Python写日志文件的方法包括使用内置的logging模块、配置日志记录器、设置日志记录的级别、格式化日志消息等。、通过logging配置文件进行配置、使用第三方日志库如loguru。其中,使用内置的logging模块是最常见和推荐的方法。下面将详细介绍其中一种方法,即使用logging模块的详细步骤和注意事项。
一、配置logging模块
Python的logging模块是一个功能强大的日志记录工具,提供了多个日志记录级别和灵活的配置选项。以下是使用logging模块的基本步骤:
1、导入logging模块
首先需要导入logging模块:
import logging
2、创建日志记录器
接下来,创建一个日志记录器(logger),用于记录日志信息:
logger = logging.getLogger(__name__)
3、设置日志记录级别
日志记录级别决定了哪些日志信息会被记录,常见的日志级别包括DEBUG、INFO、WARNING、ERROR、CRITICAL:
logger.setLevel(logging.DEBUG)
4、创建日志处理器
日志处理器(handler)决定了日志信息的输出方式,可以输出到控制台、文件等。这里创建一个文件处理器,将日志信息输出到文件:
file_handler = logging.FileHandler('app.log')
5、设置日志格式
日志格式(formatter)决定了日志信息的格式,这里定义一个简单的格式:
formatter = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s')
file_handler.setFormatter(formatter)
6、将处理器添加到日志记录器
将配置好的文件处理器添加到日志记录器中:
logger.addHandler(file_handler)
7、记录日志信息
最后,可以使用日志记录器记录日志信息:
logger.debug('This is a debug message')
logger.info('This is an info message')
logger.warning('This is a warning message')
logger.error('This is an error message')
logger.critical('This is a critical message')
二、使用logging配置文件
除了在代码中进行配置,logging模块还支持通过配置文件进行配置。使用配置文件可以更方便地管理和修改日志设置。以下是一个logging配置文件的示例:
1、创建配置文件
创建一个名为logging.conf的配置文件,内容如下:
[loggers]
keys=root,simpleExample
[handlers]
keys=consoleHandler,fileHandler
[formatters]
keys=defaultFormatter
[logger_root]
level=DEBUG
handlers=consoleHandler
[logger_simpleExample]
level=DEBUG
handlers=consoleHandler,fileHandler
qualname=simpleExample
propagate=0
[handler_consoleHandler]
class=StreamHandler
level=DEBUG
formatter=defaultFormatter
args=(sys.stdout,)
[handler_fileHandler]
class=FileHandler
level=DEBUG
formatter=defaultFormatter
args=('app.log', 'a')
[formatter_defaultFormatter]
format=%(asctime)s - %(name)s - %(levelname)s - %(message)s
2、加载配置文件
在代码中加载配置文件并进行日志记录:
import logging
import logging.config
logging.config.fileConfig('logging.conf')
logger = logging.getLogger('simpleExample')
logger.debug('This is a debug message')
logger.info('This is an info message')
logger.warning('This is a warning message')
logger.error('This is an error message')
logger.critical('This is a critical message')
三、使用第三方日志库loguru
loguru是一个第三方日志库,提供了更简洁的API和更多的功能。以下是使用loguru记录日志的示例:
1、安装loguru
首先,需要安装loguru库:
pip install loguru
2、导入loguru
在代码中导入loguru并进行日志记录:
from loguru import logger
logger.add('app.log', format="{time} {level} {message}", level="DEBUG")
logger.debug('This is a debug message')
logger.info('This is an info message')
logger.warning('This is a warning message')
logger.error('This is an error message')
logger.critical('This is a critical message')
四、日志轮转与归档
在实际应用中,日志文件可能会变得非常大,因此需要进行日志轮转和归档。logging模块的RotatingFileHandler和TimedRotatingFileHandler可以实现日志轮转。
1、使用RotatingFileHandler
RotatingFileHandler根据文件大小进行日志轮转:
import logging
from logging.handlers import RotatingFileHandler
logger = logging.getLogger(__name__)
logger.setLevel(logging.DEBUG)
file_handler = RotatingFileHandler('app.log', maxBytes=2000, backupCount=5)
formatter = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s')
file_handler.setFormatter(formatter)
logger.addHandler(file_handler)
logger.debug('This is a debug message')
logger.info('This is an info message')
logger.warning('This is a warning message')
logger.error('This is an error message')
logger.critical('This is a critical message')
2、使用TimedRotatingFileHandler
TimedRotatingFileHandler根据时间进行日志轮转:
import logging
from logging.handlers import TimedRotatingFileHandler
logger = logging.getLogger(__name__)
logger.setLevel(logging.DEBUG)
file_handler = TimedRotatingFileHandler('app.log', when='midnight', interval=1, backupCount=7)
formatter = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s')
file_handler.setFormatter(formatter)
logger.addHandler(file_handler)
logger.debug('This is a debug message')
logger.info('This is an info message')
logger.warning('This is a warning message')
logger.error('This is an error message')
logger.critical('This is a critical message')
五、总结
通过本文的介绍,了解了Python写日志文件的多种方法,包括使用内置的logging模块、通过配置文件进行配置、使用第三方日志库loguru等。推荐使用内置的logging模块,因为其功能强大且易于配置。使用配置文件可以更方便地管理日志设置,而第三方日志库loguru提供了更简洁的API。此外,还介绍了日志轮转与归档的实现方法,以避免日志文件过大。掌握这些方法,可以更有效地进行日志记录和管理。
相关问答FAQs:
如何在Python中配置日志记录的基本设置?
在Python中,可以使用内置的logging
模块来配置日志记录的基本设置。使用logging.basicConfig()
方法可以定义日志的级别、格式和输出位置。比如,可以设置日志级别为DEBUG,这样所有级别的日志信息都会被记录。你还可以指定输出到控制台或文件,通过filename
参数来指定日志文件的位置。
Python的日志记录中,如何自定义日志格式?
自定义日志格式主要通过在logging.basicConfig()
中使用format
参数来实现。你可以定义需要记录的信息,比如时间戳、日志级别、消息等。常见的格式示例为'%(asctime)s - %(levelname)s - %(message)s'
,这会将时间、日志级别和消息内容一起记录到日志文件中。
如何在Python中记录不同级别的日志信息?
Python的logging
模块支持多种日志级别,包括DEBUG、INFO、WARNING、ERROR和CRITICAL。根据重要性不同,使用不同的级别来记录信息。例如,使用logging.debug()
记录调试信息,使用logging.error()
记录错误信息。通过调整日志级别,可以控制输出到日志文件中的信息量。
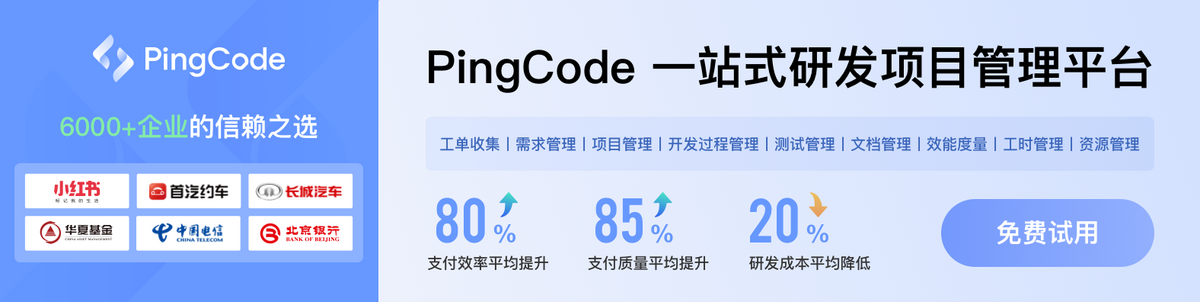