在Python中爬取网站视频的方法包括使用网络请求库、解析HTML内容的库和视频下载工具。常用的方法包括使用requests库获取网页内容、使用BeautifulSoup解析视频链接、使用youtube-dl下载视频,接下来我们将详细介绍其中的一种方法:使用youtube-dl
库下载视频。
一、获取网页内容
在爬取网站视频的过程中,首先需要获取网页内容。我们可以使用Python的requests
库来完成这一任务。
import requests
url = 'https://example.com/video-page'
response = requests.get(url)
html_content = response.content
在上面的代码中,我们使用requests.get()
方法获取网页内容,并将其存储在html_content
变量中。
二、解析HTML内容
获取网页内容后,我们需要解析HTML内容以找到视频链接。我们可以使用BeautifulSoup
库来完成这一任务。
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
video_tag = soup.find('video')
video_url = video_tag['src']
在上面的代码中,我们使用BeautifulSoup
解析HTML内容,并找到<video>
标签。然后,从该标签中提取视频链接。
三、使用youtube-dl下载视频
下载视频是爬取网站视频的最后一步。我们可以使用youtube-dl
库来完成这一任务。
import youtube_dl
ydl_opts = {}
with youtube_dl.YoutubeDL(ydl_opts) as ydl:
ydl.download([video_url])
在上面的代码中,我们创建一个youtube_dl.YoutubeDL
对象,并使用download()
方法下载视频。
四、处理动态加载的内容
有些网站的视频链接是通过JavaScript动态加载的,这种情况我们需要使用selenium
库来模拟浏览器行为。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
driver.get('https://example.com/video-page')
video_element = driver.find_element(By.TAG_NAME, 'video')
video_url = video_element.get_attribute('src')
driver.quit()
在上面的代码中,我们使用selenium
库打开网页,并找到<video>
标签。然后,从该标签中提取视频链接。
五、处理反爬虫机制
许多网站具有反爬虫机制,这可能会阻止您爬取视频。在这种情况下,我们可以使用一些技术来绕过反爬虫机制。
- 伪装请求头:通过伪装请求头,可以模拟真实用户的请求。
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
- 使用代理:通过使用代理,可以隐藏您的真实IP地址。
proxies = {
'http': 'http://your_proxy:port',
'https': 'https://your_proxy:port'
}
response = requests.get(url, headers=headers, proxies=proxies)
- 模拟用户行为:通过模拟用户行为,可以避免触发反爬虫机制。
from selenium.webdriver.common.action_chains import ActionChains
actions = ActionChains(driver)
actions.move_to_element(video_element).perform()
六、总结
在使用Python爬取网站视频时,获取网页内容、解析HTML内容、使用youtube-dl下载视频、处理动态加载内容和反爬虫机制是关键步骤。通过结合这些技术,可以成功地爬取网站视频。需要注意的是,在进行爬虫操作时,一定要遵守网站的robots.txt
文件和相关法律法规。
七、完整示例代码
import requests
from bs4 import BeautifulSoup
import youtube_dl
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
Step 1: Use requests to get the webpage content
url = 'https://example.com/video-page'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
html_content = response.content
Step 2: Use BeautifulSoup to parse the HTML content
soup = BeautifulSoup(html_content, 'html.parser')
video_tag = soup.find('video')
video_url = video_tag['src'] if video_tag else None
Step 3: If video_url is None, use selenium to handle dynamic content
if not video_url:
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
driver.get(url)
video_element = driver.find_element(By.TAG_NAME, 'video')
video_url = video_element.get_attribute('src')
driver.quit()
Step 4: Use youtube-dl to download the video
if video_url:
ydl_opts = {}
with youtube_dl.YoutubeDL(ydl_opts) as ydl:
ydl.download([video_url])
else:
print("Failed to find video URL.")
通过上述代码,我们可以全面地爬取网站视频,并处理各种潜在的问题。
相关问答FAQs:
如何使用Python爬取特定网站的视频?
要爬取特定网站的视频,首先需要分析该网站的结构,确定视频的源URL。可以使用Python中的库,如requests获取网页内容,BeautifulSoup解析HTML。对于动态加载的视频,可能需要使用Selenium等工具模拟浏览器行为。确保遵循网站的爬虫协议和法律规定。
爬取视频时需要注意哪些法律和道德问题?
在爬取视频内容时,必须遵循相关法律法规,包括版权法和网站的服务条款。确保您获得适当的授权或许可,特别是对于受版权保护的内容。此外,避免对网站造成过多的请求负担,以免影响其正常运行。
哪些Python库适合用于视频爬取?
常用的Python库包括requests(用于发送HTTP请求)、BeautifulSoup(用于解析HTML文档)、Selenium(用于处理动态网页)和PyTube(专门用于下载YouTube视频)。此外,使用正则表达式也能有效提取视频链接。根据具体需求选择合适的工具将提高爬取效率。
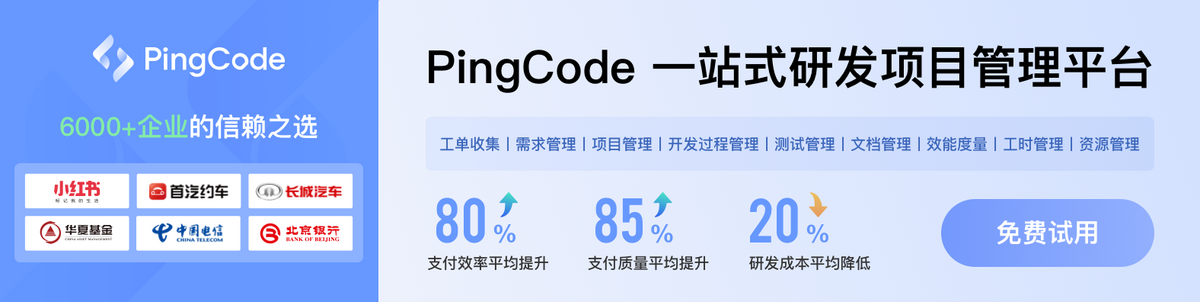