要在Python中制作象棋游戏,你需要掌握的关键技术包括:图形界面编程、游戏逻辑编写、棋盘绘制、棋子移动规则、对局规则实现、AI算法应用。其中,图形界面编程是一个重要的部分,它直接关系到用户的体验。我们可以使用Python的Pygame库来实现这一部分。
一、安装必要的库
在开始之前,确保你已经安装了Python和Pygame库。你可以通过以下命令安装Pygame:
pip install pygame
二、创建棋盘和棋子
-
绘制棋盘
在Pygame中创建一个窗口,并在其中绘制棋盘。棋盘是一个8×8的网格,每个格子的大小可以根据窗口的大小来确定。
import pygame
import sys
初始化Pygame
pygame.init()
设置窗口大小
window_size = 800
screen = pygame.display.set_mode((window_size, window_size))
pygame.display.set_caption('象棋')
定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
绘制棋盘
def draw_chessboard():
block_size = window_size // 8
colors = [pygame.Color("white"), pygame.Color("gray")]
for y in range(8):
for x in range(8):
color = colors[(x + y) % 2]
pygame.draw.rect(screen, color, pygame.Rect(x * block_size, y * block_size, block_size, block_size))
主循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
draw_chessboard()
pygame.display.flip()
-
加载棋子图像
可以使用象棋棋子的图像文件来表示棋子。将这些图像加载到程序中,并在棋盘上绘制它们。
def load_images():
pieces = ['r', 'n', 'b', 'q', 'k', 'p', 'R', 'N', 'B', 'Q', 'K', 'P']
images = {}
for piece in pieces:
images[piece] = pygame.image.load(f'images/{piece}.png')
return images
images = load_images()
然后,在棋盘上绘制棋子:
def draw_pieces(board):
block_size = window_size // 8
for y in range(8):
for x in range(8):
piece = board[y][x]
if piece != '.':
screen.blit(images[piece], pygame.Rect(x * block_size, y * block_size, block_size, block_size))
示例棋盘
board = [
['r', 'n', 'b', 'q', 'k', 'b', 'n', 'r'],
['p', 'p', 'p', 'p', 'p', 'p', 'p', 'p'],
['.', '.', '.', '.', '.', '.', '.', '.'],
['.', '.', '.', '.', '.', '.', '.', '.'],
['.', '.', '.', '.', '.', '.', '.', '.'],
['.', '.', '.', '.', '.', '.', '.', '.'],
['P', 'P', 'P', 'P', 'P', 'P', 'P', 'P'],
['R', 'N', 'B', 'Q', 'K', 'B', 'N', 'R']
]
在主循环中调用draw_pieces
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
draw_chessboard()
draw_pieces(board)
pygame.display.flip()
三、实现棋子移动逻辑
-
定义棋子移动规则
对于象棋,每个棋子的移动规则都不同。需要为每个棋子定义其合法的移动方式。可以使用一个类来表示棋子,并在其中定义其移动规则。
class Piece:
def __init__(self, color):
self.color = color
def get_moves(self, board, x, y):
raise NotImplementedError
class Pawn(Piece):
def get_moves(self, board, x, y):
moves = []
direction = -1 if self.color == 'white' else 1
if board[y + direction][x] == '.':
moves.append((x, y + direction))
# TODO: Add more rules for capturing and initial two-step move
return moves
类似的,你可以为其他棋子定义它们的移动规则
-
更新棋盘状态
当玩家选择一个棋子并移动它时,需要更新棋盘的状态。可以通过以下步骤实现:
- 检测玩家点击事件,确定选中的棋子。
- 根据选中的棋子和移动规则,生成合法的移动列表。
- 玩家选择一个合法的移动位置,更新棋盘状态。
selected_piece = None
selected_moves = []
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
x, y = event.pos
x, y = x // block_size, y // block_size
if selected_piece:
if (x, y) in selected_moves:
# 移动棋子
board[selected_piece[1]][selected_piece[0]] = '.'
board[y][x] = selected_piece[2]
selected_piece = None
selected_moves = []
else:
selected_piece = None
selected_moves = []
else:
piece = board[y][x]
if piece != '.':
selected_piece = (x, y, piece)
selected_moves = get_moves(piece, x, y)
draw_chessboard()
draw_pieces(board)
pygame.display.flip()
四、实现AI对手
-
基本AI算法
可以使用简单的随机算法来实现一个初步的AI对手。AI可以随机选择一个棋子,并随机选择一个合法的移动位置。
import random
def ai_move(board):
pieces = [(x, y, board[y][x]) for y in range(8) for x in range(8) if board[y][x] != '.']
piece = random.choice(pieces)
moves = get_moves(piece[2], piece[0], piece[1])
if moves:
move = random.choice(moves)
board[piece[1]][piece[0]] = '.'
board[move[1]][move[0]] = piece[2]
-
提升AI智能
要提升AI的智能,可以使用更加复杂的算法,如MiniMax算法。MiniMax算法通过递归地评估棋局的可能状态,选择对AI最有利的移动。你可以结合α-β剪枝优化算法来提高效率。
def minimax(board, depth, alpha, beta, maximizing_player):
if depth == 0 or game_over(board):
return evaluate_board(board)
if maximizing_player:
max_eval = float('-inf')
for move in get_all_moves(board, 'ai'):
make_move(board, move)
eval = minimax(board, depth - 1, alpha, beta, False)
undo_move(board, move)
max_eval = max(max_eval, eval)
alpha = max(alpha, eval)
if beta <= alpha:
break
return max_eval
else:
min_eval = float('inf')
for move in get_all_moves(board, 'player'):
make_move(board, move)
eval = minimax(board, depth - 1, alpha, beta, True)
undo_move(board, move)
min_eval = min(min_eval, eval)
beta = min(beta, eval)
if beta <= alpha:
break
return min_eval
五、完成游戏
-
游戏循环
将所有部分结合起来,形成完整的游戏循环。包括玩家移动和AI移动的交替。
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
if player_turn:
x, y = event.pos
x, y = x // block_size, y // block_size
if selected_piece:
if (x, y) in selected_moves:
board[selected_piece[1]][selected_piece[0]] = '.'
board[y][x] = selected_piece[2]
player_turn = False
selected_piece = None
selected_moves = []
else:
selected_piece = None
selected_moves = []
else:
piece = board[y][x]
if piece != '.':
selected_piece = (x, y, piece)
selected_moves = get_moves(piece, x, y)
if not player_turn:
ai_move(board)
player_turn = True
draw_chessboard()
draw_pieces(board)
pygame.display.flip()
-
结束游戏条件
添加游戏结束的条件检测,例如将军和将死的检测。当一个玩家将死时,显示游戏结束的消息,并提供重新开始或退出的选项。
def game_over(board):
# 简单的将死检测
return 'k' not in [piece for row in board for piece in row] or 'K' not in [piece for row in board for piece in row]
while True:
if game_over(board):
print("Game Over")
break
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
if player_turn:
x, y = event.pos
x, y = x // block_size, y // block_size
if selected_piece:
if (x, y) in selected_moves:
board[selected_piece[1]][selected_piece[0]] = '.'
board[y][x] = selected_piece[2]
player_turn = False
selected_piece = None
selected_moves = []
else:
selected_piece = None
selected_moves = []
else:
piece = board[y][x]
if piece != '.':
selected_piece = (x, y, piece)
selected_moves = get_moves(piece, x, y)
if not player_turn:
ai_move(board)
player_turn = True
draw_chessboard()
draw_pieces(board)
pygame.display.flip()
通过以上步骤,你可以在Python中制作一个简单的象棋游戏,并逐步完善它的功能和AI算法,提升游戏体验和挑战性。
相关问答FAQs:
如何开始使用Python编写象棋程序?
要开始使用Python编写象棋程序,您首先需要了解象棋的基本规则和棋盘布局。建议您学习Python的基本语法,并熟悉一些常用的图形库,如Pygame或Tkinter,以便能够创建图形界面。可以从简单的文本界面开始,逐步添加图形和其他功能。
有哪些Python库可以帮助我开发象棋游戏?
在Python中,有多个库可以帮助您开发象棋游戏。Pygame是一个流行的库,适合创建2D游戏,并提供了处理图形、音效和用户输入的工具。另一个选择是python-chess库,它专注于象棋逻辑的实现,能够处理棋局状态、合法移动和游戏规则,适合想要快速实现象棋逻辑的开发者。
如何实现象棋的AI对战功能?
实现象棋的AI对战功能可以通过构建一个评分系统来评估棋局状态。可以使用基本的启发式算法,如极大极小算法(Minimax),结合α-β剪枝来提高效率。随着对AI技术的深入了解,您还可以尝试使用更复杂的机器学习算法来优化决策过程。
如何处理象棋中的特殊规则,如将军和升变?
在编写象棋程序时,处理特殊规则是非常重要的。您需要在代码中实现检测将军、将死和升变的逻辑。可以为每种情况编写独立的函数来检查当前棋局状态,并根据这些状态更新游戏规则,以确保游戏的合法性和趣味性。
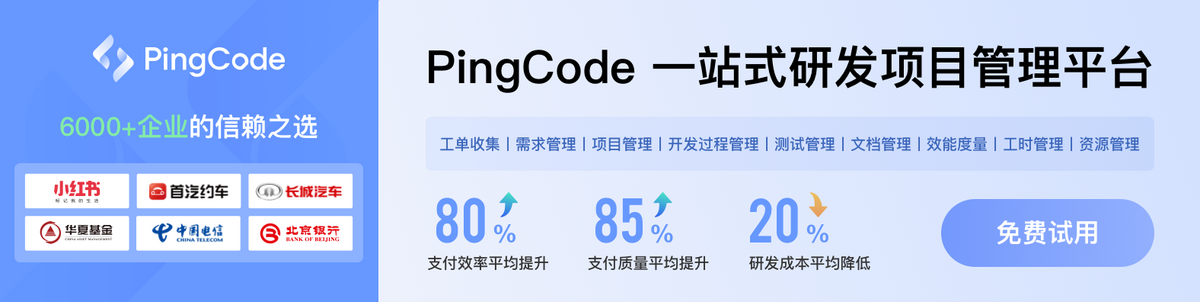