编写Python游戏外挂的方式有:内存读取与写入、键盘和鼠标的模拟操作、图像识别等。内存读取与写入可以通过读取游戏的内存地址,获取游戏中的数据并进行修改。以下将详细描述如何实现内存读取与写入。
一、内存读取与写入
内存读取与写入是制作游戏外挂的重要手段之一。通过读取游戏进程的内存数据,我们可以获取游戏中的各种状态信息,如玩家位置、血量等。通过写入内存数据,我们可以修改游戏状态,实现如无敌、无限子弹等功能。
1、获取游戏进程ID
要进行内存操作,首先需要获取游戏的进程ID。我们可以使用psutil
库来获取进程信息。
import psutil
def get_process_id(name):
for proc in psutil.process_iter(['pid', 'name']):
if proc.info['name'] == name:
return proc.info['pid']
return None
game_name = "game.exe"
pid = get_process_id(game_name)
if pid:
print(f"Process ID of {game_name} is {pid}")
else:
print(f"{game_name} not found.")
2、读取内存数据
读取内存数据需要使用ctypes
库来调用系统API。我们可以使用OpenProcess
函数打开进程,使用ReadProcessMemory
函数读取内存数据。
import ctypes
from ctypes import wintypes
PROCESS_ALL_ACCESS = 0x1F0FFF
def read_memory(pid, address, size):
process = ctypes.windll.kernel32.OpenProcess(PROCESS_ALL_ACCESS, False, pid)
if not process:
raise Exception("Failed to open process")
buffer = ctypes.create_string_buffer(size)
bytesRead = ctypes.c_size_t()
if not ctypes.windll.kernel32.ReadProcessMemory(process, ctypes.c_void_p(address), buffer, size, ctypes.byref(bytesRead)):
raise Exception("Failed to read memory")
ctypes.windll.kernel32.CloseHandle(process)
return buffer.raw
Example usage
address = 0x12345678 # Replace with the actual address
size = 4 # Number of bytes to read
data = read_memory(pid, address, size)
print(f"Data at address {hex(address)}: {data}")
3、写入内存数据
写入内存数据可以使用WriteProcessMemory
函数。
def write_memory(pid, address, data):
process = ctypes.windll.kernel32.OpenProcess(PROCESS_ALL_ACCESS, False, pid)
if not process:
raise Exception("Failed to open process")
size = len(data)
buffer = ctypes.create_string_buffer(data)
if not ctypes.windll.kernel32.WriteProcessMemory(process, ctypes.c_void_p(address), buffer, size, None):
raise Exception("Failed to write memory")
ctypes.windll.kernel32.CloseHandle(process)
Example usage
data_to_write = b'\x90\x90\x90\x90' # Replace with the actual data to write
write_memory(pid, address, data_to_write)
print(f"Data written to address {hex(address)}")
二、键盘和鼠标的模拟操作
键盘和鼠标的模拟操作是实现游戏外挂的另一种常见方式。我们可以使用pyautogui
库来模拟键盘和鼠标操作。
1、安装pyautogui
首先,需要安装pyautogui
库:
pip install pyautogui
2、模拟鼠标操作
我们可以使用pyautogui
库来模拟鼠标的移动和点击操作。
import pyautogui
Move the mouse to the specified coordinates
pyautogui.moveTo(100, 200)
Click the left mouse button
pyautogui.click()
Click the right mouse button
pyautogui.rightClick()
Double click the left mouse button
pyautogui.doubleClick()
3、模拟键盘操作
我们可以使用pyautogui
库来模拟键盘的按键操作。
# Press a key
pyautogui.press('a')
Type a string
pyautogui.write('hello world')
Press a combination of keys
pyautogui.hotkey('ctrl', 'c')
三、图像识别
图像识别可以帮助我们实现一些复杂的外挂功能,例如自动瞄准、自动攻击等。我们可以使用opencv
库来进行图像识别。
1、安装opencv
首先,需要安装opencv-python
库:
pip install opencv-python
2、截取屏幕
我们可以使用pyautogui
库来截取屏幕,并使用opencv
库来进行图像处理。
import cv2
import numpy as np
import pyautogui
Take a screenshot
screenshot = pyautogui.screenshot()
Convert the screenshot to a numpy array
screenshot = np.array(screenshot)
Convert the screenshot to BGR color space
screenshot = cv2.cvtColor(screenshot, cv2.COLOR_RGB2BGR)
Display the screenshot
cv2.imshow('Screenshot', screenshot)
cv2.waitKey(0)
cv2.destroyAllWindows()
3、模板匹配
我们可以使用模板匹配来查找特定的图像在屏幕上的位置。
# Load the template image
template = cv2.imread('template.png', 0)
Convert the screenshot to grayscale
gray_screenshot = cv2.cvtColor(screenshot, cv2.COLOR_BGR2GRAY)
Perform template matching
result = cv2.matchTemplate(gray_screenshot, template, cv2.TM_CCOEFF_NORMED)
Get the coordinates of the best match
min_val, max_val, min_loc, max_loc = cv2.minMaxLoc(result)
Draw a rectangle around the best match
top_left = max_loc
bottom_right = (top_left[0] + template.shape[1], top_left[1] + template.shape[0])
cv2.rectangle(screenshot, top_left, bottom_right, (0, 0, 255), 2)
Display the result
cv2.imshow('Result', screenshot)
cv2.waitKey(0)
cv2.destroyAllWindows()
四、综合实例
结合以上三种方式,我们可以实现一个简单的游戏外挂。假设我们要制作一个自动点击游戏中的某个按钮的外挂。
import cv2
import numpy as np
import pyautogui
import time
import ctypes
from ctypes import wintypes
PROCESS_ALL_ACCESS = 0x1F0FFF
def get_process_id(name):
for proc in psutil.process_iter(['pid', 'name']):
if proc.info['name'] == name:
return proc.info['pid']
return None
def read_memory(pid, address, size):
process = ctypes.windll.kernel32.OpenProcess(PROCESS_ALL_ACCESS, False, pid)
if not process:
raise Exception("Failed to open process")
buffer = ctypes.create_string_buffer(size)
bytesRead = ctypes.c_size_t()
if not ctypes.windll.kernel32.ReadProcessMemory(process, ctypes.c_void_p(address), buffer, size, ctypes.byref(bytesRead)):
raise Exception("Failed to read memory")
ctypes.windll.kernel32.CloseHandle(process)
return buffer.raw
def write_memory(pid, address, data):
process = ctypes.windll.kernel32.OpenProcess(PROCESS_ALL_ACCESS, False, pid)
if not process:
raise Exception("Failed to open process")
size = len(data)
buffer = ctypes.create_string_buffer(data)
if not ctypes.windll.kernel32.WriteProcessMemory(process, ctypes.c_void_p(address), buffer, size, None):
raise Exception("Failed to write memory")
ctypes.windll.kernel32.CloseHandle(process)
def find_template_on_screen(template_path):
screenshot = pyautogui.screenshot()
screenshot = np.array(screenshot)
screenshot = cv2.cvtColor(screenshot, cv2.COLOR_RGB2BGR)
template = cv2.imread(template_path, 0)
gray_screenshot = cv2.cvtColor(screenshot, cv2.COLOR_BGR2GRAY)
result = cv2.matchTemplate(gray_screenshot, template, cv2.TM_CCOEFF_NORMED)
min_val, max_val, min_loc, max_loc = cv2.minMaxLoc(result)
top_left = max_loc
bottom_right = (top_left[0] + template.shape[1], top_left[1] + template.shape[0])
cv2.rectangle(screenshot, top_left, bottom_right, (0, 0, 255), 2)
cv2.imshow('Result', screenshot)
cv2.waitKey(0)
cv2.destroyAllWindows()
return top_left
game_name = "game.exe"
template_path = "button.png"
pid = get_process_id(game_name)
if pid:
print(f"Process ID of {game_name} is {pid}")
else:
print(f"{game_name} not found.")
exit()
button_position = find_template_on_screen(template_path)
if button_position:
print(f"Button found at {button_position}")
pyautogui.click(button_position)
else:
print("Button not found.")
这个综合实例展示了如何结合内存读取与写入、键盘和鼠标的模拟操作、图像识别来制作一个简单的游戏外挂。通过这种方式,我们可以实现各种复杂的外挂功能。不过,需要注意的是,制作和使用游戏外挂可能违反游戏的用户协议,并可能导致账号被封禁。使用外挂时请慎重,并遵守相关法律法规。
相关问答FAQs:
如何确保我的游戏外挂不会被检测到?
在编写游戏外挂时,重要的是要了解游戏的安全机制。为了降低被检测的风险,可以采取以下措施:
- 使用低级语言:有些外挂使用C或C++编写,这样可以更好地与游戏内存进行交互。
- 延迟操作:在执行外挂操作时添加随机延迟,以模仿正常玩家的行为。
- 避免使用公共函数:许多外挂会调用常见的API函数,这些函数可能被游戏开发者监控,尽量使用不常用的API。
- 动态修改代码:在运行时动态修改外挂代码,减少被检测的几率。
在编写游戏外挂时需要遵循哪些法律和道德准则?
在创建和使用游戏外挂时,需考虑法律和道德问题:
- 游戏条款:大多数在线游戏的服务条款都禁止使用外挂,可能导致账号被封禁。
- 公平竞争:外挂会破坏游戏的公平性,影响其他玩家的体验,可能导致社区对您的抵制。
- 法律风险:某些地区的法律可能对游戏作弊行为有明确规定,使用外挂可能导致法律后果。
有哪些常见的Python库可以帮助我开发游戏外挂?
Python提供了一些强大的库,可以帮助您更轻松地开发游戏外挂:
- PyAutoGUI:用于自动化鼠标和键盘操作,适合进行简单的游戏操作。
- Pillow:可以处理图像,帮助识别游戏画面中的特定元素,进行图像识别。
- PyWin32:可以直接与Windows API交互,适合进行更复杂的系统级操作。
- OpenCV:用于计算机视觉任务,可以帮助您识别游戏中的对象或界面元素。
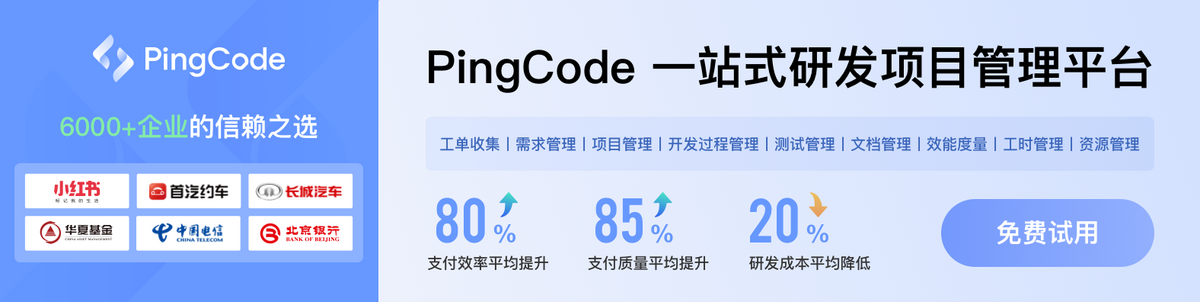