在Python中统计空格数量的方法有多种,包括使用字符串的内置方法、列表推导式、正则表达式等。 其中,字符串的内置方法最为简单和直观,例如使用count
方法统计空格的数量。通过使用count
方法,可以快速且高效地统计字符串中的空格数量。
string = "Hello World, this is a test string."
space_count = string.count(' ')
print(f"The number of spaces in the string is: {space_count}")
在上面的代码中,count
方法遍历字符串并统计空格字符的出现次数。除此之外,我们还可以使用其他方法来统计空格数量,例如使用for
循环和条件判断、列表推导式、正则表达式等。
一、使用字符串内置方法
字符串对象有许多内置方法可以帮助我们处理文本数据。其中,count
方法是统计字符出现次数的常用方法之一。下面是一些例子:
string = "Hello World, this is a test string."
使用count方法统计空格数量
space_count = string.count(' ')
print(f"The number of spaces in the string is: {space_count}")
二、使用for循环和条件判断
通过遍历字符串并检查每个字符是否为空格,我们也可以统计空格数量。这种方法虽然不如内置方法简洁,但对初学者来说是很好的练习。
string = "Hello World, this is a test string."
space_count = 0
遍历字符串并统计空格数量
for char in string:
if char == ' ':
space_count += 1
print(f"The number of spaces in the string is: {space_count}")
三、使用列表推导式
列表推导式是一种简洁的Python语法,允许我们用单行代码创建列表。通过列表推导式,我们可以统计空格数量:
string = "Hello World, this is a test string."
使用列表推导式统计空格数量
space_count = sum([1 for char in string if char == ' '])
print(f"The number of spaces in the string is: {space_count}")
四、使用正则表达式
正则表达式是处理字符串的强大工具,适用于更复杂的模式匹配任务。我们可以使用re
模块来统计空格数量:
import re
string = "Hello World, this is a test string."
使用正则表达式统计空格数量
space_count = len(re.findall(r'\s', string))
print(f"The number of spaces in the string is: {space_count}")
五、效率对比
在实际应用中,效率是一个需要考虑的重要因素。不同的方法在处理大数据量时可能会表现出明显的性能差异。下面我们对上述方法进行简单的性能测试:
import time
string = "Hello World, this is a test string." * 100000
方法1:字符串内置方法
start_time = time.time()
space_count = string.count(' ')
end_time = time.time()
print(f"Count method: {end_time - start_time} seconds")
方法2:for循环和条件判断
start_time = time.time()
space_count = 0
for char in string:
if char == ' ':
space_count += 1
end_time = time.time()
print(f"For loop method: {end_time - start_time} seconds")
方法3:列表推导式
start_time = time.time()
space_count = sum([1 for char in string if char == ' '])
end_time = time.time()
print(f"List comprehension method: {end_time - start_time} seconds")
方法4:正则表达式
start_time = time.time()
space_count = len(re.findall(r'\s', string))
end_time = time.time()
print(f"Regex method: {end_time - start_time} seconds")
从上面的性能测试中,我们可以看到不同方法的时间消耗。一般来说,字符串的count
方法是最为高效的,因为它是由C语言实现的底层操作,性能优越。正则表达式方法在处理更复杂的模式匹配时可能会更加灵活,但在简单统计任务中性能稍逊。for循环和列表推导式在语法上各有优劣,适用于不同的编程风格。
六、实用性和可维护性
在实际项目中,代码的可读性和可维护性同样重要。选择适当的方法不仅要考虑效率,还要考虑代码的简洁性和易读性。对于简单的统计任务,推荐使用内置方法,如count
方法,因为它直观且性能优越。如果代码需要处理更复杂的字符串模式匹配任务,正则表达式是不二选择。
七、扩展应用
统计空格数量的方法不仅限于空格字符,我们还可以扩展这些方法来统计其他字符或模式。例如,统计特定单词的出现次数、统计标点符号数量等。下面是一些示例:
统计特定单词的出现次数
string = "Hello World, this is a test string. This string is just a test."
word = "test"
word_count = string.count(word)
print(f"The word '{word}' appears {word_count} times in the string.")
统计标点符号数量
import string
text = "Hello, World! This is a test string, with some punctuation."
punctuation_count = sum([1 for char in text if char in string.punctuation])
print(f"The number of punctuation marks in the text is: {punctuation_count}")
八、总结
在Python中统计空格数量的方法有多种,包括使用字符串的内置方法、for循环和条件判断、列表推导式、正则表达式等。每种方法都有其优缺点和适用场景。在选择方法时,应根据具体需求考虑效率、可读性和可维护性。通过合理选择和使用这些方法,我们可以有效地处理文本数据,完成各种统计任务。
无论是初学者还是有经验的开发者,掌握这些方法不仅能提高编程效率,还能增强解决实际问题的能力。希望本文提供的内容对您有所帮助,能够在实际项目中应用这些技术,提升代码质量和工作效率。
相关问答FAQs:
如何在Python中有效统计字符串中的空格数量?
可以使用Python内置的count()
方法来统计字符串中的空格数量。只需调用该方法,并将空格字符作为参数传入,例如:string.count(' ')
。这种方法简单明了,适合处理较短的字符串。
在处理大型文本文件时,如何统计空格数量?
对于大型文本文件,可以逐行读取文件并统计每行中的空格数量。可以使用open()
函数打开文件,并结合循环和count()
方法来实现。例如:
total_spaces = 0
with open('yourfile.txt', 'r') as file:
for line in file:
total_spaces += line.count(' ')
这种方法有效地处理了大量数据,确保了内存的高效使用。
如果我想统计不同类型空格(如制表符或换行符)呢?
除了普通空格,还可以使用正则表达式来统计各种空白字符。re
模块提供了灵活的方式来匹配空白,包括制表符和换行符。示例代码如下:
import re
with open('yourfile.txt', 'r') as file:
content = file.read()
spaces = len(re.findall(r'\s', content))
这种方法能够全面统计所有类型的空白字符,适用于需要精确分析文本的场合。
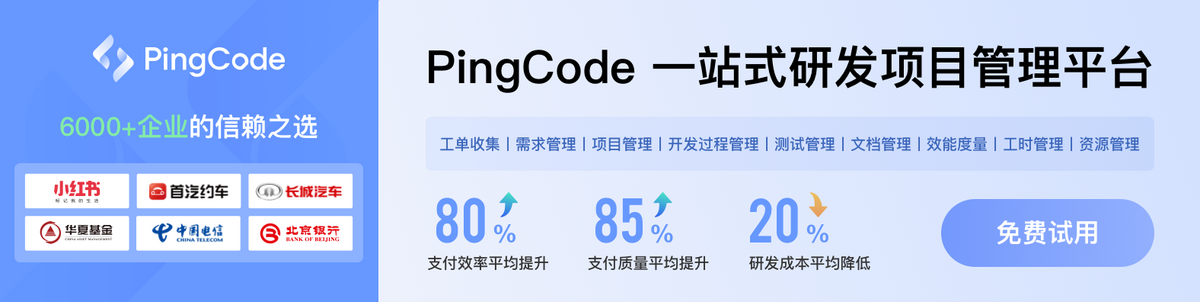