在Python中写脚本,首先需要了解基本的编程概念、掌握Python的语法、使用适当的编辑器或IDE、以及了解常用的库和工具。
以下是一些关键步骤:
1. 学习基础语法、2. 选择合适的编辑器或IDE、3. 编写并运行脚本、4. 使用库和模块、5. 调试和优化代码
首先,学习基础语法是写脚本的第一步。Python是一种高级编程语言,它的语法简洁明了,适合初学者。了解变量、数据类型、控制结构(如循环和条件语句)、函数和类的定义和使用,是写脚本的基础。
例如,编写一个简单的Python脚本,可以从以下步骤开始:
- 安装Python:从Python官方网站下载并安装Python解释器。
- 选择编辑器:选择一个文本编辑器或IDE,如VS Code、PyCharm、Sublime Text等。
- 编写代码:在编辑器中编写Python代码。
- 运行脚本:在命令行或编辑器中运行脚本,查看输出。
接下来,我们将详细介绍在Python中写脚本的各个方面。
一、学习基础语法
Python的基础语法是编写脚本的前提,下面我们将介绍一些常见的语法和概念。
变量和数据类型
在Python中,变量不需要显式声明类型,可以直接赋值。例如:
x = 10
name = "Alice"
is_active = True
常见的数据类型包括整数(int)、浮点数(float)、字符串(str)、布尔值(bool)等。
控制结构
控制结构包括条件语句和循环语句,用于控制程序的执行流程。
条件语句:
age = 18
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
循环语句:
# for loop
for i in range(5):
print(i)
while loop
count = 0
while count < 5:
print(count)
count += 1
函数和类
函数和类是Python中重要的结构,用于组织和封装代码。
函数:
def greet(name):
return f"Hello, {name}!"
message = greet("Alice")
print(message)
类:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def display_info(self):
print(f"Name: {self.name}, Age: {self.age}")
person = Person("Alice", 30)
person.display_info()
二、选择合适的编辑器或IDE
选择一个合适的编辑器或IDE可以提高编写脚本的效率。以下是一些常用的编辑器和IDE:
VS Code
Visual Studio Code(VS Code)是一款免费的开源编辑器,支持多种编程语言,包括Python。它具有丰富的扩展和插件,可以提高编写代码的效率。
PyCharm
PyCharm是一款专业的Python IDE,提供了强大的代码分析、调试、测试和重构功能。PyCharm分为社区版(免费)和专业版(收费)。
Sublime Text
Sublime Text是一款轻量级的文本编辑器,支持多种编程语言,具有强大的插件系统,可以通过安装插件支持Python编程。
三、编写并运行脚本
编写Python脚本时,可以在编辑器或IDE中编写代码,并保存为以.py
为扩展名的文件。例如,创建一个名为hello.py
的文件,内容如下:
print("Hello, world!")
运行脚本
可以通过命令行或编辑器中的运行按钮来运行脚本。在命令行中,使用以下命令运行脚本:
python hello.py
四、使用库和模块
Python的强大之处在于其丰富的库和模块,可以大大简化编程任务。以下是一些常用的库和模块:
标准库
Python的标准库包含了许多常用的模块,例如:
os
:提供操作系统相关的功能。sys
:提供与Python解释器相关的功能。datetime
:提供日期和时间处理功能。math
:提供数学计算功能。
使用标准库时,可以通过import
语句导入模块。例如:
import os
import sys
import datetime
import math
print(os.getcwd()) # 获取当前工作目录
print(sys.version) # 获取Python版本
print(datetime.datetime.now()) # 获取当前日期和时间
print(math.sqrt(16)) # 计算平方根
第三方库
除了标准库,Python还有丰富的第三方库,可以通过包管理工具pip
安装。例如,安装和使用requests
库来发送HTTP请求:
pip install requests
import requests
response = requests.get("https://api.github.com")
print(response.status_code)
print(response.json())
五、调试和优化代码
在编写脚本的过程中,调试和优化代码是必不可少的步骤。以下是一些常用的方法和工具:
调试
调试是发现和修复代码错误的过程。常用的调试方法包括:
- 打印调试:通过
print
语句打印变量值和程序状态。 - 断点调试:使用编辑器或IDE中的调试工具设置断点,逐步执行代码,查看变量值和程序状态。
- 日志记录:使用
logging
模块记录日志信息,便于追踪和分析程序运行过程。
例如,使用logging
模块记录日志:
import logging
logging.basicConfig(level=logging.DEBUG, format="%(asctime)s - %(levelname)s - %(message)s")
logging.debug("This is a debug message.")
logging.info("This is an info message.")
logging.warning("This is a warning message.")
logging.error("This is an error message.")
logging.critical("This is a critical message.")
优化
优化代码可以提高程序的性能和效率。常用的优化方法包括:
- 算法优化:选择高效的算法和数据结构,减少时间复杂度和空间复杂度。
- 代码优化:减少不必要的计算和操作,使用高效的编程技巧。
- 性能分析:使用性能分析工具,如
cProfile
、timeit
等,分析程序的性能瓶颈,进行针对性优化。
例如,使用timeit
模块测量代码执行时间:
import timeit
def test():
return [i for i in range(10000)]
execution_time = timeit.timeit(test, number=1000)
print(f"Execution time: {execution_time} seconds")
六、实战示例
通过一个具体的示例,我们将展示如何从头开始编写一个Python脚本,包括学习基础语法、选择编辑器、编写代码、使用库和模块、调试和优化代码的全过程。
项目背景
我们将编写一个简单的天气查询脚本,使用requests
库从天气API获取天气信息,并显示在终端。
步骤一:安装Python和编辑器
- 从Python官方网站下载并安装Python。
- 下载并安装VS Code编辑器。
步骤二:创建项目文件
在VS Code中创建一个新文件夹,命名为weather_app
。在该文件夹中创建一个名为weather.py
的文件。
步骤三:编写代码
在weather.py
文件中编写代码,查询并显示天气信息。
import requests
def get_weather(city):
api_key = "your_api_key" # 替换为你的API密钥
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}&units=metric"
response = requests.get(url)
if response.status_code == 200:
weather_data = response.json()
return weather_data
else:
return None
def display_weather(weather_data):
if weather_data:
city = weather_data["name"]
temp = weather_data["main"]["temp"]
description = weather_data["weather"][0]["description"]
print(f"Weather in {city}:")
print(f"Temperature: {temp}°C")
print(f"Description: {description}")
else:
print("Failed to retrieve weather data.")
def main():
city = input("Enter city name: ")
weather_data = get_weather(city)
display_weather(weather_data)
if __name__ == "__main__":
main()
步骤四:运行脚本
在命令行中运行脚本:
python weather.py
输入城市名称,例如"London",查看天气信息。
步骤五:调试和优化
使用logging
模块记录日志信息,便于调试和分析:
import requests
import logging
logging.basicConfig(level=logging.DEBUG, format="%(asctime)s - %(levelname)s - %(message)s")
def get_weather(city):
api_key = "your_api_key" # 替换为你的API密钥
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}&units=metric"
response = requests.get(url)
logging.debug(f"Request URL: {url}")
if response.status_code == 200:
weather_data = response.json()
logging.debug(f"Weather data: {weather_data}")
return weather_data
else:
logging.error(f"Failed to retrieve weather data. Status code: {response.status_code}")
return None
def display_weather(weather_data):
if weather_data:
city = weather_data["name"]
temp = weather_data["main"]["temp"]
description = weather_data["weather"][0]["description"]
logging.info(f"Weather in {city}: Temperature: {temp}°C, Description: {description}")
print(f"Weather in {city}:")
print(f"Temperature: {temp}°C")
print(f"Description: {description}")
else:
logging.warning("No weather data to display.")
print("Failed to retrieve weather data.")
def main():
city = input("Enter city name: ")
weather_data = get_weather(city)
display_weather(weather_data)
if __name__ == "__main__":
main()
步骤六:性能优化
优化代码,提高性能。例如,缓存查询结果,减少API请求次数:
import requests
import logging
from functools import lru_cache
logging.basicConfig(level=logging.DEBUG, format="%(asctime)s - %(levelname)s - %(message)s")
@lru_cache(maxsize=10)
def get_weather(city):
api_key = "your_api_key" # 替换为你的API密钥
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}&units=metric"
response = requests.get(url)
logging.debug(f"Request URL: {url}")
if response.status_code == 200:
weather_data = response.json()
logging.debug(f"Weather data: {weather_data}")
return weather_data
else:
logging.error(f"Failed to retrieve weather data. Status code: {response.status_code}")
return None
def display_weather(weather_data):
if weather_data:
city = weather_data["name"]
temp = weather_data["main"]["temp"]
description = weather_data["weather"][0]["description"]
logging.info(f"Weather in {city}: Temperature: {temp}°C, Description: {description}")
print(f"Weather in {city}:")
print(f"Temperature: {temp}°C")
print(f"Description: {description}")
else:
logging.warning("No weather data to display.")
print("Failed to retrieve weather data.")
def main():
city = input("Enter city name: ")
weather_data = get_weather(city)
display_weather(weather_data)
if __name__ == "__main__":
main()
通过以上步骤,我们完成了一个简单的天气查询脚本的编写、调试和优化过程。希望通过这个示例,您能够更好地理解如何在Python中写脚本。
相关问答FAQs:
在Python中,如何创建一个新的脚本文件?
要创建一个新的Python脚本文件,您可以使用任何文本编辑器(如Notepad、VSCode或PyCharm)。只需打开编辑器,输入您的Python代码,然后将文件保存为以“.py”结尾的文件名,例如“myscript.py”。确保在保存时选择“所有文件”格式,以避免文本编辑器自动添加其他扩展名。
Python脚本的基本结构是什么样的?
Python脚本通常由一系列的语句和函数组成。基本结构包括导入所需的库(使用import语句)、定义函数(使用def关键字)、编写主要逻辑以及使用条件语句和循环来控制程序的流程。一个简单的示例脚本可能如下所示:
import math
def calculate_area(radius):
return math.pi * radius ** 2
if __name__ == "__main__":
r = 5
print(f"Area of circle with radius {r} is {calculate_area(r)}")
如何在命令行中运行Python脚本?
要在命令行中运行Python脚本,您需要打开命令提示符或终端,导航到脚本所在的目录。使用cd
命令改变目录后,输入python yourscript.py
(将“yourscript.py”替换为您的脚本文件名)并按回车键。如果您的Python安装正确,脚本将会执行并显示输出结果。
如何在Python脚本中处理错误和异常?
在Python脚本中,您可以使用try-except语句来处理潜在的错误和异常。通过这种方式,您可以确保程序在遇到错误时不会崩溃,而是可以优雅地处理错误。例如:
try:
result = 10 / 0
except ZeroDivisionError:
print("Error: You cannot divide by zero!")
这种方法使得用户可以更好地理解程序的运行状态,同时也能提供更友好的错误提示。
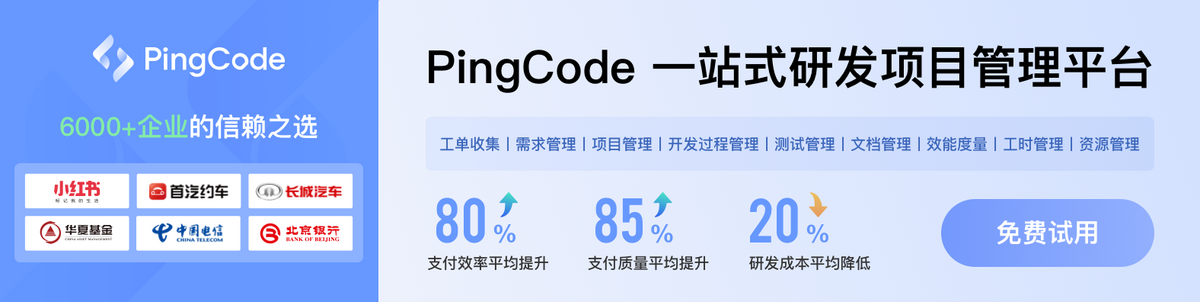