Python可以通过多种方式打包给Java调用,包括使用Py4J、Jython、RESTful API、gRPC等。 其中,使用Py4J 是一种常见且便捷的方法。Py4J是一个让Python程序可以与Java程序互操作的库。它允许Java程序调用Python代码,并且能够通过网络协议进行通信。
详细描述:
Py4J 是一个开源的库,提供了在Java虚拟机(JVM)中调用Python代码的功能。它通过在Python和Java之间创建一个网关来实现这一点。这个网关可以在Java代码中创建,并通过它可以调用Python的函数和对象。这样,Java代码可以像调用本地方法一样调用Python代码,极大地简化了跨语言的调用过程。
下面将详细讲解几种不同的方式,并提供相应的示例代码和注意事项。
一、使用Py4J
安装Py4J
要使用Py4J,首先需要安装这个库。可以通过pip进行安装:
pip install py4j
Python代码
在Python中,我们需要创建一个可以被Java调用的类,并启动一个Py4J网关:
from py4j.java_gateway import JavaGateway, GatewayServer
class PythonClass:
def say_hello(self, name):
return f"Hello, {name}!"
if __name__ == "__main__":
gateway = GatewayServer(port=25333)
gateway.start()
python_class = PythonClass()
gateway.java_gateway_server.entry_point.setPythonClass(python_class)
input("Press any key to exit\n")
gateway.shutdown()
Java代码
在Java中,我们通过Py4J网关来调用Python代码:
import py4j.GatewayServer;
public class JavaClass {
private PythonClass pythonClass;
public void setPythonClass(PythonClass pythonClass) {
this.pythonClass = pythonClass;
}
public static void main(String[] args) {
JavaClass app = new JavaClass();
GatewayServer server = new GatewayServer(app);
server.start();
System.out.println("Gateway Server Started");
String result = app.pythonClass.sayHello("Java");
System.out.println(result);
server.shutdown();
}
}
运行
- 启动Python代码,它将启动一个Py4J网关服务器。
- 启动Java代码,它将连接到Py4J网关并调用Python代码。
注意事项
- 确保Python和Java代码使用相同的端口号。
- Py4J的性能取决于网络通信的速度,因此在高频调用场景下,可能需要考虑性能优化。
二、使用Jython
安装Jython
Jython是Python语言的Java实现,允许在Java平台上直接运行Python代码。可以从Jython的官方网站下载并安装Jython。
使用Jython运行Python代码
通过Jython,可以直接在Java代码中嵌入Python代码,或者调用Python脚本:
import org.python.util.PythonInterpreter;
public class JavaClass {
public static void main(String[] args) {
PythonInterpreter interpreter = new PythonInterpreter();
interpreter.exec("print('Hello from Python')");
interpreter.exec("def say_hello(name): return f'Hello, {name}!'");
String result = interpreter.eval("repr(say_hello('Java'))").toString();
System.out.println(result);
}
}
注意事项
- Jython不支持所有的Python库,特别是那些依赖于C扩展的库。
- Jython的性能可能不如原生Python,因此在性能敏感的场景中需要进行测试和优化。
三、使用RESTful API
创建Python RESTful API
通过使用Flask或FastAPI等框架,可以创建一个Python RESTful API,供Java代码调用:
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/say_hello', methods=['GET'])
def say_hello():
name = request.args.get('name')
return jsonify({'message': f'Hello, {name}!'})
if __name__ == "__main__":
app.run(port=5000)
Java代码调用RESTful API
在Java中,可以使用HttpURLConnection或第三方库(如Apache HttpClient)来调用Python RESTful API:
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class JavaClass {
public static void main(String[] args) throws Exception {
String url = "http://localhost:5000/say_hello?name=Java";
HttpURLConnection conn = (HttpURLConnection) new URL(url).openConnection();
conn.setRequestMethod("GET");
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
StringBuilder content = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
content.append(inputLine);
}
in.close();
conn.disconnect();
System.out.println(content.toString());
}
}
注意事项
- RESTful API的性能取决于网络通信的速度。
- 需要处理API的安全性和认证机制。
四、使用gRPC
创建Python gRPC服务
gRPC是一种高性能、开源的远程过程调用(RPC)框架,可以用于跨语言调用。首先需要定义.proto文件,并生成Python和Java代码:
syntax = "proto3";
service HelloService {
rpc SayHello (HelloRequest) returns (HelloResponse);
}
message HelloRequest {
string name = 1;
}
message HelloResponse {
string message = 1;
}
Python代码
生成Python代码后,创建gRPC服务:
from concurrent import futures
import grpc
import hello_pb2
import hello_pb2_grpc
class HelloService(hello_pb2_grpc.HelloServiceServicer):
def SayHello(self, request, context):
return hello_pb2.HelloResponse(message=f"Hello, {request.name}!")
def serve():
server = grpc.server(futures.ThreadPoolExecutor(max_workers=10))
hello_pb2_grpc.add_HelloServiceServicer_to_server(HelloService(), server)
server.add_insecure_port('[::]:50051')
server.start()
server.wait_for_termination()
if __name__ == "__main__":
serve()
Java代码
生成Java代码后,创建gRPC客户端:
import io.grpc.ManagedChannel;
import io.grpc.ManagedChannelBuilder;
import io.grpc.helloworld.HelloServiceGrpc;
import io.grpc.helloworld.HelloRequest;
import io.grpc.helloworld.HelloResponse;
public class JavaClass {
public static void main(String[] args) {
ManagedChannel channel = ManagedChannelBuilder.forAddress("localhost", 50051)
.usePlaintext()
.build();
HelloServiceGrpc.HelloServiceBlockingStub stub = HelloServiceGrpc.newBlockingStub(channel);
HelloResponse response = stub.sayHello(HelloRequest.newBuilder()
.setName("Java")
.build());
System.out.println(response.getMessage());
channel.shutdown();
}
}
注意事项
- gRPC的性能较高,但需要处理网络通信的安全性。
- 需要注意gRPC的版本兼容性。
五、其他方法
使用JNI(Java Native Interface)
JNI允许Java代码调用本地代码(如C/C++编写的代码),但也可以用于调用Python代码。需要通过JNI创建一个C/C++中间层,来调用Python代码。这个方法较为复杂,不常用。
使用消息队列
可以使用消息队列(如RabbitMQ、Kafka)来实现Java和Python代码之间的异步通信。Java代码发送消息到队列,Python代码从队列中读取消息并处理,然后将结果发送回队列。消息队列适用于需要解耦和异步处理的场景。
使用数据库
可以将数据存储在数据库中,Java代码将数据写入数据库,Python代码从数据库中读取数据并处理,然后将结果写回数据库。这种方法适用于需要持久化数据和跨语言处理的场景。
注意事项
- 选择合适的跨语言调用方法取决于具体的应用场景和需求。
- 需要考虑性能、安全性、可维护性等因素。
- 在跨语言调用过程中,需要处理数据的序列化和反序列化,以及数据类型的转换。
总结
通过上述几种方法,可以实现Python代码打包给Java调用。每种方法都有其优缺点和适用场景。在实际应用中,可以根据具体需求选择合适的方法。Py4J、Jython、RESTful API、gRPC 是常见的几种方法,其中Py4J和RESTful API较为简单易用,而gRPC则提供了更高的性能和灵活性。希望通过本文的介绍,能够帮助读者更好地理解和实现Python代码与Java代码的互操作。
相关问答FAQs:
1. 如何将Python代码打包成Java可以调用的格式?
要将Python代码打包成Java可以调用的格式,可以使用一些工具和库,例如Jython或Py4J。Jython是一种Java平台上的Python实现,可以直接在Java中调用Python代码。而Py4J则允许Python程序与Java虚拟机进行通信,使得Java可以调用Python对象和方法。选择合适的工具后,您可以将Python代码封装为一个可调用的接口,并在Java中通过相应的API进行调用。
2. 打包后的Python库在Java中如何使用?
一旦您将Python代码打包后,可以在Java项目中通过添加相关的库依赖来使用。例如,如果使用Py4J,可以在Java代码中创建一个GatewayServer对象,连接到Python程序,并通过该对象调用Python方法。确保在运行Java程序之前,Python环境已正确设置并且相关服务正在运行。
3. 在打包过程中需要注意哪些问题?
在打包Python代码以供Java调用时,需注意几个关键点。首先,确保Python代码与Java数据类型的兼容性,例如,如何在Python和Java之间转换数据类型。其次,处理Python中的异常并确保它们能够被Java代码捕获。此外,性能也是一个需要考虑的问题,尤其是在高频率调用的场景下,可能需要优化Python代码的执行效率,以减少延迟和资源消耗。
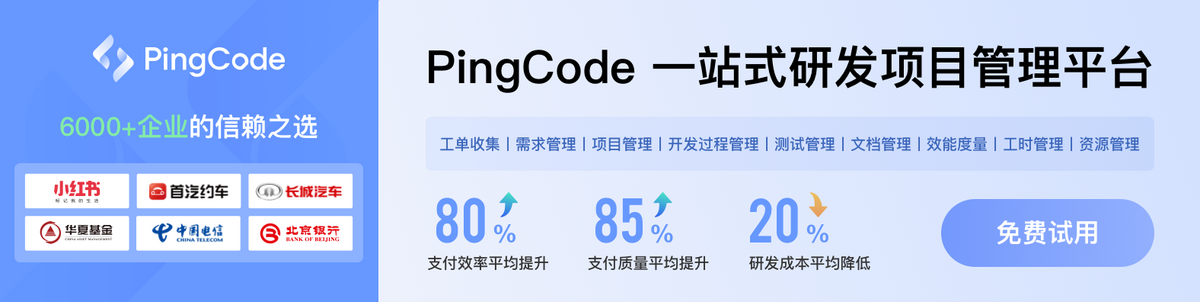