Python访问文件的地址可以使用多种方法,包括使用内置的 open
函数、使用 os
和 pathlib
模块等。最常见的方法是使用 open
函数、os.path
模块、pathlib
模块。下面将详细介绍其中一种方法来解释如何使用 open
函数进行文件访问。
使用open
函数: 该方法是最基础的文件访问方式。它可以打开文件进行读、写、追加操作。首先,你需要知道文件的路径,并用 open
函数打开它。然后,你可以对文件进行各种操作,例如读取文件内容、写入数据等。打开文件后,别忘了关闭文件,以释放资源。
# 打开文件进行读取
file_path = "example.txt"
file = open(file_path, "r")
content = file.read()
print(content)
file.close()
现在,让我们详细介绍Python访问文件地址的其他方法。
一、使用 open
函数访问文件
1、打开文件进行读取
使用 open
函数可以轻松地打开文件并读取内容。需要提供文件路径和模式参数。
file_path = "example.txt"
with open(file_path, "r") as file:
content = file.read()
print(content)
在这个示例中,文件路径是 example.txt
,模式是 "r"
(读取模式)。使用 with
关键字可以确保文件在操作完成后自动关闭。
2、写入文件
如果你需要向文件中写入数据,可以使用 "w"
模式打开文件。这将覆盖文件的现有内容。
file_path = "example.txt"
with open(file_path, "w") as file:
file.write("Hello, World!")
使用 "w"
模式打开文件将创建一个新文件(如果文件不存在)或覆盖现有文件的内容。
3、追加写入文件
如果你想保留文件的现有内容,并在末尾追加数据,可以使用 "a"
模式。
file_path = "example.txt"
with open(file_path, "a") as file:
file.write("\nThis is an appended line.")
4、读取文件的每一行
有时候,你可能需要逐行读取文件内容。你可以使用 readlines
方法或直接迭代文件对象。
file_path = "example.txt"
with open(file_path, "r") as file:
lines = file.readlines()
for line in lines:
print(line.strip())
或使用迭代方式:
file_path = "example.txt"
with open(file_path, "r") as file:
for line in file:
print(line.strip())
二、使用 os.path
模块访问文件
1、检查文件是否存在
在访问文件之前,通常需要检查文件是否存在。os.path
模块提供了 exists
方法来实现这一点。
import os
file_path = "example.txt"
if os.path.exists(file_path):
print("File exists.")
else:
print("File does not exist.")
2、获取文件的绝对路径
你可以使用 os.path.abspath
方法获取文件的绝对路径。
import os
file_path = "example.txt"
absolute_path = os.path.abspath(file_path)
print(absolute_path)
3、获取文件的目录名和基名
os.path
模块还提供了 dirname
和 basename
方法来获取文件的目录名和基名。
import os
file_path = "example.txt"
directory_name = os.path.dirname(file_path)
base_name = os.path.basename(file_path)
print("Directory:", directory_name)
print("Base name:", base_name)
4、拼接路径
os.path.join
方法可以用于拼接路径,以生成平台独立的文件路径。
import os
directory = "/path/to/directory"
file_name = "example.txt"
file_path = os.path.join(directory, file_name)
print(file_path)
三、使用 pathlib
模块访问文件
pathlib
模块是 Python 3.4 引入的,用于处理文件路径的面向对象模块。
1、创建路径对象
你可以使用 Path
类创建路径对象,并进行文件操作。
from pathlib import Path
file_path = Path("example.txt")
2、检查文件是否存在
使用 exists
方法检查文件是否存在。
from pathlib import Path
file_path = Path("example.txt")
if file_path.exists():
print("File exists.")
else:
print("File does not exist.")
3、读取文件内容
使用 read_text
方法读取文件内容。
from pathlib import Path
file_path = Path("example.txt")
content = file_path.read_text()
print(content)
4、写入文件内容
使用 write_text
方法写入文件内容。
from pathlib import Path
file_path = Path("example.txt")
file_path.write_text("Hello, World!")
5、获取绝对路径
使用 resolve
方法获取文件的绝对路径。
from pathlib import Path
file_path = Path("example.txt")
absolute_path = file_path.resolve()
print(absolute_path)
6、迭代目录中的文件
你可以使用 iterdir
方法迭代目录中的文件和子目录。
from pathlib import Path
directory = Path("/path/to/directory")
for file in directory.iterdir():
print(file)
四、处理不同操作系统的路径差异
不同操作系统的路径格式不一样,Python 提供了一些工具来处理这些差异。
1、使用 os.path
处理路径
os.path
模块可以处理不同操作系统的路径差异。
import os
file_path = "example.txt"
absolute_path = os.path.abspath(file_path)
print(absolute_path)
2、使用 pathlib
模块处理路径
pathlib
模块也可以处理不同操作系统的路径差异,并且提供了一种更为直观的路径操作方式。
from pathlib import Path
file_path = Path("example.txt")
absolute_path = file_path.resolve()
print(absolute_path)
3、使用 os
模块的 sep
属性
os
模块的 sep
属性可以获取当前操作系统的路径分隔符。
import os
separator = os.sep
print("Path separator:", separator)
4、处理路径中的特殊字符
有时路径中可能包含特殊字符,如空格或其他非 ASCII 字符。你可以使用 os.path
或 pathlib
模块来处理这些路径。
import os
file_path = "example file.txt"
absolute_path = os.path.abspath(file_path)
print(absolute_path)
from pathlib import Path
file_path = Path("example file.txt")
absolute_path = file_path.resolve()
print(absolute_path)
五、其他文件操作
1、删除文件
你可以使用 os.remove
或 pathlib.Path.unlink
方法删除文件。
import os
file_path = "example.txt"
if os.path.exists(file_path):
os.remove(file_path)
print("File deleted.")
else:
print("File does not exist.")
from pathlib import Path
file_path = Path("example.txt")
if file_path.exists():
file_path.unlink()
print("File deleted.")
else:
print("File does not exist.")
2、重命名文件
你可以使用 os.rename
或 pathlib.Path.rename
方法重命名文件。
import os
old_path = "example.txt"
new_path = "new_example.txt"
if os.path.exists(old_path):
os.rename(old_path, new_path)
print("File renamed.")
else:
print("File does not exist.")
from pathlib import Path
old_path = Path("example.txt")
new_path = Path("new_example.txt")
if old_path.exists():
old_path.rename(new_path)
print("File renamed.")
else:
print("File does not exist.")
3、创建和删除目录
你可以使用 os.mkdir
和 os.rmdir
方法创建和删除目录,或者使用 pathlib.Path.mkdir
和 pathlib.Path.rmdir
方法。
import os
directory = "new_directory"
if not os.path.exists(directory):
os.mkdir(directory)
print("Directory created.")
else:
print("Directory already exists.")
import os
directory = "new_directory"
if os.path.exists(directory):
os.rmdir(directory)
print("Directory deleted.")
else:
print("Directory does not exist.")
from pathlib import Path
directory = Path("new_directory")
if not directory.exists():
directory.mkdir()
print("Directory created.")
else:
print("Directory already exists.")
from pathlib import Path
directory = Path("new_directory")
if directory.exists():
directory.rmdir()
print("Directory deleted.")
else:
print("Directory does not exist.")
六、总结
在这篇文章中,我们详细介绍了如何在Python中访问文件的地址。包括使用open
函数、os.path
模块和pathlib
模块进行文件访问和操作。每种方法都有其独特的优点和适用场景。通过这些方法,你可以轻松地在Python中进行文件操作,无论是读取文件内容、写入文件、检查文件是否存在、获取文件路径等。希望这些内容能帮助你更好地理解和使用Python进行文件操作。
相关问答FAQs:
如何在Python中获取文件的绝对路径?
在Python中,可以使用os.path.abspath()
函数来获取文件的绝对路径。你只需要传入相对路径或文件名,函数会返回该文件的完整路径。例如:
import os
file_name = "example.txt"
absolute_path = os.path.abspath(file_name)
print(absolute_path)
这样可以确保你获取到的路径是完整的,方便后续的文件操作。
如何验证文件路径是否存在?
在处理文件之前,验证路径的存在性是非常重要的。可以使用os.path.exists()
函数来检查文件路径是否有效。示例代码如下:
import os
file_path = "example.txt"
if os.path.exists(file_path):
print("文件存在")
else:
print("文件不存在")
这能够帮助你避免因路径错误而导致的文件操作异常。
如何在Python中构建文件路径以提高跨平台兼容性?
使用os.path.join()
函数可以帮助你构建适用于不同操作系统的文件路径。它会自动处理路径分隔符问题。示例如下:
import os
directory = "folder"
file_name = "example.txt"
file_path = os.path.join(directory, file_name)
print(file_path)
这样即使在不同的操作系统上运行,代码仍然可以正常工作,确保路径的正确性。
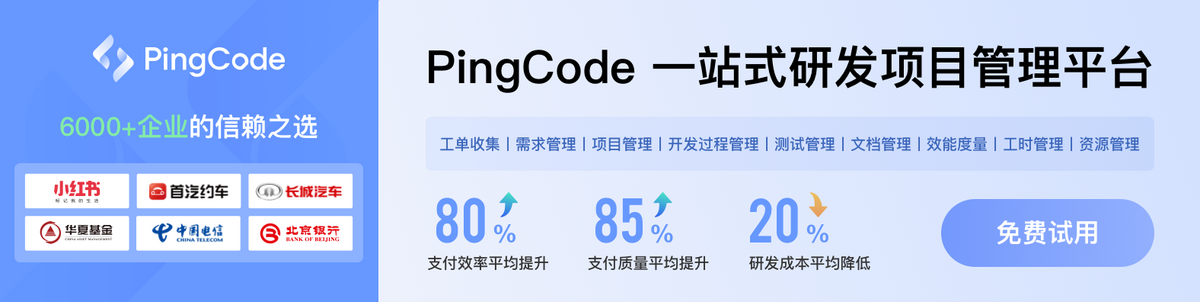