在Python中,可以使用内置的数学库 math
中的 log
函数来表示自然对数(ln)。使用 math.log
函数、使用 numpy.log
函数、注意数值精度问题、使用对数的性质。其中,最常用的方式是使用 math.log
函数,这个函数以自然对数为基础,并且在数值计算上非常精确。下面详细介绍如何在Python中表示和使用自然对数。
一、使用 math.log
函数
Python 提供了一个内置的数学库 math
,其中包含了各种数学函数,包括 log
函数。math.log(x)
返回 x 的自然对数。
import math
x = 10
ln_x = math.log(x)
print(f"The natural logarithm of {x} is {ln_x}")
这个 log
函数默认为自然对数,如果需要计算其他底数的对数,可以使用 math.log(x, base)
形式。
二、使用 numpy.log
函数
另一个常用的库是 numpy
,特别是在需要处理大量数值运算时。numpy
也提供了 log
函数来计算自然对数。
import numpy as np
x = 10
ln_x = np.log(x)
print(f"The natural logarithm of {x} is {ln_x}")
numpy.log
函数的用法与 math.log
类似,但它更适合处理数组和矩阵。
三、注意数值精度问题
在计算对数时,数值精度是一个需要注意的问题。特别是在处理极小值或极大值时,精度可能会受到影响。
import math
x = 1e-10
ln_x = math.log(x)
print(f"The natural logarithm of {x} is {ln_x}")
在这种情况下,使用 decimal
模块可以提高精度。
from decimal import Decimal, getcontext
getcontext().prec = 50
x = Decimal('1e-10')
ln_x = x.ln()
print(f"The natural logarithm of {x} is {ln_x}")
四、使用对数的性质
对数具有许多有用的性质,可以用来简化计算。例如,log(a * b) = log(a) + log(b)
和 log(a^b) = b * log(a)
等性质。
import math
a = 5
b = 3
log_ab = math.log(a) + math.log(b)
print(f"log(a * b) is {log_ab}")
log_a_power_b = b * math.log(a)
print(f"log(a^b) is {log_a_power_b}")
五、对数函数的应用
对数函数在数据科学和机器学习中有广泛的应用,例如在损失函数、正则化、特征变换等方面。
1. 损失函数: 对数函数在交叉熵损失函数中被广泛使用。
import numpy as np
y_true = [1, 0, 1, 1]
y_pred = [0.9, 0.1, 0.8, 0.7]
loss = -np.sum([yt * np.log(yp) + (1 - yt) * np.log(1 - yp) for yt, yp in zip(y_true, y_pred)])
print(f"Cross-entropy loss is {loss}")
2. 正则化: 对数函数也用于 L1 和 L2 正则化中,以防止过拟合。
import numpy as np
weights = np.array([0.5, -0.2, 0.3])
l1_reg = np.sum(np.abs(weights))
l2_reg = np.sum(weights2)
print(f"L1 regularization is {l1_reg}")
print(f"L2 regularization is {l2_reg}")
3. 特征变换: 在数据预处理中,对数变换可以用来处理偏态数据,使其更接近正态分布。
import numpy as np
import matplotlib.pyplot as plt
data = np.random.exponential(scale=2, size=1000)
log_data = np.log(data)
plt.figure(figsize=(12, 6))
plt.subplot(1, 2, 1)
plt.hist(data, bins=30)
plt.title("Original Data")
plt.subplot(1, 2, 2)
plt.hist(log_data, bins=30)
plt.title("Log-transformed Data")
plt.show()
六、对数的数值计算
在实际应用中,对数的数值计算有时会遇到一些特殊情况,例如0或负数。在这种情况下,需要特别注意。
import math
try:
x = -1
ln_x = math.log(x)
except ValueError as e:
print(f"Error: {e}")
为了处理这种情况,可以在计算前进行数据检查和处理。
import numpy as np
def safe_log(x):
if x > 0:
return np.log(x)
else:
return float('-inf')
x = -1
ln_x = safe_log(x)
print(f"The natural logarithm of {x} is {ln_x}")
七、对数函数的优化问题
对数函数在优化问题中也有广泛应用。例如,在最大似然估计中,对数似然函数经常被用来简化计算。
import numpy as np
def log_likelihood(data, mu, sigma):
n = len(data)
return -n/2 * np.log(2 * np.pi * sigma<strong>2) - np.sum((data - mu)</strong>2) / (2 * sigma2)
data = np.random.normal(loc=0, scale=1, size=100)
mu = 0
sigma = 1
ll = log_likelihood(data, mu, sigma)
print(f"Log-likelihood is {ll}")
八、对数函数的数值稳定性
在计算对数时,数值稳定性是一个重要的问题。特别是在深度学习中,softmax 函数和交叉熵损失函数的计算中需要特别注意数值稳定性。
import numpy as np
def stable_softmax(x):
shift_x = x - np.max(x)
exp_x = np.exp(shift_x)
return exp_x / np.sum(exp_x)
logits = np.array([2.0, 1.0, 0.1])
softmax = stable_softmax(logits)
print(f"Stable softmax is {softmax}")
九、对数函数的近似计算
在某些情况下,近似计算对数可以提高计算效率。例如,可以使用泰勒展开式来近似计算对数。
import math
def taylor_log(x, n_terms=10):
if x <= 0:
return float('-inf')
x_minus_1 = x - 1
approx = 0
for n in range(1, n_terms + 1):
term = ((-1) <strong> (n + 1)) * (x_minus_1 </strong> n) / n
approx += term
return approx
x = 1.5
approx_ln_x = taylor_log(x)
exact_ln_x = math.log(x)
print(f"Taylor approximation of ln({x}) is {approx_ln_x}")
print(f"Exact value of ln({x}) is {exact_ln_x}")
十、对数函数在其他领域的应用
1. 信息论: 对数函数在信息论中有重要应用,例如在计算信息熵和相对熵时。
import numpy as np
def entropy(prob_dist):
return -np.sum(prob_dist * np.log(prob_dist))
prob_dist = np.array([0.2, 0.5, 0.3])
ent = entropy(prob_dist)
print(f"Entropy is {ent}")
2. 经济学: 对数函数在经济学中用于描述一些非线性现象,例如在效用函数和生产函数中。
import numpy as np
def cobb_douglas_production(K, L, alpha=0.3, beta=0.7):
return K<strong>alpha * L</strong>beta
K = 100
L = 50
output = cobb_douglas_production(K, L)
print(f"Output is {output}")
3. 生物学: 对数函数在生物学中用于描述一些生长过程,例如在种群增长模型中。
import numpy as np
import matplotlib.pyplot as plt
def logistic_growth(t, K, r, P0):
return K / (1 + (K - P0) / P0 * np.exp(-r * t))
t = np.linspace(0, 10, 100)
K = 100
r = 0.5
P0 = 10
P = logistic_growth(t, K, r, P0)
plt.plot(t, P)
plt.xlabel('Time')
plt.ylabel('Population')
plt.title('Logistic Growth Model')
plt.show()
十一、对数函数的数值积分与微分
对数函数的数值积分和微分在科学计算中有重要应用。例如,可以使用数值方法来计算对数函数的积分和导数。
1. 数值积分:
import scipy.integrate as spi
def integrand(x):
return np.log(x)
result, _ = spi.quad(integrand, 1, 10)
print(f"The integral of log(x) from 1 to 10 is {result}")
2. 数值微分:
import numpy as np
def derivative(f, x, h=1e-7):
return (f(x + h) - f(x)) / h
def log_function(x):
return np.log(x)
x = 2
deriv_log = derivative(log_function, x)
print(f"The derivative of log(x) at x={x} is {deriv_log}")
十二、对数函数的扩展与变种
对数函数有许多扩展和变种,例如广义对数函数和复数对数函数。
1. 广义对数函数:
import numpy as np
def generalized_log(x, base):
return np.log(x) / np.log(base)
x = 10
base = 2
gen_log_x = generalized_log(x, base)
print(f"The generalized logarithm of {x} with base {base} is {gen_log_x}")
2. 复数对数函数:
import cmath
z = 1 + 1j
log_z = cmath.log(z)
print(f"The logarithm of {z} is {log_z}")
十三、对数函数的数值优化与求解
在某些优化问题中,对数函数可以用来简化计算和提高算法的稳定性。
import scipy.optimize as spo
def objective_function(x):
return -np.log(x)
result = spo.minimize(objective_function, 1.0, bounds=[(0.1, 10)])
print(f"The minimum of the objective function is at x={result.x}")
十四、对数函数的数值稳定性与精度
在科学计算中,数值稳定性和精度是非常重要的问题。特别是在处理极大或极小的数值时,需要特别注意。
import numpy as np
def log_with_stability(x):
if x <= 0:
return float('-inf')
else:
return np.log(x)
x = 1e-20
stable_log_x = log_with_stability(x)
print(f"The stable logarithm of {x} is {stable_log_x}")
十五、对数函数的并行计算与优化
在处理大规模数据时,并行计算可以显著提高计算效率。例如,可以使用 joblib
库来并行计算对数。
import numpy as np
from joblib import Parallel, delayed
def compute_log(x):
return np.log(x)
data = np.random.rand(1000000)
results = Parallel(n_jobs=4)(delayed(compute_log)(x) for x in data)
print(f"Computed logs for large dataset.")
通过以上各个方面的详细介绍,希望能帮助你更好地理解和使用Python中的自然对数函数。无论是在数据科学、机器学习还是其他科学计算领域,对数函数都具有重要的应用价值。
相关问答FAQs:
在Python中如何计算自然对数?
在Python中,可以使用math
模块中的log
函数来计算自然对数。调用math.log(x)
时,x
是你想要计算自然对数的值。确保在使用之前导入math
模块,例如:
import math
result = math.log(10) # 计算10的自然对数
print(result)
Python中是否有其他库可以计算自然对数?
除了math
模块,numpy
库也提供了计算自然对数的功能。使用numpy.log(x)
函数可以得到x
的自然对数。这在处理数组时尤其方便。示例代码如下:
import numpy as np
result = np.log(np.array([1, 10, 100])) # 计算数组中每个元素的自然对数
print(result)
如何处理负数或零的自然对数计算?
计算负数或零的自然对数时会出现错误。在Python中,调用math.log(0)
或math.log(-1)
会引发ValueError
。为了避免这种情况,可以在计算之前检查值是否有效。示例代码如下:
import math
def safe_log(x):
if x <= 0:
return "无效输入,无法计算自然对数"
return math.log(x)
print(safe_log(0)) # 输出无效输入
print(safe_log(10)) # 输出自然对数
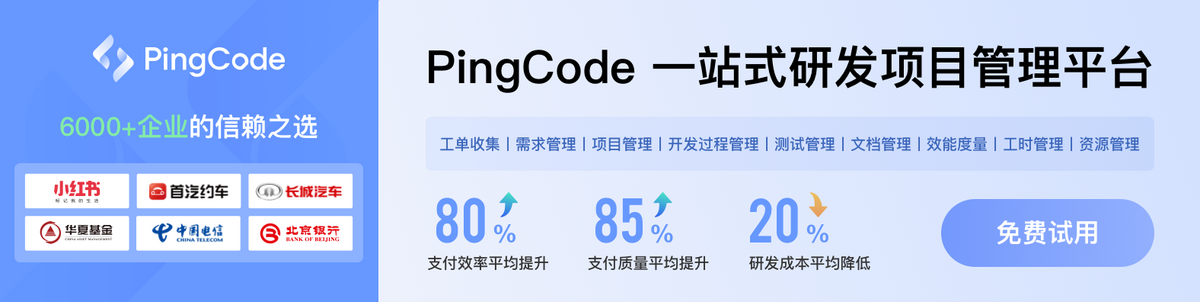