Python进程人为阻塞的方法有很多,如使用time.sleep()
、threading.Event
、threading.Lock
、queue.Queue
等。 其中一种常见的方法是使用time.sleep()
,它可以使当前线程暂停执行指定的时间(以秒为单位)。例如,在多线程编程中,我们可以使用threading.Event
来实现线程间的同步,或者使用threading.Lock
来避免多个线程同时访问共享资源。下面我们将详细介绍这些方法的原理和使用方式。
一、time.sleep()
time.sleep()
是Python标准库中的一个函数,它能够使当前线程暂停执行一段时间。这在需要人为阻塞进程时非常有用,尤其是在模拟延迟、控制执行频率等场景下。
使用方法
import time
print("Start")
time.sleep(5) # 阻塞5秒
print("End")
在这个例子中,程序会在输出“Start”后暂停5秒钟,然后再输出“End”。这种方法非常简单,但只能用于短时间的阻塞,因为长时间的time.sleep
会导致程序无法响应其他事件。
二、threading.Event
threading.Event
是Python的threading
模块提供的一个用于线程间通信的同步原语。通过设置和清除事件标志,线程可以等待某个事件的发生或通知其他线程事件的发生。
使用方法
import threading
event = threading.Event()
def worker():
print("Worker waiting for event...")
event.wait() # 阻塞,等待事件被触发
print("Worker received event!")
创建并启动线程
thread = threading.Thread(target=worker)
thread.start()
print("Main thread sleeping for 5 seconds...")
time.sleep(5)
print("Main thread setting event.")
event.set() # 触发事件
在这个例子中,工作线程会等待事件的发生,主线程在5秒后触发事件,工作线程继续执行。这种方法适用于多线程程序中需要线程间同步的场景。
三、threading.Lock
threading.Lock
是Python的标准库threading
模块提供的另一种同步原语,用于保护共享资源,防止多个线程同时访问造成数据不一致。
使用方法
import threading
lock = threading.Lock()
shared_resource = 0
def worker():
global shared_resource
print("Worker trying to acquire lock...")
with lock:
print("Worker acquired lock.")
shared_resource += 1
time.sleep(5)
print("Worker released lock.")
print("Worker finished.")
创建并启动多个线程
threads = [threading.Thread(target=worker) for _ in range(3)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
print(f"Shared resource value: {shared_resource}")
在这个例子中,多个线程尝试访问共享资源时会先尝试获取锁,只有获得锁的线程才能修改共享资源。其他线程会被阻塞,直到锁被释放。这种方法适用于需要保护共享资源的多线程程序。
四、queue.Queue
queue.Queue
是Python标准库queue
模块提供的一个线程安全的队列,常用于线程间的任务调度和通信。通过队列的阻塞操作,可以实现线程的同步。
使用方法
import queue
import threading
q = queue.Queue()
def producer():
for i in range(5):
print(f"Producing item {i}")
q.put(i)
time.sleep(1)
def consumer():
while True:
item = q.get()
if item is None:
break
print(f"Consuming item {item}")
q.task_done()
创建并启动生产者和消费者线程
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
producer_thread.start()
consumer_thread.start()
producer_thread.join()
q.put(None) # 发送信号结束消费者线程
consumer_thread.join()
在这个例子中,生产者线程向队列中放入项目,消费者线程从队列中取出项目进行处理。队列的阻塞操作确保了生产者和消费者之间的同步。
五、threading.Condition
threading.Condition
是Python的threading
模块提供的另一种同步原语,用于实现复杂的线程间同步。通过条件变量,可以实现线程间的等待和通知机制。
使用方法
import threading
condition = threading.Condition()
shared_resource = 0
def producer():
global shared_resource
with condition:
for i in range(5):
shared_resource += 1
print(f"Producing item {shared_resource}")
condition.notify()
condition.wait()
def consumer():
global shared_resource
with condition:
while shared_resource < 5:
condition.wait()
print(f"Consuming item {shared_resource}")
condition.notify()
创建并启动生产者和消费者线程
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
producer_thread.start()
consumer_thread.start()
producer_thread.join()
consumer_thread.join()
在这个例子中,生产者线程和消费者线程通过条件变量进行同步,生产者线程在生产一个项目后通知消费者线程,消费者线程在消费一个项目后通知生产者线程。这种方法适用于需要复杂同步机制的多线程程序。
六、multiprocessing.Event
multiprocessing.Event
是Python的multiprocessing
模块提供的一个用于进程间通信的同步原语,类似于threading.Event
,但用于多进程环境。
使用方法
import multiprocessing
import time
event = multiprocessing.Event()
def worker():
print("Worker waiting for event...")
event.wait() # 阻塞,等待事件被触发
print("Worker received event!")
创建并启动进程
process = multiprocessing.Process(target=worker)
process.start()
print("Main process sleeping for 5 seconds...")
time.sleep(5)
print("Main process setting event.")
event.set() # 触发事件
process.join()
在这个例子中,工作进程会等待事件的发生,主进程在5秒后触发事件,工作进程继续执行。这种方法适用于多进程程序中需要进程间同步的场景。
七、multiprocessing.Queue
multiprocessing.Queue
是Python标准库multiprocessing
模块提供的一个线程安全的队列,常用于进程间的任务调度和通信。通过队列的阻塞操作,可以实现进程的同步。
使用方法
import multiprocessing
q = multiprocessing.Queue()
def producer():
for i in range(5):
print(f"Producing item {i}")
q.put(i)
time.sleep(1)
def consumer():
while True:
item = q.get()
if item is None:
break
print(f"Consuming item {item}")
创建并启动生产者和消费者进程
producer_process = multiprocessing.Process(target=producer)
consumer_process = multiprocessing.Process(target=consumer)
producer_process.start()
consumer_process.start()
producer_process.join()
q.put(None) # 发送信号结束消费者进程
consumer_process.join()
在这个例子中,生产者进程向队列中放入项目,消费者进程从队列中取出项目进行处理。队列的阻塞操作确保了生产者和消费者之间的同步。
八、asyncio
asyncio
是Python 3.4引入的一个标准库,提供了支持异步I/O、事件循环、协程和任务的基础设施。通过await
关键字,可以在协程中实现阻塞操作。
使用方法
import asyncio
async def worker():
print("Worker waiting for event...")
await asyncio.sleep(5) # 阻塞5秒
print("Worker received event!")
async def main():
print("Main coroutine starting worker...")
await worker()
print("Main coroutine finished.")
运行主协程
asyncio.run(main())
在这个例子中,协程worker
会等待5秒后继续执行,主协程等待worker
完成后继续执行。这种方法适用于异步编程场景。
九、socket
阻塞
socket
编程中,默认的socket
操作是阻塞的,即在等待数据发送或接收时,进程会被阻塞。
使用方法
import socket
def server():
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind(('localhost', 12345))
s.listen(1)
print("Server waiting for connection...")
conn, addr = s.accept() # 阻塞,等待连接
print("Server accepted connection from", addr)
conn.close()
s.close()
def client():
time.sleep(1) # 等待服务器启动
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect(('localhost', 12345))
print("Client connected to server")
s.close()
创建并启动服务器和客户端线程
server_thread = threading.Thread(target=server)
client_thread = threading.Thread(target=client)
server_thread.start()
client_thread.start()
server_thread.join()
client_thread.join()
在这个例子中,服务器线程会在accept
调用时阻塞,直到有客户端连接,客户端线程连接后,服务器线程继续执行。这种方法适用于网络编程场景。
十、select
select
模块提供了一个基于I/O多路复用的机制,可以同时监控多个文件描述符的状态,实现非阻塞的I/O操作。
使用方法
import select
import socket
def server():
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind(('localhost', 12345))
s.listen(1)
inputs = [s]
print("Server waiting for connection...")
while inputs:
readable, _, _ = select.select(inputs, [], [])
for sock in readable:
if sock is s:
conn, addr = s.accept()
print("Server accepted connection from", addr)
inputs.append(conn)
else:
data = sock.recv(1024)
if not data:
inputs.remove(sock)
sock.close()
def client():
time.sleep(1) # 等待服务器启动
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect(('localhost', 12345))
print("Client connected to server")
s.close()
创建并启动服务器和客户端线程
server_thread = threading.Thread(target=server)
client_thread = threading.Thread(target=client)
server_thread.start()
client_thread.start()
server_thread.join()
client_thread.join()
在这个例子中,服务器线程使用select
来监控多个文件描述符,实现非阻塞的I/O操作。这种方法适用于需要同时处理多个I/O事件的网络编程场景。
十一、multiprocessing.Condition
multiprocessing.Condition
是Python的multiprocessing
模块提供的一个用于进程间同步的条件变量,通过条件变量,可以实现进程间的等待和通知机制。
使用方法
import multiprocessing
condition = multiprocessing.Condition()
shared_resource = 0
def producer():
global shared_resource
with condition:
for i in range(5):
shared_resource += 1
print(f"Producing item {shared_resource}")
condition.notify()
condition.wait()
def consumer():
global shared_resource
with condition:
while shared_resource < 5:
condition.wait()
print(f"Consuming item {shared_resource}")
condition.notify()
创建并启动生产者和消费者进程
producer_process = multiprocessing.Process(target=producer)
consumer_process = multiprocessing.Process(target=consumer)
producer_process.start()
consumer_process.start()
producer_process.join()
consumer_process.join()
在这个例子中,生产者进程和消费者进程通过条件变量进行同步,生产者进程在生产一个项目后通知消费者进程,消费者进程在消费一个项目后通知生产者进程。这种方法适用于需要复杂同步机制的多进程程序。
十二、multiprocessing.Semaphore
multiprocessing.Semaphore
是Python的multiprocessing
模块提供的一个用于进程间同步的信号量,通过信号量,可以控制同时访问共享资源的进程数量。
使用方法
import multiprocessing
semaphore = multiprocessing.Semaphore(2)
shared_resource = 0
def worker():
global shared_resource
with semaphore:
shared_resource += 1
print(f"Worker incremented shared resource to {shared_resource}")
time.sleep(5)
shared_resource -= 1
print(f"Worker decremented shared resource to {shared_resource}")
创建并启动多个进程
processes = [multiprocessing.Process(target=worker) for _ in range(5)]
for process in processes:
process.start()
for process in processes:
process.join()
在这个例子中,多个进程尝试访问共享资源时会先尝试获取信号量,只有获得信号量的进程才能访问共享资源。其他进程会被阻塞,直到信号量被释放。这种方法适用于需要控制并发访问共享资源数量的多进程程序。
总结
以上介绍了多种人为阻塞Python进程的方法,包括time.sleep()
、threading.Event
、threading.Lock
、queue.Queue
、threading.Condition
、multiprocessing.Event
、multiprocessing.Queue
、asyncio
、socket
阻塞、select
、multiprocessing.Condition
和multiprocessing.Semaphore
。每种方法都有其适用的场景和特点,开发者可以根据具体需求选择合适的阻塞方法来实现进程或线程的同步和控制。
相关问答FAQs:
如何在Python中实现进程阻塞?
在Python中,可以通过多种方式实现进程阻塞。最常见的方法是使用time.sleep()
函数,该函数可以暂停当前进程指定的时间。除此之外,还可以使用锁(如threading.Lock()
)来阻塞一个进程直到获得锁。使用这些方法可以帮助管理多进程之间的同步和资源共享。
什么情况下需要人为阻塞Python进程?
人为阻塞进程通常在需要控制并发行为、避免资源争用或确保数据一致性时使用。例如,在处理文件操作或数据库事务时,可能需要阻塞其他进程以防止数据损坏。此外,当一个进程需要等待某个条件或事件发生时,阻塞也是一种有效的手段。
在Python中使用队列如何实现进程阻塞?
使用queue.Queue()
可以轻松实现进程间的通信和阻塞。当队列为空时,调用get()
方法会阻塞当前进程,直到有数据可用。这种机制非常适合生产者-消费者模式,确保数据的安全传输和处理。同时,队列还提供了线程安全的特性,使得多进程环境下的阻塞操作更加可靠。
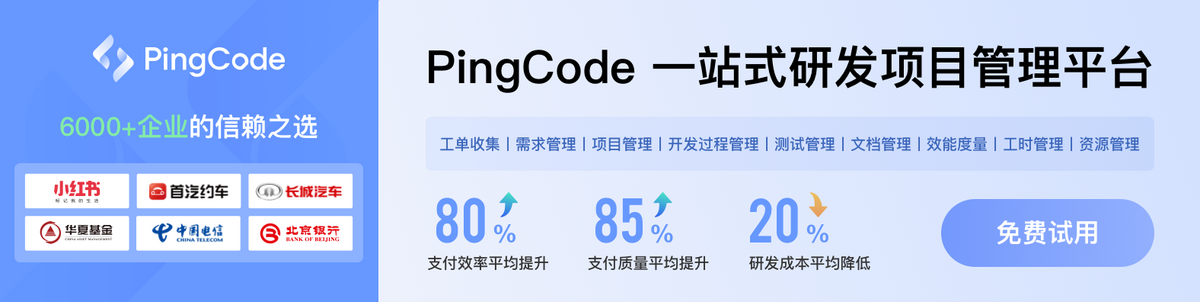