Python可以通过多种方式实现时刻监听状态,例如使用回调函数、多线程、异步编程、轮询等方式。其中,使用多线程和异步编程是比较常见且高效的方式。在这里,我们将详细描述如何使用多线程来实现时刻监听状态。
多线程编程是一种允许程序同时执行多个操作的技术。在Python中,多线程可以通过threading
模块来实现。通过创建多个线程,每个线程负责监听不同的状态,从而实现对多个状态的实时监听。
一、线程与多线程编程
1、什么是线程
线程是操作系统能够进行运算调度的最小单位。它被包含在进程之中,是进程中的实际运作单位。一个线程指的是进程中的一个单一顺序的控制流程。
2、Python中的线程
在Python中,线程主要通过threading
模块来实现。threading
模块提供了一些方便的函数和类来创建和管理线程。
import threading
def thread_function(name):
print(f"Thread {name}: starting")
# 模拟任务
import time
time.sleep(2)
print(f"Thread {name}: finishing")
if __name__ == "__main__":
# 创建线程
threads = []
for index in range(3):
x = threading.Thread(target=thread_function, args=(index,))
threads.append(x)
x.start()
# 等待所有线程完成
for thread in threads:
thread.join()
二、使用多线程监听状态
1、定义需要监听的状态
首先,我们需要定义一个状态,并模拟状态的变化。例如,我们可以定义一个简单的变量来表示状态,并使用一个线程来定期更改这个状态。
import threading
import time
status = "initial"
def change_status():
global status
while True:
status = "changed"
time.sleep(1)
status = "initial"
time.sleep(1)
status_thread = threading.Thread(target=change_status)
status_thread.start()
2、定义监听器
接下来,我们可以定义一个监听器线程,该线程将持续检查状态的变化。
def status_listener():
global status
while True:
if status == "changed":
print("Status has been changed!")
time.sleep(0.5)
listener_thread = threading.Thread(target=status_listener)
listener_thread.start()
通过上述代码,我们创建了一个状态并定期改变它,同时定义了一个监听器线程来实时监听状态的变化。
三、使用异步编程监听状态
1、异步编程简介
异步编程是一种编程范式,通过使用异步操作,可以在等待某些任务完成时继续执行其他任务,从而提高程序的效率。在Python中,异步编程主要通过asyncio
模块来实现。
2、使用asyncio
实现状态监听
asyncio
模块提供了一种异步I/O框架,可以方便地实现异步编程。下面是一个使用asyncio
实现状态监听的示例。
import asyncio
status = "initial"
async def change_status():
global status
while True:
status = "changed"
await asyncio.sleep(1)
status = "initial"
await asyncio.sleep(1)
async def status_listener():
global status
while True:
if status == "changed":
print("Status has been changed!")
await asyncio.sleep(0.5)
async def main():
await asyncio.gather(
change_status(),
status_listener(),
)
asyncio.run(main())
通过上述代码,我们使用asyncio
模块实现了异步状态监听。asyncio
模块通过await
关键字来等待异步操作完成,从而避免了阻塞主线程。
四、轮询实现状态监听
1、什么是轮询
轮询是一种不断检查某个条件是否满足的技术。通过定期检查某个状态,可以实现对状态变化的监听。
2、使用轮询实现状态监听
下面是一个使用轮询实现状态监听的示例。
import time
status = "initial"
def change_status():
global status
while True:
status = "changed"
time.sleep(1)
status = "initial"
time.sleep(1)
def status_listener():
global status
while True:
if status == "changed":
print("Status has been changed!")
time.sleep(0.5)
status_thread = threading.Thread(target=change_status)
status_thread.start()
status_listener()
通过上述代码,我们使用轮询技术实现了状态监听。虽然轮询技术比较简单,但它会消耗大量的CPU资源,不适合高效的状态监听任务。
五、回调函数实现状态监听
1、什么是回调函数
回调函数是一种通过函数指针调用的函数。当某个事件发生时,会调用预先定义的回调函数。回调函数广泛应用于异步编程和事件驱动编程中。
2、使用回调函数实现状态监听
下面是一个使用回调函数实现状态监听的示例。
import threading
import time
status = "initial"
callbacks = []
def register_callback(callback):
callbacks.append(callback)
def change_status():
global status
while True:
status = "changed"
for callback in callbacks:
callback(status)
time.sleep(1)
status = "initial"
for callback in callbacks:
callback(status)
time.sleep(1)
def status_listener(status):
if status == "changed":
print("Status has been changed!")
register_callback(status_listener)
status_thread = threading.Thread(target=change_status)
status_thread.start()
通过上述代码,我们使用回调函数实现了状态监听。当状态发生变化时,会调用预先定义的回调函数,从而实现对状态变化的监听。
六、总结
通过本文,我们介绍了Python中多种实现时刻监听状态的方法,包括多线程编程、异步编程、轮询、回调函数等技术。每种技术都有其优缺点,开发者可以根据具体需求选择合适的技术来实现时刻监听状态。
多线程编程能够充分利用多核CPU的优势,提高程序的并发性能,但需要注意线程同步和资源竞争问题。异步编程通过非阻塞的方式实现高效的I/O操作,适用于大量I/O密集型任务。轮询技术实现简单,但会消耗大量的CPU资源,适合简单的状态监听任务。回调函数广泛应用于异步编程和事件驱动编程,能够实现灵活的事件处理机制。
在实际开发中,可以结合多种技术来实现复杂的状态监听任务,从而提高程序的性能和效率。
相关问答FAQs:
如何在Python中实现状态监听的功能?
在Python中,可以使用多种方法来实现状态监听的功能。常见的方式是使用观察者模式,这种模式允许一个对象(被观察者)通知多个观察者对象其状态的变化。此外,可以利用回调函数、事件驱动框架(如asyncio
)或多线程来实现状态监听。选择合适的方法取决于具体的应用场景和需求。
如何监控外部系统的状态变化?
如果需要监控外部系统的状态变化,可以使用API调用、定时任务(如schedule
库或cron
作业)或Webhooks。通过定期发送请求或接收来自外部系统的通知,可以有效地掌握其状态变化。这种方式适用于需要与外部服务或设备集成的情况。
在Python中如何处理状态变化通知?
处理状态变化通知可以通过实现回调函数来完成。当状态发生变化时,被观察者会调用这些回调函数,传递新的状态信息。可以使用functools
库中的partial
函数来简化回调函数的参数传递。此外,利用事件循环(如asyncio
)可以实现更高效的异步状态处理,特别是在需要处理大量并发请求时。
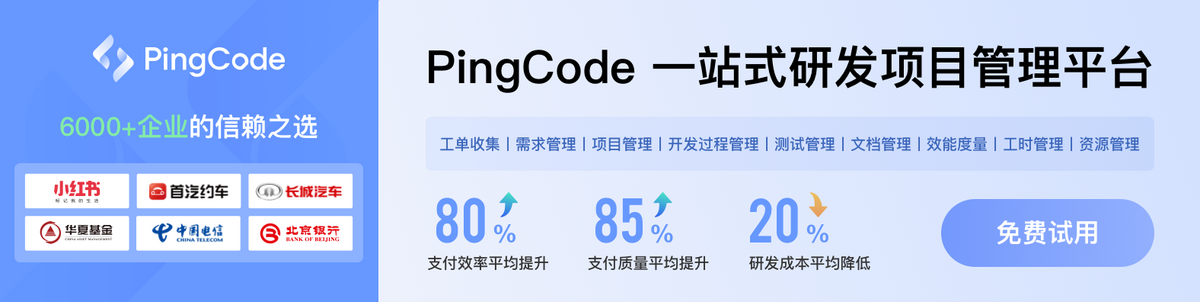