在Python中去除标点符号可以使用正则表达式、字符串翻译表、NLP库等方法,其中正则表达式是最常用的方法。
为了详细描述正则表达式的方法,我们可以使用Python的 re
模块来实现。首先,我们需要导入该模块,并定义一个包含所有标点符号的正则表达式模式。然后,我们可以使用 re.sub
函数来替换字符串中的所有标点符号。
例如:
import re
def remove_punctuation(text):
pattern = r'[^\w\s]'
return re.sub(pattern, '', text)
text = "Hello, world! Welcome to Python programming."
cleaned_text = remove_punctuation(text)
print(cleaned_text)
上面的代码会输出:
Hello world Welcome to Python programming
这种方法简单且高效,适用于大多数情况下的标点符号去除需求。
接下来,我们将详细介绍各种方法,包括正则表达式、字符串翻译表、NLP库等,以便您在不同的场景中选择合适的方法。
一、正则表达式
1、基本原理
正则表达式是一种强大的文本处理工具,它通过定义模式来匹配字符串中的特定部分。在Python中,可以使用 re
模块来处理正则表达式。
2、示例代码
上面已经展示了一个简单的示例代码,这里我们再深入一些,介绍如何处理多行文本和不同类型的标点符号。
import re
def remove_punctuation(text):
# 定义标点符号模式
pattern = r'[^\w\s]'
# 使用re.sub进行替换
cleaned_text = re.sub(pattern, '', text)
return cleaned_text
text = """Hello, world!
Welcome to Python programming.
Let's write some code: def func(x): return x * 2
"""
cleaned_text = remove_punctuation(text)
print(cleaned_text)
输出结果为:
Hello world
Welcome to Python programming
Lets write some code def funcx return x 2
3、注意事项
- 效率:正则表达式在处理较大文本时效率较高,但在极大规模文本中仍需注意性能。
- 字符集:确保正则表达式模式包含所有可能的标点符号,特别是非英语字符集的标点符号。
二、字符串翻译表
1、基本原理
字符串翻译表是一种通过映射来替换或删除字符的方法。在Python中,可以使用 str.maketrans
和 str.translate
方法来创建和应用翻译表。
2、示例代码
def remove_punctuation(text):
# 创建标点符号翻译表
punctuation = '''!()-[]{};:'"\,<>./?@#$%^&*_~'''
translator = str.maketrans('', '', punctuation)
# 使用translate进行替换
cleaned_text = text.translate(translator)
return cleaned_text
text = "Hello, world! Welcome to Python programming."
cleaned_text = remove_punctuation(text)
print(cleaned_text)
输出结果为:
Hello world Welcome to Python programming
3、注意事项
- 定制性:可以根据需求定制翻译表,添加或删除特定符号。
- 效率:对于较小文本,翻译表方法效率较高,但在处理大量文本时,性能可能不如正则表达式。
三、NLP库
1、基本原理
自然语言处理(NLP)库如 NLTK 和 SpaCy 提供了丰富的文本处理功能,包括去除标点符号。这些库可以处理多种语言和复杂文本结构。
2、示例代码
使用NLTK:
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
import string
nltk.download('punkt')
nltk.download('stopwords')
def remove_punctuation(text):
tokens = word_tokenize(text)
words = [word for word in tokens if word.isalnum()]
cleaned_text = ' '.join(words)
return cleaned_text
text = "Hello, world! Welcome to Python programming."
cleaned_text = remove_punctuation(text)
print(cleaned_text)
输出结果为:
Hello world Welcome to Python programming
使用SpaCy:
import spacy
nlp = spacy.load("en_core_web_sm")
def remove_punctuation(text):
doc = nlp(text)
tokens = [token.text for token in doc if not token.is_punct]
cleaned_text = ' '.join(tokens)
return cleaned_text
text = "Hello, world! Welcome to Python programming."
cleaned_text = remove_punctuation(text)
print(cleaned_text)
输出结果为:
Hello world Welcome to Python programming
3、注意事项
- 依赖性:需要安装和下载相应的NLP库和模型。
- 功能丰富:NLP库提供了更多的文本处理功能,如词性标注、命名实体识别等,适用于复杂文本处理需求。
四、对比与选择
1、正则表达式 vs 字符串翻译表
- 正则表达式:适用于复杂模式匹配和替换,处理较大文本时效率高。
- 字符串翻译表:适用于简单字符替换,处理较小文本时效率高。
2、NLP库 vs 其他方法
- NLP库:适用于需要更多文本处理功能的场景,如词性标注、命名实体识别等。
- 其他方法:适用于简单的标点符号去除需求。
五、总结
在Python中去除标点符号有多种方法可供选择,包括正则表达式、字符串翻译表、NLP库等。不同的方法适用于不同的场景和需求。正则表达式方法灵活且高效,适用于大多数情况;字符串翻译表方法简单高效,适用于较小文本;NLP库提供了丰富的功能,适用于复杂文本处理需求。在实际应用中,可以根据具体情况选择合适的方法,以达到最佳的处理效果。
通过本文的详细介绍,希望能帮助您更好地理解和应用Python中的标点符号去除技术。如果您有其他问题或需要进一步的指导,请随时联系我。
相关问答FAQs:
如何在Python中去除字符串中的所有标点符号?
在Python中,可以使用str.translate()
方法和str.maketrans()
函数来去除字符串中的标点符号。首先,需要创建一个翻译表,将所有标点符号映射到None
,然后调用translate()
方法进行替换。例如:
import string
text = "Hello, world! How's it going?"
translator = str.maketrans('', '', string.punctuation)
cleaned_text = text.translate(translator)
print(cleaned_text) # 输出: Hello world Hows it going
在数据清洗过程中,去除标点符号有什么意义?
去除标点符号在数据清洗中非常重要,尤其是在文本分析和自然语言处理(NLP)领域。标点符号通常不携带语义信息,因此去除它们有助于减少噪声,提高模型的准确性。这样,分析的结果更能反映文本的真实含义,有助于词频统计、情感分析等任务的有效进行。
有没有其他方法可以在Python中去除标点符号?
除了使用str.translate()
,还可以使用正则表达式(re
模块)来去除标点符号。通过使用re.sub()
方法,可以通过指定正则模式替换掉所有标点符号。示例代码如下:
import re
text = "Hello, world! How's it going?"
cleaned_text = re.sub(r'[^\w\s]', '', text)
print(cleaned_text) # 输出: Hello world Hows it going
这种方法灵活性较高,可以根据需求调整匹配的模式。
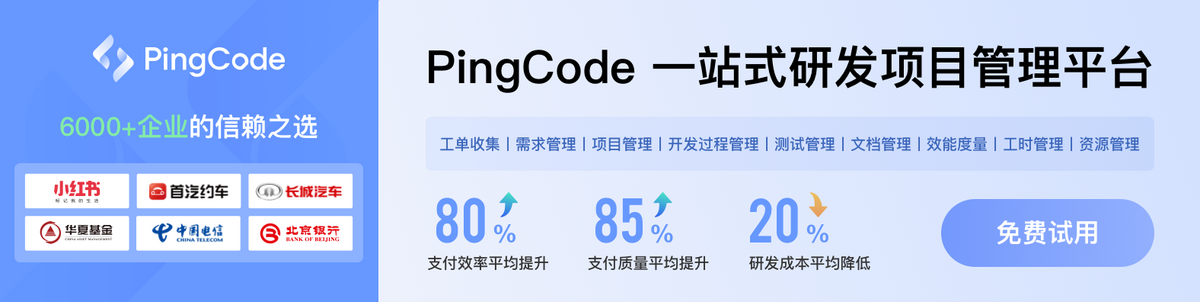