一、使用Python查找Word文档中的图片可以通过以下几种方法:使用python-docx库、使用comtypes库、使用PyWin32库。其中,使用python-docx库是最常见且相对简单的方法。下面将详细介绍如何使用python-docx库来查找Word文档中的图片。
使用python-docx库查找Word文档中的图片
python-docx
是一个用于创建和更新Microsoft Word(.docx)文件的Python库。该库支持读取和写入文档,包括文本、表格、图像等内容。通过python-docx库,我们可以轻松地遍历Word文档的内容,找到并提取图片。
首先,需要安装python-docx库,可以使用以下命令进行安装:
pip install python-docx
接下来,我们可以使用以下代码查找Word文档中的图片:
from docx import Document
from docx.oxml.ns import qn
from docx.oxml import OxmlElement
def find_images_in_docx(docx_path):
document = Document(docx_path)
image_files = []
for rel in document.part.rels.values():
if "image" in rel.target_ref:
image_files.append(rel.target_ref)
return image_files
docx_path = 'path/to/your/document.docx'
images = find_images_in_docx(docx_path)
print(images)
以上代码定义了一个函数find_images_in_docx
,该函数接收Word文档的路径作为参数,并返回文档中所有图片的路径。代码的核心部分是遍历文档的关系(rels),找到与图片相关的关系,并将图片路径添加到结果列表中。
二、使用comtypes库
comtypes
是一个用于调用COM对象的Python库,可以用来操作Microsoft Word等应用程序。通过comtypes库,我们可以直接使用Word的COM接口来遍历文档的内容,找到并提取图片。
首先,需要安装comtypes库,可以使用以下命令进行安装:
pip install comtypes
接下来,我们可以使用以下代码查找Word文档中的图片:
import comtypes.client
def find_images_in_docx(docx_path):
word = comtypes.client.CreateObject('Word.Application')
word.Visible = False
document = word.Documents.Open(docx_path)
image_files = []
for shape in document.InlineShapes:
if shape.Type == 3: # InlineShapeType.Picture
image_files.append(shape.LinkFormat.SourceFullName)
document.Close(False)
word.Quit()
return image_files
docx_path = 'path/to/your/document.docx'
images = find_images_in_docx(docx_path)
print(images)
以上代码定义了一个函数find_images_in_docx
,该函数接收Word文档的路径作为参数,并返回文档中所有图片的路径。代码的核心部分是使用comtypes库创建Word应用程序对象,并打开指定的文档,然后遍历文档中的所有InlineShapes,找到图片并将其路径添加到结果列表中。
三、使用PyWin32库
PyWin32
是一个用于访问Windows API的Python库,可以用来操作Microsoft Word等应用程序。通过PyWin32库,我们可以直接使用Word的COM接口来遍历文档的内容,找到并提取图片。
首先,需要安装PyWin32库,可以使用以下命令进行安装:
pip install pywin32
接下来,我们可以使用以下代码查找Word文档中的图片:
import win32com.client
def find_images_in_docx(docx_path):
word = win32com.client.Dispatch('Word.Application')
word.Visible = False
document = word.Documents.Open(docx_path)
image_files = []
for shape in document.InlineShapes:
if shape.Type == 3: # InlineShapeType.Picture
image_files.append(shape.LinkFormat.SourceFullName)
document.Close(False)
word.Quit()
return image_files
docx_path = 'path/to/your/document.docx'
images = find_images_in_docx(docx_path)
print(images)
以上代码定义了一个函数find_images_in_docx
,该函数接收Word文档的路径作为参数,并返回文档中所有图片的路径。代码的核心部分是使用PyWin32库创建Word应用程序对象,并打开指定的文档,然后遍历文档中的所有InlineShapes,找到图片并将其路径添加到结果列表中。
总结:
使用python-docx库、使用comtypes库、使用PyWin32库是查找Word文档中图片的三种常见方法。使用python-docx库是最常见且相对简单的方法,因为它不需要依赖于Windows操作系统和Microsoft Word应用程序。对于跨平台的应用程序,推荐使用python-docx库来查找Word文档中的图片。而comtypes库和PyWin32库则更适合在Windows环境下使用,特别是当需要操作Word应用程序的其他高级功能时。
四、深入理解和优化
在实际应用中,查找Word文档中的图片可能需要更复杂的操作,例如提取图片的元数据、保存图片到本地文件系统等。下面将进一步介绍如何实现这些功能。
提取图片的元数据
在查找Word文档中的图片时,我们可能需要提取图片的元数据,例如图片的大小、格式等信息。使用python-docx库可以实现这一功能。以下是示例代码:
from docx import Document
from docx.oxml.ns import qn
from docx.oxml import OxmlElement
def find_images_with_metadata(docx_path):
document = Document(docx_path)
images_metadata = []
for rel in document.part.rels.values():
if "image" in rel.target_ref:
image_part = document.part.related_parts[rel.rId]
image_data = image_part.blob
image_size = len(image_data)
image_format = rel.target_ref.split('.')[-1]
images_metadata.append({
'path': rel.target_ref,
'size': image_size,
'format': image_format
})
return images_metadata
docx_path = 'path/to/your/document.docx'
images_metadata = find_images_with_metadata(docx_path)
for metadata in images_metadata:
print(metadata)
以上代码在查找图片的同时提取了图片的元数据,包括图片的大小和格式。可以根据需要进一步扩展以提取更多的元数据信息。
保存图片到本地文件系统
在查找Word文档中的图片后,我们可能需要将图片保存到本地文件系统。以下是示例代码:
from docx import Document
from docx.oxml.ns import qn
from docx.oxml import OxmlElement
import os
def save_images_to_local(docx_path, output_dir):
document = Document(docx_path)
if not os.path.exists(output_dir):
os.makedirs(output_dir)
for rel in document.part.rels.values():
if "image" in rel.target_ref:
image_part = document.part.related_parts[rel.rId]
image_data = image_part.blob
image_name = os.path.basename(rel.target_ref)
image_path = os.path.join(output_dir, image_name)
with open(image_path, 'wb') as f:
f.write(image_data)
print(f'Saved image to {image_path}')
docx_path = 'path/to/your/document.docx'
output_dir = 'path/to/save/images'
save_images_to_local(docx_path, output_dir)
以上代码定义了一个函数save_images_to_local
,该函数接收Word文档的路径和保存图片的目录作为参数,并将文档中的所有图片保存到指定目录。代码的核心部分是遍历文档的关系,找到与图片相关的关系,提取图片数据,并将图片数据写入本地文件。
处理嵌入式图片
在Word文档中,除了内联图片(InlineShapes),还有可能存在嵌入式图片(Shapes)。嵌入式图片通常是浮动的,可以包含在文本框、图表等对象中。使用python-docx库可以处理嵌入式图片。以下是示例代码:
from docx import Document
from docx.oxml.ns import qn
from docx.oxml import OxmlElement
def find_all_images(docx_path):
document = Document(docx_path)
image_files = []
# 查找内联图片
for rel in document.part.rels.values():
if "image" in rel.target_ref:
image_files.append(rel.target_ref)
# 查找嵌入式图片
for shape in document.inline_shapes:
if shape.type == 3: # InlineShapeType.Picture
image_files.append(shape._inline.graphic.graphicData.pic.blipFill.blip.embed)
return image_files
docx_path = 'path/to/your/document.docx'
images = find_all_images(docx_path)
print(images)
以上代码在查找内联图片的基础上,进一步查找嵌入式图片,并将所有图片的路径添加到结果列表中。
处理大型文档
在处理大型Word文档时,查找图片的操作可能会变得非常耗时。为了提高性能,可以考虑以下优化措施:
- 并行处理:使用多线程或多进程并行处理文档的不同部分,以加快查找图片的速度。
- 缓存:将已处理的文档和图片信息缓存起来,避免重复处理同一个文档。
- 增量处理:对于频繁更新的文档,可以采用增量处理的方法,只处理新增或修改的部分。
以下是使用多线程并行处理的示例代码:
from docx import Document
from docx.oxml.ns import qn
from docx.oxml import OxmlElement
from concurrent.futures import ThreadPoolExecutor
import os
def find_images_in_part(doc_part):
image_files = []
for rel in doc_part.rels.values():
if "image" in rel.target_ref:
image_files.append(rel.target_ref)
return image_files
def find_images_in_docx(docx_path):
document = Document(docx_path)
parts = [document.part] + list(document.part.related_parts.values())
image_files = []
with ThreadPoolExecutor() as executor:
results = executor.map(find_images_in_part, parts)
for result in results:
image_files.extend(result)
return image_files
docx_path = 'path/to/your/document.docx'
images = find_images_in_docx(docx_path)
print(images)
以上代码使用ThreadPoolExecutor并行处理文档的不同部分,以加快查找图片的速度。通过这种方式,可以显著提高处理大型文档的性能。
五、总结
本文详细介绍了使用Python查找Word文档中图片的多种方法,包括使用python-docx库、使用comtypes库、使用PyWin32库。其中,使用python-docx库是最常见且相对简单的方法,适用于跨平台的应用程序。对于Windows环境下的高级功能操作,可以选择使用comtypes库或PyWin32库。我们还进一步介绍了如何提取图片的元数据、保存图片到本地文件系统、处理嵌入式图片、以及优化处理大型文档的性能。希望通过本文的介绍,读者可以掌握查找Word文档中图片的技巧,并能够灵活应用于实际项目中。
相关问答FAQs:
如何在Python中找到Word文档中的所有图片?
要在Word文档中找到所有图片,可以使用python-docx
库。这是一个流行的库,能够读取和修改Word文件。通过遍历文档的每个段落和形状,您可以提取出所有的图片。示例代码如下:
from docx import Document
doc = Document('your_document.docx')
for rel in doc.part.rels.values():
if "image" in rel.reltype:
print(rel.target_ref)
该代码将打印出所有图片的路径。
我可以在Word文档中提取图片并保存到本地吗?
当然可以。在找到图片后,可以使用PIL
库将其保存到本地。以下是一个示例,展示如何提取并保存图片:
from docx import Document
from PIL import Image
import io
doc = Document('your_document.docx')
for rel in doc.part.rels.values():
if "image" in rel.reltype:
image_stream = rel.target_part.blob
image = Image.open(io.BytesIO(image_stream))
image.save(f'output_image_{rel.target_ref.split("/")[-1]}')
这段代码会将所有图片保存为本地文件。
使用Python查找Word文档中的图片需要安装哪些库?
要实现查找和处理Word文档中的图片,您需要安装python-docx
和Pillow
(用于处理图像)。可以通过以下命令安装这些库:
pip install python-docx Pillow
安装完成后,您就可以开始编写代码,提取和处理Word文档中的图片。
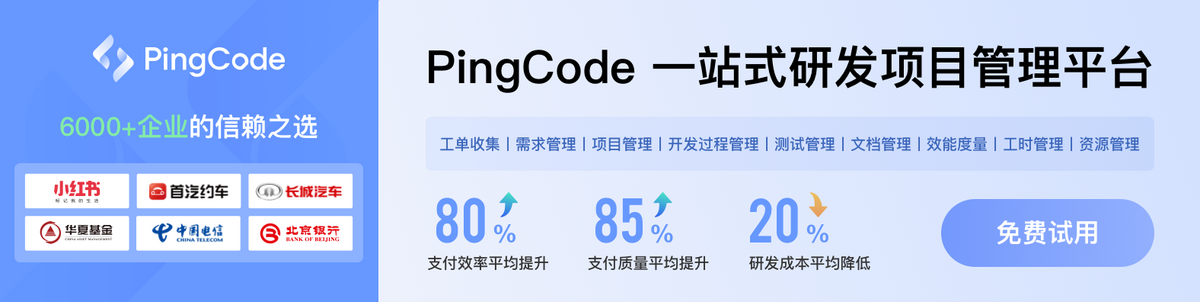