使用Python编写多线程代码、使用多进程、使用异步编程是实现并发编程的三种常见方法。本文将详细介绍如何在Python中使用这些方法编写高效的并发代码。
一、使用多线程
多线程是指在一个进程内创建多个线程,线程是CPU调度的基本单位。Python通过threading
模块来实现多线程编程。在Python中,由于全局解释器锁(GIL)的存在,多线程在计算密集型任务中并不能真正并行执行,但是对于I/O密集型任务,多线程仍然是非常有效的解决方案。
1.1 创建并启动线程
通过继承threading.Thread
类,我们可以创建自己的线程类,并重写run
方法来定义线程的任务。
import threading
import time
class MyThread(threading.Thread):
def __init__(self, name):
threading.Thread.__init__(self)
self.name = name
def run(self):
print(f"Thread {self.name} started")
time.sleep(2)
print(f"Thread {self.name} finished")
创建并启动线程
thread1 = MyThread("A")
thread2 = MyThread("B")
thread1.start()
thread2.start()
thread1.join()
thread2.join()
print("All threads completed")
1.2 线程同步
在多线程编程中,数据共享可能会导致竞争条件。为了避免这种情况,我们可以使用线程同步机制,例如锁(Lock)。
import threading
import time
class SafeCounter:
def __init__(self):
self.lock = threading.Lock()
self.count = 0
def increment(self):
with self.lock:
temp = self.count
time.sleep(0.1)
self.count = temp + 1
counter = SafeCounter()
def worker():
for _ in range(10):
counter.increment()
threads = []
for _ in range(5):
t = threading.Thread(target=worker)
threads.append(t)
t.start()
for t in threads:
t.join()
print(f"Final count: {counter.count}")
二、使用多进程
多进程是指在操作系统中创建多个进程,每个进程有独立的内存空间。Python通过multiprocessing
模块来实现多进程编程。多进程可以绕过GIL的限制,真正实现并行计算,非常适合计算密集型任务。
2.1 创建并启动进程
通过继承multiprocessing.Process
类,我们可以创建自己的进程类,并重写run
方法来定义进程的任务。
import multiprocessing
import time
class MyProcess(multiprocessing.Process):
def __init__(self, name):
multiprocessing.Process.__init__(self)
self.name = name
def run(self):
print(f"Process {self.name} started")
time.sleep(2)
print(f"Process {self.name} finished")
创建并启动进程
process1 = MyProcess("A")
process2 = MyProcess("B")
process1.start()
process2.start()
process1.join()
process2.join()
print("All processes completed")
2.2 进程间通信
在多进程编程中,进程间通信(IPC)是非常重要的。我们可以使用管道(Pipe)和队列(Queue)来实现进程间通信。
import multiprocessing
def worker(pipe):
pipe.send("Hello from child process!")
print(f"Child process received: {pipe.recv()}")
parent_conn, child_conn = multiprocessing.Pipe()
p = multiprocessing.Process(target=worker, args=(child_conn,))
p.start()
print(f"Parent process received: {parent_conn.recv()}")
parent_conn.send("Hello from parent process!")
p.join()
三、使用异步编程
异步编程是一种并发编程的范式,通过异步I/O操作实现并发。Python通过asyncio
模块来实现异步编程。异步编程在处理大量I/O密集型任务时非常高效。
3.1 定义异步函数
通过使用async def
关键字来定义异步函数,并使用await
关键字来执行异步操作。
import asyncio
async def say_hello(name):
print(f"Hello {name}!")
await asyncio.sleep(1)
print(f"Goodbye {name}!")
async def main():
await asyncio.gather(
say_hello("Alice"),
say_hello("Bob"),
)
asyncio.run(main())
3.2 异步任务调度
我们可以使用asyncio.create_task
函数来创建异步任务,并调度执行。
import asyncio
async def say_hello(name):
print(f"Hello {name}!")
await asyncio.sleep(1)
print(f"Goodbye {name}!")
async def main():
task1 = asyncio.create_task(say_hello("Alice"))
task2 = asyncio.create_task(say_hello("Bob"))
await task1
await task2
asyncio.run(main())
四、结合多种技术
在实际应用中,我们可以结合使用多线程、多进程和异步编程来实现高效的并发程序。下面是一个结合多进程和异步编程的示例。
import multiprocessing
import asyncio
def worker(pipe):
async def async_task():
print("Async task started")
await asyncio.sleep(1)
print("Async task finished")
async def main():
await async_task()
pipe.send("Message from async task")
asyncio.run(main())
parent_conn, child_conn = multiprocessing.Pipe()
p = multiprocessing.Process(target=worker, args=(child_conn,))
p.start()
print(f"Parent process received: {parent_conn.recv()}")
p.join()
五、总结
Python提供了多种并发编程的方法,包括多线程、多进程和异步编程。多线程适用于I/O密集型任务,多进程适用于计算密集型任务,而异步编程适用于大量I/O操作的任务。在实际开发中,我们可以根据具体需求选择合适的并发编程方法,并结合使用多种技术来实现高效的并发程序。
相关问答FAQs:
如何在Python中实现代码重用?
在Python中实现代码重用的常见方法是通过定义函数和类。函数允许您将代码块封装在一个可重复调用的单位中,而类则提供了面向对象编程的能力,使您能够创建具有特定属性和方法的对象。使用模块和包也是一种有效的方式,可以将功能分开并在不同的项目中共享。
使用Python编写代码时,怎样提高代码的可读性?
提高Python代码可读性的重要方法包括使用有意义的变量和函数命名、遵循PEP 8编码规范、合理使用注释和文档字符串。保持代码结构清晰,避免过长的函数或类也是提升可读性的关键。适当的缩进和空行有助于增强代码的层次感,使其更易于理解。
在Python中如何进行代码调试和测试?
在Python中进行代码调试和测试的常用工具包括内置的pdb调试器、pytest测试框架和unittest模块。您可以通过设置断点、单步执行代码来检查变量的状态,以及使用断言语句来验证代码行为。编写单元测试可以帮助确保您的代码在修改后仍然正常工作,从而提高代码的稳定性和可靠性。
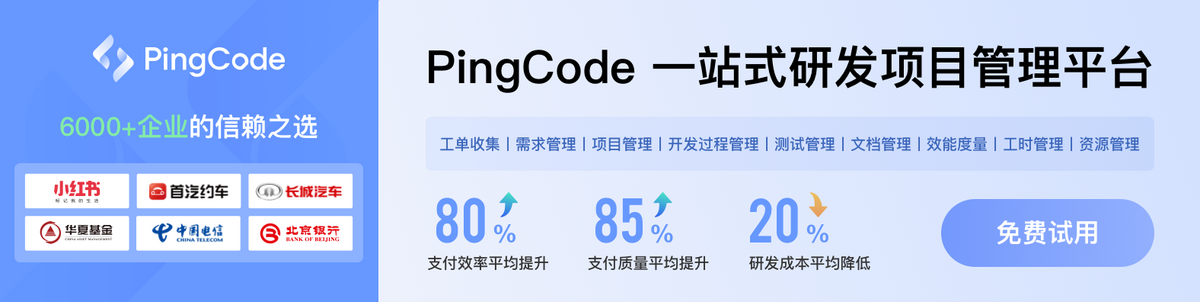