用Python写账户密码管理系统时,可以通过使用标准库和第三方库来创建一个安全、易用的系统。核心步骤包括创建用户注册、登录功能、密码加密与验证、账户数据存储等。 其中,密码加密是确保账户密码安全的关键步骤。详细来说,密码加密可以通过Python的hashlib
库或bcrypt
库来实现。下面将详细展开如何实现密码加密功能。
一、账户密码管理系统概述
账户密码管理系统通常包括以下几个部分:
- 用户注册
- 用户登录
- 密码加密与验证
- 数据存储
这些部分共同构成了一个完整的系统,确保用户的账户信息安全、操作简便。
二、用户注册
用户注册是账户密码管理系统的第一步,用户需要提供用户名和密码进行注册。注册过程中,我们需要对用户输入的信息进行验证,并将密码加密后存储。
1. 用户输入验证
在用户注册时,需要验证输入的用户名和密码是否符合规范,例如用户名是否已经存在,密码是否符合安全要求(长度、复杂度等)。
import re
def validate_username(username):
# 检查用户名是否已经存在
# 这里假设我们有一个函数 user_exists(username) 来检查用户名是否存在
if user_exists(username):
return False, "Username already exists"
return True, "Username is valid"
def validate_password(password):
# 检查密码长度和复杂度
if len(password) < 8:
return False, "Password must be at least 8 characters long"
if not re.search(r"[A-Za-z]", password) or not re.search(r"[0-9]", password):
return False, "Password must contain both letters and numbers"
return True, "Password is valid"
2. 密码加密
密码加密是确保用户密码安全的关键步骤。我们可以使用hashlib
库或bcrypt
库来实现。
import hashlib
def hash_password(password):
return hashlib.sha256(password.encode()).hexdigest()
使用bcrypt
库可以更安全地加密密码,因为它提供了更强的加密算法和内置的盐值处理。
import bcrypt
def hash_password(password):
salt = bcrypt.gensalt()
hashed = bcrypt.hashpw(password.encode(), salt)
return hashed
3. 存储用户信息
在用户注册成功后,需要将用户名和加密后的密码存储到数据库中。这里我们使用一个简单的字典来模拟数据库。
users_db = {}
def register_user(username, password):
valid_username, msg = validate_username(username)
if not valid_username:
return False, msg
valid_password, msg = validate_password(password)
if not valid_password:
return False, msg
hashed_password = hash_password(password)
users_db[username] = hashed_password
return True, "User registered successfully"
三、用户登录
用户登录时,需要验证用户名和密码是否正确。首先检查用户名是否存在,然后验证密码是否正确。
def check_password(stored_password, provided_password):
return bcrypt.checkpw(provided_password.encode(), stored_password)
def login_user(username, password):
if username not in users_db:
return False, "Username does not exist"
stored_password = users_db[username]
if not check_password(stored_password, password):
return False, "Incorrect password"
return True, "User logged in successfully"
四、数据存储
在实际应用中,我们会使用数据库(如SQLite、MySQL、PostgreSQL等)来存储用户信息。以下是使用SQLite存储用户信息的示例。
import sqlite3
def init_db():
conn = sqlite3.connect('users.db')
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS users
(username TEXT PRIMARY KEY, password TEXT)''')
conn.commit()
conn.close()
def store_user_in_db(username, password):
conn = sqlite3.connect('users.db')
cursor = conn.cursor()
cursor.execute('INSERT INTO users (username, password) VALUES (?, ?)', (username, password))
conn.commit()
conn.close()
def user_exists_in_db(username):
conn = sqlite3.connect('users.db')
cursor = conn.cursor()
cursor.execute('SELECT * FROM users WHERE username = ?', (username,))
user = cursor.fetchone()
conn.close()
return user is not None
def get_user_password_from_db(username):
conn = sqlite3.connect('users.db')
cursor = conn.cursor()
cursor.execute('SELECT password FROM users WHERE username = ?', (username,))
password = cursor.fetchone()
conn.close()
return password[0] if password else None
将数据存储部分结合到注册和登录功能中:
def validate_username(username):
if user_exists_in_db(username):
return False, "Username already exists"
return True, "Username is valid"
def register_user(username, password):
valid_username, msg = validate_username(username)
if not valid_username:
return False, msg
valid_password, msg = validate_password(password)
if not valid_password:
return False, msg
hashed_password = hash_password(password)
store_user_in_db(username, hashed_password)
return True, "User registered successfully"
def login_user(username, password):
if not user_exists_in_db(username):
return False, "Username does not exist"
stored_password = get_user_password_from_db(username)
if not check_password(stored_password, password):
return False, "Incorrect password"
return True, "User logged in successfully"
五、密码重置
密码重置是账户密码管理系统的重要功能。用户可以通过提供用户名和一些验证信息来重置密码。
def reset_password(username, new_password):
if not user_exists_in_db(username):
return False, "Username does not exist"
valid_password, msg = validate_password(new_password)
if not valid_password:
return False, msg
hashed_password = hash_password(new_password)
conn = sqlite3.connect('users.db')
cursor = conn.cursor()
cursor.execute('UPDATE users SET password = ? WHERE username = ?', (hashed_password, username))
conn.commit()
conn.close()
return True, "Password reset successfully"
六、总结
通过以上步骤,我们实现了一个基本的账户密码管理系统,包括用户注册、登录、密码加密与验证、数据存储和密码重置功能。这个系统可以进一步扩展,例如添加多因素认证、账户锁定等功能,以提高系统的安全性和用户体验。
注意事项:
- 密码加密:务必使用强加密算法,推荐使用
bcrypt
等库。 - 数据存储:实际应用中建议使用专业的数据库系统,如MySQL、PostgreSQL等。
- 安全性:确保系统的各个环节都符合安全规范,保护用户信息。
通过这些步骤和注意事项,您可以构建一个安全、可靠的账户密码管理系统。
相关问答FAQs:
如何用Python实现账户密码的存储和验证?
在Python中,可以通过字典或数据库来存储账户密码。使用字典时,可以将用户名作为键,密码作为值。为了提高安全性,建议对密码进行哈希处理,例如使用hashlib
库中的sha256
方法。验证时,只需将输入密码进行哈希处理并与存储的哈希值进行比较即可。
存储账户密码时有哪些安全措施?
在存储账户密码时,采用加盐(salt)技术是非常重要的。加盐可以在密码哈希之前添加随机数据,这样即使两个用户的密码相同,存储后的哈希值也会不同。此外,使用强加密算法(如bcrypt或argon2)来存储密码也是一种良好的实践,可以有效防止暴力破解。
如何处理用户忘记密码的情况?
处理用户忘记密码时,可以提供一个重置密码的功能。用户输入注册时的邮箱地址后,系统可以生成一个唯一的重置链接,并发送到用户邮箱。用户点击链接后,可以创建新的密码。在这个过程中,确保链接的有效性和时间限制,以提高安全性,避免恶意用户的利用。
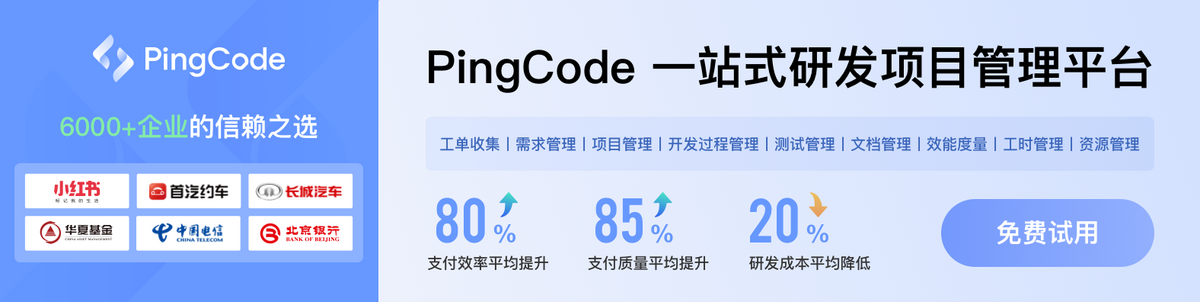