使用Python制作词云的主要步骤包括安装相关库、准备文本数据、生成词云图像、调整词云外观和保存结果等。 在这些步骤中,最关键的部分是生成词云图像,通常使用WordCloud库。以下是具体步骤的详细介绍:
一、安装相关库
在制作词云之前,需要安装一些必需的Python库。主要包括wordcloud
、matplotlib
和numpy
。可以通过以下命令来安装这些库:
pip install wordcloud matplotlib numpy
二、准备文本数据
准备文本数据是制作词云的基础。可以从各种来源获取文本数据,例如文件、网页或API。为了简单起见,我们可以使用一个简单的文本字符串作为示例:
text = "Python is a powerful programming language. It is widely used in data science, machine learning, web development, and more."
三、生成词云图像
使用WordCloud库生成词云图像是制作词云的核心步骤。以下是生成词云的基本代码:
from wordcloud import WordCloud
import matplotlib.pyplot as plt
创建词云对象
wordcloud = WordCloud(width=800, height=400, background_color='white').generate(text)
显示词云图像
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
四、调整词云外观
为了使词云更具吸引力和个性化,可以调整词云的外观。例如,可以更改字体、颜色、形状等。以下是一些常见的调整方法:
- 更改字体:可以通过指定
font_path
参数来更改词云的字体。 - 调整颜色:可以通过自定义颜色函数来调整词云的颜色。
- 更改形状:可以使用
mask
参数来更改词云的形状,使其匹配特定的图像轮廓。
# 更改字体
wordcloud = WordCloud(width=800, height=400, background_color='white', font_path='path/to/font.ttf').generate(text)
自定义颜色函数
def custom_color_func(word, font_size, position, orientation, random_state=None, kwargs):
return "hsl(210, 100%%, %d%%)" % random.randint(40, 80)
wordcloud = WordCloud(width=800, height=400, background_color='white', color_func=custom_color_func).generate(text)
使用遮罩图像来更改形状
from PIL import Image
import numpy as np
mask = np.array(Image.open('path/to/mask_image.png'))
wordcloud = WordCloud(width=800, height=400, background_color='white', mask=mask).generate(text)
五、保存结果
最后,可以将生成的词云图像保存到文件中,以便于后续使用或分享。可以使用WordCloud
对象的to_file
方法来保存图像:
# 保存词云图像
wordcloud.to_file('wordcloud.png')
通过以上步骤,您可以使用Python制作出个性化的词云图像。以下是更详细的介绍每一个步骤中的具体内容和技巧。
一、安装相关库
在安装库时,除了wordcloud
、matplotlib
和numpy
外,可能还需要安装其他一些库来处理特定的需求。例如:
pip install pillow # 用于图像处理
pip install jieba # 用于中文文本分词
安装完成后,可以导入这些库来进行词云制作。
二、准备文本数据
文本数据的准备工作非常重要,因为它直接影响词云的效果。可以通过以下几种方式获取文本数据:
1、从文件读取文本
with open('path/to/textfile.txt', 'r', encoding='utf-8') as file:
text = file.read()
2、从网页抓取文本
使用requests
和beautifulsoup4
库从网页抓取文本数据:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
text = soup.get_text()
3、从API获取文本
使用API获取文本数据,例如从Twitter API获取推文内容:
import tweepy
设置API密钥和令牌
api_key = 'your_api_key'
api_key_secret = 'your_api_key_secret'
access_token = 'your_access_token'
access_token_secret = 'your_access_token_secret'
认证API
auth = tweepy.OAuth1UserHandler(api_key, api_key_secret, access_token, access_token_secret)
api = tweepy.API(auth)
获取推文内容
tweets = api.user_timeline(screen_name='username', count=100)
text = ' '.join([tweet.text for tweet in tweets])
三、生成词云图像
生成词云图像时,可以根据具体需求调整WordCloud
对象的参数。以下是一些常见参数的介绍:
1、width和height
设置词云图像的宽度和高度:
wordcloud = WordCloud(width=800, height=400).generate(text)
2、background_color
设置词云图像的背景颜色:
wordcloud = WordCloud(background_color='white').generate(text)
3、max_words
设置词云图像中显示的最大单词数:
wordcloud = WordCloud(max_words=200).generate(text)
4、stopwords
设置词云图像中需要排除的停用词:
stopwords = set(['is', 'in', 'and', 'the', 'of'])
wordcloud = WordCloud(stopwords=stopwords).generate(text)
四、调整词云外观
在生成基本的词云图像后,可以通过以下方式进一步调整其外观,使其更加美观和个性化。
1、更改字体
使用自定义字体:
wordcloud = WordCloud(font_path='path/to/font.ttf').generate(text)
2、调整颜色
使用自定义颜色函数:
import random
def custom_color_func(word, font_size, position, orientation, random_state=None, kwargs):
return "hsl(210, 100%%, %d%%)" % random.randint(40, 80)
wordcloud = WordCloud(color_func=custom_color_func).generate(text)
3、更改形状
使用遮罩图像来更改词云的形状:
from PIL import Image
import numpy as np
mask = np.array(Image.open('path/to/mask_image.png'))
wordcloud = WordCloud(mask=mask).generate(text)
4、更多参数调整
可以结合多个参数进行调整,以达到最佳效果:
wordcloud = WordCloud(
width=800,
height=400,
background_color='white',
max_words=200,
stopwords=stopwords,
font_path='path/to/font.ttf',
mask=mask,
color_func=custom_color_func
).generate(text)
五、保存结果
生成词云图像后,可以将其保存为文件,以便于后续使用或分享:
wordcloud.to_file('wordcloud.png')
此外,还可以将词云图像嵌入到网页中,方便在线展示。例如,可以将词云图像保存为HTML文件:
from wordcloud import WordCloud
html = wordcloud.to_html()
with open('wordcloud.html', 'w', encoding='utf-8') as file:
file.write(html)
通过以上步骤,您可以使用Python制作出个性化的词云图像。以下是一个完整的示例代码,包括所有步骤:
import requests
from bs4 import BeautifulSoup
from wordcloud import WordCloud
import matplotlib.pyplot as plt
from PIL import Image
import numpy as np
import random
获取文本数据
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
text = soup.get_text()
设置停用词
stopwords = set(['is', 'in', 'and', 'the', 'of'])
自定义颜色函数
def custom_color_func(word, font_size, position, orientation, random_state=None, kwargs):
return "hsl(210, 100%%, %d%%)" % random.randint(40, 80)
使用遮罩图像
mask = np.array(Image.open('path/to/mask_image.png'))
创建词云对象
wordcloud = WordCloud(
width=800,
height=400,
background_color='white',
max_words=200,
stopwords=stopwords,
font_path='path/to/font.ttf',
mask=mask,
color_func=custom_color_func
).generate(text)
显示词云图像
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
保存词云图像
wordcloud.to_file('wordcloud.png')
通过以上完整示例代码,可以看到如何从网页抓取文本数据、设置停用词、自定义颜色函数、使用遮罩图像生成词云,并最终显示和保存词云图像。希望这个详细的介绍能帮助您更好地理解和使用Python制作词云。
相关问答FAQs:
如何选择合适的文本数据来制作词云?
制作词云的第一步是选择合适的文本数据。你可以使用文章、社交媒体评论、书籍或任何其他文本资料。确保数据能够代表你想要表达的主题或情感。同时,清洗数据以去除无关信息,例如标点符号、停用词等,这样有助于提高词云的质量。
制作词云时有哪些常用的Python库?
在Python中,有几个常用的库可以帮助你制作词云。最常用的是wordcloud
库,它提供了简单易用的接口生成高质量的词云。此外,matplotlib
库可以用于可视化词云,pandas
库则可以处理和分析文本数据。结合这些库,你可以轻松创建出美观的词云。
如何自定义词云的外观和形状?
自定义词云的外观和形状可以通过设置参数来实现。例如,你可以更改词云的颜色、字体、背景色,甚至使用自定义的形状掩膜来决定词云的轮廓。通过调整WordCloud
类中的参数,如width
、height
、background_color
和mask
等,可以让你的词云更加个性化,符合你的项目需求。
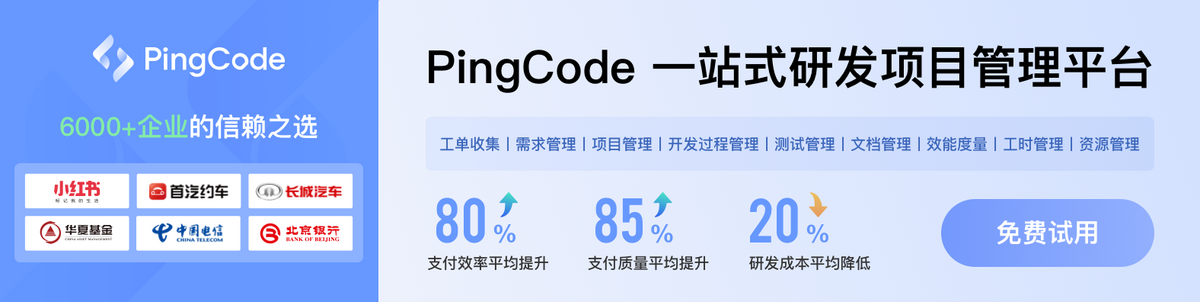