Python 实现边读边写的常用方法包括使用文件的读写模式、使用生成器、使用多线程和多进程。其中,使用生成器是一种非常高效的方法,可以处理大文件,避免内存占用过高。生成器通过惰性计算的方式,只在需要时才生成数据,从而实现边读边写的效果。
生成器的详细描述:
生成器是Python中的一种特殊的迭代器,通过yield
关键字生成值。在处理大文件时,可以用生成器逐行读取文件内容,再逐行写入到另一个文件中。这样可以避免一次性将整个文件读入内存,从而实现边读边写。
一、使用文件的读写模式
在Python中,可以通过指定文件的读写模式实现边读边写。常用的模式包括r
(读取)、w
(写入)、a
(追加)和r+
(读写)。例如,可以使用r+
模式同时读取和写入文件:
with open('input.txt', 'r+') as file:
lines = file.readlines()
file.seek(0)
for line in lines:
file.write(line.upper())
这种方法适用于文件较小的情况,因为file.readlines()
会将整个文件读取到内存中。
二、使用生成器
生成器可以在处理大文件时实现边读边写,避免内存占用过高。例如:
def read_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line
def write_file(input_path, output_path):
with open(output_path, 'w') as out_file:
for line in read_file(input_path):
out_file.write(line.upper())
write_file('input.txt', 'output.txt')
这种方法通过生成器逐行读取文件内容,再逐行写入到另一个文件中,非常适合处理大文件。
三、多线程
多线程可以实现同时读取和写入文件,提高处理效率。例如:
import threading
def read_file(file_path, queue):
with open(file_path, 'r') as file:
for line in file:
queue.put(line)
queue.put(None)
def write_file(output_path, queue):
with open(output_path, 'w') as file:
while True:
line = queue.get()
if line is None:
break
file.write(line.upper())
import queue
q = queue.Queue()
read_thread = threading.Thread(target=read_file, args=('input.txt', q))
write_thread = threading.Thread(target=write_file, args=('output.txt', q))
read_thread.start()
write_thread.start()
read_thread.join()
write_thread.join()
这种方法通过线程之间的队列实现数据传递,从而实现边读边写。
四、多进程
多进程可以进一步提高处理效率,适用于CPU密集型任务。例如:
from multiprocessing import Process, Queue
def read_file(file_path, queue):
with open(file_path, 'r') as file:
for line in file:
queue.put(line)
queue.put(None)
def write_file(output_path, queue):
with open(output_path, 'w') as file:
while True:
line = queue.get()
if line is None:
break
file.write(line.upper())
if __name__ == '__main__':
q = Queue()
read_process = Process(target=read_file, args=('input.txt', q))
write_process = Process(target=write_file, args=('output.txt', q))
read_process.start()
write_process.start()
read_process.join()
write_process.join()
这种方法通过进程间的队列实现数据传递,从而实现边读边写,适用于处理大文件或复杂计算任务。
总结
通过使用文件的读写模式、生成器、多线程和多进程,都可以实现Python中边读边写的功能。其中,生成器是一种非常高效的方法,适合处理大文件,避免内存占用过高。多线程和多进程则适用于提高处理效率,尤其适用于CPU密集型任务。根据具体需求选择合适的方法,可以有效实现边读边写。
相关问答FAQs:
如何在Python中实现文件的边读边写?
在Python中,实现边读边写可以使用文件的读写模式。在打开文件时,可以选择'r+'模式,这允许你在读取文件内容的同时进行写入。使用file.read()
和file.write()
方法,可以在处理数据时进行读写操作。
在边读边写时,有哪些常见的错误需要注意?
常见的错误包括文件指针的位置管理。如果在写入时没有正确移动文件指针,可能会导致覆盖意外的数据。此外,确保在写入之前已经读取了需要的数据,避免出现数据丢失或读取不完整的情况。
如何提高边读边写的效率?
为了提高效率,可以采用缓冲区的方式进行读写操作。通过一次性读取较大的数据块,然后在内存中处理这些数据,再写回文件,能够减少文件I/O操作的次数,从而加快整体处理速度。此外,使用with open()
语句可以确保在操作完成后,文件会自动关闭,减少资源浪费。
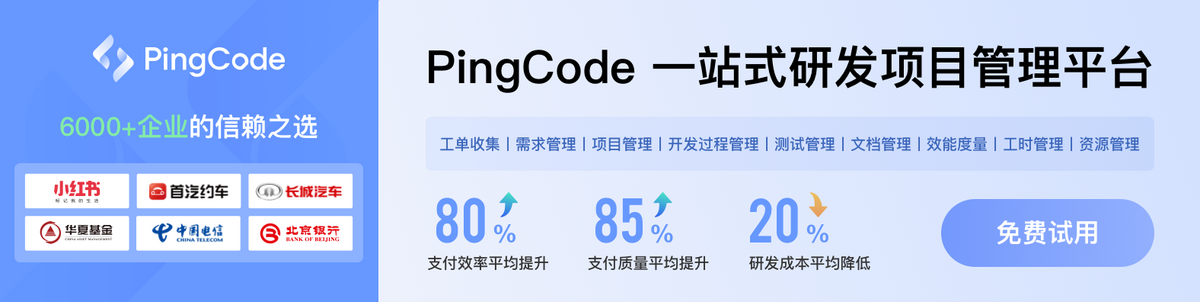