在Python中,判断数据非空的方法有多种,具体取决于数据的类型。常见的数据类型包括字符串、列表、元组、字典等。一般而言,可以使用以下几种方法来判断数据是否非空:使用if语句、len()函数、not运算符、is None判断。其中,使用if语句是最常见和直观的方法。
例如,对于字符串、列表、字典等数据类型,可以通过if语句直接判断数据是否非空:
data = "example"
if data:
print("Data is not empty")
else:
print("Data is empty")
这种方法适用于大多数数据类型,而且代码简洁易读。接下来将详细描述其他几种方法。
一、使用if语句
1、字符串判断非空
对于字符串,可以直接使用if语句来判断是否非空。空字符串会被视为False,非空字符串会被视为True。
string = "Hello, World!"
if string:
print("String is not empty")
else:
print("String is empty")
2、列表判断非空
同样地,对于列表、元组等序列类型,也可以直接使用if语句来判断是否非空。
lst = [1, 2, 3]
if lst:
print("List is not empty")
else:
print("List is empty")
3、字典判断非空
对于字典类型,使用if语句判断是否非空同样适用。
dictionary = {"key": "value"}
if dictionary:
print("Dictionary is not empty")
else:
print("Dictionary is empty")
二、使用len()函数
1、字符串长度判断
使用len()函数可以获取数据的长度,长度为0即为空。
string = "Hello, World!"
if len(string) > 0:
print("String is not empty")
else:
print("String is empty")
2、列表长度判断
对于列表,可以使用len()函数判断长度是否大于0。
lst = [1, 2, 3]
if len(lst) > 0:
print("List is not empty")
else:
print("List is empty")
3、字典长度判断
同样,字典也可以通过len()函数来判断是否非空。
dictionary = {"key": "value"}
if len(dictionary) > 0:
print("Dictionary is not empty")
else:
print("Dictionary is empty")
三、使用not运算符
1、字符串非空判断
not运算符可以反转布尔值,适用于判断数据是否为空。
string = "Hello, World!"
if not not string:
print("String is not empty")
else:
print("String is empty")
2、列表非空判断
对于列表,not运算符同样适用。
lst = [1, 2, 3]
if not not lst:
print("List is not empty")
else:
print("List is empty")
3、字典非空判断
对于字典,使用not运算符来判断是否非空。
dictionary = {"key": "value"}
if not not dictionary:
print("Dictionary is not empty")
else:
print("Dictionary is empty")
四、is None判断
1、判断变量是否为None
有时我们需要判断一个变量是否为None。None是Python中的一个特殊类型,表示空值或无值。
value = None
if value is not None:
print("Value is not None")
else:
print("Value is None")
2、适用于函数返回值判断
当函数可能返回None时,使用is None判断可以避免错误。
def get_value():
return None
value = get_value()
if value is not None:
print("Function returned a value")
else:
print("Function returned None")
3、结合其他条件判断
有时需要结合其他条件进行判断,此时可以使用is None判断。
value = None
if value is not None and isinstance(value, int):
print("Value is an integer and not None")
else:
print("Value is either None or not an integer")
五、综合使用
1、综合判断字符串、列表、字典非空
在实际开发中,我们可能需要判断多个数据类型是否非空,可以综合使用上述方法。
string = "Hello, World!"
lst = [1, 2, 3]
dictionary = {"key": "value"}
if string and len(lst) > 0 and dictionary:
print("All data structures are not empty")
else:
print("One or more data structures are empty")
2、函数封装非空判断
为了提高代码的复用性,可以将非空判断封装成函数。
def is_not_empty(data):
if data:
return True
return False
string = "Hello, World!"
lst = [1, 2, 3]
dictionary = {"key": "value"}
if is_not_empty(string) and is_not_empty(lst) and is_not_empty(dictionary):
print("All data structures are not empty")
else:
print("One or more data structures are empty")
3、适用于多种数据类型的判断
函数封装后,可以适用于多种数据类型的非空判断,提高代码的通用性。
def is_not_empty(data):
if data:
return True
return False
data_list = ["Hello, World!", [1, 2, 3], {"key": "value"}, 123, 0.0, None]
for data in data_list:
if is_not_empty(data):
print(f"{data} is not empty")
else:
print(f"{data} is empty or None")
六、实际应用中的非空判断
1、读取文件内容判断非空
在读取文件内容时,我们需要判断文件是否为空。
with open("example.txt", "r") as file:
content = file.read()
if content:
print("File is not empty")
else:
print("File is empty")
2、数据库查询结果判断非空
在处理数据库查询结果时,判断查询结果是否为空非常重要。
import sqlite3
conn = sqlite3.connect("example.db")
cursor = conn.cursor()
cursor.execute("SELECT * FROM users")
rows = cursor.fetchall()
if rows:
print("Query returned results")
else:
print("Query returned no results")
3、API请求返回数据判断非空
在处理API请求返回的数据时,判断返回数据是否为空可以避免错误。
import requests
response = requests.get("https://api.example.com/data")
data = response.json()
if data:
print("API returned data")
else:
print("API returned no data")
4、表单输入数据判断非空
在处理用户表单输入时,判断输入数据是否为空是常见需求。
form_data = {"username": "user123", "password": "pass123"}
if form_data["username"] and form_data["password"]:
print("Form data is complete")
else:
print("Form data is incomplete")
七、特殊情况下的非空判断
1、空白字符判断
有时需要判断字符串是否仅包含空白字符。
string = " "
if string.strip():
print("String is not empty or only whitespace")
else:
print("String is empty or only whitespace")
2、None与空字符串的区别
需要区分None与空字符串时,可以结合is None判断。
value = ""
if value is None:
print("Value is None")
elif value == "":
print("Value is an empty string")
else:
print("Value is not None or empty")
3、嵌套数据结构判断
对于嵌套的数据结构,可以递归判断是否非空。
def is_not_empty_recursive(data):
if isinstance(data, (list, tuple, set)):
return any(is_not_empty_recursive(item) for item in data)
elif isinstance(data, dict):
return any(is_not_empty_recursive(value) for value in data.values())
else:
return bool(data)
nested_data = {"key1": {"subkey": []}, "key2": {"subkey": "value"}}
if is_not_empty_recursive(nested_data):
print("Nested data is not empty")
else:
print("Nested data is empty")
4、结合异常处理判断
在处理可能抛出异常的代码时,可以结合异常处理进行非空判断。
try:
value = int("123")
except ValueError:
value = None
if value is not None:
print("Value is a valid integer")
else:
print("Value is None or invalid")
八、总结
在Python中,判断数据非空的方法非常多样化,根据具体的使用场景选择合适的方法可以提高代码的可读性和健壮性。使用if语句、len()函数、not运算符、is None判断是最常用的方法。对于复杂的情况,可以结合多种方法进行综合判断,甚至封装成函数以提高代码的复用性和通用性。在实际应用中,无论是处理文件、数据库、API请求还是用户输入,都需要进行非空判断以确保程序的正确性和稳定性。通过掌握这些方法,可以更好地编写健壮和高效的Python代码。
相关问答FAQs:
如何在Python中判断列表是否为空?
在Python中,可以使用if not list_name:
语句来判断一个列表是否为空。如果列表为空,条件将返回True。例如:
my_list = []
if not my_list:
print("列表为空")
这种方法简洁明了,适用于各种类型的可迭代对象。
如何检查字符串是否为空或仅包含空格?
使用Python的strip()
方法可以去除字符串前后的空格,从而判断字符串是否为空或仅含空格。可以通过以下代码实现:
my_string = " "
if not my_string.strip():
print("字符串为空或仅包含空格")
这种方法能有效过滤掉仅由空格组成的字符串。
在Python中,如何判断字典是否为空?
判断字典是否为空可以使用if not dict_name:
语句。示例代码如下:
my_dict = {}
if not my_dict:
print("字典为空")
这种方式适用于所有类型的字典,并且能够快速确认字典的状态。
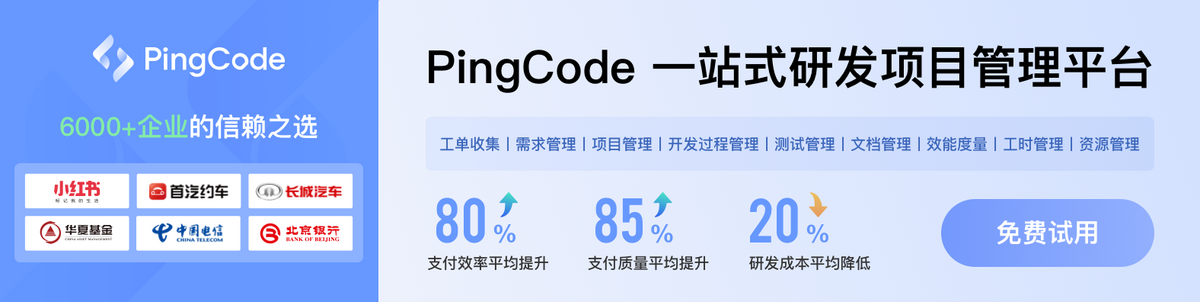