如何访问Python中的文件:
Python提供了多种方法来访问文件、包括内置的open()函数、使用上下文管理器、以及通过库如os和shutil。使用上下文管理器是一种推荐的方法,因为它能自动管理文件的打开和关闭。 例如:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
使用上下文管理器可以确保文件在使用后自动关闭,避免资源泄漏。
一、使用open()函数访问文件
Python中最常用的文件访问方法是使用内置的open()函数。open()函数可以打开文件进行读取、写入或追加操作。
- 读取文件
with open('example.txt', 'r') as file:
content = file.read()
print(content)
在上面的代码中,我们使用'r'模式打开文件进行读取。文件内容被读入变量content并打印出来。使用with语句可以确保文件在使用后自动关闭。
- 写入文件
with open('example.txt', 'w') as file:
file.write('Hello, World!')
在上面的代码中,我们使用'w'模式打开文件进行写入。如果文件不存在,将创建一个新文件。如果文件已存在,其内容将被清空。
- 追加内容
with open('example.txt', 'a') as file:
file.write('\nHello again!')
在上面的代码中,我们使用'a'模式打开文件进行追加操作。新内容将被追加到文件末尾。
二、使用os模块访问文件
os模块提供了一些与操作系统相关的功能,可以用于操作文件和目录。
- 检查文件是否存在
import os
if os.path.exists('example.txt'):
print('File exists')
else:
print('File does not exist')
在上面的代码中,我们使用os.path.exists()函数检查文件是否存在。
- 获取文件大小
import os
file_size = os.path.getsize('example.txt')
print(f'File size: {file_size} bytes')
在上面的代码中,我们使用os.path.getsize()函数获取文件的大小。
- 重命名文件
import os
os.rename('example.txt', 'new_example.txt')
在上面的代码中,我们使用os.rename()函数重命名文件。
三、使用shutil模块访问文件
shutil模块提供了一些高级文件操作功能,例如复制和移动文件。
- 复制文件
import shutil
shutil.copy('example.txt', 'copy_of_example.txt')
在上面的代码中,我们使用shutil.copy()函数复制文件。
- 移动文件
import shutil
shutil.move('example.txt', 'new_folder/example.txt')
在上面的代码中,我们使用shutil.move()函数移动文件。
四、文件的读取方式
在Python中,除了使用read()方法读取整个文件内容外,还有其他几种常用的读取方式。
- 按行读取
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
在上面的代码中,我们使用for循环按行读取文件内容,并使用strip()方法去除每行末尾的换行符。
- 使用readlines()方法
with open('example.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line.strip())
在上面的代码中,我们使用readlines()方法一次读取文件的所有行,并将其存储在一个列表中。
五、文件的写入方式
除了使用write()方法写入文件外,还有其他几种常用的写入方式。
- 写入多行内容
lines = ['Hello, World!', 'Welcome to Python programming.']
with open('example.txt', 'w') as file:
file.writelines(lines)
在上面的代码中,我们使用writelines()方法将一个字符串列表写入文件。
- 使用print()函数写入文件
with open('example.txt', 'w') as file:
print('Hello, World!', file=file)
在上面的代码中,我们使用print()函数将内容写入文件。
六、文件的二进制操作
有时我们需要读取和写入二进制文件,例如图像文件或音频文件。
- 读取二进制文件
with open('example.jpg', 'rb') as file:
content = file.read()
print(content)
在上面的代码中,我们使用'rb'模式打开文件进行二进制读取。
- 写入二进制文件
with open('example.jpg', 'rb') as file:
content = file.read()
with open('copy_of_example.jpg', 'wb') as file:
file.write(content)
在上面的代码中,我们使用'wb'模式打开文件进行二进制写入。
七、文件的随机访问
有时我们需要在文件中进行随机访问,即在文件的任意位置读取或写入数据。
- 使用seek()和tell()方法
with open('example.txt', 'r') as file:
file.seek(5) # 移动到文件的第6个字节
content = file.read(10) # 读取接下来的10个字节
print(content)
position = file.tell() # 获取当前文件指针的位置
print(f'Current position: {position}')
在上面的代码中,我们使用seek()方法移动文件指针,并使用tell()方法获取当前文件指针的位置。
- 使用with语句进行随机访问
with open('example.txt', 'r+') as file:
file.seek(5)
file.write('Python')
file.seek(0)
content = file.read()
print(content)
在上面的代码中,我们使用'r+'模式打开文件进行读写操作,并使用seek()方法移动文件指针。
八、文件的压缩和解压缩
有时我们需要对文件进行压缩和解压缩操作,以节省存储空间或传输时间。
- 使用gzip模块进行压缩和解压缩
import gzip
with open('example.txt', 'rb') as file_in:
with gzip.open('example.txt.gz', 'wb') as file_out:
file_out.writelines(file_in)
with gzip.open('example.txt.gz', 'rb') as file_in:
with open('uncompressed_example.txt', 'wb') as file_out:
file_out.writelines(file_in)
在上面的代码中,我们使用gzip模块对文件进行压缩和解压缩操作。
- 使用zipfile模块进行压缩和解压缩
import zipfile
with zipfile.ZipFile('example.zip', 'w') as zipf:
zipf.write('example.txt')
with zipfile.ZipFile('example.zip', 'r') as zipf:
zipf.extractall('extracted_files')
在上面的代码中,我们使用zipfile模块对文件进行压缩和解压缩操作。
九、文件的权限管理
在某些情况下,我们需要管理文件的权限,以确保文件的安全性。
- 查看文件权限
import os
permissions = os.stat('example.txt').st_mode
print(oct(permissions))
在上面的代码中,我们使用os.stat()函数获取文件的权限,并使用oct()函数将其转换为八进制表示。
- 修改文件权限
import os
os.chmod('example.txt', 0o644)
在上面的代码中,我们使用os.chmod()函数修改文件的权限。
十、文件的锁定
在某些情况下,我们需要锁定文件以防止其他进程同时访问文件。
- 使用fcntl模块进行文件锁定
import fcntl
with open('example.txt', 'r+') as file:
fcntl.flock(file, fcntl.LOCK_EX)
file.write('Locked file')
fcntl.flock(file, fcntl.LOCK_UN)
在上面的代码中,我们使用fcntl模块对文件进行锁定和解锁操作。
- 使用portalocker模块进行文件锁定
import portalocker
with open('example.txt', 'r+') as file:
portalocker.lock(file, portalocker.LOCK_EX)
file.write('Locked file')
portalocker.unlock(file)
在上面的代码中,我们使用portalocker模块对文件进行锁定和解锁操作。
十一、文件的临时操作
有时我们需要创建和使用临时文件或临时目录。
- 使用tempfile模块创建临时文件
import tempfile
with tempfile.TemporaryFile() as temp_file:
temp_file.write(b'Temporary file content')
temp_file.seek(0)
content = temp_file.read()
print(content)
在上面的代码中,我们使用tempfile模块创建和使用临时文件。
- 使用tempfile模块创建临时目录
import tempfile
import os
with tempfile.TemporaryDirectory() as temp_dir:
print(f'Temporary directory: {temp_dir}')
temp_file_path = os.path.join(temp_dir, 'temp_file.txt')
with open(temp_file_path, 'w') as temp_file:
temp_file.write('Temporary file content')
在上面的代码中,我们使用tempfile模块创建和使用临时目录。
十二、文件的编码处理
在处理文本文件时,我们需要注意文件的编码格式,以确保文件内容正确读取和写入。
- 读取不同编码格式的文件
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
在上面的代码中,我们使用encoding参数指定文件的编码格式。
- 写入不同编码格式的文件
with open('example.txt', 'w', encoding='utf-8') as file:
file.write('Hello, World!')
在上面的代码中,我们使用encoding参数指定文件的编码格式。
十三、文件的异常处理
在进行文件操作时,我们需要处理可能出现的异常,以确保程序的健壮性。
- 捕获文件操作异常
try:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print('File not found')
except IOError:
print('An I/O error occurred')
在上面的代码中,我们使用try-except结构捕获文件操作过程中可能出现的异常。
- 使用finally块确保文件关闭
file = None
try:
file = open('example.txt', 'r')
content = file.read()
print(content)
except FileNotFoundError:
print('File not found')
except IOError:
print('An I/O error occurred')
finally:
if file:
file.close()
在上面的代码中,我们使用finally块确保文件在操作结束后关闭。
十四、文件的路径处理
在进行文件操作时,我们需要处理文件的路径,以确保文件正确访问。
- 使用os.path处理文件路径
import os
file_path = os.path.join('folder', 'example.txt')
print(file_path)
absolute_path = os.path.abspath('example.txt')
print(absolute_path)
在上面的代码中,我们使用os.path模块处理文件路径。
- 使用pathlib模块处理文件路径
from pathlib import Path
file_path = Path('folder') / 'example.txt'
print(file_path)
absolute_path = Path('example.txt').resolve()
print(absolute_path)
在上面的代码中,我们使用pathlib模块处理文件路径。
十五、文件的备份和恢复
在进行重要文件操作之前,我们通常需要备份文件,以防止数据丢失。
- 备份文件
import shutil
shutil.copy('example.txt', 'backup_example.txt')
在上面的代码中,我们使用shutil.copy()函数备份文件。
- 恢复文件
import shutil
shutil.copy('backup_example.txt', 'example.txt')
在上面的代码中,我们使用shutil.copy()函数恢复文件。
总结
通过本文,我们详细介绍了如何在Python中访问文件的各种方法和技巧,包括使用open()函数、os模块、shutil模块、文件的读取和写入方式、二进制操作、随机访问、压缩和解压缩、权限管理、文件锁定、临时操作、编码处理、异常处理、路径处理以及文件的备份和恢复。希望这些内容能够帮助您更好地理解和掌握Python中的文件操作。
相关问答FAQs:
如何在Python中打开和读取文本文件?
在Python中,可以使用内置的open()
函数打开文件。通过指定文件路径和访问模式(如“r”表示只读),可以读取文件内容。例如,使用以下代码打开并读取文本文件:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
这种方式使用with
语句确保文件在读取后自动关闭。
Python支持哪些文件访问模式?
Python的open()
函数支持多种文件访问模式。常见的模式包括:
'r'
:只读模式,文件必须存在。'w'
:写入模式,如果文件存在则覆盖,不存在则创建。'a'
:追加模式,数据会被添加到文件末尾。'b'
:二进制模式,常用于处理非文本文件,如图像和音频。
如何处理文件访问中的异常?
在访问文件时,可能会遇到一些异常情况,比如文件不存在或权限不足。可以使用try...except
结构来捕获和处理这些异常。例如:
try:
with open('example.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print("文件未找到,请检查文件路径。")
except PermissionError:
print("没有权限访问该文件。")
这种方式能有效增强代码的健壮性,确保程序在遇到错误时不会崩溃。
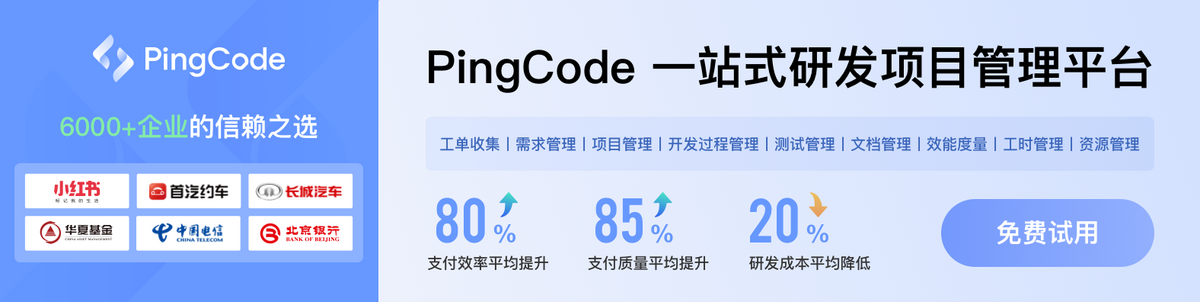