Python处理多行输入可以通过以下几种方式:使用循环读取、使用多行字符串、使用输入函数等。循环读取、使用多行字符串、使用输入函数。其中,循环读取是一种常见且灵活的处理多行输入的方法。
循环读取是指使用for
循环或while
循环来读取多行输入,直到满足某个条件为止。通常,我们会使用input()
函数来逐行读取用户输入,并使用一个空行或特定的标志来结束输入。例如:
lines = []
while True:
line = input("Enter a line (or leave empty to finish): ")
if line:
lines.append(line)
else:
break
print("You entered:")
for line in lines:
print(line)
这种方法的优点是简单易用,适用于大多数需要处理多行输入的场景。此外,还可以根据需要对输入进行预处理或过滤。
接下来,我们将详细介绍Python处理多行输入的几种方法,并探讨它们的优缺点和适用场景。
一、循环读取
1、使用while
循环
使用while
循环来读取多行输入是一种常见的方法。通常,我们会使用一个空行或特定的标志来结束输入。
lines = []
while True:
line = input("Enter a line (or leave empty to finish): ")
if line:
lines.append(line)
else:
break
print("You entered:")
for line in lines:
print(line)
这种方法的优点是简单易用,适用于大多数需要处理多行输入的场景。此外,还可以根据需要对输入进行预处理或过滤。
2、使用for
循环
使用for
循环也可以实现多行输入的读取,通常需要指定一个固定的行数。
n = int(input("Enter the number of lines: "))
lines = []
for _ in range(n):
line = input()
lines.append(line)
print("You entered:")
for line in lines:
print(line)
这种方法适用于已知输入行数的情况,可以确保读取到固定数量的行。
二、使用多行字符串
多行字符串(Triple-quoted strings)是一种方便的方式来处理多行输入,特别是在脚本中直接嵌入多行文本时。
multi_line_string = """This is the first line.
This is the second line.
And this is the third line."""
lines = multi_line_string.splitlines()
print("You entered:")
for line in lines:
print(line)
这种方法的优点是简洁直观,适用于处理固定内容的多行输入。然而,对于动态输入的场景,这种方法并不适用。
三、使用输入函数
1、sys.stdin.read()
在某些情况下,我们可以使用sys.stdin.read()
来读取标准输入中的所有内容,并将其处理为多行输入。
import sys
print("Enter your input (Ctrl+D to finish):")
input_data = sys.stdin.read()
lines = input_data.splitlines()
print("You entered:")
for line in lines:
print(line)
这种方法适用于需要从标准输入读取大块文本的情况,例如从文件重定向输入或通过管道传输数据。
2、fileinput.input()
fileinput
模块提供了一种方便的方式来处理文件输入和标准输入,可以用于多行输入的处理。
import fileinput
print("Enter your input (Ctrl+D to finish):")
lines = []
for line in fileinput.input():
lines.append(line.strip())
print("You entered:")
for line in lines:
print(line)
这种方法适用于处理文件输入和标准输入的混合场景,具有较高的灵活性。
四、使用列表推导式
列表推导式是一种简洁的方式来处理多行输入,特别是对于已知行数的情况。
n = int(input("Enter the number of lines: "))
lines = [input() for _ in range(n)]
print("You entered:")
for line in lines:
print(line)
这种方法简洁明了,适用于处理已知行数的多行输入。
五、处理多行输入的高级技巧
1、输入预处理
在处理多行输入时,可能需要对输入进行预处理,例如去除空白字符、过滤无效行等。
lines = []
while True:
line = input("Enter a line (or leave empty to finish): ").strip()
if line:
lines.append(line)
else:
break
print("You entered:")
for line in lines:
print(line)
这种预处理可以提高输入数据的质量,减少后续处理的复杂度。
2、使用正则表达式
使用正则表达式可以对多行输入进行更加灵活的处理,例如匹配特定格式的行、提取信息等。
import re
lines = []
while True:
line = input("Enter a line (or leave empty to finish): ")
if line:
if re.match(r'^\d{4}-\d{2}-\d{2}$', line): # Example: match date format
lines.append(line)
else:
print("Invalid format, please enter a date (YYYY-MM-DD).")
else:
break
print("You entered:")
for line in lines:
print(line)
这种方法适用于需要对输入格式进行严格控制的场景。
六、处理大规模多行输入
1、使用生成器
对于大规模多行输入,使用生成器可以避免将所有输入一次性加载到内存中,从而提高效率。
def read_lines():
while True:
line = input("Enter a line (or leave empty to finish): ")
if line:
yield line
else:
break
lines = list(read_lines())
print("You entered:")
for line in lines:
print(line)
这种方法适用于处理大规模数据的场景,可以有效节省内存。
2、批量处理
对于需要批量处理的多行输入,可以将输入分批次读取和处理,从而提高处理效率。
batch_size = 5
lines = []
while True:
batch = []
for _ in range(batch_size):
line = input("Enter a line (or leave empty to finish): ")
if line:
batch.append(line)
else:
break
if batch:
lines.extend(batch)
else:
break
print("You entered:")
for line in lines:
print(line)
这种方法适用于需要分批处理大规模数据的场景,可以提高处理效率。
七、总结
Python提供了多种方式来处理多行输入,包括循环读取、多行字符串、输入函数等。不同的方法适用于不同的场景和需求,可以根据具体情况选择合适的方法进行处理。循环读取、多行字符串、输入函数等方法在处理多行输入时各有优缺点,可以根据实际需求进行选择和组合使用。此外,通过输入预处理、使用正则表达式、生成器和批量处理等高级技巧,可以进一步提高多行输入处理的效率和灵活性。
相关问答FAQs:
如何在Python中读取多行输入?
在Python中,可以使用input()
函数结合循环来处理多行输入。用户可以在输入完每一行后按下回车键,直到输入特定的结束符号(例如“exit”)为止。示例代码如下:
lines = []
while True:
line = input("请输入一行文本(输入'exit'结束):")
if line == 'exit':
break
lines.append(line)
print("您输入的内容是:", lines)
如何将多行输入存储在列表中?
在处理多行输入时,可以将每一行的内容存储在列表中,这样方便后续的处理和访问。上面的示例代码演示了如何使用append()
方法将每一行添加到列表中,最终可以通过索引访问或遍历列表中的所有输入。
如何处理多行输入的文件?
若要处理来自文件的多行输入,可以使用open()
函数读取文件内容。文件的每一行可以通过readlines()
方法获取,并将其存储在一个列表中。例如:
with open('input.txt', 'r') as file:
lines = file.readlines()
lines = [line.strip() for line in lines] # 去除每行的换行符
print("文件中的内容是:", lines)
这种方式适合于需要处理大量数据或预先定义的输入时使用。
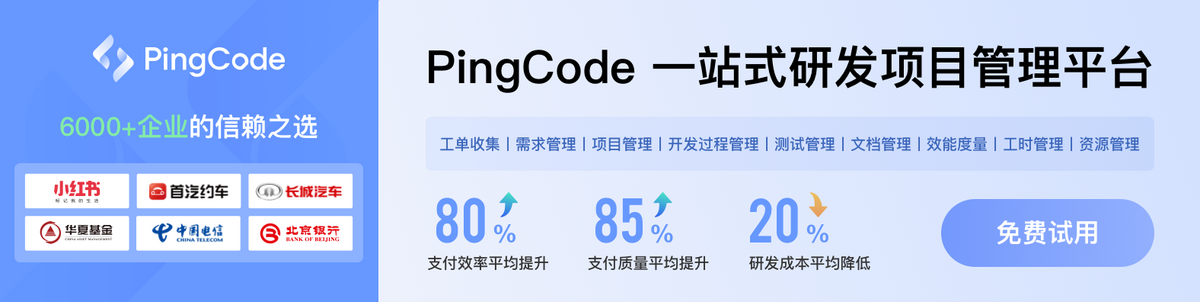