要在Python中循环查找某个文本,可以使用多种方法来实现,比如使用内置的字符串方法、正则表达式库等。以下是一些有效的方法:使用for循环、使用while循环、使用正则表达式。其中,使用正则表达式是一种强大且灵活的方法,可以用来查找复杂模式的文本。下面详细介绍如何使用正则表达式来循环查找某个文本。
在Python中,re
模块提供了正则表达式匹配操作。通过使用re.finditer()
,我们可以在循环中逐一查找所有匹配的文本。以下是一个示例代码:
import re
def find_all_occurrences(text, pattern):
matches = re.finditer(pattern, text)
for match in matches:
print(f"Found {match.group()} at {match.start()}-{match.end()}")
示例文本和查找模式
text = "Python is amazing. Python is versatile. Python is easy to learn."
pattern = r"Python"
find_all_occurrences(text, pattern)
上面的代码使用re.finditer()
来查找所有的pattern
匹配,并在循环中打印每个匹配的文本及其位置。通过这种方法,可以灵活地处理复杂的文本查找任务。
接下来,我们将详细探讨不同的方法,并提供实际的代码示例。
一、使用for循环
在Python中,可以使用for循环来遍历字符串并查找某个特定的文本。以下是实现此方法的详细步骤和示例代码:
1、直接遍历字符串
可以通过直接遍历字符串的每一个字符来查找特定文本。
def find_text_with_for(text, target):
target_len = len(target)
for i in range(len(text) - target_len + 1):
if text[i:i + target_len] == target:
print(f"Found '{target}' at position {i}")
示例
text = "Python is amazing. Python is versatile. Python is easy to learn."
target = "Python"
find_text_with_for(text, target)
在这个示例中,我们通过for循环遍历字符串的每个字符,并检查从当前位置开始的子字符串是否与目标文本匹配。如果匹配,则打印匹配的位置。
2、使用字符串方法find()
Python字符串方法find()
可以用来查找子字符串在字符串中的位置。通过在循环中使用它,可以找到所有出现的匹配项。
def find_text_with_find(text, target):
index = text.find(target)
while index != -1:
print(f"Found '{target}' at position {index}")
index = text.find(target, index + 1)
示例
text = "Python is amazing. Python is versatile. Python is easy to learn."
target = "Python"
find_text_with_find(text, target)
在这个示例中,我们使用find()
方法查找目标文本第一次出现的位置,并在循环中继续查找下一次出现的位置,直到找不到为止。
二、使用while循环
除了for循环,while循环也是一种有效的方法来循环查找某个文本。以下是实现此方法的详细步骤和示例代码:
1、使用字符串方法find()与while循环
和上面的方法类似,我们可以将字符串方法find()
和while循环结合起来使用。
def find_text_with_while(text, target):
index = 0
while index < len(text):
index = text.find(target, index)
if index == -1:
break
print(f"Found '{target}' at position {index}")
index += len(target) # Move past the current match
示例
text = "Python is amazing. Python is versatile. Python is easy to learn."
target = "Python"
find_text_with_while(text, target)
在这个示例中,我们使用while循环和find()
方法来查找每个匹配的位置,并在找到匹配后,更新索引以继续查找下一个匹配。
2、使用字符串方法index()与while循环
字符串方法index()
类似于find()
,但在找不到匹配时会抛出异常。我们可以使用try-except结构来处理这个情况。
def find_text_with_while_index(text, target):
index = 0
while index < len(text):
try:
index = text.index(target, index)
print(f"Found '{target}' at position {index}")
index += len(target)
except ValueError:
break
示例
text = "Python is amazing. Python is versatile. Python is easy to learn."
target = "Python"
find_text_with_while_index(text, target)
在这个示例中,我们使用index()
方法查找每个匹配的位置,并在找不到匹配时通过捕获异常来终止循环。
三、使用正则表达式
正则表达式是一种强大的工具,用于匹配复杂模式。Python的re
模块提供了丰富的正则表达式操作功能。以下是使用正则表达式查找文本的详细步骤和示例代码:
1、使用re.finditer()
re.finditer()
返回一个迭代器,可以生成所有匹配对象。
import re
def find_all_occurrences(text, pattern):
matches = re.finditer(pattern, text)
for match in matches:
print(f"Found {match.group()} at {match.start()}-{match.end()}")
示例
text = "Python is amazing. Python is versatile. Python is easy to learn."
pattern = r"Python"
find_all_occurrences(text, pattern)
在这个示例中,我们使用re.finditer()
查找所有的匹配,并在循环中打印每个匹配的文本及其位置。
2、使用re.findall()
re.findall()
返回所有匹配的文本,虽然它不能直接提供匹配位置,但我们可以结合其他方法来获得位置。
import re
def find_all_occurrences_with_findall(text, pattern):
matches = re.findall(pattern, text)
index = 0
for match in matches:
index = text.find(match, index)
print(f"Found {match} at position {index}")
index += len(match)
示例
text = "Python is amazing. Python is versatile. Python is easy to learn."
pattern = r"Python"
find_all_occurrences_with_findall(text, pattern)
在这个示例中,我们首先使用re.findall()
获取所有匹配的文本,然后使用字符串方法find()
找到每个匹配的位置。
3、使用re.search()与while循环
re.search()
可以在循环中逐步查找匹配项。
import re
def find_all_occurrences_with_search(text, pattern):
index = 0
while index < len(text):
match = re.search(pattern, text[index:])
if not match:
break
start = index + match.start()
end = index + match.end()
print(f"Found {match.group()} at {start}-{end}")
index = end
示例
text = "Python is amazing. Python is versatile. Python is easy to learn."
pattern = r"Python"
find_all_occurrences_with_search(text, pattern)
在这个示例中,我们使用re.search()
在循环中逐步查找匹配项,并在找到匹配后更新索引以继续查找下一个匹配。
四、综合实例应用
为了更好地理解和应用上述方法,我们可以结合实际情况,创建一个综合的实例应用。假设我们需要在一个大型文本文件中查找特定的关键词,并统计其出现的频率和位置。
1、读取文件内容
首先,我们需要读取文件内容。可以使用Python内置的文件操作函数来完成这一任务。
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
return file.read()
示例
file_path = 'example.txt'
text = read_file(file_path)
2、查找关键词并统计频率和位置
接下来,我们使用前面介绍的方法之一来查找关键词,并统计其出现的频率和位置。这里我们选择使用正则表达式。
import re
def find_keyword_statistics(text, keyword):
pattern = re.compile(re.escape(keyword))
matches = pattern.finditer(text)
occurrences = 0
positions = []
for match in matches:
occurrences += 1
positions.append((match.start(), match.end()))
return occurrences, positions
示例
keyword = 'Python'
occurrences, positions = find_keyword_statistics(text, keyword)
print(f"Keyword '{keyword}' found {occurrences} times at positions {positions}")
在这个示例中,我们使用re.compile()
编译正则表达式,并使用finditer()
查找所有的匹配项。然后,我们统计匹配的次数并记录每个匹配的位置。
3、输出结果
最后,我们将统计结果输出到屏幕或写入文件。
def output_statistics(file_path, keyword, occurrences, positions):
with open(file_path, 'w', encoding='utf-8') as file:
file.write(f"Keyword '{keyword}' found {occurrences} times.\n")
file.write("Positions:\n")
for start, end in positions:
file.write(f"Start: {start}, End: {end}\n")
示例
output_file_path = 'output.txt'
output_statistics(output_file_path, keyword, occurrences, positions)
在这个示例中,我们将统计结果写入到一个输出文件中,包括关键词的出现次数和每个匹配的位置。
五、总结
在Python中,有多种方法可以用来循环查找某个文本,包括使用for循环、while循环和正则表达式。每种方法都有其优点和适用场景:
- for循环适用于简单的文本查找任务,尤其是当我们希望逐字符遍历字符串时。
- while循环提供了更灵活的控制结构,适用于需要动态更新索引的位置查找。
- 正则表达式是最强大的工具,适用于复杂模式的匹配和查找任务。
通过结合实际情况选择合适的方法,可以有效地解决各种文本查找问题。在实际应用中,还可以将这些方法与文件操作、数据统计等功能结合起来,实现更复杂的文本处理任务。
相关问答FAQs:
如何在Python中实现循环查找文本的功能?
在Python中,可以使用for
循环结合字符串的方法来查找文本。可以使用str.find()
或str.index()
方法来获取文本在目标字符串中的位置。例如,可以使用一个循环来查找某个文本在给定字符串中的所有出现位置。示例代码如下:
text = "这是一个示例文本。示例文本包含多个示例。"
keyword = "示例"
positions = [i for i in range(len(text)) if text.startswith(keyword, i)]
print(positions) # 输出所有出现"示例"的起始索引
这种方法灵活且易于理解。
在Python中查找文本时,如何处理大小写问题?
如果需要进行不区分大小写的查找,可以使用str.lower()
或str.upper()
方法将目标字符串和要查找的文本统一为小写或大写。例如:
text = "这是一个示例文本。示例文本包含多个示例。"
keyword = "示例"
text_lower = text.lower()
keyword_lower = keyword.lower()
positions = [i for i in range(len(text_lower)) if text_lower.startswith(keyword_lower, i)]
print(positions)
这样可以确保在查找时不会因为大小写不同而漏掉匹配的文本。
有没有推荐的Python库可以简化文本查找的过程?
当然,可以考虑使用re
库,它提供了更强大的正则表达式功能来进行复杂的文本查找。例如,可以使用re.findall()
来查找所有匹配的文本:
import re
text = "这是一个示例文本。示例文本包含多个示例。"
keyword = "示例"
matches = re.findall(keyword, text)
print(matches) # 输出所有匹配的"示例"
使用正则表达式可以满足更多样化的查找需求,如查找模式匹配等。
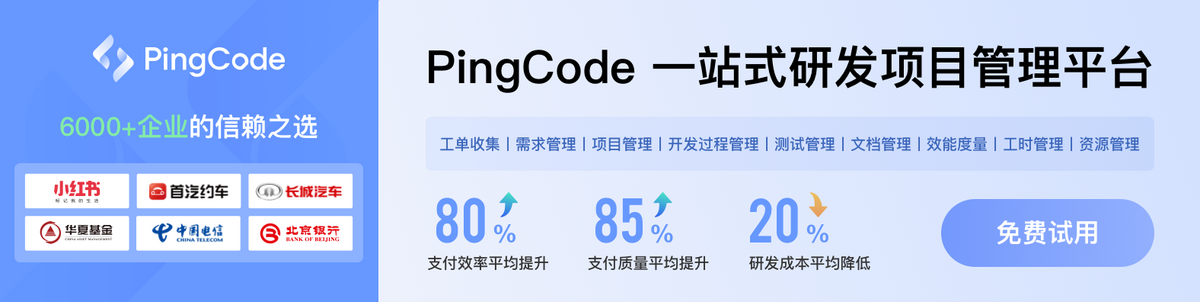