开头段落:
使用字符串方法、使用正则表达式、使用内置搜索模块。其中,使用正则表达式 是一种非常强大的方法,它不仅支持简单的关键字搜索,还可以处理复杂的模式匹配。例如,你可以使用 re
模块来搜索关键字,并利用正则表达式的强大功能指定各种搜索条件,如区分大小写、匹配单词边界等。下面将详细介绍如何使用这些方法来进行关键字搜索。
一、使用字符串方法
Python 的字符串方法是进行关键字搜索的最基本方式。这些方法简单易用,适用于大多数场景。
1. 使用 find()
方法
find()
方法返回子字符串在字符串中的最低索引位置。如果未找到子字符串,则返回 -1。
text = "Python is an amazing programming language."
keyword = "amazing"
position = text.find(keyword)
if position != -1:
print(f"Keyword '{keyword}' found at position {position}.")
else:
print(f"Keyword '{keyword}' not found.")
2. 使用 in
操作符
in
操作符用于判断子字符串是否存在于字符串中,返回布尔值。
text = "Python is an amazing programming language."
keyword = "amazing"
if keyword in text:
print(f"Keyword '{keyword}' found.")
else:
print(f"Keyword '{keyword}' not found.")
3. 使用 index()
方法
index()
方法与 find()
类似,但如果未找到子字符串,则会引发 ValueError
异常。
text = "Python is an amazing programming language."
keyword = "amazing"
try:
position = text.index(keyword)
print(f"Keyword '{keyword}' found at position {position}.")
except ValueError:
print(f"Keyword '{keyword}' not found.")
二、使用正则表达式
Python 的 re
模块提供了强大的正则表达式功能,可以进行复杂的模式匹配。
1. 基本用法
首先,导入 re
模块,然后使用 re.search()
方法进行关键字搜索。
import re
text = "Python is an amazing programming language."
keyword = "amazing"
match = re.search(keyword, text)
if match:
print(f"Keyword '{keyword}' found at position {match.start()}.")
else:
print(f"Keyword '{keyword}' not found.")
2. 忽略大小写搜索
使用 re.IGNORECASE
标志来忽略大小写。
import re
text = "Python is an AMAZING programming language."
keyword = "amazing"
match = re.search(keyword, text, re.IGNORECASE)
if match:
print(f"Keyword '{keyword}' found at position {match.start()}.")
else:
print(f"Keyword '{keyword}' not found.")
3. 使用 re.findall()
re.findall()
方法返回所有与模式匹配的子字符串列表。
import re
text = "Python is amazing. Programming is amazing."
keyword = "amazing"
matches = re.findall(keyword, text)
print(f"Found {len(matches)} occurrences of keyword '{keyword}'.")
4. 使用 re.finditer()
re.finditer()
返回一个迭代器,生成所有匹配对象。
import re
text = "Python is amazing. Programming is amazing."
keyword = "amazing"
matches = re.finditer(keyword, text)
for match in matches:
print(f"Keyword '{keyword}' found at position {match.start()}.")
三、使用内置搜索模块
Python 还提供了一些内置模块,可以用于更高级的搜索功能。
1. glob
模块
glob
模块用于文件系统中的模式匹配,可以搜索文件名中包含特定关键字的文件。
import glob
files = glob.glob("*.txt")
keyword = "example"
for file in files:
if keyword in file:
print(f"Keyword '{keyword}' found in file name '{file}'.")
2. fnmatch
模块
fnmatch
模块用于比较文件名与指定模式。
import fnmatch
import os
files = os.listdir(".")
keyword = "example"
for file in files:
if fnmatch.fnmatch(file, f"*{keyword}*"):
print(f"Keyword '{keyword}' found in file name '{file}'.")
3. whoosh
模块
whoosh
是一个用于全文搜索和索引的第三方库。
from whoosh.index import create_in
from whoosh.fields import Schema, TEXT
from whoosh.qparser import QueryParser
创建索引
schema = Schema(title=TEXT(stored=True), content=TEXT)
index_dir = "indexdir"
os.makedirs(index_dir, exist_ok=True)
ix = create_in(index_dir, schema)
writer = ix.writer()
writer.add_document(title="First document", content="Python is an amazing programming language.")
writer.add_document(title="Second document", content="Programming is fun.")
writer.commit()
搜索关键字
searcher = ix.searcher()
query = QueryParser("content", ix.schema).parse("amazing")
results = searcher.search(query)
for result in results:
print(result["title"])
四、结合使用多种方法
在实际应用中,你可能需要结合多种方法进行关键字搜索。以下是一些示例。
1. 搜索文件内容中的关键字
结合使用 os
和 re
模块,搜索文件内容中的关键字。
import os
import re
directory = "path/to/directory"
keyword = "amazing"
for root, dirs, files in os.walk(directory):
for file in files:
if file.endswith(".txt"):
with open(os.path.join(root, file), "r") as f:
content = f.read()
if re.search(keyword, content):
print(f"Keyword '{keyword}' found in file '{file}'.")
2. 从网页内容中搜索关键字
结合使用 requests
和 re
模块,从网页内容中搜索关键字。
import requests
import re
url = "https://www.example.com"
keyword = "amazing"
response = requests.get(url)
content = response.text
if re.search(keyword, content):
print(f"Keyword '{keyword}' found in webpage content.")
3. 使用多线程进行关键字搜索
使用多线程提高搜索效率,特别是在处理大量文件或网页时。
import os
import re
import threading
directory = "path/to/directory"
keyword = "amazing"
def search_file(file):
with open(file, "r") as f:
content = f.read()
if re.search(keyword, content):
print(f"Keyword '{keyword}' found in file '{file}'.")
threads = []
for root, dirs, files in os.walk(directory):
for file in files:
if file.endswith(".txt"):
file_path = os.path.join(root, file)
thread = threading.Thread(target=search_file, args=(file_path,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
4. 搜索日志文件中的关键字
结合使用 logging
和 re
模块,搜索日志文件中的关键字。
import logging
import re
配置日志
logging.basicConfig(filename="example.log", level=logging.DEBUG)
logging.debug("This is a debug message.")
logging.info("This is an info message.")
logging.warning("This is a warning message.")
logging.error("This is an error message.")
logging.critical("This is a critical message.")
搜索关键字
keyword = "error"
with open("example.log", "r") as f:
content = f.read()
matches = re.findall(keyword, content, re.IGNORECASE)
print(f"Found {len(matches)} occurrences of keyword '{keyword}' in log file.")
5. 从数据库中搜索关键字
结合使用 sqlite3
和 re
模块,从数据库中搜索关键字。
import sqlite3
import re
创建数据库
conn = sqlite3.connect(":memory:")
cursor = conn.cursor()
cursor.execute("CREATE TABLE documents (id INTEGER PRIMARY KEY, content TEXT)")
cursor.execute("INSERT INTO documents (content) VALUES ('Python is an amazing programming language.')")
cursor.execute("INSERT INTO documents (content) VALUES ('Programming is fun.')")
conn.commit()
搜索关键字
keyword = "amazing"
cursor.execute("SELECT content FROM documents")
rows = cursor.fetchall()
for row in rows:
content = row[0]
if re.search(keyword, content):
print(f"Keyword '{keyword}' found in content: {content}")
conn.close()
6. 搜索邮件内容中的关键字
结合使用 imaplib
和 re
模块,从邮件内容中搜索关键字。
import imaplib
import email
import re
连接到邮箱
mail = imaplib.IMAP4_SSL("imap.gmail.com")
mail.login("your-email@gmail.com", "your-password")
mail.select("inbox")
搜索邮件
status, messages = mail.search(None, "ALL")
keyword = "amazing"
for num in messages[0].split():
status, data = mail.fetch(num, "(RFC822)")
raw_email = data[0][1].decode("utf-8")
msg = email.message_from_string(raw_email)
content = msg.get_payload(decode=True).decode("utf-8")
if re.search(keyword, content):
print(f"Keyword '{keyword}' found in email with subject: {msg['subject']}")
mail.logout()
结论
通过上述方法,你可以在不同的场景中灵活应用关键字搜索技术。使用字符串方法 是最基础的,适用于简单的搜索任务;使用正则表达式 则提供了更强大的模式匹配能力,适用于复杂的搜索需求;使用内置搜索模块 则可以处理更高级的搜索场景,如文件系统、数据库、网页内容等。结合多种方法,可以进一步提高搜索效率和精度。希望这些内容对你有所帮助!
相关问答FAQs:
如何在Python中实现关键字搜索的基本方法?
在Python中,可以使用内置的字符串方法如str.find()
和str.index()
来搜索关键字。这些方法可以在字符串中查找子字符串的位置。如果需要更复杂的搜索功能,可以使用正则表达式模块re
,通过re.search()
、re.match()
和re.findall()
等函数进行关键字搜索,支持模式匹配和更灵活的搜索条件。
是否可以在文本文件中搜索关键字?
当然可以。可以使用Python的文件操作功能来打开并读取文本文件,然后逐行检查每一行是否包含所需的关键字。使用with open('filename.txt', 'r') as file:
语句可以方便地打开文件并确保在操作完成后自动关闭文件。结合字符串方法或正则表达式,可以高效地实现关键字搜索。
如何提高关键字搜索的效率?
为了提高搜索效率,可以考虑使用数据结构如字典或集合来存储待搜索的文本内容,这样在进行关键字查询时,可以利用哈希表的查找效率。此外,使用第三方库如Whoosh
或elasticsearch-py
等,可以实现更高效、更强大的全文搜索功能,适合处理大规模文本数据。
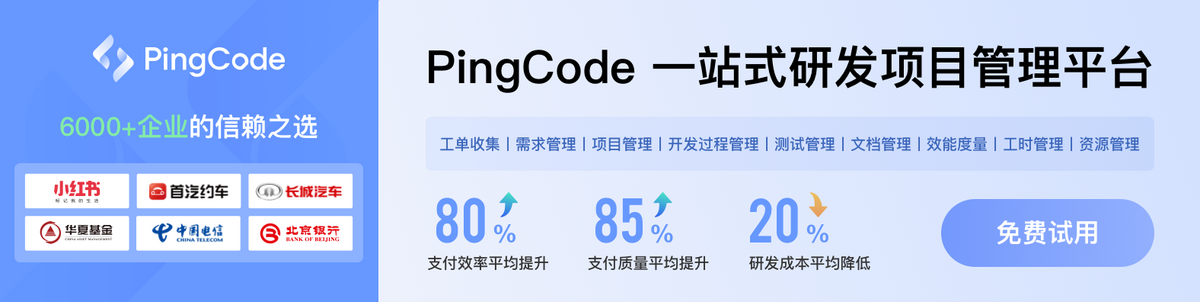