要获取文件的编码,可以使用以下几种方法:chardet、cchardet、open()函数中的encoding参数、文件头的BOM(字节顺序标记)。其中,chardet 是一个 Python 库,用于检测文件的编码,它既支持文本文件也支持二进制文件。下面详细介绍其中一种方法:使用chardet库。
使用chardet库:首先需要安装chardet库,使用命令 pip install chardet
。然后可以通过以下代码来检测文件编码:
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
encoding = result['encoding']
return encoding
file_path = 'example.txt'
encoding = detect_encoding(file_path)
print(f'The encoding of the file is: {encoding}')
chardet库的优点是使用简单,支持多种编码检测,返回结果包含编码类型和置信度。chardet 使用统计和机器学习算法来检测文件编码,通常能够提供较为准确的检测结果。
一、安装和使用chardet库
chardet 是一个用于检测文本编码的第三方库,通过统计分析来推测文本的编码类型。首先需要安装 chardet 库,可以使用以下命令:
pip install chardet
安装完成后,可以使用 chardet 来检测文件的编码。下面是一个简单的示例:
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
encoding = result['encoding']
confidence = result['confidence']
return encoding, confidence
file_path = 'example.txt'
encoding, confidence = detect_encoding(file_path)
print(f'The encoding of the file is: {encoding}, with confidence: {confidence}')
这段代码读取文件的原始字节数据,然后使用 chardet.detect 方法来检测其编码。返回的结果包含编码类型和置信度。
二、使用cchardet库
cchardet 是 chardet 的一个高性能替代品,基于 C++ 编写,因此在性能上有显著提升。首先需要安装 cchardet 库:
pip install cchardet
使用 cchardet 库的代码与 chardet 类似:
import cchardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = cchardet.detect(raw_data)
encoding = result['encoding']
confidence = result['confidence']
return encoding, confidence
file_path = 'example.txt'
encoding, confidence = detect_encoding(file_path)
print(f'The encoding of the file is: {encoding}, with confidence: {confidence}')
cchardet 的使用方法与 chardet 非常类似,但在处理大文件或大量文件时,cchardet 的性能更佳。
三、使用open()函数中的encoding参数
在 Python 中,open()
函数可以指定文件的编码。虽然这不是直接检测文件编码的方法,但在已知文件编码的情况下,可以使用该参数来正确读取文件:
file_path = 'example.txt'
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content)
如果文件的编码未知,可以结合 chardet 或 cchardet 检测结果来指定编码:
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
return result['encoding']
file_path = 'example.txt'
encoding = detect_encoding(file_path)
with open(file_path, 'r', encoding=encoding) as file:
content = file.read()
print(content)
这样可以确保文件内容被正确读取并解码。
四、文件头的BOM(字节顺序标记)
有些文件在开头会包含一个 BOM(字节顺序标记),用于指示文件的编码。常见的 BOM 包括 UTF-8、UTF-16LE、UTF-16BE 等。可以通过读取文件的前几个字节来检测 BOM 并判断编码:
def detect_bom(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read(4)
if raw_data.startswith(b'\xff\xfe\x00\x00') or raw_data.startswith(b'\x00\x00\xfe\xff'):
return 'UTF-32'
elif raw_data.startswith(b'\xff\xfe') or raw_data.startswith(b'\xfe\xff'):
return 'UTF-16'
elif raw_data.startswith(b'\xef\xbb\xbf'):
return 'UTF-8'
else:
return None
file_path = 'example.txt'
encoding = detect_bom(file_path)
if encoding:
print(f'The file has a BOM indicating the encoding is: {encoding}')
else:
print('No BOM found, encoding is unknown')
这种方法可以快速判断文件的编码,但仅适用于包含 BOM 的文件。
五、结合多种方法提高准确性
在实际应用中,可以结合多种方法来提高文件编码检测的准确性。例如,先通过 BOM 检测编码,如果未检测到 BOM,再使用 chardet 或 cchardet 进行进一步检测:
import chardet
def detect_bom(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read(4)
if raw_data.startswith(b'\xff\xfe\x00\x00') or raw_data.startswith(b'\x00\x00\xfe\xff'):
return 'UTF-32'
elif raw_data.startswith(b'\xff\xfe') or raw_data.startswith(b'\xfe\xff'):
return 'UTF-16'
elif raw_data.startswith(b'\xef\xbb\xbf'):
return 'UTF-8'
else:
return None
def detect_encoding(file_path):
encoding = detect_bom(file_path)
if encoding:
return encoding
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
return result['encoding']
file_path = 'example.txt'
encoding = detect_encoding(file_path)
print(f'The encoding of the file is: {encoding}')
通过结合多种方法,可以提高文件编码检测的准确性和可靠性。
六、应用场景和注意事项
1. 多语言文本处理
在处理多语言文本时,正确识别文件编码是非常重要的。不同语言的文本可能使用不同的编码,例如 UTF-8、GBK、Shift_JIS 等。使用 chardet 或 cchardet 可以有效检测文件的编码,确保文本被正确读取和处理。
2. 大文件处理
在处理大文件时,cchardet 的性能优势尤为明显。cchardet 使用 C++ 编写,性能较 chardet 更佳,适合在需要高效处理大文件的场景中使用。
3. 文件编码转换
在某些情况下,可能需要将文件从一种编码转换为另一种编码。例如,将 GBK 编码的文件转换为 UTF-8 编码:
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
return result['encoding']
def convert_encoding(input_file, output_file, target_encoding='utf-8'):
source_encoding = detect_encoding(input_file)
with open(input_file, 'r', encoding=source_encoding) as file:
content = file.read()
with open(output_file, 'w', encoding=target_encoding) as file:
file.write(content)
input_file = 'example_gbk.txt'
output_file = 'example_utf8.txt'
convert_encoding(input_file, output_file)
这种方法可以确保文件内容在不同编码之间转换时保持一致性。
4. 数据分析和处理
在数据分析和处理过程中,可能会遇到来自不同来源的文本数据。这些数据可能使用不同的编码格式,正确检测和处理这些编码对于数据分析的准确性至关重要。使用 chardet 或 cchardet 可以有效解决这一问题。
5. 网络爬虫
在编写网络爬虫时,爬取的网页内容可能使用不同的编码格式。通过检测网页内容的编码,可以确保爬取的数据被正确解码和处理:
import requests
import chardet
def fetch_url_content(url):
response = requests.get(url)
raw_data = response.content
result = chardet.detect(raw_data)
encoding = result['encoding']
content = raw_data.decode(encoding)
return content
url = 'https://example.com'
content = fetch_url_content(url)
print(content)
通过检测网页内容的编码,可以确保爬取的数据被正确解码,避免乱码问题。
七、常见问题和解决方案
1. 检测结果不准确
在某些情况下,chardet 或 cchardet 的检测结果可能不够准确。这通常是由于文本数据较少或数据本身包含多种编码格式。可以尝试提供更多的文本数据进行检测,或者结合其他方法(如 BOM 检测)提高准确性。
2. 文件中包含特殊字符
文件中包含特殊字符(如控制字符)可能会影响编码检测的准确性。可以尝试清理或过滤这些特殊字符,然后重新进行编码检测。
3. 多种编码格式混合
在处理包含多种编码格式的文件时,单一的编码检测方法可能无法准确识别所有编码。可以考虑将文件按段落或行分割,分别进行编码检测和处理。
4. 性能问题
在处理大量文件或大文件时,编码检测可能会成为性能瓶颈。可以考虑使用性能更高的 cchardet 库,或者在多线程或多进程环境中进行编码检测,以提高处理效率。
八、总结
本文介绍了多种获取文件编码的方法,包括 chardet、cchardet、open()
函数中的 encoding
参数以及文件头的 BOM(字节顺序标记)。通过结合多种方法,可以提高文件编码检测的准确性和可靠性。在实际应用中,正确识别文件编码对于多语言文本处理、大文件处理、文件编码转换、数据分析和处理以及网络爬虫等场景具有重要意义。
无论是使用 chardet 还是 cchardet,都可以通过简单的代码实现文件编码检测,并结合其他方法和技巧,解决实际应用中的各种问题。希望本文的内容能够帮助读者更好地理解和应用文件编码检测技术。
相关问答FAQs:
如何判断一个文件的编码格式?
可以使用Python中的chardet
库来判断文件的编码格式。通过读取文件的一部分内容,chardet
能够分析并返回最可能的编码类型。示例代码如下:
import chardet
with open('yourfile.txt', 'rb') as f:
rawdata = f.read(10000) # 读取文件的前10000个字节
result = chardet.detect(rawdata)
print(result['encoding'])
在Python中如何处理不同编码的文件?
处理不同编码的文件需要在打开文件时指定正确的编码格式。若不知道编码,可以先使用chardet
库获取编码,然后用该编码打开文件。示例代码如下:
import chardet
with open('yourfile.txt', 'rb') as f:
rawdata = f.read(10000)
result = chardet.detect(rawdata)
encoding = result['encoding']
with open('yourfile.txt', 'r', encoding=encoding) as f:
content = f.read()
print(content)
使用Python读取文件时如何处理编码错误?
在读取文件时,可以通过设置errors
参数来处理编码错误。常用的选项包括ignore
(忽略错误)和replace
(用替代字符替换错误)。例如:
with open('yourfile.txt', 'r', encoding='utf-8', errors='ignore') as f:
content = f.read()
print(content)
这种方式能够避免因编码错误导致的程序崩溃,同时尽可能保留可读内容。
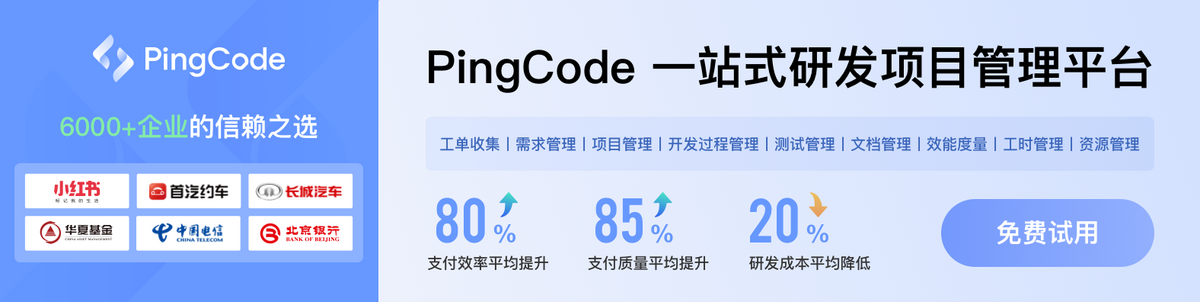