使用Python制作动图可以通过多种方法实现,常见的工具有matplotlib、Pillow、imageio、manim等。每种工具都有其独特的优势和适用场景。 其中,matplotlib 是一个强大的绘图库,适合用来生成数据驱动的动画;Pillow 则是一个图像处理库,适用于处理和合成图像;imageio 是一个灵活的图像与视频处理库,适合于创建和编辑动图;manim 是一个动画引擎,适用于生成高质量的数学动画。下面将详细介绍如何使用matplotlib制作动图。
一、MATPLOTLIB制作动图
matplotlib 是Python中一个非常强大的绘图库,支持绘制静态和动态图像。matplotlib.animation 模块提供了创建动画的功能。
1、安装matplotlib
首先确保你已经安装了matplotlib,可以通过以下命令安装:
pip install matplotlib
2、基础动画实现
下面是一个简单的例子,演示如何使用matplotlib制作一个简单的动态正弦波动画。
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.animation as animation
创建一个图和一个子图
fig, ax = plt.subplots()
设置x轴和y轴的范围
ax.set_xlim((0, 2 * np.pi))
ax.set_ylim((-1, 1))
初始化一条空线
line, = ax.plot([], [], lw=2)
初始化函数,用于初始化背景
def init():
line.set_data([], [])
return (line,)
动画函数,用于更新每一帧
def animate(i):
x = np.linspace(0, 2 * np.pi, 1000)
y = np.sin(x - 0.1 * i)
line.set_data(x, y)
return (line,)
创建动画对象
ani = animation.FuncAnimation(fig, animate, init_func=init, frames=200, interval=20, blit=True)
保存动画
ani.save('sine_wave.gif', writer='imagemagick')
plt.show()
在这个例子中,我们创建了一条动态正弦波。首先设置图形的范围,然后初始化一条空线。init
函数用于初始化背景,animate
函数用于更新每一帧的数据。FuncAnimation
函数将这两个函数结合起来,生成一个动画对象。最后,我们将动画保存为一个GIF文件。
3、复杂动画实现
为了展示更复杂的动画,我们可以考虑一个动态的散点图,其中点的颜色和大小也会变化。
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.animation as animation
fig, ax = plt.subplots()
x = np.random.rand(100)
y = np.random.rand(100)
colors = np.random.rand(100)
area = (30 * np.random.rand(100))2
scatter = ax.scatter(x, y, s=area, c=colors, alpha=0.5)
def init():
scatter.set_offsets(np.c_[x, y])
return scatter,
def animate(i):
x = np.random.rand(100)
y = np.random.rand(100)
scatter.set_offsets(np.c_[x, y])
return scatter,
ani = animation.FuncAnimation(fig, animate, init_func=init, frames=50, interval=200, blit=True)
ani.save('scatter.gif', writer='imagemagick')
plt.show()
在这个例子中,我们生成了一个动态的散点图,每一帧都会更新点的位置。通过设置点的颜色和大小,动画会显得更加生动。
二、PILLOW制作动图
Pillow 是Python Imaging Library(PIL)的一个分支,提供了丰富的图像处理功能。我们可以使用Pillow来创建和处理GIF动图。
1、安装Pillow
首先确保你已经安装了Pillow,可以通过以下命令安装:
pip install pillow
2、创建简单动图
下面是一个简单的例子,演示如何使用Pillow创建一个简单的GIF动图。
from PIL import Image, ImageDraw
frames = []
for i in range(20):
frame = Image.new('RGB', (100, 100), (255, 255, 255))
draw = ImageDraw.Draw(frame)
draw.ellipse((i*5, i*5, 100-i*5, 100-i*5), fill=(255, 0, 0))
frames.append(frame)
frames[0].save('circle.gif', save_all=True, append_images=frames[1:], loop=0, duration=100)
在这个例子中,我们创建了一个简单的动图,其中一个红色的圆圈从小变大。每一帧都是一个独立的图像,我们将这些图像组合成一个GIF文件。
3、复杂动图实现
为了展示更复杂的动图,我们可以考虑一个逐帧绘制的例子,其中图像的内容会随着时间变化。
from PIL import Image, ImageDraw
import numpy as np
frames = []
for i in range(20):
frame = Image.new('RGB', (200, 200), (255, 255, 255))
draw = ImageDraw.Draw(frame)
x = np.linspace(0, 200, 1000)
y = 100 + 80 * np.sin(x * 0.1 + i * 0.1)
for j in range(len(x)-1):
draw.line((x[j], y[j], x[j+1], y[j+1]), fill=(0, 0, 255), width=2)
frames.append(frame)
frames[0].save('wave.gif', save_all=True, append_images=frames[1:], loop=0, duration=100)
在这个例子中,我们创建了一个动图,其中一条正弦波随着时间变化。每一帧都是一个独立的图像,我们将这些图像组合成一个GIF文件。
三、IMAGEIO制作动图
imageio 是一个灵活的图像与视频处理库,支持多种格式的读写操作。我们可以使用imageio来创建和处理GIF动图。
1、安装imageio
首先确保你已经安装了imageio,可以通过以下命令安装:
pip install imageio
2、创建简单动图
下面是一个简单的例子,演示如何使用imageio创建一个简单的GIF动图。
import imageio
import numpy as np
import matplotlib.pyplot as plt
images = []
for i in range(20):
fig, ax = plt.subplots()
x = np.linspace(0, 2 * np.pi, 1000)
y = np.sin(x - 0.1 * i)
ax.plot(x, y)
fig.canvas.draw()
image = np.frombuffer(fig.canvas.tostring_rgb(), dtype='uint8')
image = image.reshape(fig.canvas.get_width_height()[::-1] + (3,))
images.append(image)
plt.close(fig)
imageio.mimsave('sine_wave.gif', images, duration=0.1)
在这个例子中,我们使用matplotlib生成了一系列图像,然后使用imageio将这些图像组合成一个GIF文件。
3、复杂动图实现
为了展示更复杂的动图,我们可以考虑一个逐帧绘制的例子,其中图像的内容会随着时间变化。
import imageio
from PIL import Image, ImageDraw
import numpy as np
images = []
for i in range(20):
frame = Image.new('RGB', (200, 200), (255, 255, 255))
draw = ImageDraw.Draw(frame)
x = np.linspace(0, 200, 1000)
y = 100 + 80 * np.sin(x * 0.1 + i * 0.1)
for j in range(len(x)-1):
draw.line((x[j], y[j], x[j+1], y[j+1]), fill=(0, 0, 255), width=2)
images.append(np.array(frame))
imageio.mimsave('wave.gif', images, duration=0.1)
在这个例子中,我们使用Pillow生成了一系列图像,然后使用imageio将这些图像组合成一个GIF文件。
四、MANIM制作动图
manim 是一个动画引擎,适用于生成高质量的数学动画。它提供了丰富的功能,可以帮助我们创建复杂的动画。
1、安装manim
首先确保你已经安装了manim,可以通过以下命令安装:
pip install manim
2、创建简单动画
下面是一个简单的例子,演示如何使用manim创建一个简单的动画。
from manim import *
class SquareToCircle(Scene):
def construct(self):
square = Square()
self.play(Create(square))
self.play(Transform(square, Circle()))
self.play(FadeOut(square))
if __name__ == "__main__":
scene = SquareToCircle()
scene.render()
在这个例子中,我们创建了一个简单的动画,其中一个正方形变成了一个圆,然后消失。SquareToCircle
类继承自Scene
类,我们在construct
方法中定义动画的内容。
3、复杂动画实现
为了展示更复杂的动画,我们可以考虑一个包含多种动画效果的例子。
from manim import *
class ComplexAnimation(Scene):
def construct(self):
circle = Circle()
square = Square()
self.play(Create(circle))
self.play(circle.animate.shift(LEFT))
self.play(Transform(circle, square))
self.play(square.animate.shift(RIGHT))
triangle = Triangle()
self.play(ReplacementTransform(square, triangle))
self.play(FadeOut(triangle))
if __name__ == "__main__":
scene = ComplexAnimation()
scene.render()
在这个例子中,我们创建了一个复杂的动画,其中一个圆形变成了一个正方形,然后变成了一个三角形,最后消失。通过组合多个动画效果,我们可以创建更加生动的动画。
总结
通过以上的介绍,我们了解了如何使用Python的四种常见工具:matplotlib、Pillow、imageio、manim 来制作动图。matplotlib 适合生成数据驱动的动画,Pillow 适用于处理和合成图像,imageio 是一个灵活的图像与视频处理库,而manim 则适用于生成高质量的数学动画。通过选择合适的工具,我们可以根据需求制作出丰富多彩的动图,提高我们的数据可视化和演示效果。
相关问答FAQs:
如何选择适合制作动图的Python库?
在Python中,有几个流行的库可以用于制作动图。其中,Pillow是一个强大的图像处理库,适用于简单的动图制作;Matplotlib则适合用于数据可视化动图;而MoviePy则可以处理视频编辑,制作更复杂的动图。根据需求选择合适的库,可以提高制作效率和效果。
制作动图时需要注意哪些关键参数?
在制作动图时,帧率、分辨率和颜色深度是非常重要的参数。帧率决定了动图的流畅度,通常在10到30帧每秒之间选择最佳值。分辨率影响动图的清晰度,过高的分辨率可能导致文件过大,而过低则会影响视觉效果。颜色深度则会影响动图的颜色表现,选择合适的颜色模式可以提升动图的视觉吸引力。
动图制作完成后,如何优化文件大小?
动图的文件大小往往较大,因此优化是必要的。可以通过减少帧数、降低分辨率、使用合适的颜色调色板以及采用压缩算法来优化文件大小。此外,使用GIF优化工具,如Gifsicle,可以进一步减少文件的体积,确保动图在网页或社交媒体上加载更快。
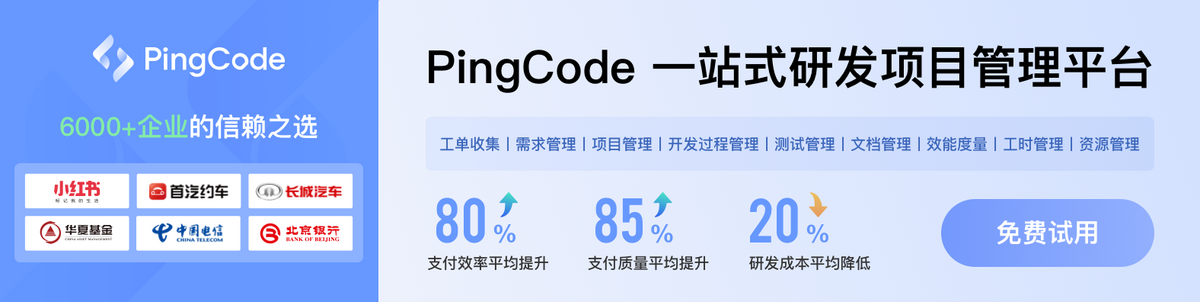