Python创建多个类对象的方法有多种,主要包括:使用循环创建对象、使用列表或字典存储对象、使用工厂模式创建对象。其中,使用循环创建对象是最常见的方法。下面将详细描述使用循环创建对象的方法。
使用循环创建多个类对象
在Python中,可以通过循环来创建多个类对象,并将这些对象存储在一个列表或字典中。假设我们有一个名为Person
的类,我们可以通过循环来创建多个Person
对象。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
使用列表存储多个Person对象
persons = []
for i in range(5):
person = Person(f'Person_{i}', 20 + i)
persons.append(person)
查看创建的对象
for person in persons:
print(f'Name: {person.name}, Age: {person.age}')
在上述代码中,我们定义了一个Person
类,然后使用一个for
循环创建了五个Person
对象,并将这些对象存储在一个列表persons
中。最后,通过另一个for
循环来遍历列表中的对象,并打印每个对象的属性。
一、使用列表存储对象
使用列表存储对象是一种常见的方法,可以方便地管理和操作多个对象。我们可以使用列表来存储不同类型的对象,并通过索引来访问这些对象。
class Animal:
def __init__(self, name, species):
self.name = name
self.species = species
创建多个Animal对象并存储在列表中
animals = [Animal('Lion', 'Mammal'), Animal('Eagle', 'Bird'), Animal('Shark', 'Fish')]
访问列表中的对象
for animal in animals:
print(f'Name: {animal.name}, Species: {animal.species}')
在上述代码中,我们定义了一个Animal
类,并创建了三个Animal
对象。这些对象被存储在一个列表animals
中,然后通过循环遍历列表来访问每个对象的属性。
二、使用字典存储对象
使用字典存储对象可以通过键值对的形式来管理对象,这样可以更加方便地通过键来访问特定的对象。
class Car:
def __init__(self, brand, model):
self.brand = brand
self.model = model
创建多个Car对象并存储在字典中
cars = {
'car1': Car('Toyota', 'Corolla'),
'car2': Car('Honda', 'Civic'),
'car3': Car('Ford', 'Focus')
}
访问字典中的对象
for key, car in cars.items():
print(f'Key: {key}, Brand: {car.brand}, Model: {car.model}')
在上述代码中,我们定义了一个Car
类,并创建了三个Car
对象。这些对象被存储在一个字典cars
中,通过键值对的形式来管理对象。然后,通过循环遍历字典来访问每个对象的属性。
三、使用工厂模式创建对象
工厂模式是一种设计模式,通过定义一个创建对象的接口来创建对象,而不是直接实例化对象。这种方式可以使代码更加灵活和可扩展。
class Shape:
def __init__(self, shape_type):
self.shape_type = shape_type
def draw(self):
print(f'Drawing a {self.shape_type}')
class ShapeFactory:
@staticmethod
def create_shape(shape_type):
return Shape(shape_type)
使用工厂模式创建多个Shape对象
shapes = []
for shape_type in ['Circle', 'Square', 'Triangle']:
shape = ShapeFactory.create_shape(shape_type)
shapes.append(shape)
访问创建的对象
for shape in shapes:
shape.draw()
在上述代码中,我们定义了一个Shape
类和一个ShapeFactory
类。ShapeFactory
类包含一个静态方法create_shape
,该方法用于创建Shape
对象。然后,我们通过工厂模式来创建多个Shape
对象,并将这些对象存储在一个列表shapes
中。最后,通过循环遍历列表来访问每个对象的属性和方法。
四、使用生成器创建对象
生成器是一种特殊的迭代器,可以通过yield
关键字来生成一系列值。在Python中,可以使用生成器来创建多个类对象。
class Book:
def __init__(self, title, author):
self.title = title
self.author = author
def book_generator(titles, authors):
for title, author in zip(titles, authors):
yield Book(title, author)
使用生成器创建多个Book对象
titles = ['Book1', 'Book2', 'Book3']
authors = ['Author1', 'Author2', 'Author3']
books = list(book_generator(titles, authors))
访问生成的对象
for book in books:
print(f'Title: {book.title}, Author: {book.author}')
在上述代码中,我们定义了一个Book
类和一个生成器函数book_generator
。book_generator
函数接受两个列表titles
和authors
,通过yield
关键字生成多个Book
对象。然后,我们使用生成器创建了多个Book
对象,并将这些对象存储在一个列表books
中。最后,通过循环遍历列表来访问每个对象的属性。
五、使用类方法创建对象
类方法是一种绑定到类而不是实例的方法,可以通过类本身来调用。在Python中,可以使用类方法来创建多个类对象。
class Employee:
def __init__(self, name, position):
self.name = name
self.position = position
@classmethod
def create_employees(cls, names, positions):
return [cls(name, position) for name, position in zip(names, positions)]
使用类方法创建多个Employee对象
names = ['Alice', 'Bob', 'Charlie']
positions = ['Manager', 'Engineer', 'Analyst']
employees = Employee.create_employees(names, positions)
访问创建的对象
for employee in employees:
print(f'Name: {employee.name}, Position: {employee.position}')
在上述代码中,我们定义了一个Employee
类和一个类方法create_employees
。create_employees
方法接受两个列表names
和positions
,通过列表解析创建多个Employee
对象。然后,我们使用类方法创建了多个Employee
对象,并将这些对象存储在一个列表employees
中。最后,通过循环遍历列表来访问每个对象的属性。
六、使用元类创建对象
元类是一种用于创建类的类,可以控制类的创建和行为。在Python中,可以使用元类来创建多个类对象。
class Meta(type):
def __new__(cls, name, bases, dct):
return super().__new__(cls, name, bases, dct)
class Product(metaclass=Meta):
def __init__(self, product_name, price):
self.product_name = product_name
self.price = price
使用元类创建多个Product对象
products = [Product(f'Product_{i}', 10 * i) for i in range(5)]
访问创建的对象
for product in products:
print(f'Product Name: {product.product_name}, Price: {product.price}')
在上述代码中,我们定义了一个元类Meta
和一个使用该元类的类Product
。然后,我们通过列表解析创建了多个Product
对象,并将这些对象存储在一个列表products
中。最后,通过循环遍历列表来访问每个对象的属性。
七、使用闭包创建对象
闭包是一种函数对象,可以捕获并记住其所在作用域的变量。在Python中,可以使用闭包来创建多个类对象。
class Device:
def __init__(self, device_name, device_type):
self.device_name = device_name
self.device_type = device_type
def device_closure():
devices = []
def create_device(device_name, device_type):
devices.append(Device(device_name, device_type))
return create_device, devices
使用闭包创建多个Device对象
create_device, devices = device_closure()
create_device('Device1', 'Type1')
create_device('Device2', 'Type2')
create_device('Device3', 'Type3')
访问创建的对象
for device in devices:
print(f'Device Name: {device.device_name}, Device Type: {device.device_type}')
在上述代码中,我们定义了一个Device
类和一个闭包device_closure
。device_closure
函数返回一个函数create_device
和一个列表devices
,create_device
函数用于创建Device
对象并将其添加到列表devices
中。然后,我们通过调用create_device
函数创建了多个Device
对象,并将这些对象存储在列表devices
中。最后,通过循环遍历列表来访问每个对象的属性。
八、使用装饰器创建对象
装饰器是一种特殊的函数,可以在不改变原函数的情况下扩展其功能。在Python中,可以使用装饰器来创建多个类对象。
class Tool:
def __init__(self, tool_name, tool_type):
self.tool_name = tool_name
self.tool_type = tool_type
def tool_decorator(func):
tools = []
def wrapper(*args, kwargs):
tool = func(*args, kwargs)
tools.append(tool)
return tool
wrapper.tools = tools
return wrapper
@tool_decorator
def create_tool(tool_name, tool_type):
return Tool(tool_name, tool_type)
使用装饰器创建多个Tool对象
create_tool('Tool1', 'Type1')
create_tool('Tool2', 'Type2')
create_tool('Tool3', 'Type3')
访问创建的对象
for tool in create_tool.tools:
print(f'Tool Name: {tool.tool_name}, Tool Type: {tool.tool_type}')
在上述代码中,我们定义了一个Tool
类和一个装饰器tool_decorator
。tool_decorator
函数接受一个函数作为参数,并返回一个新的函数wrapper
。wrapper
函数在调用原函数时,会将返回的Tool
对象添加到一个列表tools
中。然后,我们使用装饰器来装饰create_tool
函数,通过调用create_tool
函数创建了多个Tool
对象,并将这些对象存储在列表tools
中。最后,通过循环遍历列表来访问每个对象的属性。
九、使用模块创建对象
在Python中,可以通过将类定义在模块中,并在不同的地方导入该模块来创建多个类对象。
# module.py
class Gadget:
def __init__(self, gadget_name, gadget_type):
self.gadget_name = gadget_name
self.gadget_type = gadget_type
main.py
import module
使用模块创建多个Gadget对象
gadgets = [module.Gadget(f'Gadget_{i}', f'Type_{i}') for i in range(5)]
访问创建的对象
for gadget in gadgets:
print(f'Gadget Name: {gadget.gadget_name}, Gadget Type: {gadget.gadget_type}')
在上述代码中,我们定义了一个模块module
,其中包含一个Gadget
类。在主程序main.py
中,我们导入了module
模块,并通过列表解析创建了多个Gadget
对象。这些对象被存储在一个列表gadgets
中,最后通过循环遍历列表来访问每个对象的属性。
十、使用多线程创建对象
在Python中,可以使用多线程来创建多个类对象,以提高创建对象的效率。
import threading
class Item:
def __init__(self, item_name, item_category):
self.item_name = item_name
self.item_category = item_category
def create_item(item_name, item_category, items):
item = Item(item_name, item_category)
items.append(item)
使用多线程创建多个Item对象
items = []
threads = []
for i in range(5):
thread = threading.Thread(target=create_item, args=(f'Item_{i}', f'Category_{i}', items))
threads.append(thread)
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
访问创建的对象
for item in items:
print(f'Item Name: {item.item_name}, Item Category: {item.item_category}')
在上述代码中,我们定义了一个Item
类和一个函数create_item
。create_item
函数用于创建Item
对象并将其添加到列表items
中。然后,我们使用多线程来创建多个Item
对象,并将每个线程添加到列表threads
中。通过调用thread.start()
来启动线程,并通过thread.join()
来等待所有线程完成。最后,通过循环遍历列表items
来访问每个对象的属性。
十一、使用多进程创建对象
在Python中,可以使用多进程来创建多个类对象,以提高创建对象的效率。
import multiprocessing
class Component:
def __init__(self, component_name, component_type):
self.component_name = component_name
self.component_type = component_type
def create_component(component_name, component_type, components):
component = Component(component_name, component_type)
components.append(component)
使用多进程创建多个Component对象
components = multiprocessing.Manager().list()
processes = []
for i in range(5):
process = multiprocessing.Process(target=create_component, args=(f'Component_{i}', f'Type_{i}', components))
processes.append(process)
process.start()
等待所有进程完成
for process in processes:
process.join()
访问创建的对象
for component in components:
print(f'Component Name: {component.component_name}, Component Type: {component.component_type}')
在上述代码中,我们定义了一个Component
类和一个函数create_component
。create_component
函数用于创建Component
对象并将其添加到共享列表components
中。然后,我们使用多进程来创建多个Component
对象,并将每个进程添加到列表processes
中。通过调用process.start()
来启动进程,并通过process.join()
来等待所有进程完成。最后,通过循环遍历列表components
来访问每个对象的属性。
十二、使用协程创建对象
在Python中,可以使用协程来创建多个类对象,以提高创建对象的效率。
import asyncio
class Element:
def __init__(self, element_name, element_type):
self.element_name = element_name
self.element_type = element_type
async def create_element(element_name, element_type, elements):
element = Element(element_name, element_type)
elements.append(element)
使用协程创建多个Element对象
elements = []
async def main():
tasks = [create_element(f'Element_{i}', f'Type_{i}', elements) for i in range(5)]
await asyncio.gather(*tasks)
asyncio.run(main())
访问创建的对象
for element in elements:
print(f'Element Name: {element.element_name}, Element Type: {element.element_type}')
在上述代码中,我们定义了一个Element
类和一个协程函数create_element
。create_element
函数用于创建Element
对象并将其添加到列表elements
中。然后,我们使用协程来创建多个Element
对象,并通过asyncio.gather
来并发执行这些协程。最后,通过循环遍历列表elements
来访问每个对象的属性。
总结
在Python中,有多种方法可以创建多个类对象,包括使用循环、列表、字典、工厂模式、生成器、类方法、元类、闭包、装饰器、模块、多线程、多进程和协程。每种方法都有其优点和适用场景,可以根据实际需求选择合适的方法来创建和管理多个类对象。无论选择哪种方法,核心在于高效地创建和管理对象,并确保代码的可读性和维护性。
相关问答FAQs:
如何在Python中创建多个类对象?
在Python中,创建多个类对象非常简单。您只需定义一个类,然后使用该类创建多个实例。例如,您可以定义一个类Car
,并通过多次调用该类的构造函数来创建多个汽车对象。代码示例如下:
class Car:
def __init__(self, brand, model):
self.brand = brand
self.model = model
# 创建多个Car对象
car1 = Car("Toyota", "Corolla")
car2 = Car("Honda", "Civic")
car3 = Car("Ford", "Mustang")
通过以上代码,您成功创建了三个不同的汽车对象。
如何使用循环创建多个类对象?
使用循环创建类对象是一个非常有效的方法,特别是在需要生成大量对象时。您可以使用for
循环结合列表来实现这一点。例如:
class Book:
def __init__(self, title):
self.title = title
# 使用循环创建多个Book对象
book_titles = ["Python Basics", "Data Science", "Machine Learning"]
books = [Book(title) for title in book_titles]
在这个示例中,我们使用列表推导式来创建多个Book
对象,使得代码更加简洁。
创建类对象时可以传递哪些参数?
在创建类对象时,您可以根据类的定义传递不同的参数。类的构造函数__init__
可以接收多个参数,以便为每个对象初始化不同的属性。例如,如果您的类需要颜色和型号作为参数,可以这样定义:
class Bike:
def __init__(self, color, model):
self.color = color
self.model = model
# 创建Bike对象并传递不同参数
bike1 = Bike("Red", "Mountain")
bike2 = Bike("Blue", "Road")
通过这种方式,您可以为每个对象设置独特的属性,以便更好地满足您的需求。
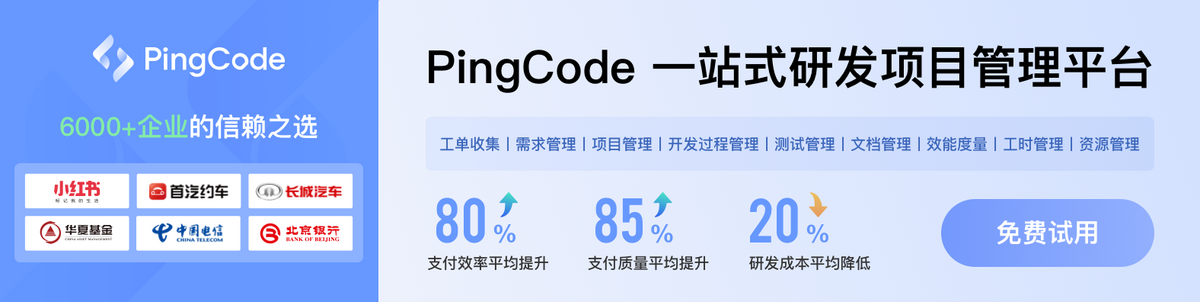