设计Python程序界面时,可以使用Tkinter、PyQt、Kivy等工具。这些工具都有各自的优缺点,选择哪一种取决于具体需求和个人偏好。下面将详细介绍其中的一种——Tkinter的使用方法。
一、Tkinter简介
Tkinter是Python的标准GUI库。Python在安装时自带了Tkinter,因此可以直接使用。Tkinter提供了一种快速而简单的方法来创建GUI应用程序。
优点:
- 内置库,无需额外安装。
- 简单易学,适合初学者。
- 跨平台,支持Windows、Mac和Linux。
缺点:
- 功能相对简单,不适合复杂的GUI需求。
- 外观比较传统,不如其他库美观。
二、Tkinter基础
1、导入Tkinter库
在开始使用Tkinter创建GUI应用程序之前,首先需要导入Tkinter库。
import tkinter as tk
from tkinter import ttk
2、创建主窗口
使用tk.Tk()
创建主窗口,这是所有Tkinter应用程序的基础。
root = tk.Tk()
root.title("My Application")
root.geometry("400x300")
3、添加控件
在主窗口中添加各种控件,如按钮、标签、文本框等。
label = tk.Label(root, text="Hello, Tkinter!")
label.pack()
button = tk.Button(root, text="Click Me", command=lambda: print("Button Clicked"))
button.pack()
4、运行主循环
最后,使用mainloop()
方法运行主循环,使应用程序保持打开状态。
root.mainloop()
三、Tkinter常用控件
1、Label(标签)
标签用于显示文本或图片。
label = tk.Label(root, text="This is a Label")
label.pack()
2、Button(按钮)
按钮用于响应用户点击事件。
button = tk.Button(root, text="Press Me", command=lambda: print("Button Pressed"))
button.pack()
3、Entry(文本框)
文本框用于接收用户输入。
entry = tk.Entry(root)
entry.pack()
4、Text(文本区域)
文本区域用于显示或接收多行文本。
text = tk.Text(root, height=5, width=30)
text.pack()
四、布局管理
1、pack()
pack()
方法将控件按顺序排列。
label1 = tk.Label(root, text="Label 1")
label1.pack(side="top")
label2 = tk.Label(root, text="Label 2")
label2.pack(side="bottom")
2、grid()
grid()
方法将控件放置在网格中。
label1 = tk.Label(root, text="Label 1")
label1.grid(row=0, column=0)
label2 = tk.Label(root, text="Label 2")
label2.grid(row=0, column=1)
3、place()
place()
方法使用绝对坐标来放置控件。
label1 = tk.Label(root, text="Label 1")
label1.place(x=50, y=50)
label2 = tk.Label(root, text="Label 2")
label2.place(x=100, y=100)
五、事件处理
1、按钮事件
使用command
参数绑定按钮事件。
button = tk.Button(root, text="Press Me", command=lambda: print("Button Pressed"))
button.pack()
2、鼠标事件
使用bind()
方法绑定鼠标事件。
def on_click(event):
print("Clicked at", event.x, event.y)
root.bind("<Button-1>", on_click)
3、键盘事件
使用bind()
方法绑定键盘事件。
def on_key(event):
print("Pressed", event.char)
root.bind("<Key>", on_key)
六、消息框
1、显示信息
使用messagebox
模块显示信息对话框。
from tkinter import messagebox
messagebox.showinfo("Information", "This is an information message")
2、确认对话框
显示确认对话框,并根据用户选择执行操作。
if messagebox.askyesno("Confirmation", "Do you want to proceed?"):
print("User chose Yes")
else:
print("User chose No")
七、菜单栏
1、创建菜单栏
使用Menu
类创建菜单栏,并添加菜单和菜单项。
menu = tk.Menu(root)
root.config(menu=menu)
file_menu = tk.Menu(menu)
menu.add_cascade(label="File", menu=file_menu)
file_menu.add_command(label="Open", command=lambda: print("Open File"))
file_menu.add_command(label="Save", command=lambda: print("Save File"))
file_menu.add_separator()
file_menu.add_command(label="Exit", command=root.quit)
八、使用ttk模块
ttk模块提供了更现代、更美观的控件。
1、导入ttk模块
from tkinter import ttk
2、使用ttk控件
button = ttk.Button(root, text="Press Me", command=lambda: print("Button Pressed"))
button.pack()
九、样式与主题
1、设置样式
使用ttk.Style
类设置控件的样式。
style = ttk.Style()
style.configure("TButton", font=("Helvetica", 12), foreground="blue")
button = ttk.Button(root, text="Styled Button")
button.pack()
2、设置主题
使用style.theme_use()
方法切换主题。
style.theme_use("clam")
十、项目实战:简单计算器
1、创建主窗口
root = tk.Tk()
root.title("Simple Calculator")
root.geometry("400x400")
2、创建显示屏
display = tk.Entry(root, font=("Helvetica", 18), bd=10, insertwidth=2, width=14, borderwidth=4)
display.grid(row=0, column=0, columnspan=4)
3、按钮点击事件
def button_click(number):
current = display.get()
display.delete(0, tk.END)
display.insert(0, current + str(number))
4、创建按钮
buttons = [
('7', 1, 0), ('8', 1, 1), ('9', 1, 2), ('/', 1, 3),
('4', 2, 0), ('5', 2, 1), ('6', 2, 2), ('*', 2, 3),
('1', 3, 0), ('2', 3, 1), ('3', 3, 2), ('-', 3, 3),
('0', 4, 0), ('.', 4, 1), ('+', 4, 2), ('=', 4, 3)
]
for (text, row, col) in buttons:
button = tk.Button(root, text=text, padx=20, pady=20, font=("Helvetica", 18),
command=lambda t=text: button_click(t))
button.grid(row=row, column=col)
5、计算结果
def calculate():
try:
result = eval(display.get())
display.delete(0, tk.END)
display.insert(0, str(result))
except Exception as e:
display.delete(0, tk.END)
display.insert(0, "Error")
6、绑定等号按钮
button_equal = tk.Button(root, text='=', padx=20, pady=20, font=("Helvetica", 18), command=calculate)
button_equal.grid(row=4, column=3)
十一、总结
通过以上步骤,我们创建了一个简单的计算器应用程序。Tkinter提供了丰富的控件和布局管理器,能够快速构建简单的GUI应用程序。对于更复杂的需求,可以考虑使用PyQt或Kivy等更强大的GUI库。无论选择哪种库,理解基本的GUI编程概念和事件处理机制都是非常重要的。希望本文能帮助你更好地理解和使用Tkinter来设计Python程序界面。
相关问答FAQs:
如何选择适合的Python GUI库?
在设计Python程序界面时,选择合适的GUI库是至关重要的。常用的库包括Tkinter、PyQt、wxPython等。Tkinter是Python标准库的一部分,适合简单应用;PyQt功能强大,适合复杂界面;而wxPython则提供了原生的窗口效果。根据项目需求和个人熟悉程度来选择合适的库将提高开发效率和用户体验。
如何实现Python GUI界面的布局设计?
布局设计在界面开发中起着关键作用。使用所选的GUI库,通常会有多种布局管理器可供选择。例如,Tkinter提供了pack、grid和place三种布局方式。通过合理使用这些布局管理器,可以确保界面元素整齐排列,提升视觉效果和用户友好性。设计时应考虑用户操作的流畅性和界面的可读性。
如何提高Python程序界面的用户体验?
改善用户体验可以通过多种方式实现。首先,保持界面的简洁性,避免信息过载。其次,使用一致的风格和颜色方案,让用户感到舒适。提供明确的反馈,例如按钮被点击后的变化,也能增强互动感。此外,确保界面在不同设备和屏幕尺寸下都能良好显示,提升整体用户满意度。
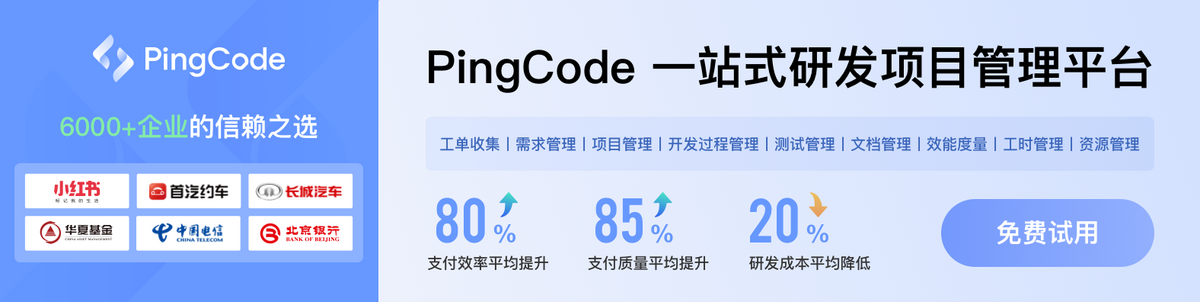