要在Python中显示网页源代码,可以使用requests库、BeautifulSoup库、urllib库等方式。通过requests库获取网页内容、使用BeautifulSoup库解析HTML、使用urllib库获取网页内容。下面将详细描述其中的一种方式,即通过requests库获取网页内容并显示源代码。
requests库获取网页内容:
requests库是Python中非常流行的HTTP库,它可以用来发送HTTP请求,并接收服务器的响应。通过requests库,我们可以很方便地获取网页的内容并显示其源代码。以下是一个基本的示例:
import requests
发送HTTP GET请求
response = requests.get('http://example.com')
获取网页源代码
source_code = response.text
显示网页源代码
print(source_code)
在这个示例中,我们首先导入了requests库,然后通过requests.get()函数发送一个HTTP GET请求到指定的URL。请求成功后,服务器会返回一个响应对象,我们可以通过response.text属性获取网页的源代码,并将其打印出来。
一、使用requests库显示网页源代码
1. 安装requests库
在使用requests库之前,需要先安装它。你可以通过以下命令安装requests库:
pip install requests
2. 发送HTTP请求
使用requests库发送HTTP请求非常简单。你可以使用requests.get()方法发送GET请求,requests.post()方法发送POST请求,等等。以下是发送GET请求的示例:
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
print('请求成功')
else:
print('请求失败')
在这个示例中,我们首先定义了一个URL,然后通过requests.get()方法发送GET请求。如果请求成功,response.status_code将等于200。
3. 获取网页源代码
在获取响应对象后,可以通过response.text属性获取网页的源代码。以下是示例:
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
source_code = response.text
print(source_code)
else:
print('请求失败')
在这个示例中,我们在请求成功后,通过response.text属性获取网页源代码,并将其打印出来。
二、使用BeautifulSoup库解析HTML
1. 安装BeautifulSoup库
BeautifulSoup是一个用于解析HTML和XML的Python库。你可以通过以下命令安装BeautifulSoup库:
pip install beautifulsoup4
2. 导入BeautifulSoup库
在使用BeautifulSoup库之前,需要先导入它。以下是示例:
from bs4 import BeautifulSoup
3. 解析HTML
在获取网页源代码后,可以使用BeautifulSoup库解析HTML。以下是示例:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
source_code = response.text
soup = BeautifulSoup(source_code, 'html.parser')
print(soup.prettify())
else:
print('请求失败')
在这个示例中,我们首先通过requests库获取网页源代码,然后使用BeautifulSoup库解析HTML。最后,通过soup.prettify()方法将解析后的HTML格式化并打印出来。
三、使用urllib库获取网页内容
1. 导入urllib库
urllib是Python内置的一个用于处理URL的库。你可以通过以下方式导入urllib库:
import urllib.request
2. 发送HTTP请求
使用urllib库发送HTTP请求也非常简单。你可以使用urllib.request.urlopen()方法发送GET请求。以下是示例:
import urllib.request
url = 'http://example.com'
response = urllib.request.urlopen(url)
if response.status == 200:
print('请求成功')
else:
print('请求失败')
在这个示例中,我们首先定义了一个URL,然后通过urllib.request.urlopen()方法发送GET请求。如果请求成功,response.status将等于200。
3. 获取网页源代码
在获取响应对象后,可以通过response.read()方法获取网页的源代码。以下是示例:
import urllib.request
url = 'http://example.com'
response = urllib.request.urlopen(url)
if response.status == 200:
source_code = response.read().decode('utf-8')
print(source_code)
else:
print('请求失败')
在这个示例中,我们在请求成功后,通过response.read()方法获取网页源代码,并通过decode('utf-8')方法将其解码为字符串,然后将其打印出来。
四、使用selenium库获取动态网页内容
1. 安装selenium库
Selenium是一个用于自动化Web浏览器的工具,可以用于获取动态网页的内容。你可以通过以下命令安装Selenium库:
pip install selenium
2. 安装浏览器驱动
Selenium需要使用浏览器驱动来控制浏览器。例如,如果你使用Chrome浏览器,需要下载并安装ChromeDriver。你可以从以下链接下载ChromeDriver:
https://sites.google.com/a/chromium.org/chromedriver/downloads
下载并解压后,将ChromeDriver的路径添加到系统的环境变量中。
3. 导入Selenium库
在使用Selenium库之前,需要先导入它。以下是示例:
from selenium import webdriver
4. 获取动态网页内容
使用Selenium库获取动态网页内容非常简单。你可以使用webdriver对象控制浏览器,获取网页内容。以下是示例:
from selenium import webdriver
url = 'http://example.com'
driver = webdriver.Chrome()
driver.get(url)
source_code = driver.page_source
print(source_code)
driver.quit()
在这个示例中,我们首先创建了一个webdriver对象,然后通过driver.get()方法访问指定的URL。获取网页内容后,通过driver.page_source属性获取网页的源代码,并将其打印出来。最后,通过driver.quit()方法关闭浏览器。
五、处理网页内容中的动态数据
有时候,网页内容是通过JavaScript动态加载的,这种情况下,使用requests库或urllib库可能无法获取完整的网页源代码。此时,可以使用Selenium库来处理这种情况。
以下是一个示例,展示如何使用Selenium库处理动态数据:
from selenium import webdriver
import time
url = 'http://example.com'
driver = webdriver.Chrome()
driver.get(url)
time.sleep(5) # 等待页面加载完成
source_code = driver.page_source
print(source_code)
driver.quit()
在这个示例中,我们通过time.sleep()方法等待页面加载完成,然后获取网页的源代码。
六、解析网页内容中的特定元素
在获取网页源代码后,可以使用BeautifulSoup库解析HTML,并提取特定元素的内容。以下是示例:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
source_code = response.text
soup = BeautifulSoup(source_code, 'html.parser')
# 提取特定元素
title = soup.title.string
print('标题:', title)
# 提取所有链接
links = soup.find_all('a')
for link in links:
print('链接:', link.get('href'))
else:
print('请求失败')
在这个示例中,我们首先通过requests库获取网页源代码,然后使用BeautifulSoup库解析HTML。接着,通过soup.title.string获取网页的标题,通过soup.find_all('a')获取网页中的所有链接,并将它们打印出来。
七、处理网页内容中的表单数据
在某些情况下,你可能需要处理网页中的表单数据,例如提交表单、获取表单中的数据等。以下是一个示例,展示如何使用requests库处理表单数据:
import requests
url = 'http://example.com/form'
data = {
'username': 'testuser',
'password': 'testpassword'
}
response = requests.post(url, data=data)
if response.status_code == 200:
print('表单提交成功')
else:
print('表单提交失败')
在这个示例中,我们首先定义了一个URL和一个包含表单数据的字典,然后通过requests.post()方法发送POST请求提交表单。如果请求成功,response.status_code将等于200。
八、处理网页内容中的Cookies
在某些情况下,你可能需要处理网页中的Cookies,例如获取Cookies、设置Cookies等。以下是一个示例,展示如何使用requests库处理Cookies:
import requests
url = 'http://example.com'
session = requests.Session()
response = session.get(url)
if response.status_code == 200:
cookies = session.cookies.get_dict()
print('Cookies:', cookies)
else:
print('请求失败')
设置Cookies
cookies = {
'sessionid': '123456'
}
response = session.get(url, cookies=cookies)
if response.status_code == 200:
print('请求成功')
else:
print('请求失败')
在这个示例中,我们首先创建了一个Session对象,然后通过session.get()方法发送GET请求获取网页内容。如果请求成功,可以通过session.cookies.get_dict()获取Cookies。接着,我们可以通过cookies参数设置Cookies,并发送请求。
九、处理网页内容中的Headers
在某些情况下,你可能需要处理网页中的Headers,例如设置自定义Headers、获取响应Headers等。以下是一个示例,展示如何使用requests库处理Headers:
import requests
url = 'http://example.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36'
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
print('请求成功')
print('响应Headers:', response.headers)
else:
print('请求失败')
在这个示例中,我们首先定义了一个URL和一个包含自定义Headers的字典,然后通过requests.get()方法发送GET请求并设置Headers。如果请求成功,可以通过response.headers获取响应Headers。
十、处理网页内容中的代理
在某些情况下,你可能需要使用代理来访问网页。以下是一个示例,展示如何使用requests库处理代理:
import requests
url = 'http://example.com'
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get(url, proxies=proxies)
if response.status_code == 200:
print('请求成功')
else:
print('请求失败')
在这个示例中,我们首先定义了一个URL和一个包含代理服务器地址的字典,然后通过requests.get()方法发送GET请求并设置代理。如果请求成功,response.status_code将等于200。
十一、处理网页内容中的重定向
在某些情况下,网页可能会发生重定向。以下是一个示例,展示如何使用requests库处理重定向:
import requests
url = 'http://example.com'
response = requests.get(url, allow_redirects=True)
if response.status_code == 200:
print('请求成功')
print('最终URL:', response.url)
else:
print('请求失败')
在这个示例中,我们通过requests.get()方法发送GET请求,并设置allow_redirects=True以允许重定向。如果请求成功,可以通过response.url获取最终的URL。
十二、处理网页内容中的超时
在某些情况下,网页请求可能会超时。以下是一个示例,展示如何使用requests库处理超时:
import requests
url = 'http://example.com'
try:
response = requests.get(url, timeout=5)
if response.status_code == 200:
print('请求成功')
else:
print('请求失败')
except requests.exceptions.Timeout:
print('请求超时')
在这个示例中,我们通过requests.get()方法发送GET请求,并设置timeout=5以指定超时时间为5秒。如果请求超时,将会抛出requests.exceptions.Timeout异常。
十三、处理网页内容中的认证
在某些情况下,网页可能需要认证。以下是一个示例,展示如何使用requests库处理认证:
import requests
from requests.auth import HTTPBasicAuth
url = 'http://example.com'
response = requests.get(url, auth=HTTPBasicAuth('username', 'password'))
if response.status_code == 200:
print('请求成功')
else:
print('请求失败')
在这个示例中,我们通过requests.get()方法发送GET请求,并使用HTTPBasicAuth进行基本认证。如果请求成功,response.status_code将等于200。
十四、处理网页内容中的文件下载
在某些情况下,你可能需要下载网页中的文件。以下是一个示例,展示如何使用requests库下载文件:
import requests
url = 'http://example.com/file.zip'
response = requests.get(url, stream=True)
if response.status_code == 200:
with open('file.zip', 'wb') as file:
for chunk in response.iter_content(chunk_size=1024):
file.write(chunk)
print('文件下载成功')
else:
print('文件下载失败')
在这个示例中,我们通过requests.get()方法发送GET请求,并设置stream=True以流式下载文件。然后,通过response.iter_content(chunk_size=1024)以1024字节为单位迭代下载内容,并将其写入文件。
十五、处理网页内容中的多部分表单数据
在某些情况下,你可能需要处理网页中的多部分表单数据,例如上传文件。以下是一个示例,展示如何使用requests库处理多部分表单数据:
import requests
url = 'http://example.com/upload'
files = {
'file': ('filename.txt', open('filename.txt', 'rb'))
}
response = requests.post(url, files=files)
if response.status_code == 200:
print('文件上传成功')
else:
print('文件上传失败')
在这个示例中,我们通过requests.post()方法发送POST请求,并使用files参数上传文件。如果请求成功,response.status_code将等于200。
总结起来,Python提供了多种方式来显示网页源代码,包括使用requests库、BeautifulSoup库、urllib库、Selenium库等。通过结合使用这些库,可以处理各种复杂的网页内容,包括动态加载的数据、表单数据、Cookies、Headers、代理、重定向、超时、认证、文件下载和多部分表单数据等。希望本文能够帮助你更好地理解和使用Python来显示和处理网页源代码。
相关问答FAQs:
如何在Python中获取网页源代码?
要在Python中获取网页源代码,您可以使用requests
库。首先,确保您已安装此库。可以使用命令pip install requests
进行安装。然后,您可以编写以下代码:
import requests
url = 'https://example.com' # 替换为您要获取源代码的网页地址
response = requests.get(url)
source_code = response.text
print(source_code)
这段代码将发送一个GET请求到指定的URL,并返回网页的源代码。
使用Python查看网页源代码的其他方法有哪些?
除了requests
库,您还可以使用urllib
库来获取网页源代码。以下是一个使用urllib
的示例:
import urllib.request
url = 'https://example.com' # 替换为您要获取源代码的网页地址
response = urllib.request.urlopen(url)
source_code = response.read().decode('utf-8')
print(source_code)
这种方法同样可以让您访问网页的源代码,适用于不同的场景。
如何使用Beautiful Soup解析网页源代码?
获取网页源代码后,您可能希望解析和提取特定信息。Beautiful Soup
是一个强大的库,可以帮助您完成这项任务。您可以通过以下步骤使用它:
- 安装
Beautiful Soup
库:pip install beautifulsoup4
。 - 使用以下代码解析网页源代码:
from bs4 import BeautifulSoup
import requests
url = 'https://example.com' # 替换为您要获取源代码的网页地址
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 示例:提取网页标题
title = soup.title.string
print(title)
这段代码将获取网页源代码并使用Beautiful Soup解析,从中提取网页标题。
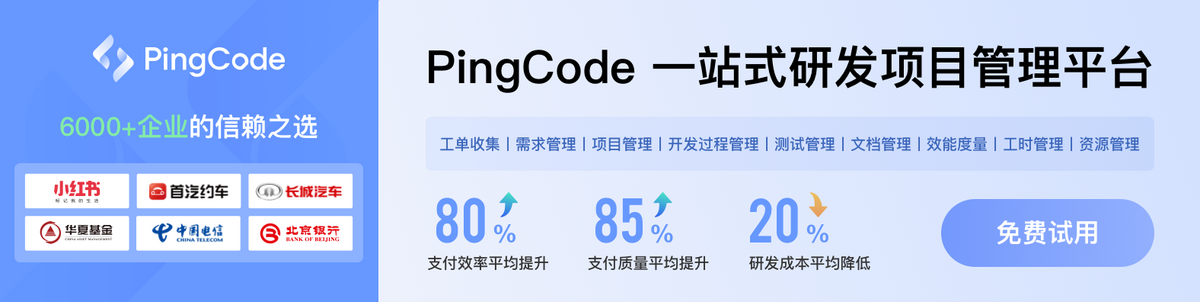