在Python中导入文本并进行词频统计的主要步骤包括:读取文本文件、分词处理、统计词频以及展示结果。首先,你可以使用内置的open()
函数或pandas
库来读取文本文件、然后使用collections.Counter
来统计词频、最后将结果进行展示。下面将详细介绍其中一种方法:
使用open()
函数读取文本文件:
# 使用内置的 open() 函数读取文本文件
with open('example.txt', 'r', encoding='utf-8') as file:
text = file.read()
步骤一、读取文本文件
读取文本文件是进行词频统计的第一步。可以使用内置的open()
函数或pandas
库的read_csv()
函数来读取文本文件。以下是使用open()
函数读取文本文件的示例:
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
file_path = 'example.txt'
text = read_file(file_path)
步骤二、分词处理
在读取文本之后,需要对文本进行分词处理。分词是将文本分割成一个个单词或词组的过程。在Python中,可以使用nltk
库或re
库来进行分词处理。以下是使用nltk
库进行分词处理的示例:
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
def tokenize_text(text):
tokens = word_tokenize(text)
return tokens
tokens = tokenize_text(text)
步骤三、统计词频
在分词处理之后,可以使用collections.Counter
来统计词频。Counter
是一个专门用于计数的字典,可以方便地统计每个单词出现的次数。以下是使用Counter
进行词频统计的示例:
from collections import Counter
def count_word_frequencies(tokens):
word_frequencies = Counter(tokens)
return word_frequencies
word_frequencies = count_word_frequencies(tokens)
步骤四、展示结果
在统计完词频之后,可以将结果进行展示。可以使用pandas
库将结果转换成DataFrame格式,方便进行排序和展示。以下是展示结果的示例:
import pandas as pd
def display_word_frequencies(word_frequencies):
df = pd.DataFrame(word_frequencies.items(), columns=['Word', 'Frequency'])
df = df.sort_values(by='Frequency', ascending=False)
print(df)
display_word_frequencies(word_frequencies)
总结
以上步骤展示了如何在Python中导入文本并进行词频统计。首先,使用open()
函数读取文本文件,然后使用nltk
库进行分词处理,接着使用collections.Counter
进行词频统计,最后使用pandas
库将结果进行展示。通过这些步骤,你可以方便地对文本文件进行词频统计,并对结果进行分析和展示。
一、读取文本文件
在进行词频统计之前,首先需要将文本文件导入到Python中。可以使用内置的open()
函数或pandas
库来读取文本文件。以下是详细介绍:
1. 使用 open()
函数读取文本文件
open()
函数是Python内置的函数,用于打开文件并返回文件对象。可以使用该函数读取文本文件的内容。以下是使用 open()
函数读取文本文件的示例:
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
file_path = 'example.txt'
text = read_file(file_path)
在这个示例中,read_file
函数接受文件路径作为参数,并使用 open()
函数以只读模式 ('r'
) 打开文件。通过指定编码为 utf-8
,可以确保正确读取包含非ASCII字符的文本文件。然后,使用 read()
方法读取文件内容,并将其存储在 text
变量中。
2. 使用 pandas
库读取文本文件
如果文本文件是以CSV或其他表格格式存储的,可以使用 pandas
库来读取文本文件。pandas
提供了强大的数据处理和分析功能,适用于处理结构化数据。以下是使用 pandas
库读取文本文件的示例:
import pandas as pd
def read_csv_file(file_path):
df = pd.read_csv(file_path, encoding='utf-8')
return df
file_path = 'example.csv'
df = read_csv_file(file_path)
在这个示例中,read_csv_file
函数接受文件路径作为参数,并使用 pandas
库的 read_csv()
函数读取CSV文件。通过指定编码为 utf-8
,可以确保正确读取包含非ASCII字符的文本文件。读取的结果是一个DataFrame对象,可以方便地进行数据处理和分析。
二、分词处理
在读取文本文件之后,需要对文本进行分词处理。分词是将文本分割成一个个单词或词组的过程。在Python中,可以使用 nltk
库或 re
库来进行分词处理。以下是详细介绍:
1. 使用 nltk
库进行分词处理
nltk
库是Python中自然语言处理的常用库,提供了丰富的文本处理功能。可以使用 nltk
库的 word_tokenize()
函数进行分词处理。以下是使用 nltk
库进行分词处理的示例:
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
def tokenize_text(text):
tokens = word_tokenize(text)
return tokens
tokens = tokenize_text(text)
在这个示例中,首先需要下载 nltk
库的 punkt
数据包,它包含了用于分词的预训练模型。然后,使用 word_tokenize()
函数将文本分割成单词列表。
2. 使用 re
库进行分词处理
如果不想依赖外部库,也可以使用Python内置的 re
库进行分词处理。re
库提供了正则表达式的功能,可以用来匹配和分割文本。以下是使用 re
库进行分词处理的示例:
import re
def tokenize_text(text):
tokens = re.findall(r'\b\w+\b', text)
return tokens
tokens = tokenize_text(text)
在这个示例中,使用 re.findall()
函数匹配所有的单词,并将其存储在列表中。正则表达式 \b\w+\b
用于匹配单词边界内的一个或多个字母、数字或下划线字符。
三、统计词频
在分词处理之后,可以使用 collections.Counter
来统计词频。Counter
是一个专门用于计数的字典,可以方便地统计每个单词出现的次数。以下是详细介绍:
1. 使用 collections.Counter
统计词频
collections.Counter
是Python标准库中的一个类,用于计数和统计数据。可以使用 Counter
来统计分词后的单词列表中的每个单词出现的次数。以下是使用 Counter
进行词频统计的示例:
from collections import Counter
def count_word_frequencies(tokens):
word_frequencies = Counter(tokens)
return word_frequencies
word_frequencies = count_word_frequencies(tokens)
在这个示例中,count_word_frequencies
函数接受分词后的单词列表作为参数,并使用 Counter
对其进行计数。返回的结果是一个 Counter
对象,它是一个字典,其中键是单词,值是单词出现的次数。
四、展示结果
在统计完词频之后,可以将结果进行展示。可以使用 pandas
库将结果转换成DataFrame格式,方便进行排序和展示。以下是详细介绍:
1. 使用 pandas
库展示结果
pandas
库提供了强大的数据处理和分析功能,可以将词频统计结果转换成DataFrame格式,方便进行排序和展示。以下是使用 pandas
库展示结果的示例:
import pandas as pd
def display_word_frequencies(word_frequencies):
df = pd.DataFrame(word_frequencies.items(), columns=['Word', 'Frequency'])
df = df.sort_values(by='Frequency', ascending=False)
print(df)
display_word_frequencies(word_frequencies)
在这个示例中,display_word_frequencies
函数接受 Counter
对象作为参数,并将其转换成DataFrame格式。通过 sort_values()
方法按词频降序排序,并打印结果。
五、综合示例
下面是一个综合示例,展示了如何将上述步骤整合在一起,完成从读取文本文件到展示词频统计结果的全过程:
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
from collections import Counter
import pandas as pd
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
def tokenize_text(text):
tokens = word_tokenize(text)
return tokens
def count_word_frequencies(tokens):
word_frequencies = Counter(tokens)
return word_frequencies
def display_word_frequencies(word_frequencies):
df = pd.DataFrame(word_frequencies.items(), columns=['Word', 'Frequency'])
df = df.sort_values(by='Frequency', ascending=False)
print(df)
file_path = 'example.txt'
text = read_file(file_path)
tokens = tokenize_text(text)
word_frequencies = count_word_frequencies(tokens)
display_word_frequencies(word_frequencies)
在这个综合示例中,首先使用 read_file
函数读取文本文件,然后使用 tokenize_text
函数进行分词处理,接着使用 count_word_frequencies
函数统计词频,最后使用 display_word_frequencies
函数展示结果。通过这些步骤,可以方便地对文本文件进行词频统计,并对结果进行分析和展示。
六、处理更复杂的文本
在实际应用中,可能会遇到更复杂的文本,需要进行更复杂的处理。例如,处理标点符号、大小写转换、去除停用词等。以下是一些常见的处理方法:
1. 处理标点符号
在进行词频统计时,通常需要去除标点符号。可以使用正则表达式来去除文本中的标点符号。以下是去除标点符号的示例:
import re
def remove_punctuation(text):
text = re.sub(r'[^\w\s]', '', text)
return text
text = remove_punctuation(text)
在这个示例中,使用 re.sub()
函数将文本中的标点符号替换为空字符。正则表达式 [^\w\s]
用于匹配所有非字母、数字和空白字符的标点符号。
2. 大小写转换
为了避免同一个单词因大小写不同而被统计为不同的词,可以将文本转换为小写。以下是将文本转换为小写的示例:
def convert_to_lowercase(text):
text = text.lower()
return text
text = convert_to_lowercase(text)
在这个示例中,使用字符串的 lower()
方法将文本转换为小写。
3. 去除停用词
停用词是指在文本中频繁出现但没有实际意义的词,例如“the”、“and”、“is”等。可以使用 nltk
库的停用词列表来去除文本中的停用词。以下是去除停用词的示例:
from nltk.corpus import stopwords
nltk.download('stopwords')
def remove_stopwords(tokens):
stop_words = set(stopwords.words('english'))
tokens = [word for word in tokens if word not in stop_words]
return tokens
tokens = remove_stopwords(tokens)
在这个示例中,首先下载 nltk
库的停用词数据包,然后使用 stopwords.words('english')
获取英文的停用词列表。接着,使用列表推导式去除单词列表中的停用词。
七、综合处理示例
下面是一个综合处理示例,展示了如何处理更复杂的文本,包括去除标点符号、大小写转换和去除停用词:
import nltk
nltk.download('punkt')
nltk.download('stopwords')
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
from collections import Counter
import pandas as pd
import re
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
def remove_punctuation(text):
text = re.sub(r'[^\w\s]', '', text)
return text
def convert_to_lowercase(text):
text = text.lower()
return text
def tokenize_text(text):
tokens = word_tokenize(text)
return tokens
def remove_stopwords(tokens):
stop_words = set(stopwords.words('english'))
tokens = [word for word in tokens if word not in stop_words]
return tokens
def count_word_frequencies(tokens):
word_frequencies = Counter(tokens)
return word_frequencies
def display_word_frequencies(word_frequencies):
df = pd.DataFrame(word_frequencies.items(), columns=['Word', 'Frequency'])
df = df.sort_values(by='Frequency', ascending=False)
print(df)
file_path = 'example.txt'
text = read_file(file_path)
text = remove_punctuation(text)
text = convert_to_lowercase(text)
tokens = tokenize_text(text)
tokens = remove_stopwords(tokens)
word_frequencies = count_word_frequencies(tokens)
display_word_frequencies(word_frequencies)
在这个综合处理示例中,首先使用 read_file
函数读取文本文件,然后依次使用 remove_punctuation
、convert_to_lowercase
、tokenize_text
和 remove_stopwords
函数对文本进行处理,接着使用 count_word_frequencies
函数统计词频,最后使用 display_word_frequencies
函数展示结果。通过这些步骤,可以对更复杂的文本进行词频统计,并对结果进行分析和展示。
八、处理大规模文本
在处理大规模文本时,可能会遇到内存不足的问题。可以使用生成器和分块处理的方法来处理大规模文本,以节省内存。以下是处理大规模文本的示例:
1. 使用生成器读取大规模文本
生成器是一种特殊的迭代器,可以逐行读取大规模文本,避免一次性将整个文件加载到内存中。以下是使用生成器读取大规模文本的示例:
def read_large_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
yield line
file_path = 'large_example.txt'
for line in read_large_file(file_path):
print(line.strip())
在这个示例中,read_large_file
函数使用生成器逐行读取大规模文本文件。通过使用 yield
关键字,可以在每次迭代时返回一行文本,而不是一次性将整个文件加载到内存中。
2. 分块处理大规模文本
可以将大规模文本分成多个小块,逐块进行处理,以节省内存。以下是分块处理大规模文本的示例:
def process_large_file(file_path, chunk_size=1000):
word_frequencies = Counter()
with open(file_path, 'r', encoding='utf-8') as file:
chunk = []
for line in file:
chunk.append(line.strip())
if len(chunk) >= chunk_size:
text = ' '.join(chunk)
tokens = word_tokenize(text)
word_frequencies.update(tokens)
chunk = []
if chunk:
text = ' '.join(chunk)
tokens = word_tokenize(text)
word_frequencies.update(tokens)
return word_frequencies
file_path = 'large_example.txt'
word_frequencies = process_large_file(file_path)
display_word_frequencies(word_frequencies)
在这个示例中,process_large_file
函数将大规模文本分成多个小块进行处理。每次读取 chunk_size
行文本,并将其合并成一个字符串,然后进行分词和词频统计。通过逐块处理,可以有效节省内存。
九、总结
在Python中导入文本并进行词频统计的主要步骤包括:读取文本文件、分词处理、统计词频以及展示结果。可以使用内置的 open()
函数或 pandas
库来读取文本文件
相关问答FAQs:
如何在Python中读取文本文件以进行词频统计?
在Python中,可以使用内置的open()
函数来读取文本文件。打开文件后,可以使用read()
方法读取整个文件内容,或使用readlines()
方法逐行读取。读取文本后,利用split()
方法将文本分割为单词,以便进行词频统计。
是否可以从其他数据源导入文本进行词频分析?
除了本地文本文件,Python还支持从网络、数据库或API导入文本数据。例如,可以使用requests
库从网页获取文本,或使用pandas
库从CSV文件中读取数据。确保在读取数据之前清洗文本,以提高词频统计的准确性。
使用Python进行词频统计时有哪些常用的库?
在进行词频统计时,常用的库包括collections
中的Counter
,它可以轻松统计文本中每个单词的出现次数。此外,nltk
和spaCy
等自然语言处理库也提供了丰富的功能,可以帮助进行更复杂的文本分析和处理。使用这些库可以显著提高词频统计的效率和准确性。
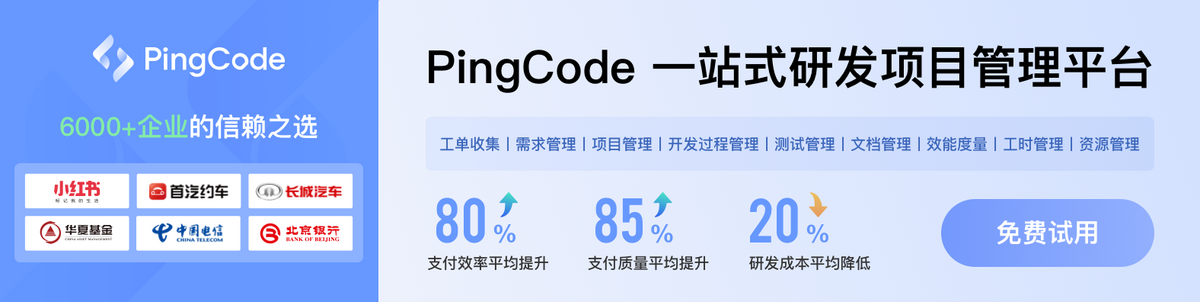