在Python中显示得分的方法有很多,这取决于你所使用的具体库和框架。常用的方法包括在终端中打印得分、使用GUI库显示得分、在网页上显示得分。其中,使用print
函数在终端中打印得分是最简单和基础的方式。你只需使用print
函数将得分输出到控制台即可。下面我将详细介绍如何在终端、GUI、网页等不同环境中显示得分的方法。
一、终端中显示得分
在终端中显示得分是最基础的方式,通常使用print
函数即可实现。这种方式适合于简单的程序,如控制台游戏或脚本。
def display_score(score):
print(f"Current score: {score}")
score = 0
display_score(score)
进行一些操作改变得分
score += 10
display_score(score)
在这个示例中,我们定义了一个display_score
函数,用于打印当前得分。每当得分发生变化时,调用该函数即可显示最新得分。
二、GUI中显示得分
如果你开发的是图形用户界面(GUI)程序,可以使用诸如Tkinter
、PyQt
或Kivy
等库来显示得分。
1、使用Tkinter显示得分
Tkinter
是Python的标准GUI库,适合于创建简单的桌面应用程序。
import tkinter as tk
def update_score():
global score
score += 10
score_label.config(text=f"Score: {score}")
root = tk.Tk()
root.title("Score Display")
score = 0
score_label = tk.Label(root, text=f"Score: {score}", font=("Helvetica", 16))
score_label.pack()
update_button = tk.Button(root, text="Update Score", command=update_score)
update_button.pack()
root.mainloop()
在这个示例中,我们创建了一个简单的Tkinter
窗口,包含一个显示得分的标签和一个更新得分的按钮。每当点击按钮时,得分增加,并更新标签中的文本。
2、使用PyQt显示得分
PyQt
是一个功能强大的GUI库,适合于创建复杂的桌面应用程序。
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QPushButton, QVBoxLayout
class ScoreDisplay(QWidget):
def __init__(self):
super().__init__()
self.score = 0
self.initUI()
def initUI(self):
self.score_label = QLabel(f"Score: {self.score}", self)
self.update_button = QPushButton("Update Score", self)
self.update_button.clicked.connect(self.update_score)
layout = QVBoxLayout()
layout.addWidget(self.score_label)
layout.addWidget(self.update_button)
self.setLayout(layout)
self.setWindowTitle("Score Display")
self.show()
def update_score(self):
self.score += 10
self.score_label.setText(f"Score: {self.score}")
app = QApplication(sys.argv)
ex = ScoreDisplay()
sys.exit(app.exec_())
在这个示例中,我们创建了一个PyQt
窗口,包含一个显示得分的标签和一个更新得分的按钮。每当点击按钮时,得分增加,并更新标签中的文本。
三、网页中显示得分
如果你开发的是一个Web应用程序,可以使用诸如Flask
或Django
等Web框架来显示得分。
1、使用Flask显示得分
Flask
是一个轻量级的Web框架,适合于创建简单的Web应用程序。
from flask import Flask, render_template_string
app = Flask(__name__)
score = 0
@app.route('/')
def index():
global score
return render_template_string('''
<h1>Score: {{ score }}</h1>
<button onclick="location.href='/update'">Update Score</button>
''', score=score)
@app.route('/update')
def update():
global score
score += 10
return render_template_string('''
<h1>Score: {{ score }}</h1>
<button onclick="location.href='/update'">Update Score</button>
''', score=score)
if __name__ == '__main__':
app.run(debug=True)
在这个示例中,我们创建了一个简单的Flask
应用程序,包含一个显示得分的网页和一个更新得分的按钮。每当点击按钮时,得分增加,并更新页面中的文本。
2、使用Django显示得分
Django
是一个功能强大的Web框架,适合于创建复杂的Web应用程序。
首先,创建一个新的Django项目和应用程序:
django-admin startproject score_display
cd score_display
django-admin startapp main
然后,在main/views.py
中定义视图函数:
from django.shortcuts import render
score = 0
def index(request):
global score
return render(request, 'index.html', {'score': score})
def update(request):
global score
score += 10
return render(request, 'index.html', {'score': score})
接下来,在score_display/urls.py
中定义URL模式:
from django.contrib import admin
from django.urls import path
from main import views
urlpatterns = [
path('admin/', admin.site.urls),
path('', views.index),
path('update/', views.update),
]
最后,在main/templates/index.html
中定义HTML模板:
<!DOCTYPE html>
<html>
<head>
<title>Score Display</title>
</head>
<body>
<h1>Score: {{ score }}</h1>
<button onclick="location.href='/update/'">Update Score</button>
</body>
</html>
在这个示例中,我们创建了一个简单的Django
应用程序,包含一个显示得分的网页和一个更新得分的按钮。每当点击按钮时,得分增加,并更新页面中的文本。
四、游戏开发中显示得分
在游戏开发中,显示得分是一个常见需求。可以使用诸如Pygame
等游戏开发库来实现。
import pygame
import sys
pygame.init()
设置屏幕大小
screen = pygame.display.set_mode((640, 480))
pygame.display.set_caption("Score Display")
设置字体
font = pygame.font.Font(None, 36)
初始化得分
score = 0
def display_score(screen, score):
score_text = font.render(f"Score: {score}", True, (255, 255, 255))
screen.blit(score_text, (10, 10))
游戏主循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
score += 10
screen.fill((0, 0, 0))
display_score(screen, score)
pygame.display.flip()
在这个示例中,我们创建了一个简单的Pygame
程序,包含一个显示得分的文本和一个增加得分的功能。每当按下空格键时,得分增加,并更新屏幕上的文本。
五、使用第三方库显示得分
除了上述方法外,还有许多第三方库可以用来显示得分。例如,matplotlib
可以用来绘制得分变化的图表。
1、使用Matplotlib绘制得分变化图表
Matplotlib
是一个用于绘制图表的库,适合于数据分析和可视化。
import matplotlib.pyplot as plt
scores = [0]
def update_score(new_score):
scores.append(new_score)
plt.plot(scores)
plt.xlabel('Updates')
plt.ylabel('Score')
plt.title('Score Changes')
plt.draw()
plt.pause(0.1)
plt.ion() # 开启交互模式
plt.figure()
update_score(10)
update_score(20)
update_score(30)
update_score(40)
plt.ioff() # 关闭交互模式
plt.show()
在这个示例中,我们使用Matplotlib
绘制了得分变化的图表。每当调用update_score
函数时,得分增加,并更新图表。
2、使用Bokeh绘制实时得分图表
Bokeh
是一个用于创建交互式图表的库,适合于Web应用程序。
from bokeh.plotting import figure, curdoc
from bokeh.models import ColumnDataSource
from bokeh.layouts import column
from bokeh.io import push_notebook
source = ColumnDataSource(data=dict(x=[], y=[]))
plot = figure(title="Score Changes", x_axis_label='Updates', y_axis_label='Score')
plot.line('x', 'y', source=source)
def update_score(new_score):
new_data = dict(x=[len(source.data['x'])], y=[new_score])
source.stream(new_data)
push_notebook()
update_score(10)
update_score(20)
update_score(30)
update_score(40)
curdoc().add_root(column(plot))
在这个示例中,我们使用Bokeh
创建了一个实时更新的得分图表。每当调用update_score
函数时,得分增加,并更新图表。
六、总结
在Python中显示得分的方法有很多,根据具体需求选择合适的方法是关键。对于简单的脚本,可以使用print
函数在终端中显示得分;对于桌面应用程序,可以使用Tkinter
或PyQt
等GUI库;对于Web应用程序,可以使用Flask
或Django
等Web框架;对于游戏开发,可以使用Pygame
等游戏开发库;对于数据可视化,可以使用Matplotlib
或Bokeh
等图表库。无论选择哪种方法,关键在于了解其使用方法和适用场景,以便更好地实现得分显示功能。
相关问答FAQs:
如何在Python中创建得分显示功能?
在Python中,可以使用控制台打印或图形界面库(如Tkinter)来显示得分。若使用控制台,可以通过print()
函数动态输出得分值。而使用Tkinter等库,则可以设计一个窗口,实时更新得分信息。可以根据用户的输入或游戏状态来更新得分显示。
我可以在Python中使用哪些库来增强得分显示效果?
可以使用多种库来增强得分显示效果,例如Matplotlib可以用于绘制得分变化图表,Pygame适合于游戏开发中显示得分。若需要更复杂的图形界面,可以考虑使用PyQt或Tkinter,这些库都提供了丰富的界面组件以满足不同的需求。
如何在Python游戏中更新和显示实时得分?
在Python游戏中,可以通过游戏循环来不断更新得分。在每次得分变化时,通过更新显示组件(如文本框或标签)来实时显示得分。使用Pygame时,可以在主循环中调用pygame.display.update()
来刷新屏幕,确保得分的变化及时反映在用户界面上。
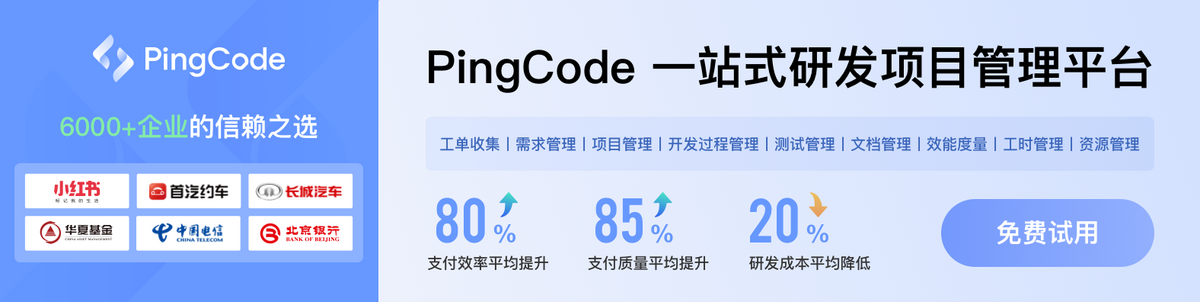