通过多种方法,Python 可以改变字符串:使用字符串方法、切片、拼接、正则表达式等。 其中,字符串方法 是最常用且便捷的一种方式。例如,可以使用 replace()
方法来替换字符串中的某些子字符串,或者使用 split()
和 join()
方法来拆分和重新组合字符串。
详细描述:字符串方法 提供了多种操作字符串的便捷方式。例如,使用 replace()
方法可以替换字符串中的特定子字符串。假设有一个字符串 s = "hello world"
,如果想把 "world" 替换成 "Python",可以使用 s.replace("world", "Python")
,结果将会是 "hello Python"
。这种方法非常适合用于简单的替换操作。
一、字符串方法
Python 提供了一系列内置的字符串方法,可以用于改变字符串的内容或格式。以下是一些常用的字符串方法及其用法:
1. replace()
方法
replace()
方法用于将字符串中的某些子字符串替换成新的子字符串。例如:
s = "hello world"
new_s = s.replace("world", "Python")
print(new_s) # 输出: hello Python
2. split()
和 join()
方法
split()
方法用于将字符串拆分成列表,而 join()
方法则可以将列表中的元素组合成字符串。例如:
s = "hello world"
words = s.split() # 拆分成列表 ['hello', 'world']
new_s = "-".join(words) # 组合成字符串 "hello-world"
print(new_s) # 输出: hello-world
3. upper()
和 lower()
方法
upper()
方法用于将字符串中的所有字母转换为大写,而 lower()
方法用于将字符串中的所有字母转换为小写。例如:
s = "Hello World"
print(s.upper()) # 输出: HELLO WORLD
print(s.lower()) # 输出: hello world
4. strip()
方法
strip()
方法用于去除字符串两端的空白字符。例如:
s = " hello world "
print(s.strip()) # 输出: hello world
二、字符串切片
字符串切片是另一种改变字符串内容的方法。通过使用索引,可以从字符串中提取子字符串,甚至可以重组字符串。例如:
s = "hello world"
提取子字符串 "hello"
sub_s = s[:5]
print(sub_s) # 输出: hello
提取子字符串 "world"
sub_s = s[6:]
print(sub_s) # 输出: world
切片还可以与拼接结合使用来重组字符串。例如:
s = "hello world"
替换 "world" 为 "Python"
new_s = s[:6] + "Python"
print(new_s) # 输出: hello Python
三、字符串拼接
字符串拼接是将多个字符串合并成一个字符串的过程。Python 提供了多种拼接字符串的方法:
1. 使用 +
操作符
最简单的拼接方法是使用 +
操作符:
s1 = "hello"
s2 = "world"
new_s = s1 + " " + s2
print(new_s) # 输出: hello world
2. 使用 join()
方法
join()
方法可以用于高效地拼接多个字符串,尤其是在拼接大量字符串时:
strings = ["hello", "world"]
new_s = " ".join(strings)
print(new_s) # 输出: hello world
四、正则表达式
正则表达式(Regular Expression,简称 regex)是一种强大的字符串匹配和替换工具。Python 的 re
模块提供了对正则表达式的支持,可以用于复杂的字符串操作。
1. 匹配字符串
可以使用 re.match()
方法来匹配字符串的开头:
import re
s = "hello world"
match = re.match(r"hello", s)
if match:
print("匹配成功")
else:
print("匹配失败")
2. 查找子字符串
可以使用 re.search()
方法在字符串中查找子字符串:
import re
s = "hello world"
search = re.search(r"world", s)
if search:
print("查找到子字符串")
else:
print("未找到子字符串")
3. 替换子字符串
可以使用 re.sub()
方法来替换字符串中的子字符串:
import re
s = "hello world"
new_s = re.sub(r"world", "Python", s)
print(new_s) # 输出: hello Python
五、格式化字符串
格式化字符串是指将变量的值嵌入到字符串中的过程。Python 提供了多种格式化字符串的方法:
1. 使用 %
操作符
这是最古老的格式化字符串的方法:
name = "Alice"
age = 25
s = "My name is %s and I am %d years old." % (name, age)
print(s) # 输出: My name is Alice and I am 25 years old.
2. 使用 format()
方法
format()
方法提供了更灵活的字符串格式化方式:
name = "Alice"
age = 25
s = "My name is {} and I am {} years old.".format(name, age)
print(s) # 输出: My name is Alice and I am 25 years old.
3. 使用 f-string(格式化字符串字面量)
f-string 是 Python 3.6 引入的一种格式化字符串的方式,使用起来更加简洁:
name = "Alice"
age = 25
s = f"My name is {name} and I am {age} years old."
print(s) # 输出: My name is Alice and I am 25 years old.
六、字符串编码和解码
在处理不同编码的字符串时,可能需要对字符串进行编码和解码操作。Python 提供了 encode()
和 decode()
方法来实现这些操作。
1. 编码字符串
可以使用 encode()
方法将字符串编码为字节对象:
s = "hello world"
encoded_s = s.encode("utf-8")
print(encoded_s) # 输出: b'hello world'
2. 解码字符串
可以使用 decode()
方法将字节对象解码为字符串:
encoded_s = b'hello world'
decoded_s = encoded_s.decode("utf-8")
print(decoded_s) # 输出: hello world
七、字符串模板
Python 的 string
模块提供了 Template
类,可以用于创建模板字符串。模板字符串允许在字符串中插入占位符,并在运行时替换占位符。
1. 创建模板字符串
可以使用 Template
类创建模板字符串,并使用 substitute()
方法替换占位符:
from string import Template
template = Template("My name is $name and I am $age years old.")
s = template.substitute(name="Alice", age=25)
print(s) # 输出: My name is Alice and I am 25 years old.
2. 使用字典替换占位符
还可以使用字典来替换模板字符串中的占位符:
from string import Template
template = Template("My name is $name and I am $age years old.")
data = {"name": "Alice", "age": 25}
s = template.substitute(data)
print(s) # 输出: My name is Alice and I am 25 years old.
八、字符串的常见操作
在处理字符串时,可能需要进行一些常见的操作,如查找、比较、统计等。以下是一些常见的字符串操作:
1. 查找子字符串
可以使用 find()
方法查找子字符串在字符串中的位置:
s = "hello world"
index = s.find("world")
print(index) # 输出: 6
2. 比较字符串
可以使用比较操作符(如 ==
, !=
, <
, >
, <=
, >=
)比较字符串:
s1 = "hello"
s2 = "world"
print(s1 == s2) # 输出: False
print(s1 < s2) # 输出: True
3. 统计字符串长度
可以使用 len()
函数统计字符串的长度:
s = "hello world"
length = len(s)
print(length) # 输出: 11
4. 统计子字符串出现次数
可以使用 count()
方法统计子字符串在字符串中出现的次数:
s = "hello world"
count = s.count("l")
print(count) # 输出: 3
九、字符串的高级操作
在一些高级应用中,可能需要对字符串进行更复杂的操作,如反转字符串、检查回文等。
1. 反转字符串
可以使用切片操作反转字符串:
s = "hello world"
reversed_s = s[::-1]
print(reversed_s) # 输出: dlrow olleh
2. 检查回文
回文是指正读和反读都相同的字符串。可以通过反转字符串并与原字符串比较来检查回文:
s = "madam"
is_palindrome = s == s[::-1]
print(is_palindrome) # 输出: True
十、字符串与数据类型转换
在处理数据时,可能需要在字符串和其他数据类型之间进行转换。以下是一些常见的转换操作:
1. 字符串转换为整数
可以使用 int()
函数将字符串转换为整数:
s = "123"
num = int(s)
print(num) # 输出: 123
2. 字符串转换为浮点数
可以使用 float()
函数将字符串转换为浮点数:
s = "123.45"
num = float(s)
print(num) # 输出: 123.45
3. 整数转换为字符串
可以使用 str()
函数将整数转换为字符串:
num = 123
s = str(num)
print(s) # 输出: 123
4. 浮点数转换为字符串
可以使用 str()
函数将浮点数转换为字符串:
num = 123.45
s = str(num)
print(s) # 输出: 123.45
十一、字符串的编码解码
在处理不同语言的文本时,字符串的编码和解码是一个非常重要的问题。Python 提供了多种编码方式,例如 UTF-8、UTF-16、ASCII 等。
1. 编码字符串
可以使用 encode()
方法将字符串编码为字节对象:
s = "你好世界"
encoded_s = s.encode("utf-8")
print(encoded_s) # 输出: b'\xe4\xbd\xa0\xe5\xa5\xbd\xe4\xb8\x96\xe7\x95\x8c'
2. 解码字符串
可以使用 decode()
方法将字节对象解码为字符串:
encoded_s = b'\xe4\xbd\xa0\xe5\xa5\xbd\xe4\xb8\x96\xe7\x95\x8c'
decoded_s = encoded_s.decode("utf-8")
print(decoded_s) # 输出: 你好世界
十二、字符串的文件读写
在处理文件时,可能需要读写字符串。Python 提供了内置的文件操作函数,可以方便地进行文件读写。
1. 写入字符串到文件
可以使用 open()
函数打开文件,并使用 write()
方法将字符串写入文件:
s = "hello world"
with open("output.txt", "w") as file:
file.write(s)
2. 从文件读取字符串
可以使用 open()
函数打开文件,并使用 read()
方法读取文件中的字符串:
with open("output.txt", "r") as file:
s = file.read()
print(s) # 输出: hello world
十三、字符串的性能优化
在处理大规模字符串时,性能优化是一个重要的考虑因素。以下是一些优化字符串操作性能的方法:
1. 使用列表拼接字符串
在需要拼接大量字符串时,使用列表拼接比使用 +
操作符更高效:
strings = ["hello", "world", "foo", "bar"]
result = "".join(strings)
print(result) # 输出: helloworldfoobar
2. 使用生成器表达式
在需要对字符串进行复杂操作时,使用生成器表达式可以提高性能:
s = "hello world"
new_s = "".join(c.upper() for c in s if c != " ")
print(new_s) # 输出: HELLOWORLD
十四、总结
本文详细介绍了 Python 中改变字符串的各种方法,包括字符串方法、切片、拼接、正则表达式、格式化字符串、编码解码、模板字符串、常见操作、高级操作、数据类型转换、文件读写以及性能优化。通过这些方法,可以灵活地处理和操作字符串,以满足各种编程需求。希望本文对你理解和掌握 Python 字符串操作有所帮助。
相关问答FAQs:
如何在Python中转换字符串的大小写?
在Python中,可以使用字符串对象的.upper()
、.lower()
和.title()
方法来改变字符串的大小写。.upper()
会将所有字母转换为大写,.lower()
会将所有字母转换为小写,而.title()
则会将每个单词的首字母变为大写。例如:
text = "hello world"
print(text.upper()) # 输出: HELLO WORLD
print(text.lower()) # 输出: hello world
print(text.title()) # 输出: Hello World
如何在Python中替换字符串中的特定字符或子字符串?
使用字符串对象的.replace()
方法可以轻松地替换字符串中的特定字符或子字符串。该方法接受两个参数,第一个是要替换的内容,第二个是新的内容。例如:
text = "I love Python"
new_text = text.replace("Python", "programming")
print(new_text) # 输出: I love programming
这让你可以在处理文本时灵活地进行内容修改。
如何在Python中去除字符串的空格或特定字符?
若需去除字符串开头和结尾的空格或特定字符,可以使用.strip()
方法。此方法会返回一个新的字符串,去掉了指定字符(默认为空格)。例如:
text = " Hello, World! "
cleaned_text = text.strip()
print(cleaned_text) # 输出: Hello, World!
如果希望只去除特定字符,可以在括号内指定。例如,text.strip(" ")
将只去除空格。
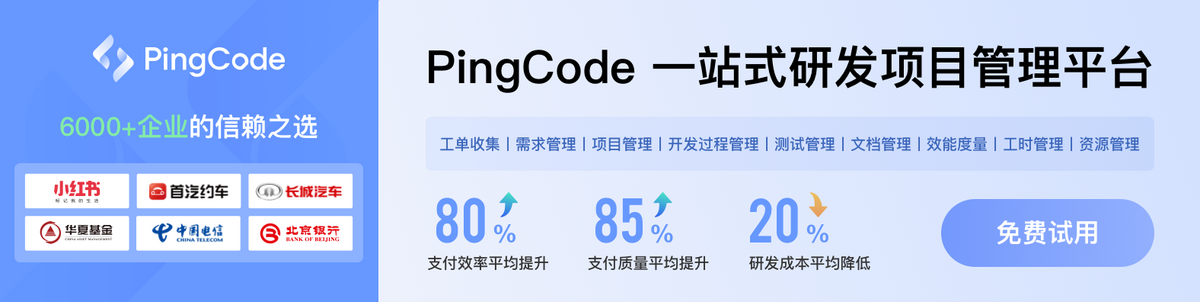