在Python中计算OD矩阵(Origin-Destination Matrix,起点-终点矩阵)可以使用多种方法和库。常见的方法包括使用Pandas、Numpy、Geopandas、NetworkX、OSMNX等库进行数据处理和分析。其中,Pandas用于处理表格数据,Numpy用于数组操作,Geopandas用于地理数据处理,NetworkX和OSMNX用于网络分析。其中,通过获取地理位置数据、构建网络图、计算最短路径等步骤,可以得到OD矩阵。以下是具体步骤的详细描述。
一、数据准备
在计算OD矩阵之前,我们需要准备一些数据。这些数据通常包括起点和终点的位置坐标。可以从多个来源获取这些数据,例如CSV文件、数据库或API。
1、读取位置数据
首先,读取包含起点和终点位置的CSV文件。假设文件包含如下信息:
origin_lat,origin_lon,destination_lat,destination_lon
34.052235,-118.243683,36.778259,-119.417931
33.748997,-84.387985,34.052235,-118.243683
...
使用Pandas读取CSV文件:
import pandas as pd
读取位置数据
df = pd.read_csv('locations.csv')
二、构建网络图
使用OSMNX库从OpenStreetMap获取道路网络,然后使用NetworkX构建网络图。这样,我们可以利用这些库的功能来计算最短路径。
1、安装OSMNX和NetworkX
pip install osmnx networkx
2、获取道路网络
使用OSMNX从OpenStreetMap获取道路网络。假设我们关注某个城市的道路网络:
import osmnx as ox
获取城市道路网络
city = 'Los Angeles, California, USA'
G = ox.graph_from_place(city, network_type='drive')
三、计算最短路径
使用NetworkX计算起点和终点之间的最短路径。可以使用地理坐标将位置映射到网络图中的最近节点。
1、定义函数获取最近节点
import networkx as nx
def get_nearest_node(G, lat, lon):
point = (lat, lon)
return ox.distance.nearest_nodes(G, lon, lat)
2、计算OD矩阵
使用Pandas DataFrame存储OD矩阵,并计算每对起点和终点之间的最短路径:
import numpy as np
初始化OD矩阵
od_matrix = np.zeros((len(df), len(df)))
计算最短路径
for i, row in df.iterrows():
origin_node = get_nearest_node(G, row['origin_lat'], row['origin_lon'])
destination_node = get_nearest_node(G, row['destination_lat'], row['destination_lon'])
try:
length = nx.shortest_path_length(G, origin_node, destination_node, weight='length')
except nx.NetworkXNoPath:
length = np.inf # 如果没有路径,则设置为无穷大
od_matrix[i, i] = length
转换为DataFrame
od_matrix_df = pd.DataFrame(od_matrix, columns=df.index, index=df.index)
四、保存结果
将计算得到的OD矩阵保存为CSV文件,便于后续分析:
od_matrix_df.to_csv('od_matrix.csv')
五、优化计算
对于大型数据集,计算最短路径可能会非常耗时。可以考虑以下优化方法:
1、使用并行计算
使用多线程或多进程来加速计算。Python的concurrent.futures
模块可以方便地实现并行计算:
from concurrent.futures import ThreadPoolExecutor
def compute_shortest_path(row):
origin_node = get_nearest_node(G, row['origin_lat'], row['origin_lon'])
destination_node = get_nearest_node(G, row['destination_lat'], row['destination_lon'])
try:
return nx.shortest_path_length(G, origin_node, destination_node, weight='length')
except nx.NetworkXNoPath:
return np.inf
with ThreadPoolExecutor(max_workers=4) as executor:
results = list(executor.map(compute_shortest_path, [row for _, row in df.iterrows()]))
更新OD矩阵
for i, length in enumerate(results):
od_matrix[i, i] = length
2、减少计算量
如果起点和终点位置较为集中,可以先将位置进行聚类(例如K-means聚类),然后计算聚类中心之间的最短路径。这样可以减少计算量,提高效率。
from sklearn.cluster import KMeans
聚类
kmeans = KMeans(n_clusters=10)
df['cluster'] = kmeans.fit_predict(df[['origin_lat', 'origin_lon']])
计算聚类中心之间的最短路径
cluster_centers = kmeans.cluster_centers_
cluster_od_matrix = np.zeros((len(cluster_centers), len(cluster_centers)))
for i, (lat1, lon1) in enumerate(cluster_centers):
for j, (lat2, lon2) in enumerate(cluster_centers):
if i != j:
origin_node = get_nearest_node(G, lat1, lon1)
destination_node = get_nearest_node(G, lat2, lon2)
try:
length = nx.shortest_path_length(G, origin_node, destination_node, weight='length')
except nx.NetworkXNoPath:
length = np.inf
cluster_od_matrix[i, j] = length
更新原始OD矩阵
for i, row in df.iterrows():
cluster = row['cluster']
for j, dest_row in df.iterrows():
dest_cluster = dest_row['cluster']
od_matrix[i, j] = cluster_od_matrix[cluster, dest_cluster]
六、可视化分析
最后,可以使用Geopandas和Matplotlib对OD矩阵进行可视化分析,以便更好地理解结果。
1、安装Geopandas和Matplotlib
pip install geopandas matplotlib
2、绘制OD矩阵热力图
import matplotlib.pyplot as plt
import seaborn as sns
绘制热力图
plt.figure(figsize=(10, 8))
sns.heatmap(od_matrix, cmap='viridis')
plt.title('OD Matrix Heatmap')
plt.xlabel('Destination')
plt.ylabel('Origin')
plt.show()
3、绘制路径图
可以在地图上绘制起点和终点之间的路径,直观地展示最短路径。使用Geopandas和Matplotlib来实现:
import geopandas as gpd
from shapely.geometry import LineString
创建GeoDataFrame
gdf = gpd.GeoDataFrame(columns=['geometry'])
for i, row in df.iterrows():
origin_node = get_nearest_node(G, row['origin_lat'], row['origin_lon'])
destination_node = get_nearest_node(G, row['destination_lat'], row['destination_lon'])
try:
path = nx.shortest_path(G, origin_node, destination_node, weight='length')
path_coords = [(G.nodes[node]['x'], G.nodes[node]['y']) for node in path]
line = LineString(path_coords)
gdf = gdf.append({'geometry': line}, ignore_index=True)
except nx.NetworkXNoPath:
continue
绘制路径图
fig, ax = plt.subplots(figsize=(10, 10))
ox.plot_graph(ox.project_graph(G), ax=ax, show=False, close=False)
gdf.plot(ax=ax, edgecolor='red')
plt.show()
通过以上步骤,可以在Python中计算OD矩阵,并对结果进行可视化分析。从数据准备、网络构建、路径计算到结果保存和优化,每一步都有详细的描述和示例代码。这种方法适用于各种规模的数据集,并且可以根据需要进行优化和扩展。
相关问答FAQs:
1. 什么是OD矩阵,它在交通分析中有什么重要性?
OD矩阵(Origin-Destination Matrix)是用于表示从一个地点(起点)到另一个地点(终点)交通流量的工具。它在交通分析和规划中至关重要,因为它帮助交通工程师了解人们的出行模式、交通需求和潜在的拥堵点。通过分析OD矩阵,城市规划者可以更有效地设计公共交通系统、优化道路布局以及提升交通效率。
2. 使用Python计算OD矩阵需要哪些库和工具?
计算OD矩阵通常需要一些数据处理和分析的库,如Pandas和NumPy。Pandas可以帮助处理数据框,进行数据清洗和转换,而NumPy则提供强大的数组和矩阵操作功能。此外,使用Matplotlib或Seaborn进行可视化展示也是个不错的选择,能够更直观地展示OD矩阵的结果。
3. 如何获取OD矩阵所需的数据?
获取OD矩阵所需的数据可以通过多种方式。常见的方法包括使用交通调查数据、GPS轨迹数据或移动电话数据等。许多城市交通管理部门会发布相关数据集,此外,开源平台如OpenStreetMap也提供了交通流量相关的信息。确保数据的准确性和代表性是计算OD矩阵的关键步骤。
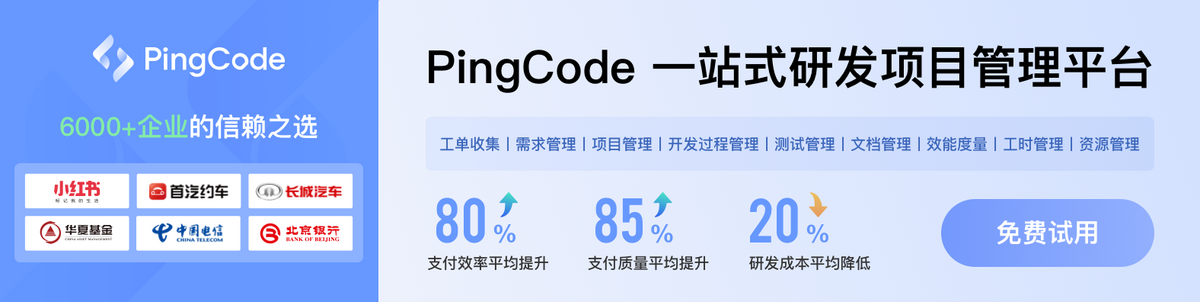