使用Python编写罗盘时钟的几种方法包括使用tkinter、pygame、matplotlib等库。 在这篇文章中,我们将详细探讨使用这些库来创建一个罗盘时钟。我们将从简单的概念介绍开始,然后逐步深入到代码实现和具体细节。
一、使用Tkinter创建罗盘时钟
Tkinter是Python的标准GUI库。使用Tkinter可以非常方便地创建图形用户界面。下面是一个使用Tkinter创建罗盘时钟的示例。
1、安装Tkinter
Tkinter通常是与Python一起安装的。如果你没有安装Tkinter,可以通过以下命令进行安装:
pip install tk
2、代码实现
下面是一个简单的使用Tkinter创建罗盘时钟的代码示例:
import tkinter as tk
import time
import math
class CompassClock(tk.Tk):
def __init__(self):
super().__init__()
self.title("Compass Clock")
self.canvas = tk.Canvas(self, width=400, height=400, bg='white')
self.canvas.pack()
self.update_clock()
def draw_hand(self, angle, length, width, color):
angle_rad = math.radians(angle)
x = 200 + length * math.sin(angle_rad)
y = 200 - length * math.cos(angle_rad)
self.canvas.create_line(200, 200, x, y, width=width, fill=color)
def update_clock(self):
self.canvas.delete("all")
self.canvas.create_oval(50, 50, 350, 350)
# Draw the compass directions
directions = ["N", "E", "S", "W"]
for i, direction in enumerate(directions):
angle = i * 90
angle_rad = math.radians(angle)
x = 200 + 150 * math.sin(angle_rad)
y = 200 - 150 * math.cos(angle_rad)
self.canvas.create_text(x, y, text=direction, font=("Helvetica", 15))
# Get the current time
now = time.localtime()
hours = now.tm_hour % 12
minutes = now.tm_min
seconds = now.tm_sec
# Calculate the angles of the hands
second_angle = seconds * 6
minute_angle = minutes * 6 + seconds * 0.1
hour_angle = hours * 30 + minutes * 0.5
# Draw the hands
self.draw_hand(second_angle, 140, 1, 'red')
self.draw_hand(minute_angle, 120, 2, 'blue')
self.draw_hand(hour_angle, 90, 4, 'black')
# Schedule the next update
self.after(1000, self.update_clock)
if __name__ == "__main__":
clock = CompassClock()
clock.mainloop()
二、使用Pygame创建罗盘时钟
Pygame是一个跨平台的Python模块,用于开发视频游戏。它包括计算机图形和声音库。使用Pygame创建罗盘时钟也非常简单。
1、安装Pygame
你可以通过以下命令安装Pygame:
pip install pygame
2、代码实现
下面是一个使用Pygame创建罗盘时钟的代码示例:
import pygame
import math
import time
Initialize Pygame
pygame.init()
Set up display
WIDTH, HEIGHT = 400, 400
win = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Compass Clock")
Colors
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
Draw hand function
def draw_hand(angle, length, width, color):
angle_rad = math.radians(angle)
x = WIDTH // 2 + length * math.sin(angle_rad)
y = HEIGHT // 2 - length * math.cos(angle_rad)
pygame.draw.line(win, color, (WIDTH // 2, HEIGHT // 2), (x, y), width)
Main loop
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
win.fill(WHITE)
pygame.draw.circle(win, BLACK, (WIDTH // 2, HEIGHT // 2), 150, 1)
# Draw the compass directions
directions = ["N", "E", "S", "W"]
for i, direction in enumerate(directions):
angle = i * 90
angle_rad = math.radians(angle)
x = WIDTH // 2 + 140 * math.sin(angle_rad)
y = HEIGHT // 2 - 140 * math.cos(angle_rad)
font = pygame.font.SysFont('Helvetica', 24)
text = font.render(direction, True, BLACK)
win.blit(text, (x - text.get_width() // 2, y - text.get_height() // 2))
# Get the current time
now = time.localtime()
hours = now.tm_hour % 12
minutes = now.tm_min
seconds = now.tm_sec
# Calculate the angles of the hands
second_angle = seconds * 6
minute_angle = minutes * 6 + seconds * 0.1
hour_angle = hours * 30 + minutes * 0.5
# Draw the hands
draw_hand(second_angle, 140, 1, RED)
draw_hand(minute_angle, 120, 2, BLUE)
draw_hand(hour_angle, 90, 4, BLACK)
pygame.display.flip()
pygame.time.delay(1000)
pygame.quit()
三、使用Matplotlib创建罗盘时钟
Matplotlib是一个Python 2D绘图库,它生成各种硬拷贝格式和跨平台的交互式环境。我们可以使用Matplotlib创建一个罗盘时钟。
1、安装Matplotlib
你可以通过以下命令安装Matplotlib:
pip install matplotlib
2、代码实现
下面是一个使用Matplotlib创建罗盘时钟的代码示例:
import matplotlib.pyplot as plt
import numpy as np
import time
def draw_hand(ax, angle, length, width, color):
angle_rad = np.deg2rad(angle)
x = length * np.sin(angle_rad)
y = length * np.cos(angle_rad)
ax.plot([0, x], [0, y], linewidth=width, color=color)
def update_clock():
plt.clf()
ax = plt.subplot(111, polar=True)
ax.set_theta_offset(np.pi / 2)
ax.set_theta_direction(-1)
ax.set_yticklabels([])
ax.set_xticks(np.linspace(0, 2 * np.pi, 4, endpoint=False))
ax.set_xticklabels(['N', 'E', 'S', 'W'])
now = time.localtime()
hours = now.tm_hour % 12
minutes = now.tm_min
seconds = now.tm_sec
second_angle = seconds * 6
minute_angle = minutes * 6 + seconds * 0.1
hour_angle = hours * 30 + minutes * 0.5
draw_hand(ax, second_angle, 1, 1, 'red')
draw_hand(ax, minute_angle, 0.8, 2, 'blue')
draw_hand(ax, hour_angle, 0.6, 4, 'black')
plt.pause(1)
if __name__ == "__main__":
plt.ion()
while True:
update_clock()
plt.ioff()
plt.show()
四、总结
通过以上示例,我们可以看到使用不同的库创建罗盘时钟的方法。使用Tkinter、Pygame和Matplotlib都有各自的优势。Tkinter适合创建简单的桌面应用程序,Pygame适合创建游戏和图形更丰富的应用程序,而Matplotlib则适合创建科学计算和数据可视化应用程序。
在实际应用中,可以根据具体需求选择合适的库和方法来创建罗盘时钟。希望这篇文章对你有所帮助,并且能够让你在学习Python编程的过程中更好地理解如何使用这些库来创建有趣的应用程序。
相关问答FAQs:
如何在Python中实现罗盘时钟的基本功能?
在Python中实现罗盘时钟的基本功能,可以使用图形界面库如Tkinter或Pygame来绘制时钟的外观。你需要获取当前的时间,并根据时间来计算指针的角度。使用三角函数可以帮助你确定每个指针的位置,从而在窗口上绘制出相应的时钟指针。此外,可以使用定时器来定期更新时钟显示,确保时钟是实时的。
有哪些Python库可以帮助创建罗盘时钟?
创建罗盘时钟时,Tkinter是一个非常流行的选择,因为它是Python标准库的一部分,易于使用。Pygame也是一个很好的选择,适合需要更复杂图形和动画效果的项目。其他可以考虑的库包括Matplotlib,用于创建更具科学感的可视化效果,或PyQt,适合开发更复杂的桌面应用程序。
如何优化罗盘时钟的性能和用户体验?
优化罗盘时钟的性能可以通过减少重绘的频率和使用更高效的绘图方法来实现。例如,使用“只更新变化部分”策略,而不是每次都重绘整个时钟。此外,确保用户界面简洁明了,使用适当的颜色和字体提高可读性。可以考虑添加一些互动功能,比如让用户选择不同的主题或时钟样式,以增强用户体验。
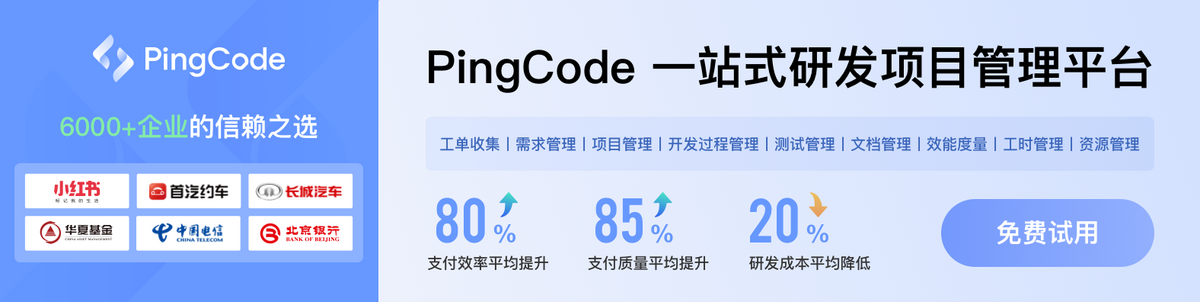