,可以使用Popen对象的stdin、stdout、stderr属性。
import subprocess
exe_path = r'C:\path\to\your\file.exe'
process = subprocess.Popen(exe_path, stdin=subprocess.PIPE, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
向子进程发送输入
process.stdin.write('input data\n')
process.stdin.flush()
读取子进程的输出
stdout, stderr = process.communicate()
print("stdout:", stdout)
print("stderr:", stderr)
4. 异常处理
在调用子进程时,可能会遇到各种异常,需要进行处理。
import subprocess
exe_path = r'C:\path\to\your\file.exe'
try:
result = subprocess.run([exe_path], check=True, capture_output=True, text=True)
print("stdout:", result.stdout)
print("stderr:", result.stderr)
except subprocess.CalledProcessError as e:
print(f"Error: {e}")
except FileNotFoundError:
print("File not found.")
except Exception as e:
print(f"Unexpected error: {e}")
- CalledProcessError: 如果子进程返回非零退出状态,将引发此异常。
- FileNotFoundError: 如果exe文件不存在,将引发此异常。
- Exception: 捕获所有其他异常。
六、os模块详细描述
1. os.system
os.system在子进程中执行系统命令,类似于在命令行中输入命令。
import os
exe_path = r'C:\path\to\your\file.exe'
exit_code = os.system(exe_path)
print(f"Exit code: {exit_code}")
- exit_code: 子进程的退出状态。
2. os.startfile
os.startfile在Windows系统中特别有用,它可以打开与文件类型关联的默认应用程序。
import os
exe_path = r'C:\path\to\your\file.exe'
os.startfile(exe_path)
七、ctypes库详细描述
1. ShellExecute
ShellExecute是Windows API函数,用于执行各种操作,包括打开文件和启动程序。
import ctypes
调用ShellExecute函数
ctypes.windll.shell32.ShellExecuteW(None, "open", r"C:\path\to\your\file.exe", None, None, 1)
- windll.shell32.ShellExecuteW: 调用Windows API的ShellExecuteW函数。
- None: 父窗口句柄,通常为None。
- "open": 动作,表示打开文件。
- 路径: 要打开的文件路径。
- 参数: 命令行参数,通常为None。
- 目录: 工作目录,通常为None。
- 显示标志: 窗口显示标志,1表示正常显示。
八、总结与建议
在Python中打开exe文件有多种方法,subprocess模块提供了最高的灵活性和功能,是最常用的方法。os模块提供了简单的方法,如果你只需要简单地打开文件,os.system或os.startfile是很好的选择。ctypes库允许调用Windows API,适用于更底层的操作。
根据具体需求选择最合适的方法,可以确保代码的可读性和可维护性。通过本文的详细介绍,相信你能够在Python中灵活地打开并控制exe文件。
相关问答FAQs:
如何使用Python打开一个exe文件?
要在Python中打开exe文件,可以使用内置的subprocess
模块。这个模块允许你启动外部程序,并与其进行交互。下面是一个简单的示例代码:
import subprocess
# 指定exe文件的路径
subprocess.run(['path_to_your_executable.exe'])
只需将path_to_your_executable.exe
替换为你要打开的exe文件的具体路径。
Python打开exe文件时可以传递参数吗?
是的,可以在使用subprocess.run()
时传递参数。例如,如果你的exe文件需要接收命令行参数,可以在列表中添加这些参数:
subprocess.run(['path_to_your_executable.exe', 'arg1', 'arg2'])
这样,arg1
和arg2
将作为参数传递给可执行文件。
打开exe文件时如何处理输出和错误信息?
在使用subprocess
模块时,可以捕获程序的输出和错误信息。例如:
result = subprocess.run(['path_to_your_executable.exe'], capture_output=True, text=True)
print("标准输出:", result.stdout)
print("错误输出:", result.stderr)
使用capture_output=True
可以捕获标准输出和错误输出,text=True
会将其作为字符串返回,方便后续处理。
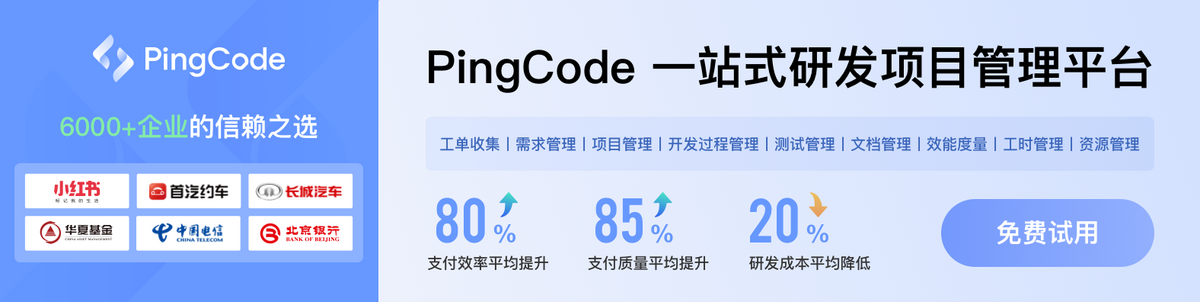