Python翻译的算法可以通过多种方式实现,如基于规则的翻译、统计机器翻译、神经机器翻译等。这几种方法各有优缺点,其中,神经机器翻译(Neural Machine Translation, NMT) 是目前应用最广泛、效果最好的方法之一。NMT通过深度学习技术,使用神经网络模型来进行翻译任务。
以神经机器翻译为例,NMT通常包括以下几个步骤:数据预处理、模型训练、模型评估和翻译。首先,数据预处理是指将平行语料库进行清洗、分词、向量化等操作,为模型输入做准备。接着,模型训练是利用预处理好的数据训练神经网络模型,使其学习源语言到目标语言的映射关系。模型评估是对训练好的模型进行性能测试,确保其能够准确地进行翻译。最后,翻译步骤是利用训练好的模型进行实际的翻译任务。下面将详细讲解这些步骤及其实现方法。
一、数据预处理
数据预处理是神经机器翻译的基础步骤,其质量直接影响到模型的性能和翻译效果。数据预处理主要包括数据清洗、分词和向量化等步骤。
1. 数据清洗
数据清洗是指对原始数据进行处理,去除噪声数据和无关信息。常见的数据清洗操作包括去除重复句子、过滤掉过长或过短的句子、去除标点符号和特殊字符等。
import re
def clean_text(text):
text = text.lower() # 转为小写
text = re.sub(r"[^a-zA-Z0-9\s]", "", text) # 去除非字母数字字符
text = re.sub(r"\s+", " ", text).strip() # 去除多余的空格
return text
示例
sample_text = "Hello, world! This is a sample text."
cleaned_text = clean_text(sample_text)
print(cleaned_text)
2. 分词
分词是将句子划分为单独的词语或子词。对于不同的语言,分词方法有所不同。常见的分词工具包括NLTK、SpaCy、jieba等。
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
sample_text = "Hello, world! This is a sample text."
tokenized_text = word_tokenize(sample_text)
print(tokenized_text)
3. 向量化
向量化是将文本数据转化为模型可以处理的数值形式。常用的方法有词袋模型(Bag of Words)、词嵌入(Word Embedding)等。词嵌入将词语映射到高维向量空间中,常用的词嵌入方法包括Word2Vec、GloVe、FastText等。
from gensim.models import Word2Vec
示例语料
sentences = [["hello", "world"], ["this", "is", "a", "sample", "text"]]
训练Word2Vec模型
model = Word2Vec(sentences, vector_size=100, window=5, min_count=1, workers=4)
获取词向量
vector = model.wv['hello']
print(vector)
二、模型训练
模型训练是神经机器翻译的核心步骤。在NMT中,常用的模型结构是编码器-解码器(Encoder-Decoder)架构,通常还会结合注意力机制(Attention Mechanism)来提高翻译效果。
1. 编码器-解码器架构
编码器-解码器架构由两个部分组成:编码器将输入序列编码为一个固定长度的上下文向量,解码器根据上下文向量生成目标序列。
import tensorflow as tf
from tensorflow.keras.layers import Input, LSTM, Dense
from tensorflow.keras.models import Model
编码器
encoder_inputs = Input(shape=(None, input_dim))
encoder = LSTM(latent_dim, return_state=True)
encoder_outputs, state_h, state_c = encoder(encoder_inputs)
encoder_states = [state_h, state_c]
解码器
decoder_inputs = Input(shape=(None, output_dim))
decoder_lstm = LSTM(latent_dim, return_sequences=True, return_state=True)
decoder_outputs, _, _ = decoder_lstm(decoder_inputs, initial_state=encoder_states)
decoder_dense = Dense(output_dim, activation='softmax')
decoder_outputs = decoder_dense(decoder_outputs)
模型
model = Model([encoder_inputs, decoder_inputs], decoder_outputs)
model.compile(optimizer='rmsprop', loss='categorical_crossentropy')
model.summary()
2. 注意力机制
注意力机制能够动态地为解码器生成每个词时分配不同的权重,使模型能够更好地捕捉长距离依赖关系。常见的注意力机制有Bahdanau Attention和Luong Attention。
from tensorflow.keras.layers import Attention
注意力机制
attention = Attention()
编码器输出和解码器输入
encoder_outputs = ... # 编码器输出
decoder_inputs = ... # 解码器输入
计算注意力权重
attention_weights = attention([decoder_inputs, encoder_outputs])
将注意力权重应用到编码器输出
context_vector = attention_weights * encoder_outputs
三、模型评估
模型评估是对训练好的模型进行性能测试,确保其能够准确地进行翻译。常见的评估指标有BLEU(Bilingual Evaluation Understudy)、ROUGE(Recall-Oriented Understudy for Gisting Evaluation)等。
1. BLEU
BLEU是最常用的机器翻译评估指标之一,它通过计算候选翻译与参考翻译之间的n-gram匹配程度来评估翻译质量。
from nltk.translate.bleu_score import sentence_bleu
reference = [['this', 'is', 'a', 'test']]
candidate = ['this', 'is', 'a', 'test']
score = sentence_bleu(reference, candidate)
print('BLEU score:', score)
2. ROUGE
ROUGE主要用于评估自动摘要的质量,但也可以用于机器翻译评估。它通过计算候选翻译与参考翻译之间的重叠部分来评估翻译质量。
from rouge import Rouge
rouge = Rouge()
scores = rouge.get_scores('this is a test', 'this is a test')
print('ROUGE scores:', scores)
四、翻译
翻译是使用训练好的模型进行实际的翻译任务。通常,翻译步骤包括输入预处理、模型预测和输出后处理。
1. 输入预处理
输入预处理是对待翻译的句子进行与训练时相同的预处理操作,如清洗、分词和向量化。
input_text = "Hello, world!"
cleaned_text = clean_text(input_text)
tokenized_text = word_tokenize(cleaned_text)
input_vector = ... # 将分词后的句子转化为向量
2. 模型预测
使用训练好的模型进行翻译预测,得到目标语言的词序列。
# 加载训练好的模型
model = ... # 训练好的模型
进行预测
predicted_sequence = model.predict(input_vector)
3. 输出后处理
输出后处理是将模型预测的词序列转化为可读的文本格式,如去除特殊字符、拼接词语等。
predicted_text = ... # 将预测的词序列转化为文本
print('Translated text:', predicted_text)
五、优化和改进
为了进一步提高翻译质量,可以对模型和数据进行优化和改进。
1. 数据增强
数据增强是通过对现有数据进行变换或生成新数据来增加训练数据的多样性,从而提高模型的泛化能力。常见的数据增强方法包括同义词替换、随机插入、随机交换等。
from random import randint, shuffle
def synonym_replacement(sentence, n):
words = sentence.split()
for _ in range(n):
word = words[randint(0, len(words)-1)]
synonym = get_synonym(word) # 获取同义词
words[words.index(word)] = synonym
return ' '.join(words)
示例
sentence = "This is a sample sentence."
augmented_sentence = synonym_replacement(sentence, 2)
print('Augmented sentence:', augmented_sentence)
2. 模型优化
模型优化是通过调整模型结构和训练参数来提高模型性能。常见的优化方法包括使用更深的网络结构、调整学习率、增加正则化等。
from tensorflow.keras.optimizers import Adam
调整学习率
optimizer = Adam(learning_rate=0.001)
model.compile(optimizer=optimizer, loss='categorical_crossentropy')
增加正则化
from tensorflow.keras.layers import Dropout
在LSTM层后添加Dropout层
decoder_lstm = LSTM(latent_dim, return_sequences=True, return_state=True, dropout=0.5)
六、应用实例
为了更好地理解Python翻译算法的实现,下面通过一个具体的应用实例来展示整个流程。
1. 数据准备
首先,准备一个平行语料库,包含源语言和目标语言的句子对。
# 示例平行语料库
source_sentences = ["Hello, world!", "This is a sample text."]
target_sentences = ["Hola, mundo!", "Este es un texto de muestra."]
2. 数据预处理
对平行语料库进行数据预处理,包括清洗、分词和向量化。
# 清洗
cleaned_source_sentences = [clean_text(sentence) for sentence in source_sentences]
cleaned_target_sentences = [clean_text(sentence) for sentence in target_sentences]
分词
tokenized_source_sentences = [word_tokenize(sentence) for sentence in cleaned_source_sentences]
tokenized_target_sentences = [word_tokenize(sentence) for sentence in cleaned_target_sentences]
向量化
source_vector = ... # 将分词后的源语言句子转化为向量
target_vector = ... # 将分词后的目标语言句子转化为向量
3. 模型训练
使用预处理好的数据训练神经机器翻译模型。
# 编码器-解码器架构
encoder_inputs = Input(shape=(None, input_dim))
encoder = LSTM(latent_dim, return_state=True)
encoder_outputs, state_h, state_c = encoder(encoder_inputs)
encoder_states = [state_h, state_c]
decoder_inputs = Input(shape=(None, output_dim))
decoder_lstm = LSTM(latent_dim, return_sequences=True, return_state=True)
decoder_outputs, _, _ = decoder_lstm(decoder_inputs, initial_state=encoder_states)
decoder_dense = Dense(output_dim, activation='softmax')
decoder_outputs = decoder_dense(decoder_outputs)
model = Model([encoder_inputs, decoder_inputs], decoder_outputs)
model.compile(optimizer='rmsprop', loss='categorical_crossentropy')
训练模型
model.fit([source_vector, target_vector], target_vector, epochs=10, batch_size=64)
4. 模型评估
对训练好的模型进行性能评估,确保其翻译效果。
# 示例评估
reference = [['hola', 'mundo']]
candidate = model.predict(...) # 使用模型预测
score = sentence_bleu(reference, candidate)
print('BLEU score:', score)
5. 翻译
使用训练好的模型进行实际的翻译任务。
# 输入预处理
input_text = "Hello, world!"
cleaned_text = clean_text(input_text)
tokenized_text = word_tokenize(cleaned_text)
input_vector = ... # 将分词后的句子转化为向量
模型预测
predicted_sequence = model.predict(input_vector)
输出后处理
predicted_text = ... # 将预测的词序列转化为文本
print('Translated text:', predicted_text)
通过以上步骤,我们可以实现一个简单的Python翻译算法。实际应用中,可以根据具体需求对模型和数据进行进一步优化和调整,以提高翻译效果。
相关问答FAQs:
如何选择合适的Python翻译库?
在实现翻译算法时,有多个Python库可供选择,如Google Translate API、DeepL API和translate库。选择合适的库应考虑翻译质量、支持的语言、使用的方便程度以及费用。Google Translate API提供了广泛的语言支持和高质量翻译,而DeepL API在某些语言对的翻译上表现优异。根据项目需求进行比较,选择最符合要求的库。
实现Python翻译算法需要哪些基本步骤?
实现翻译算法通常需要几个关键步骤:首先,安装所需的翻译库。接下来,设置API密钥(如果使用的是需要授权的服务)。然后,编写代码来调用翻译函数,并传入待翻译的文本和目标语言。最后,处理返回的翻译结果,并根据需要格式化输出。这些步骤为实现翻译功能提供了清晰的框架。
如何提高Python翻译算法的准确性和效率?
要提高翻译的准确性,可以考虑使用上下文分析和预处理技术,如文本清洗和句子分割。此外,结合机器学习模型进行自定义训练,针对特定领域的语言进行优化,也能显著提升翻译质量。为了提高效率,可以使用批量翻译功能,将多个文本一起处理,减少API调用次数,从而加快响应速度。
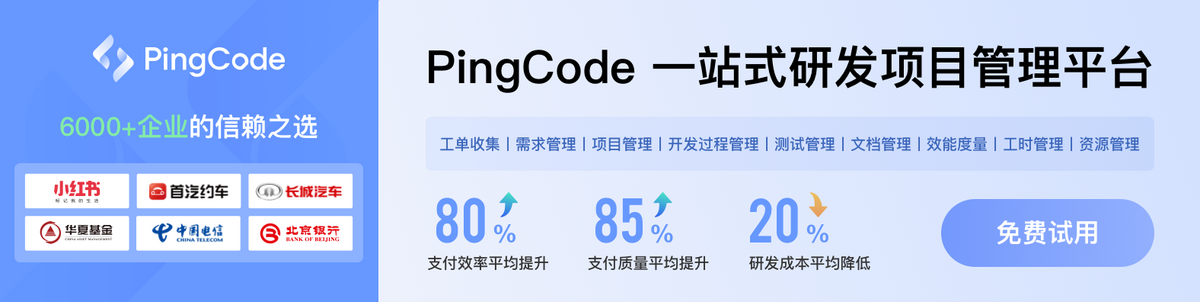