使用Python批量修改文件内容的步骤包括读取文件列表、遍历文件、读取和修改文件内容、保存修改后的文件。以下是具体步骤和详细描述:
- 获取文件列表、2. 读取文件内容、3. 修改文件内容、4. 保存修改后的文件。我们以修改文件中某个特定的词汇为例,展开详细描述。
获取文件列表是第一步,通常我们会使用os库来遍历目录,获取所有需要修改的文件。以下是一个示例代码:
import os
def get_files(directory, extension):
files = []
for root, _, filenames in os.walk(directory):
for filename in filenames:
if filename.endswith(extension):
files.append(os.path.join(root, filename))
return files
读取文件内容是第二步,可以使用内置的open函数来读取文件内容。示例如下:
def read_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
修改文件内容是第三步,我们可以使用字符串的replace方法来替换指定的内容:
def modify_content(content, old_word, new_word):
return content.replace(old_word, new_word)
保存修改后的文件是最后一步,依然使用open函数,但需要以写入模式打开文件:
def write_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
将所有步骤综合起来,我们可以写一个完整的批量修改文件内容的程序:
import os
def get_files(directory, extension):
files = []
for root, _, filenames in os.walk(directory):
for filename in filenames:
if filename.endswith(extension):
files.append(os.path.join(root, filename))
return files
def read_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
def modify_content(content, old_word, new_word):
return content.replace(old_word, new_word)
def write_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
def batch_modify_files(directory, extension, old_word, new_word):
files = get_files(directory, extension)
for file_path in files:
content = read_file(file_path)
modified_content = modify_content(content, old_word, new_word)
write_file(file_path, modified_content)
Example usage
directory = 'path_to_directory'
extension = '.txt'
old_word = 'old_word'
new_word = 'new_word'
batch_modify_files(directory, extension, old_word, new_word)
通过以上步骤,我们可以使用Python高效地批量修改文件内容。下面我们将详细描述每一步的实现以及可能遇到的问题和解决方法。
一、获取文件列表
在批量修改文件内容的过程中,首先需要确定哪些文件需要进行修改。这通常涉及遍历指定的目录,并根据文件扩展名或其他条件筛选出目标文件。使用os库的os.walk函数是一个常见且高效的方式,可以递归遍历目录及其子目录,获取文件列表。
import os
def get_files(directory, extension):
files = []
for root, _, filenames in os.walk(directory):
for filename in filenames:
if filename.endswith(extension):
files.append(os.path.join(root, filename))
return files
在这个函数中,os.walk
会遍历给定目录directory
中的所有文件和子目录。root
表示当前遍历的目录,_
是一个包含子目录的列表(在这个例子中我们不需要它,所以用_
占位),filenames
是当前目录下的所有文件名列表。我们通过检查文件名是否以指定的扩展名结尾来筛选目标文件,并将其完整路径添加到files
列表中。
二、读取文件内容
读取文件内容是修改文件的前提。Python内置的open
函数可以方便地读取文件内容。我们通常会以只读模式打开文件,并使用read
方法读取整个文件内容。
def read_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
在这个函数中,with open(file_path, 'r') as file
语句会以只读模式打开指定路径的文件,并在操作完成后自动关闭文件,确保资源得到释放。file.read()
方法会读取整个文件的内容,并将其返回。
三、修改文件内容
读取文件内容后,我们需要对内容进行修改。通常我们会使用字符串的replace
方法来替换指定的内容。这个方法会返回一个新的字符串,其中所有匹配的子字符串都被替换为新的子字符串。
def modify_content(content, old_word, new_word):
return content.replace(old_word, new_word)
在这个函数中,content.replace(old_word, new_word)
会将content
字符串中的所有old_word
替换为new_word
,并返回修改后的内容。
四、保存修改后的文件
完成内容修改后,我们需要将修改后的内容保存回文件中。依然使用open
函数,但这次需要以写入模式打开文件。
def write_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
在这个函数中,with open(file_path, 'w') as file
语句会以写入模式打开指定路径的文件。如果文件不存在,它会被创建;如果文件存在,它的内容会被清空。file.write(content)
方法会将content
字符串写入文件。
五、综合实现
将以上所有步骤综合起来,我们可以写一个完整的批量修改文件内容的程序。这个程序会遍历指定目录及其子目录中的所有目标文件,读取文件内容,进行修改,并将修改后的内容保存回文件。
import os
def get_files(directory, extension):
files = []
for root, _, filenames in os.walk(directory):
for filename in filenames:
if filename.endswith(extension):
files.append(os.path.join(root, filename))
return files
def read_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
def modify_content(content, old_word, new_word):
return content.replace(old_word, new_word)
def write_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
def batch_modify_files(directory, extension, old_word, new_word):
files = get_files(directory, extension)
for file_path in files:
content = read_file(file_path)
modified_content = modify_content(content, old_word, new_word)
write_file(file_path, modified_content)
Example usage
directory = 'path_to_directory'
extension = '.txt'
old_word = 'old_word'
new_word = 'new_word'
batch_modify_files(directory, extension, old_word, new_word)
六、处理特殊情况
在实际应用中,我们可能会遇到各种特殊情况,如文件编码问题、大文件处理、多行替换等。以下是一些常见问题及其解决方法。
1、文件编码问题
在处理文件时,文件编码问题是一个常见的坑。如果文件使用非默认编码(如UTF-8),我们需要在打开文件时指定编码。
def read_file(file_path, encoding='utf-8'):
with open(file_path, 'r', encoding=encoding) as file:
content = file.read()
return content
def write_file(file_path, content, encoding='utf-8'):
with open(file_path, 'w', encoding=encoding) as file:
file.write(content)
在这些函数中,我们添加了encoding
参数,并在open
函数中指定编码。这样可以确保正确读取和写入文件内容。
2、大文件处理
对于非常大的文件,直接读取和写入可能会导致内存不足。我们可以使用逐行读取和写入的方式来处理大文件。
def modify_file_in_place(file_path, old_word, new_word, encoding='utf-8'):
with open(file_path, 'r', encoding=encoding) as file:
lines = file.readlines()
with open(file_path, 'w', encoding=encoding) as file:
for line in lines:
modified_line = line.replace(old_word, new_word)
file.write(modified_line)
在这个函数中,我们首先逐行读取文件内容,并将每一行的内容存储到lines
列表中。然后,我们逐行处理这些内容,并将修改后的内容写回文件。
3、多行替换
有时我们需要替换的内容可能跨越多行。这时我们可以使用正则表达式来进行匹配和替换。
import re
def modify_content(content, old_pattern, new_pattern):
return re.sub(old_pattern, new_pattern, content, flags=re.DOTALL)
在这个函数中,re.sub
函数会使用正则表达式old_pattern
匹配内容,并将其替换为new_pattern
。flags=re.DOTALL
参数会使.
匹配任何字符,包括换行符,从而实现多行替换。
七、总结
通过以上步骤和示例代码,我们可以使用Python高效地批量修改文件内容。这个过程包括获取文件列表、读取文件内容、修改文件内容、保存修改后的文件等步骤。在实际应用中,我们需要根据具体需求处理各种特殊情况,如文件编码问题、大文件处理、多行替换等。
通过掌握这些技巧和方法,我们可以在Python编程中更加灵活地处理文件,提升工作效率。无论是批量替换文本、更新配置文件,还是进行数据清洗和预处理,Python都提供了强大的工具和库,帮助我们高效地完成任务。
相关问答FAQs:
如何使用Python批量修改多个文件的内容?
使用Python批量修改文件内容,可以通过编写脚本来实现。通常,您可以使用os
和re
模块来遍历文件夹中的文件,读取每个文件的内容,并根据需要进行替换或修改。例如,您可以使用正则表达式来查找特定的文本并进行替换。完成后,将修改后的内容写回原文件或新文件中。
批量修改文件内容时需要注意哪些事项?
在批量修改文件内容时,确保备份原始文件是非常重要的,以免数据丢失。此外,确认修改的内容是准确的,以避免不必要的错误。建议在小范围内测试脚本,以验证其效果后再应用于大量文件。使用版本控制系统(如Git)也是一个不错的选择,可以追踪更改历史。
有哪些常用的Python库可以帮助批量处理文件?
Python中有多个库可以帮助您批量处理文件。os
和shutil
库常用于文件和目录的操作;re
库则用于字符串的匹配和替换。对于更复杂的文件类型,您还可以考虑使用pandas
来处理CSV文件,或使用openpyxl
来处理Excel文件。选择合适的库能够提高处理效率并简化代码。
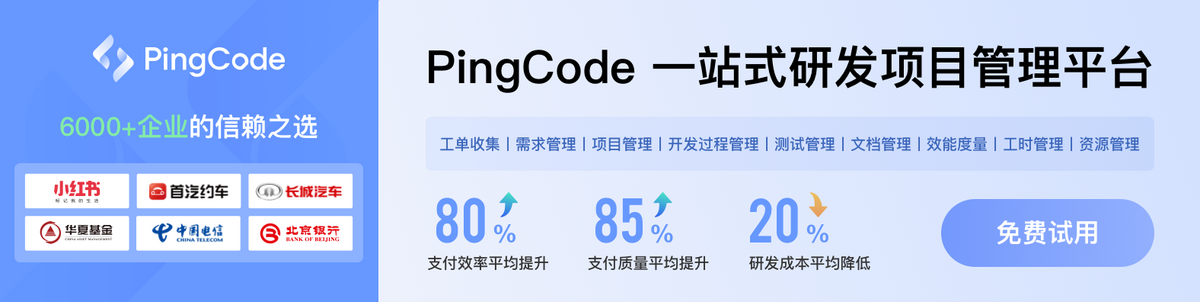